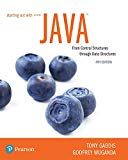
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 8, Problem 5PC
Month Class
Write a class named Month. The class should have an int field named monthNumber that holds the number of the month. For example, January would be 1, February would be 2, and so forth. In addition, provide the following methods:
- A no-arg constructor that sets the monthNumber field to 1.
- A constructor that accepts the number of the month as an argument. It should set the monthNumber field to the value passed as the argument. if a value less than 1 or greater than 12 is passed, the constructor should set monthNumber to 1.
- A constructor that accepts the name of the month, such as “January” or “February” as an argument. It should set the monthNumber field to the correct corresponding value.
- A setMonthNumber method that accepts an int argument, which is assigned to the monthNumber field. If a value less than 1 or greater than 12 is passed, the method should set monthNumber to 1.
- A getMonthNumber method that returns the value in the monthNumber field.
- A getMonthName method that returns the name of the month. For example, if the monthNumber field contains 1, then this method should return “January”.
- A toString method that returns the same value as the getMonthName method.
- An equals method that accepts a Month object as an argument. If the argument object holds the same data as the calling object, this method should return true. Otherwise, it should return false.
- A greaterThan method that accepts a Month object as an argument. If the calling object’s monthNumber field is greater than the argument’s monthNumber field, this method should return true. Otherwise, it should return false.
- A lessThan method that accepts a Month object as an argument. If the calling object’s monthNumber field is less than the argument’s monthNumber field, this method should return true. Otherwise, it should return false.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Dijkstra's Algorithm (part 1). Consider the network shown below, and Dijkstra’s link-state algorithm. Here, we are interested in computing the least cost path from node E (note: the start node here is E) to all other nodes using Dijkstra's algorithm. Using the algorithm statement used in the textbook and its visual representation, complete the "Step 0" row in the table below showing the link state algorithm’s execution by matching the table entries (i), (ii), (iii), and (iv) with their values. Write down your final [correct] answer, as you‘ll need it for the next question.
4. |z + 5 - 5i| = 7
14.
dz,
C: |z❘
C: |z❘ = 0.6
ze² - 2iz
H
Chapter 8 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 8.1 - Prob. 8.1CPCh. 8.1 - Prob. 8.2CPCh. 8.1 - Describe the limitation of static methods.Ch. 8.8 - Prob. 8.4CPCh. 8.9 - Look at the following statement, which declares an...Ch. 8.9 - Assume that the following enumerated data type has...Ch. 8.9 - Prob. 8.7CPCh. 8 - This type of method cannot access any non-static...Ch. 8 - Prob. 2MCCh. 8 - If you write this method for a class, Java will...
Ch. 8 - Making an instance of one class a field in another...Ch. 8 - This is the name of a reference variable that is...Ch. 8 - This enum method returns the position of an enum...Ch. 8 - Assuming the following declaration exists: enum...Ch. 8 - You cannot use the fully qualified name of an enum...Ch. 8 - The Java Virtual Machine periodically performs...Ch. 8 - If a class has this method, it is called...Ch. 8 - CRC stands for a. Class, Return value, Composition...Ch. 8 - True or False: A static member method may refer to...Ch. 8 - True or False: All static member variables are...Ch. 8 - Prob. 14TFCh. 8 - Prob. 15TFCh. 8 - Prob. 16TFCh. 8 - True or False: Enumerated data types are actually...Ch. 8 - True or False: enum constants have a toString...Ch. 8 - public class MyClass { private int x; private...Ch. 8 - Assume the following declaration exists : enum...Ch. 8 - Consider the following class declaration: public...Ch. 8 - Consider the following class declaration: public...Ch. 8 - A pet store sells dogs, cats, birds, and hamsters....Ch. 8 - Prob. 1SACh. 8 - Prob. 2SACh. 8 - Prob. 3SACh. 8 - Even if you do not write an equals method for a...Ch. 8 - A has a relationship can exist between classes....Ch. 8 - Prob. 6SACh. 8 - Is it advisable or not advisable to write a method...Ch. 8 - Prob. 8SACh. 8 - Look at the following declaration: enum Color {...Ch. 8 - Assuming the following enum declaration exists:...Ch. 8 - Under what circumstances does an object become a...Ch. 8 - Area Class Write a class that has three overloaded...Ch. 8 - BankAccount Class Copy Constructor Add a copy...Ch. 8 - Carpet Calculator The Westfield Carpet Company has...Ch. 8 - LandTract Class Make a LandTract class that has...Ch. 8 - Month Class Write a class named Month. The class...Ch. 8 - CashRegister Class Write a CashRegister class that...Ch. 8 - Sales Receipt File Modify the program you wrote in...Ch. 8 - Parking Ticket Simulator For this assignment you...Ch. 8 - Geometry Calculator Design a Geometry class with...Ch. 8 - Car Instrument Simulator For this assignment, you...Ch. 8 - First to One Game This game is meant for two or...Ch. 8 - Heads or TaiLs Game This game is meant for two or...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Explain how each of the following types of integrity constraints is enforced in the SQL CREATE TABLE commands: ...
Modern Database Management
This optional Google account security feature sends you a message with a code that you must enter, in addition ...
SURVEY OF OPERATING SYSTEMS
Should file stream objects be passed to functions by value or by reference? Why?
Starting Out with C++ from Control Structures to Objects (9th Edition)
In programming we use the term string to mean _____. a. many lines of code b. parallel memory locations c. stri...
Starting Out With Visual Basic (8th Edition)
Find the no-load value of υo in the circuit shown.
Find υo when RL is 150 Ω.
How much power is dissipated in th...
Electric Circuits. (11th Edition)
When displaying a Java applet, the browser invokes the _____ to interpret the bytecode into the appropriate mac...
Web Development and Design Foundations with HTML5 (8th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
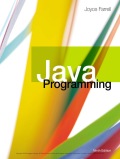
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
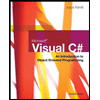
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
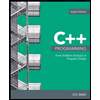
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
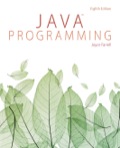
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
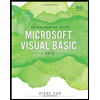
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY