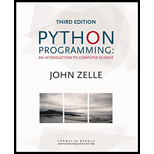
Simulates the tennis game
Program Plan:
- Import the header file.
- Define the “main” method.
- Call the “printIntro ()” method
- Call the “getInputs ()” method.
- Call the “simNMatches ()” method.
- Call the “printSummary ()” method.
- Define the “printIntro()” method.
- Print the intro statements.
- Define the “getInputs()” method.
- Get the player A possible for win from the user.
- Calculate the “probA” and “probB” values.
- Get how many games to simulate from the user.
- Define the “simNMatches()” method.
- Set the values
- Iterate “i” until it reaches “n” value
- Call the methods
- Check “matchA” is greater than “matchB”
- Increment the “winsA” value
- Otherwise, increment the “winsB” value.
- Return the values.
- Define the “simOneMatch ()” method
- Set the values
- Check the condition.
- Call the “simOneGame ()” method.
- Check “setA” is greater than “setB”
- Increment the “matchA” value
- Otherwise, increment the “matchB” value
- Return the results
- Define the “matchOver ()” method
- Check “a” or “b” is greater than 3
- Return true
-
- Otherwise, return false.
-
- Return true
- Check “a” or “b” is greater than 3
- Define the “simOneSet ()” method
- Call the method
- Set the values
- Check the condition.
- Check “scoreA” is greater than “scoreB”
- Increment the “setA” value
- Otherwise, increment the “setB” value
-
- Return the results
-
- Check “scoreA” is greater than “scoreB”
- Define the “setOver ()” method
- Check “a” or “b” is equal to 7
- Return true
-
- Check “a” or “b” is greater than or equal to 6
-
- Check “a-b” is greater than or equal to 2
- Return true
- Otherwise, return false
- Check “a-b” is greater than or equal to 2
- Otherwise, return false.
-
- Return true
- Check “a” or “b” is equal to 7
- Define the “simOneGame ()” method
- Set the values
- Check the condition
- Check “random ()” is less than “probA”
- Increment the “scoreA” value
-
- Otherwise, increment the “scoreB” value
- Return the results.
-
- Increment the “scoreA” value
- Check “random ()” is less than “probA”
- Define the “gameOver ()” method
- Check “a” or “b” is greater than or equal to 4
- Check “a-b” is greater than or equal to 2
- Return true
-
- Otherwise, return false
- Otherwise, return false.
-
- Return true
- Check “a-b” is greater than or equal to 2
- Check “a” or “b” is greater than or equal to 4
- Define the “printSummary ()” method
- Display the results.
- Call the main method.

The program is to simulate a game of tennis.
Explanation of Solution
Program:
#import the header file
from random import random
#definition of main method
def main():
#call the method
printIntro()
#call the method and store it in the variables
probA, probB, n = getInputs()
#call the method and store it in the variables
winsA, winsB = simNMatches(n, probA, probB)
#call the method
printSummary(winsA, winsB, n)
#definition of "printIntro" method
def printIntro():
#display the statements
print("This program simulates a series of tennis matches between player")
print('"A" and player "B". The abilities of each player are')
print("represented by percentage chance of winning a volley. The")
print("percentages add up to 100.")
print()
print("Game")
print("As in real tennis, each game is played through 4 points")
print("(Love, 15, 30, 40, game) where the player must win by two.")
print("Players can score on either serve.")
print()
print("Set")
print("A set is won when a player reaches 6 victorious games, and has a")
print("lead of two. If for example, sets reach 6-5, the players will play")
print("another round. If the score reaches 6-6, there will be a")
print("tiebreaking game.")
print()
print("Match")
print("A Match is won when a player reaches his/her 3rd victorious set.")
