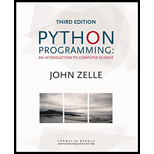
Simulates the volleyball game
Program Plan:
- Import the header file.
- Define the “main” method.
- Call the “printIntro ()” method
- Call the “getInputs ()” method.
- Call the “normalVolley ()” method.
- Call the “rallyVolley ()” method.
- Call the “printSummary ()” method.
- Define the “printIntro()” method.
- Print the intro statements.
- Define the “getInputs()” method.
- Get the player A possible for win from the user.
- Get the player B possible for win from the user.
- Get how many games to simulate from the user.
- Define the “normalVolley()” method.
- Set the values
- Iterate “i” until it reaches “n” value
- Call the method
- Check “scoreA” is greater than “scoreB”
- Increment the “winsA” value
- Otherwise, increment the “winsB” value.
- Return the value.
- Define the “simOneMatch ()” method
- Set the values
- Check “winsA” and “winsB” is not equal to 2
- Call the “simOneGame ()” method.
- Check “scoreA” is greater than “scoreB”
- Increment the “winsA” value
- Increment the “x” value
- Otherwise, increment the “winsB” value
- Increment the “x” value
- Return the result
- Define the “simOneGame ()” method
- Call the “findService ()” method
- Set the values
- Check the condition
- If “serving” is equal to “A”
- Check “random ()” is less than “probA”
- Increment the “scoreA” value
- Otherwise, set the value
- Check “random ()” is less than “probA”
- Check “serving” is equal to “B”
- Check “random ()” is less than “probB”
- Increment the “scoreB” value
- Otherwise, set the value.
- Return the results.
- If “serving” is equal to “A”
- Define the “findService ()” method
- Check result of “x%2” is equal to 0
- Return “A”
-
-
- Otherwise, return “B”.
-
-
- Return “A”
- Check result of “x%2” is equal to 0
- Define the “gameOver ()” method
- Check “a” or “b” is greater than 15
- Check “a-b” is greater than or equal to 2
- Return true.
- Otherwise, return false.
-
-
- Otherwise, return false.
-
-
- Check “a-b” is greater than or equal to 2
- Check “a” or “b” is greater than 15
- Define the “rallyVolley()” method.
- Set the values
- Iterate “i” until it reaches “n” value
- Call the method
- Check “scoreA” is greater than “scoreB”
- Increment the “winsA” value
- Otherwise, increment the “winsB” value.
- Return the value.
- Define the “simOneGame ()” method
- Call the “findService ()” method
- Set the values
- Check the condition
- If “serving” is equal to “A”
- Check “random ()” is less than “probA”
- Increment the “scoreA” value
- Otherwise, set the value.
- Increment the “scoreB” value.
- Check “random ()” is less than “probA”
- Check “serving” is equal to “B”
- Check “random ()” is less than “probB”
- Increment the “scoreB” value
- Otherwise, set the value.
- Increment the “scoreA” value.
- Return the results.
- If “serving” is equal to “A”
- Define the “findService ()” method
- Check result of “x%2” is equal to 0
- Return “A”
-
-
- Otherwise, return “B”.
-
-
- Return “A”
- Check result of “x%2” is equal to 0
- Define the “gameOver ()” method
- Check “a” or “b” is greater than 25
- Check “a-b” is greater than or equal to 2
- Return true.
- Otherwise, return false.
-
-
- Otherwise, return false.
-
-
- Check “a-b” is greater than or equal to 2
- Check “a” or “b” is greater than 25
- Define the “printSummary ()” method
- Display the results.
- Calculate the “devA” and “devB” values
- Check the condition.
- Check “devA” is greater than 0
- Display the result
- Check “devA” is less than 0
- Display the result.
- Otherwise, display scoring difference
- Check “devB” is greater than 0
- Display the result.
- Check “devB” is less than 0
- Display the result
- Check “devA” is greater than 0
- Call the main method.

The program is to simulate a game of volleyball and investigate whether rally scoring reduces, magnifies, or has no effect on the relative advantage enjoyed by the better team.
