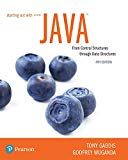
Car Instrument Simulator
For this assignment, you will design a set of classes that work together to simulate a car’s fuel gauge and odometer. The classes you will design are the following:
- The Fuel Gauge Class: This class will simulate a fuel gauge. Its responsibilities are as follows:
- To know the car’s current amount of fuel, in gallons.
- To report the car’s current amount of fuel, in gallons.
- To be able to increment the amount of fuel by 1 gallon. This simulates putting fuel in the car. (The car can hold a maximum of 15 gallons.)
- To be able to decrement the amount of fuel by 1 gallon, if the amount of fuel is greater than 0 gallons. This simulates burning fuel as the car runs.
- The Odometer Class: This class will simulate the car’s odometer. Its responsibilities are as follows:
- To know the car’s current mileage.
- To report the car’s current mileage.
- To be able to increment the current mileage by 1 mile. The maximum mileage the odometer can store is 999,999 miles. When this amount is exceeded, the odometer resets the current mileage to 0.
- To be able to work with a FuelGauge object. It should decrease the FuelGauge object’s current amount of fuel by 1 gallon for every 24 miles traveled. (The car’s fuel economy is 24 miles per gallon.)
Demonstrate the classes by creating instances of each. Simulate filling the car up with fuel, and then run a loop that increments the odometer until the car runs out of fuel. During each loop iteration, print the car’s current mileage and amount of fuel.

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Additional Engineering Textbook Solutions
Starting out with Visual C# (4th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
Computer Science: An Overview (12th Edition)
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Starting Out with C++ from Control Structures to Objects (8th Edition)
- Quadratic equations The form of a quadratic equation is ax2 + bx + c = 0. Write a program that solves a quadratic equation in all cases, including when both roots are complex numbers. Remember that the solutions are x = (-b + sqrt(b2 -4ac))/2a and (-b - sqrt(b2 -4ac))/2a For this you will need to set up the following classes: Complex: Encapsulates a complex number. ComplexPair: Encapsulates a pair of complex numbers. Quadratic: Encapsulates a quadratic equation. – Assume the coefficients are all of type int for this example. SolveEquation: Contains the main method. (I give this to you it is shown below) Along with the usual constructors, accessors and mutators you will need the following additional methods: In the Complex class a method called isReal to determine whether a complex object is real or not returning a boolean value. In the ComplexPair class a method bothIdentical that determines if both complex numbers are identical, returning a boolean value. In the…arrow_forwardPersonal Information ProgramDevelop a program, in which you’ll design a class that holds the following personal data: name, address, age, and phone number. As part of the program, you’ll then create appropriate accessor and mutator methods. Also, set up three instances of the class. One instance should hold your information, and the other two should hold your friends’ or family members’ information.Input: data for the following attributes of 3 instances of the Personal Information Class: name, address, age, and phone number NOTE: The input data do not need to be real data; they need to be entered at the keyboard, when program is run.Processing: Develop the following: Personal Information Class A program that creates three instances of the above class Output:Set up a loop and display data within each object: name, address, age, and phone numberarrow_forwardBuild a class named car. This class is defined as follows: It has the fields: Car ID, Car model , Car make, Car color, Car year. Build a constructor that accepts the five parameters (Car ID, Car model , Car make,Car color, Car year). Override the method toString() to return the string representation of Car ID, Carmodel , Car make, Car color, Car year.arrow_forward
- Question Create a class called Quadratic for performing arithmetic on and solving quadratic equations. A quadratic equation is an equation of the form ax2 + bx + c = 0 Where a !=0. Use double variables to represent the values of a, b, and c and provide a constructor that enables objects of this class to be initialized when they are created. Give default values of a = 1, b = 0, and c = 0. Create a char variable called variable to represent the variable used in the equation and give it a default value of x. The constructor should not allow the value of a to be 0. If 0 is given, assign 1 to a). Provide public member functions that perform the following tasks. add—adds two Quadratic equations by adding the corresponding values of a, b, and c. The function takes another object of type Quadratic as its parameter and adds it to the calling object. subtract—subtracts two Quadratic equations by subtracting corresponding values of a, b, and…arrow_forwardWhat is the purpose of having a destructor in a class? Your answer should be in the blanks.arrow_forwarda. Sammy's Seashore Supplies rents beach equipment such as kayaks, canoes, beach chairs, and umbrellas to tourists. In Chapters 3 and 4, you created a Rental class for the company. Now, make the following change to the class: Currently, a rental price is calculated as $40 per hour plus $1 for each minute over a full hour. This means that a customer who rents equipment for 41 or more minutes past an hour pays more than a customer who waits until the next hour to return the equipment. Change the price calculation so that a customer pays $40 for each full hour and $1 for each extra minute up to and including 40 minutes. Save the file as Rental.java. b. In Chapter 4, you modified the RentalDemo class to demonstrate a Rental object. Now, modify that class again as follows: Instantiate three Rental objects, and prompt the user for values for each object. Display the details for each object to verify that the new price calculation works correctly. Create a method that accepts two Rental…arrow_forward
