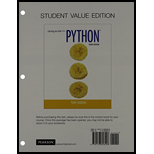
Student Value Edition for Starting Out with Python (3rd Edition)
3rd Edition
ISBN: 9780133848496
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 7, Problem 9PE
Program Plan Intro
Population data
Program plan:
- • Declare a function named “main()”. Inside the function,
- ○ Use the “try…except” block to read the population from the file.
- ○ Create a file object “fileObj” for the file “USPopulation.txt”
- ○ Now, read the populations from the file and store it in “populationList” and close the file.
- ○ Use a “for” loop to convert the populations into “float” type.
- ○ Create an empty list “populationChange” to hold the changes in the population.
- ○ Now, use the “for” loop to find the population change for the successive years and store it in “populationChange”.
- ○ Call the function “max()” to find the greatest population change.
- ○ Call the function “index()” to find the position of the greatest population change.
- ○ Call the function “min()” to find the lowest population change.
- ○ Call the function “index()” to find the position of the lowest population change.
- ○ Then, call the function “sum()” to find the sum of the population changes in the “populationChange”.
- ○ Find the average annual change.
- ○ Now, print the average annual change, greatest population change year, and lowest population change year using the “print()” function.
- ○ Handle the exceptions if any occurred using the “except” blocks.
- • In the last, call the function “main()” to execute the program.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
If you have downloaded the source code from this book’s companion Web site, you will find a file named USPopulation.txt in the Chapter 07 folder. The file contains the midyear population of the United States, in thousands, during the years 1950 through 1990. The first line in the file contains the population for 1950, the second line contains the population for 1951, and so forth.Write a program that reads the file’s contents into a list. The program should display the following data:• The average annual change in population during the time period• The year with the greatest increase in population during the time period• The year with the smallest increase in population during the time period(You can access the book’s companion Web site at www.pearsonglobaleditions.com/gaddis.)
The attached file USPopulation,txt contains the US population in thousands, during the years 1950 through 1990. The first line in the file contains the population for 1950, the second line contains the population for 1951, and so forth.
Write a program that reads the file's contents into a list. The program should display the following data:
the average annual growth in population during the time period
the year with the greatest increase in population during the time period (highest growth rate)
the year with the smallest increase in population during the time period (smallest growth rate)
151868 153982 156393 158956 161884 165069 168088 171187 174149 177135 179979 182992 185771 188483 191141 193526 195576 197457 199399 201385 203984 206827 209284 211357 213342 215465 217563 219760 222095 224567 227225 229466 231664 233792 235825 237924 240133 242289 244499 246819 249623
Laboratory Exercise No. 40 Filename: Lab_exer40 You are tasked to create a program that will get the basic salary of 5 employees from the user and these 5 values should be saved in a list named as basicSalary. The user will then enter the incentive rate (Example. 0.15) The program should compute the net salary. The net salary is computed using the formula basic salary multiplied by incentive rate. Store the computed net salary in another list which will be named as net_salary. Display the values stored in the 2 lists. Example Output: Enter basic salary : 200 Enter basic salary : 300 Enter basic salary : 450 Enter basic salary : 600 Enter basic salary : 750 Enter incentive rate : 0.50 Basic salary [200,300,450,600,750] Net Salary [100,150,225,300,375]
Chapter 7 Solutions
Student Value Edition for Starting Out with Python (3rd Edition)
Ch. 7.2 - What will the following code display? numbers =...Ch. 7.2 - Prob. 2CPCh. 7.2 - Prob. 3CPCh. 7.2 - Prob. 4CPCh. 7.2 - Prob. 5CPCh. 7.2 - Prob. 6CPCh. 7.2 - Prob. 7CPCh. 7.2 - Prob. 8CPCh. 7.3 - Prob. 9CPCh. 7.3 - Prob. 10CP
Ch. 7.3 - Prob. 11CPCh. 7.3 - Prob. 12CPCh. 7.3 - Prob. 13CPCh. 7.4 - What will the following code display? names =...Ch. 7.5 - Prob. 15CPCh. 7.5 - Prob. 16CPCh. 7.5 - Prob. 17CPCh. 7.5 - Prob. 18CPCh. 7.8 - Prob. 19CPCh. 7.8 - Prob. 20CPCh. 7.8 - Write a set of nested loops that display the...Ch. 7.9 - Prob. 22CPCh. 7.9 - Prob. 23CPCh. 7.9 - Prob. 24CPCh. 7.9 - Prob. 25CPCh. 7 - This term refers to an individual item in a list....Ch. 7 - This is a number that identifies an item in a...Ch. 7 - Prob. 3MCCh. 7 - This is the last index in a list. a. 1 b. 99 c. 0...Ch. 7 - This will happen if you try to use an index that...Ch. 7 - This function returns the length of a list. a....Ch. 7 - When the operator's left operand is a list and...Ch. 7 - This list method adds an item to the end of an...Ch. 7 - This removes an item at a specific index in a...Ch. 7 - Prob. 10MCCh. 7 - If you call the index method to locate an item in...Ch. 7 - Prob. 12MCCh. 7 - This file object method returns a list containing...Ch. 7 - Which of the following statement creates a tuple?...Ch. 7 - Prob. 1TFCh. 7 - Prob. 2TFCh. 7 - Prob. 3TFCh. 7 - Prob. 4TFCh. 7 - A file object's writelines method automatically...Ch. 7 - You can use the + operator to concatenate two...Ch. 7 - Prob. 7TFCh. 7 - You can remove an element from a tuple by calling...Ch. 7 - Prob. 1SACh. 7 - Prob. 2SACh. 7 - What will the following code display? values = [2,...Ch. 7 - Prob. 4SACh. 7 - Prob. 5SACh. 7 - Prob. 6SACh. 7 - Prob. 1AWCh. 7 - Prob. 2AWCh. 7 - Prob. 3AWCh. 7 - Prob. 4AWCh. 7 - Write a function that accepts a list as an...Ch. 7 - Prob. 6AWCh. 7 - Prob. 7AWCh. 7 - Prob. 8AWCh. 7 - Total Sales Design a program that asks the user to...Ch. 7 - Prob. 2PECh. 7 - Rainfall Statistics Design a program that lets the...Ch. 7 - Prob. 4PECh. 7 - Prob. 5PECh. 7 - Larger Than n In a program, write a function that...Ch. 7 - Drivers License Exam The local driver s license...Ch. 7 - Name Search If you have downloaded the source code...Ch. 7 - Prob. 9PECh. 7 - World Series Champions If you have downloaded the...Ch. 7 - Prob. 11PE
Knowledge Booster
Similar questions
- GirlNames.txt This file contains a list of the 200 most popular names given to girls born in the United States from the year 2000 through 2009. BoyNames.txt This file contains a list of the 200 most popular names given to boys born in the United States from the year 2000 through 2009. Write a program that reads the contents of the two files into two separate lists. The user should be able to enter a boy’s name, a girl’s name, or both, and the application will display messages indicating whether the names were among the most popular. **programming language is python** ** must use a def main(): function** *please include some comments* i aslo have the .txt files but no wya to upload themarrow_forward*python coding Driver’s License ExamThe local driver’s license office has asked you to create an application that grades the written portion of thedriver’s license exam. The exam has 20 multiple-choice questions. Hereare the correct answers:1. B 6. A 11. B 16. C2. D 7. B 12. C 17. C3. A 8. A 13. D 18. B4. A 9. C 14. A 19. D5. C 10. D 15. D 20. AYour program should store these correct answers in a list. The program should read thestudent’s answers for each of the 20 questions from a text file and store the answers inanother list. (Create your own text file to test the application.) After the student’s answershave been read from the file, the program should display a message indicating whether thestudent passed or failed the exam. (A student must correctly answer 15 of the 20 questionsto pass the exam.) It should then display the total number of correctly answered questions,the total number of incorrectly answered questions, and a list showing the question numbers of the incorrectly…arrow_forwardPython Program:Your firm has an open position and has received several job applications. The data from the applicants is asfollows (name, followed by their GPA):'John', 3.3, 'Sally', 2.2, 'Bernis', 3.8, 'Fred', 3.2, 'Victoria', 3.4, 'Valerie', 2.6, 'Eric', 2.6Write a program that will print out the highest applicant’s name and their GPA.You can store the data shown above in a list to help process it.arrow_forward
- 6. sum_highest_five This function takes a list of numbers, finds the five largest numbers in the list, and returns their sum. If the list of numbers contains fewer than five elements, raise a ValueError with whatever error message you like. Sample calls should look like: >>> sum_highest_five([10, 10, 10, 10, 10, 5, -17, 2, 3.1])50>>> sum_highest_five([5])Traceback (most recent call last): File "/usr/lib/python3.8/idlelib/run.py", line 559, in runcode exec(code, self.locals) File "<pyshell#44>", line 1, in <module> File "/homework/final.py", line 102, in sum_highest_five raise ValueError("need at least 5 numbers")ValueError: need at least 5 numbersarrow_forwardWord FrequencyWrite a python program that reads the contents of a text file. The program should create a dictio-nary inwhich the keys are the individual words found in the file and the values are the number of timeseach word appears. For example, if the word “the” appears 128 times, the dictionary wouldcontain an element with 'the' as the key and 128 as the value. The program should eitherdisplay the frequency of each word or create a second file containing a list of each word and itsfrequency.arrow_forwardstatistics.py: Write a program that reads a list of integers from the user, until they enter -12345 (the -12345 should not be considered part of the list). Then print the mean, median, and standard deviation of the list. The mean is the average of the numbers. The median is the “middle” value if n is odd, or the average of the two “middle” values if n is even. The standard deviation is the average amount of variability For example: $ python3 statistics.py154102-12345mean: 7.75median: 7standard deviation: 5.909032633745278arrow_forward