Explanation of Solution
Program:
#import the header file
from random import random
#definition of main method
def main():
#call the method
printIntro()
#call the method and store it in the variables
probA, probB, n = getInputs()
winsA1, winsB1 = normalVolley(n, probA, probB)
winsA2, winsB2 = rallyVolley(n, probA, probB)
#call the method
printSummary(winsA1, winsB1, winsA2, winsB2, n)
#definition of "printIntro" method
def printIntro():
#display the statements
print("This program is designed to compare the difference between Normal")
print("and Rally scoring in a simulation of (n) games of Volleyball")
print("given a probability (a number between 0 and 1) that reflects the")
print("likelihood of a team winning the serve.")
#definition of "getInputs" method
def getInputs():
#get the player A wins a serve
a = eval(input("What is the prob. player A wins a serve? "))
#get the player B wins a serve
b = eval(input("What is the prob. player B wins a serve? "))
#get the input from the user
n = eval(input("How many games to simulate? "))
#return the values
return a, b, n
#definition of "normalVolley" method
def normalVolley(n, probA, probB):
#set the values
winsA = winsB = 0
#iterate until "n" value
for i in range(n):
#call the method and store it in the variables
scoreA, scoreB = simOneGame(probA, probB, n)
#check "scoreA" is greater than "scoreB"
if scoreA > scoreB:
#increment the value
winsA = winsA + 1
#otherwise
else:
#increment the value
winsB = winsB + 1
#return the results
return winsA, winsB
#definition of "simOneGame" method
def simOneGame(probA, probB, n):
#call the method
serving = findService(n)
#set the values
scoreA = 0
scoreB = 0
#check the condition
while not gameOver(scoreA, scoreB):
#check "serving" is equal to "A"
if serving == "A":
#check "random()" is less than "probA"
if random() < probA:
#increment the value
scoreA = scoreA + 1
#otherwise
else:
#set the value
serving = "B"
#check "serving" is equal to "B"
if serving == "B":
#check "random()" is less than "probB"
if random() < probB:
#increment the value
scoreB = scoreB + 1
#otherwise
else:
#set the value
serving = "A"
#return the result
return scoreA, scoreB
#definition of "findService" method
def findService(x):
#check the condition
if x % 2 == 0:
#return the value
return "A"
#otherwise
else:
#return the value
return "B"
#definition of "gameOver" method
def gameOver(a, b):
#check "a" or "b" is greater than 15
if a > 15 or b > 15:
#check "a-b" is greater than or equal to 2
if abs(a-b) >= 2:
#return the value
return True
#otherwise
else:
#return the value
return False
#otherwise
else:
#return the value
return False
#definition of "rallyVolley" method
def rallyVolley(n, probA, probB):
#set the values
winsA = winsB = 0
#iterate until "n" value
for i in range(n):
#call the method and store it in the variables
scoreA, scoreB = simOneGame(probA, probB, n)
#check "scoreA" is greater than "scoreB"
if scoreA > scoreB:
#increment the value
winsA = winsA + 1
#otherwise
else:
#increment the value
winsB = winsB + 1
#return the results
return winsA, winsB
#definition of "simOneGame" method
def simOneGame(probA, probB, n):
#call the method
serving = findService(n)
#set the values
scoreA = 0
scoreB = 0
#check the condition
while not gameOver(scoreA, scoreB):
#check "serving" is equal to "A"
if serving == "A":
#check "random()" is less than "probA"
if random() < probA:
#increment the value
scoreA = scoreA + 1
#otherwise
else:
#set the value
serving = "B"
#increment the value
scoreB = scoreB + 1
#check "serving" is equal to "B"
if serving == "B":
#check "random()" is less than "probB"
if random() < probB:
#increment the value
scoreB = scoreB + 1
#otherwise
else:
#set the value
serving = "A"
#increment the value
scoreA = scoreA + 1
#return the result
return scoreA, scoreB
#definition of "findService" method
def findService(x):
#check the condition
if x % 2 == 0:
#return the value
return "A"
#otherwise
else:
#return the value
return "B"
#definition of "gameOver" method
def gameOver(a, b):
#check "a" or "b" is greater than 15
if a > 25 or b > 25:
#check "a-b" is greater than or equal to 2
if abs(a-b) >= 2:
#return the value
return True
#otherwise
else:
#return the value
return False
#otherwise
else:
#return the value
return False
#definition of "printSummary" method
def printSummary(winsA1, winsB1, winsA2, winsB2, n):
#display the results
print("\nGames simulated: ", n)
print("Results for Normal Volleyball to 15, score on own serve.")
print("Wins for A: {0} ({1:0.1%})".format(winsA1, winsA1/n))
print("Wins for B: {0} ({1:0.1%})".format(winsB1, winsB1/n))
print()
print("Results for Rally Volleyball to 25, score on any serve.")
print("Wins for A: {0} ({1:0.1%})".format(winsA2, winsA2/n))
print("Wins for B: {0} ({1:0.1%})".format(winsB2, winsB2/n))
print()
#calculate the "devA" value
devA = (winsA2/n - winsA1/n)
#calculate the "devB" value
devB = (winsB2/n - winsB1/n)
#check the condition
if (winsA1 > winsB1) and (winsA2 > winsB2):
#check "devA" greater than 0
if devA > 0:
#display the result
print("Team A's advantage was magnified {0:0.1%} in their favor".format(devA))
print("during the rally scoring match.")
#check "devA" less than 0
elif devA < 0:
#display the result
print("Team A's advantage was reduced {0:0.1%} in their favor".format(abs(devA)))
print("during the rally scoring match.")
#otherwise
else:
#display the result
print("The scoring differences were not statistically significant.")
#check the condition
elif (winsB1 > winsA1) and (winsB2 > winsA2):
#check "devB" greater than 0
if devB > 0:
#display the result
print("Team B's advantage was magnified {0:0.1%} in their favor".format(devB))
print("during the rally scoring match.")
#check "devB" less than 0
elif devB < 0:
#display the result
print("Team B's advantage was reduced {0:0.1%} in their favor".format(abs(devB)))
print("during the rally scoring match.")
#otherwise
else:
#display the result
print("The scoring differences were not statistically significant.")
#otherwise
else:
#display the results
print("Neither team's advantage was statistically significant.")
print("If the initial probabilities were near equal, it is impossible to")
print("derive significance from the data.")
print("Try a larger sample size.")
if __name__ == '__main__': main()
Output:
This program is designed to compare the difference between Normal
and Rally scoring in a simulation of (n) games of Volleyball
given a probability (a number between 0 and 1) that reflects the
likelihood of a team winning the serve.
What is the prob. player A wins a serve? 0.65
What is the prob. player B wins a serve? 0.6
How many games to simulate? 5000
Games simulated: 5000
Results for Normal Volleyball to 15, score on own serve.
Wins for A: 3312 (66.2%)
Wins for B: 1688 (33.8%)
Results for Rally Volleyball to 25, score on any serve.
Wins for A: 3325 (66.5%)
Wins for B: 1675 (33.5%)
Team A's advantage was magnified 0.3% in their favor
during the rally scoring match.
Want to see more full solutions like this?
Chapter 9 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
- Is it possible to make the design of your system more efficient using heuristics?arrow_forwardHow can we make the most of simulation models while trying to recreate situations that do not neatly fall into one of many predetermined categories?arrow_forwardWrite a program in the Julia programming language that captures a sequential game between two players in its extensive form and computes each player’s payoff using a mixed strategy.arrow_forward
- Could you comment on each of the following four key benefits of continuous event simulation that I've listed below?arrow_forwardDraw the tracing table that shows an example of execution of Finding the highest mark of the student in 8 assessments. Javaarrow_forwardCreate a working definition of the system's parts from the perspective of a computer simulation.arrow_forward
- Go to Invent with Python, Making Games with Python & PyGame and choose either Chapter 4: Slide Puzzle or Chapter 5: Simulate to complete.arrow_forwardJudging rules can be difficult – even for an objective computer program. In football (orsoccer as some people call it), the official rules say that the referee can allow the playto continue ‘when the team against which an offence has been committed will benefitfrom such an advantage’ and penalize ‘the original offence if the anticipated advantagedoes not ensue at that time’ (Federation Internationale de Football Association 2003).How would you implement this rule? What difficulties are involved in it?arrow_forwardWould you be able to provide a more in-depth explanation of each of the following four primary advantages of using continuous event simulation?arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
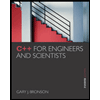