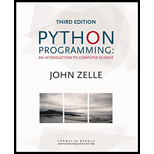
Program plan:
- Import random.
- Define “main()” function.
- Call the function “printIntro()”.
- Get input using “getInputs()” function.
- Call function “simNMatches()” to find the matches.
- Call function “printSummary()”.
- Define a function “printIntro()”.
- Print the statements.
- Define a function “getInputs()”.
- Get the value for simulation parameter “a” from the user.
- Get the value for simulation parameter “b” from the user.
- Get the value for simulation parameter “n” from the user.
- Returns the three simulation parameters.
- Define a function “simNMatches()”.
- Initialize values.
- Iterate a for loop up to “n”.
- Call the function “simOneMatch()”.
- If condition.
- Increment the value of “matchA” by 1.
- Else.
- Increment the value of “matchB” by 1.
- Return the value of “matchA” and “matchB”.
- Define a function “simOneMatch()”.
- Initialize the values “wisnA” and “WinsB” as 0.
- Accumulate number of games.
- Iterate a while loop.
- Call a function “simOneGame()”.
- If condition.
- Increment the value of “winA” by 1.
- Increment the value of “x” by 1.
- Else condition.
- Increment the value of “winsB” by 1.
- Increment the value of “x” by 1.
- Return the value of “winsA” and “winsB”.
- Define a function “simOneGame()”.
- Call a function “findService()” to a value “serving”.
- Initialize values.
- Iterate a while loop.
- If condition “serving == "A"”.
- If condition “random() < probA”.
-
- Increment the value of “scoreA” by 1.
- If not the condition.
-
- Assign a value “B” to a variable “serving”.
- Else if condition.
-
- If condition “random() < probB” increment the value of “scoreB” by 1.
- Increment the value of “scoreB” by 1.
- If not the condition.
-
- assign a value “A” to a variable “serving”
- Return “scoreA”, “scoreB”..
- assign a value “A” to a variable “serving”
- If condition “serving == "A"”.
- Define a function “findService()”.
- If condition “x % 2 == 0”.
- Return “A”.
-
-
- If not the condition.
- Return “B”.
- If not the condition.
-
-
- Return “A”.
- If condition “x % 2 == 0”.
- Define a function “gameOver()”.
- If the condition satisfies.
- Return the value of “b”.
-
-
-
- Else if condition “b == 0 and a == 7”.
- Return the value of “a”.
- Else if condition “abs(a-b) >= 2”.
- Return “True”.
- If not the condition
- Return a value “False”.
- Else if condition “b == 0 and a == 7”.
-
-
-
- Return the value of “b”.
- If the condition satisfies.
- Define a function “printSummary()”.
- Add the value of “matchA” and “matchB” and store in a variable “n”.
- Print the value of “n”.
- Print the value of “matchA”.
- Print the value of “matchB”.
- main for function call

This is a revision of the racquetball simulation. The problem has two major changes:
The program has to calculate results for best of n games.
First service is alternated between A and B. The odd numbered games of the match are served first by A and even numbered games are first served by B.
Explanation of Solution
Program:
#import random
from random import random
#define main() function
def main():
#call the function printIntro()
printIntro()
#get input using getInputs() function
probA, probB, n = getInputs()
#calling function simNMatches() to find the matches
matchA, matchB = simNMatches(n, probA, probB)
#call function printSummary()
printSummary(matchA, matchB)
#define a function printIntro()
def printIntro():
print("This program simulates a game of racquetball between two")
print('players called "A" and "B". The abilities of each player is')
print("indicated by a probability (a number between 0 and 1) that")
print("reflects the likelihood of a player winning the serve.")
print("Player A has the first serve.")
#define a function getInputs()
def getInputs():
#get the value for simulation parameter ‘a’ from the user
a = eval(input("What is the prob. player A wins a serve? "))
#get the value for simulation parameter ‘b’ from the user
b = eval(input("What is the prob. player B wins a serve? "))
#get the value for simulation parameter ‘n’ from the user
n = eval(input("How many games to simulate? "))
#Returns the three simulation parameters
return a, b, n
#define a function simNMatches()
def simNMatches(n, probA, probB):
#initialize values
matchA = matchB = 0
#iterate a for loop up to n
for i in range(n):
#call the function simOneMatch()
winsA, winsB = simOneMatch(probA, probB)
#if condition
if winsA > winsB:
#increment the value of matchA by 1
matchA = matchA + 1
#else
else:
#increment the value of matchB by 1
matchB = matchB + 1
#return the value of matchA and matchB
return matchA, matchB
#define a function simOneMatch()
def simOneMatch(probA, probB):
#initialize the values wisnA and WinsB as 0
winsA = winsB = 0
#accumulate number of games
x = 1
#iterate a while loop
while winsA !=2 and winsB !=2:
#calling a function simOneGame()
scoreA, scoreB = simOneGame(probA, probB, x)
#if condition
if scoreA > scoreB:
#increment the value of winA by 1
winsA = winsA + 1
#increment the value of x by 1
x = x+1
#else condition
else:
#increment the value of winsB by 1
winsB = winsB + 1
#increment the value of x by 1.
x = x+1
#return the value of winsA and winsB
return winsA, winsB
#define a function simOneGame()
def simOneGame(probA, probB, x):
#call a function findService() to a value “serving”
serving = findService(x)
#initialize values
scoreA = 0
scoreB = 0
#iterate a while loop.