print("No winning by two, no tie-breaker, for the purposes of this simulation")
print()
#definition of "getInputs" method
def getInputs():
#get the player A wins a serve
probA = eval(input("What is the percent prob. player A wins a volley? "))
#calculate the values
probA = probA / 100
probB = 1 - probA
#get the input from the user
n = eval(input("How many games to simulate? "))
return probA, probB, n
#definition of "simNMatches" method
def simNMatches(n, probA, probB):
#set the values
winsA = winsB = 0
#iterate until "n" value
for i in range(n):
#call the method and store it in the variables
matchA, matchB = simOneMatch(probA, probB)
#check "matchA" is greater than "matchB"
if matchA > matchB:
#increment the value
winsA = winsA + 1
#otherwise
else:
#increment the value
winsB = winsB + 1
#return the results
return winsA, winsB
#definition of "simOneMatch" method
def simOneMatch(probA, probB):
#set the values
matchA = matchB = 0
#check the condition
while not matchOver(matchA, matchB):
#call the method and store it in the variables
setA, setB = simOneSet(probA, probB)
#check "setA" is greater than "setB"
if setA > setB:
#increment the value
matchA = matchA + 1
#otherwise
else:
#increment the value
matchB = matchB + 1
#return the results
return matchA, matchB
#definition of "matchOver" method
def matchOver(a, b):
#check "a" or "b" is greater than 3
if a > 3 or b > 3:
#return the result
return True
else:
#return the result
return False
#definition of "simOneSet" method
def simOneSet(probA, probB):
#call the method and store it in the variables
scoreA, scoreB = simOneGame(probA, probB)
#set the values
setA = setB = 0
#check the condition
while not setOver(setA, setB):
#check "scoreA" is greater than "scoreB"
if scoreA > scoreB:
#increment the value
setA = setA + 1
else:
#increment the value
setB = setB + 1
#return the results
return setA, setB
#definition of "setOver" method
def setOver(a, b):
#check "a" and "b" is equal to 7
if a == 7 or b == 7:
#return the result
return True
#check "a" or "b" is greater than or equal to 6
elif a >= 6 or b >= 6:
#check "a - b" is greater than or equal to 2
if abs(a-b) >=2:
#return the result
return True
else:
#return the result
return False
else:
#return the result
return False
#definition of "simOneGame" method
def simOneGame(probA, probB):
#set the values
scoreA = scoreB = 0
#check the condition
while not gameOver(scoreA, scoreB):
#check "random()" is less than "probA"
if random() < probA:
#increment the value
scoreA = scoreA + 1
else:
#increment the value
scoreB = scoreB + 1
#return the result
return scoreA, scoreB
#definition of "gameOver" method
def gameOver(a, b):
#check "a" or "b" is greater than or equal to 4
if a >= 4 or b >= 4:
#check "a - b" is greater than or equal to 2
if abs(a-b) >=2:
#return the result
return True
else:
#return the result
return False
else:
#return the result
return False
#definition of "printSummary" method
def printSummary(winsA, winsB, n):
#display the results
print("\nGames simulated: ", n)
print("Wins for A: {0} ({1:0.1%})".format(winsA, winsA/n))
print("Wins for B: {0} ({1:0.1%})".format(winsB, winsB/n))
#call the main method
if __name__ == '__main__': main()
Output:
This program simulates a series of tennis matches between player
"A" and player "B". The abilities of each player are
represented by percentage chance of winning a volley. The
percentages add up to 100.
Game
As in real tennis, each game is played through 4 points
(Love, 15, 30, 40, game) where the player must win by two.
Players can score on either serve.
Set
A set is won when a player reaches 6 victorious games, and has a
lead of two. If for example, sets reach 6-5, the players will play
another round. If the score reaches 6-6, there will be a
tiebreaking game.
Match
A Match is won when a player reaches his/her 3rd victorious set.
No winning by two, no tie-breaker, for the purposes of this simulation
What is the percent prob. player A wins a volley? 50
How many games to simulate? 10
Games simulated: 10
Wins for A: 7 (70.0%)
Wins for B: 3 (30.0%)
Want to see more full solutions like this?
Chapter 9 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
- Find out all that you can about natural language interfaces. Are there any successful systems? For what applications are these most appropriate?arrow_forwardAny suggested modifications to the framework should anticipate and deal with any problems that might arise as a consequence of enforcing them. This has to be taken care of before going on to brainstorming potential fixes. The shift to cloud computing has the potential to solve these problems, but will it really happen? Do we really need anything else greater than this?arrow_forwardIt would be useful to have a more in-depth explanation of the Java programming language.arrow_forward
- Mechanistic and graphical methods were both used to develop mathematical models in this module. With the support of examples, briefly describe the key features of these two approachesarrow_forwardDescribe the process of simulating something. Is there anything about MATLAB that makes it stand out as one of the top simulation tools available?arrow_forwardIt is possible that a computer may provide more accurate findings from a simulation without using one. Explain.arrow_forward
- What exactly is emulation? What is the relationship between interpretation and simulation?arrow_forwardExplore the use of mathematical modeling in real-world applications like robotics, economics, and environmental science.arrow_forwardExplore the use of interface-based design patterns such as the Strategy Pattern and Observer Pattern in real-world software development.arrow_forward
- Starting Out with Java From Control Structures through Objects 6th Edition Paint Job Estimatorarrow_forwardGive a definition of the parts of a system from the perspective of a computer simulation. In order to better understand, it would be helpful to see some examples of each.arrow_forwardDynamically typed programming languages, like Lisp and Python, differ from statically typed languages like C++ and Java, which are used to create more traditional applications. Compare and contrast the benefits and drawbacks of each typing method.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
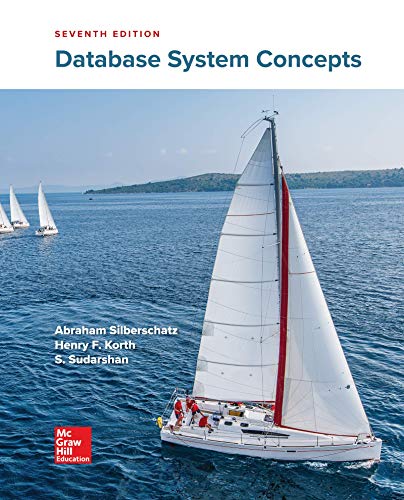
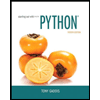
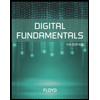
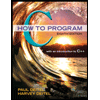
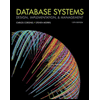
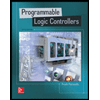