- a. The mean of a list of numbers is its arithmetic average. The median of a list is its middle value when the values are placed in order. For example, if a list contains 1, 4, 7, 8, and 10, then the mean is 6 and the median is 7. Write an application that allows you to enter five integers and displays the values, their mean, and their median. Save the files as MeanMedian.java. b. Revise the MeanMedian class so that the user can enter any number of values up to 20. If the list has an even number of values, the median is the numeric average of the values in the two middle positions. Save the file as MeanMedian2.java.arrow_forwardUSE JAVA CODE Represent the features of a basic application using the linked list data structure (menu type). The minimum requirement is that the representation of data must be a list of lists but you may further create another list within the inner list. For instance, the menus of IntelliJ IDEA have different headings (File, Edit, View, Navigate, ...) and under each heading are menu options. Show the CRUD basics (create, read, update, and delete). Some options may have sub-options. In this case, there are 3 levels of nested lists. The outer most list will be the menu headings and under each heading will contain a list of options. An option, in turn, can have a list ofsub-options.arrow_forwardThis file object method returns a list containing the file’s contents.a. to_listb. getlistc. readlined. readlinesarrow_forward
- Phyton: Program 6: Write a program that allows the user to add data from files or by hand. The user may add more data whenever they want. The print also allows the user to display the data and to print the statistics. The data is store in a list. It’s easier if you make the list global so that other functions can access it. You should have the following functions: · add_from_file() – prompts the user for a file and add the data from it. · add_by_hand() – prompts the user to enter data until user type a negative number. · print_stats() – print the stats (min, max, mean, sum). You may use the built-in functions. Handling errors such as whether the input file exist is extra credit. To print a 10-column table, you can use the code below: for i in range(len(data)): print(f"{data[i]:3}", end="") if (i+1)%10==0: print() print() Sample run: Choose an action 1) add data from a file 2) add data by hand 3) print stats 4)…arrow_forwardwrite a c++ code uisng linked list for quiz. In quiz game, questions are chosen in such a a way that they cover all fields of a typical quiz contest. The user’s general knowledge is tested with quiz questions regarding science, technology, movies, sports, general health, geography and many more. • Edit score• Help-- menu • Show record• Show score• Reset score The game ends when the user’s cash prize piles up to 100RS. For each question there are 4 options A, B, C and D and no negative markings. so money would not be deducted for wrong answersto the questions.arrow_forwardUsing introduction to Python You have been asked to write an application that automatically grades a quiz. The quiz has 15 multiple-choice questions. The correct answers are: 1.A, 2.C, 3.A, 4.B, 5.B, 6.D, 7.D, 8.A, 9.C, 10.A, 11.B, 12.C, 13.D, 14.C, 15.B The program will start by creating a list holding the quiz answers key shown above. It will then prompt the user for the student name and their answers file and read them into a separate list. It will finally compare the key vs. answers lists to calculate & display: Number of correct answersNumber of incorrect answersQuestions incorrectly answered.Whether the student has passed or failed the quizA student must correctly answer 11 out of 15 questions to pass.The user can grade as many students as they wish. Notes The file containing the student’s answers is organized as 15 lines. Each line contains the answer to the corresponding question. The responses are single character strings, from A to D, uppercase. Must use functional…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
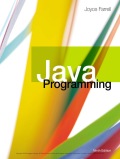
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT