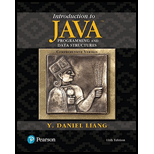
Concept explainers
(Sum elements column by column) Write a method that returns the sum of all the elements in a specified column in a matrix using the following header:
public static double sumColumn(double[][] m, int columnIndex)
Write a test

Sum of elements column by column
Program Plan:
- Include the “Scanner” package to get input values from user.
- Define the class named “Test”.
- Define the main method.
- Define the object “obj” for “Scanner” class to get input.
- Declare the multi-dimensional array named “array”, and prompt the user to get array input.
- Define the “for” loops, that get the input values for the variable “array”.
- Define the “for” loop, that call the method “sumColumn()” with two arguments, they are array and index of array.
- Define the method named “sumColumn()” with two arguments. One is a array variable “m” in type of “double” and another one is “columnIndex” with integer data type.
- Declare the variable “total” in type of “double” and initialize the variable with “0”.
- Set the “for” loop, the loop executes from “0” to length of array “m”.
- Add the column values and store it into the “total”.
- Return the value of “total”.
- Define the main method.
The following JAVA code is to sum the elements of array column by column using the method “sumColumn(double[][] m, int columnIndex)”.
Explanation of Solution
Program:
//Insert package
import java.util.Scanner;
//Class definition
public class Test
{
//Main method
public static void main(String[] args)
{
//Assign the object for "Scanner" class
Scanner obj = new Scanner(System.in);
//Print statement
System.out.print("Enter a 3 by 4 matrix row by row: ");
//Declaration of variable
double[][] array = new double[3][4];
//Outer loop
for (int i = 0; i < array.length; i++)
//Inner Loop
for (int j = 0; j <array[i].length; j++)
//Get input from user
array[i][j] = obj.nextDouble();
//Loop
for (int j = 0; j < array[0].length; j++)
{
//Print statement with function call
System.out.println("Sum of the elements at column " + j + " is " + sumColumn(array, j));
}
}
//Function definition
public static double sumColumn(double[][] m, int columnIndex)
{
//Declaration of variable
double total = 0;
//Loop
for (int i = 0; i < m.length; i++)
//Add the array values into variable
total += m[i][columnIndex];
//Return statement
return total;
}
}
Enter a 3 by 4 matrix row by row:
1.5 2 3 4
5.5 6 7 8
9.5 1 3 1
Sum of the elements at column 0 is 16.5
Sum of the elements at column 1 is 9.0
Sum of the elements at column 2 is 13.0
Sum of the elements at column 3 is 13.0
Want to see more full solutions like this?
Chapter 8 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Starting Out with Python (4th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Concepts Of Programming Languages
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Introduction To Programming Using Visual Basic (11th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
- What IETF protocol is NetFlow associated with? Group of answer choices IPX/SPX IPIX HTTPS SSHarrow_forwardHow can I perform Laplace Transformation when using integration based on this?arrow_forwardWrite an example of a personal reflection of your course. - What you liked about the course. - What you didn’t like about the course. - Suggestions for improvement. Course: Information and Decision Sciences (IDS) The Reflection Paper should be 1 or 2 pages in length.arrow_forward
- using r languagearrow_forwardI need help in explaining how I can demonstrate how the Laplace & Inverse transformations behaves in MATLAB transformation (ex: LIke in graph or something else)arrow_forwardYou have made the Web solution with Node.js. please let me know what problems and benefits I would experience while making the Web solution here, as compared to any other Web solution you have developed in the past. what problems and benefits/things to keep in mind as someone just learningarrow_forward
- PHP is the server-side scripting language. MySQL is used with PHP to store all the data. EXPLAIN in details how to install and run the PHP/MySQL on your computer. List the issues and challenges I may encounter while making this set-up? why I asked: I currently have issues logging into http://localhost/phpmyadmin/ and I tried using the command prompt in administrator to reset the password but I got the error LOCALHOST PORT not found.arrow_forwardHTML defines content, CSS defines layout, and JavaScript adds logic to the website on the client side. EXPLAIN IN DETAIL USING an example.arrow_forwardusing r languangearrow_forward
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
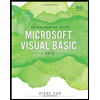
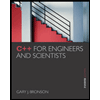
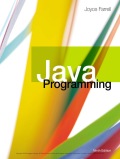
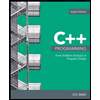
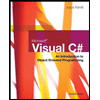