- JAVA PROGRAMMING Write two java files for this assignment: Circle.java, and Main.java. The Circle.java file will create an actual class. The Circle class: MEMBER VARIABLE double radius double x double y Eight methods: Six of the methods are simple: getter’s and setter’s for x, y, and radius. There should also be a getArea method that returns the area (derived from the radius) A doesOverlap method. This method should accept a Circle as an argument, and return true if this circle overlaps the circle that the method was invoked on. [Note: two circles overlap if the sum of their radius' is greater than or equal to the distance between their centers.] void setX(double value) double getX() void setY(double value) double getY() void setRadius(double value) double getRadius() double getArea() boolean doesOverlap(Circle otherCircle) second class will be the typical replit Main class, with its static main method .It should contain code that tests the Circle class. submit code that…arrow_forward7)Circle Class Write a Circle class that has the following fields: radius: a double PI:a final double initialized with the value 3.14159 The class should have the following methods: Constructor. Accepts the radius of the circles as an argument. Constructor. A no-arg constructor that sets the radius field to 0.0. setRadius. A mutator method for the radius field. getRadius.An accessor method for the radius field. area. Returns the area of the circle, which is calculated as area. Returns the area of the circles, which is calculated as area= PI * radius * radius diameter. Returns the diameter of the cirlce, which is calculated as diameter = radius * 2 circumference. Returns the circumference of the circle, which is calculated as circumference = 2 * PI * radius Write a program that demonstrates the Circle class by asking the user for the circles's radius, creating a Circle object, and then reporting the cirlce's area, diameter, and circumference.arrow_forwardIn the board game Scrabble, each tile contains a letter, which is used to spell words in rows and columns, and a score, which is used to determine the value of words. The point of this exercise is to practice the mechanical part of creating a new class definition: Write a definition for a class named Tile that represents Scrabble tiles. The instance variables should be a character named "letter" and an integer named "value".arrow_forward
- Question: 2Implement the design of the Chillox and Customer classes so that the following code generates the output below:print(f"It's been a good day!. \nToday, we have already served {Chillox.order_id} customers.")print("**************************************************")outlet1 = Chillox()print("1.==========================================")print("Burger Menu:")print(Chillox.menu)print("2.==========================================")outlet1.addBurger("Chicken Burger",180,"Chicken with Cheese",200)print("3.==========================================")print("Burger Menu:")print(Chillox.menu)print("4.==========================================")c1 = Customer("Samin")c1.order = "2xChicken Burger"outlet1.placeOrderof(c1)print("5.==========================================")outlet1.addBurger('Chicken Smoky BBQ Cheese',225)c2 = Customer("Rafi")c2.setOrder("2xChicken with Cheese,3xChicken Smoky BBQ…arrow_forwardA SuperDie Class Write a class SuperDie that models a single die with an arbitrary number of sides, not just six. A die instantiated with the default constructor has six sides. The methods of this class should be: • roll a die and return its value, • return the number of sides on a die, and • change the number of sides on a die. Include a main(...) method that tests all the methods of your class.arrow_forwardWrite a class called Alien. The Alien class must have one field of type String called name, one of type String called planet and one field of type boolean called humanoid. Write one constructor that takes three parameters to initialise all of these fields. The parameters must be passed in the order name, planet and humanoid status. IntelliJ hint (this won't be in the real test): put your cursor after the last field; right-click, choose Generate, choose Constructor, select the fields that are set via parameters and click Ok. Check that the constructor is correct. Write a getter method for each field. IntelliJ hint (this won't be in the real test): put your cursor after the closing curly bracket of the constructor; right click, choose Generate, choose Getter, select all of the fields; click Ok. Check that the you have three getters. Do not write setters methods. Write a method called getDetails that will return the values of the fields in one of the following two formats. For example,…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
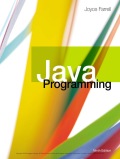
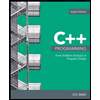
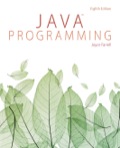
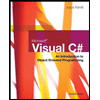