while not gameOver(scoreA, scoreB):
#if condition
if serving == "A":
#if condition
if random() < probA:
#increment the value of scoreA by 1
scoreA = scoreA + 1
#if not the condition
else:
#assign a value “B” to a variable “serving”
serving = "B"
#else if condition
elif serving == "B":
#if condition
if random() < probB:
#increment the value of scoreB by 1
scoreB = scoreB + 1
#if not the condition
else:
#assign a value “A” to a variable “serving”
serving = "A"
#return
return scoreA, scoreB
#define a function findService()
def findService(x):
#if condition
if x % 2 == 0:
#return “A”
return "A"
#if not the condition
else:
#return “B”
return "B"
#define a function gameOver()
def gameOver(a, b):
#if the condition satisfies
if a == 0 and b == 7:
#return the value of “b”
return b == 7
#else if condition
elif b == 0 and a == 7:
#return the value of a
return a == 7
#else if condition
elif abs(a-b) >= 2:
#return “True”
return True
#if not the condition
else:
#return a value “False”
return False
#define a function printSummary()
# Prints a summary of wins for each players
def printSummary(matchA, matchB):
#add the value of matchA and matchB and store in a variable n.
n = matchA + matchB
#print the value of n
print("\nGames simulated: ", n)
#print the value of matchA
print("Wins for A: {0} ({1:0.1%})".format(matchA, matchA/n))
#print the value of matchB
print("Wins for B: {0} ({1:0.1%})".format(matchB, matchB/n))
# main for function call
if __name__ == '__main__': main()
Output:
This program simulates a game of racquetball between two
players called "A" and "B". The abilities of each player is
indicated by a probability (a number between 0 and 1) that
reflects the likelihood of a player winning the serve.
Player A has the first serve.
What is the prob. player A wins a serve? 0.5
What is the prob. player B wins a serve? 0.7
How many games to simulate? 2
Games simulated: 2
Wins for A: 1 (50.0%)
Wins for B: 1 (50.0%)
Want to see more full solutions like this?
Chapter 9 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
- Write Algorithm for Synchronized simulation for Guess a Number.in: set of human participants H; set of computer participants Cout: winner wconstant: minimum number nmin; maximum number nmaxlocal: guessed number garrow_forwardImplement an approximation algorithm for the Traveling Salesperson problem, run it on your system, and study it's performances using several problem instances. in any languages and please comment code and screenshotarrow_forwardUsing Java create a computer program that will perform any or a combination of numerical methods y. For example, a program that do regression analysis, outputs a linear model and determine the correlation.arrow_forward
- Which three of the following are instances of discrete event simulations?arrow_forwardI only need Part B answered, but since it refers to Part A in the question, I went ahead and showed the question for Part Aarrow_forwardInvestigate both the iterative and the recursive methods of problem resolution, and then compare and contrast the results of your research. When would you use iteration when recursion would be more appropriate, and when would you use recursion when iteration would be more appropriate? Your answer should be justified by giving examples that are different from those that are shown on the slides of the presentation.arrow_forward
- any help would be appreciated on this coding program. print('==> Bull Kelp and Purple Urchin Population Simulator <==') print('--- Model Parameters ---')# Get parameters from user and error check each print('--- Initial Population ---')# Get populations at time t=0 from user print('--- Simulation ---')# Do simulation to get population at time t=1arrow_forwardWhat is the purpose of the given criteria below in analyzing algorithms for Games? Modularity • User-friendliness Correctness • Programmer time Maintainability • Simplicity Functionality • Extensibility Robustness • Reliabilityarrow_forwardCan you give just one example, different from the one you have answered which works with all the sub-questions a, b, and c?arrow_forward
- Create a scenario that involves solving the real-life problem using a piecewise function and give a brief explanation.arrow_forwardLook at both the iterative and recursive methods of solving problems, and see how they stack up against one another. When should you use recursion and when should you use iteration? As an alternative to relying on the examples provided in the presentation slides, explain why your point of view is correct.arrow_forwardPython This simulates the population of fish. Use this to generate a plot with a numerical solution and the exact solution on the same plot axes for model parameters, P_m = 20,000 fish with a birth rate of b=6%, a harvesting rate of h=4%, a change in t=0.5 and y_0=5000arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
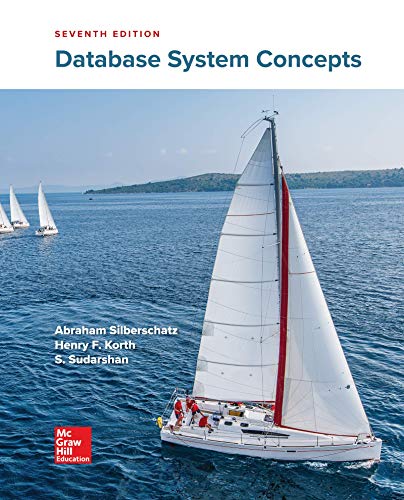
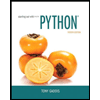
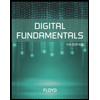
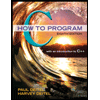
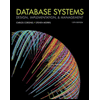
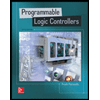