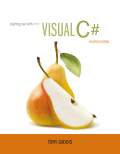
Car Class
Create a class named Car that has the following properties:
- Year—The Year property holds the car’s year model.
- Make—The Make property holds the make of the car.
- Speed—The Speed property holds the car’s current speed.
In addition, the class should have the following constructor and other methods:
- Constructor—The constructor should accept the car’s year and model and make them as arguments. These values should be assigned to the backing fields for the object’s Year and Make properties. The constructor should also assign 0 to the backing field for the Speed property.
- Accelerate—The Accelerate method should add 5 to the Speed property’s backing field each time it is called.
- Brake—The Brake method should subtract 5 from the Speed property’s backing field each time it is called.
Demonstrate the class in an application that creates a Car object. The application’s form should have an Accelerate button that calls the Accelerate method and then displays the car’s current speed each time it is clicked. The application’s form should also have a Brake button that calls the Brake method and then displays the car’s current speed each time it is clicked.

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
EBK STARTING OUT WITH VISUAL C#
Additional Engineering Textbook Solutions
Starting Out with Java: Early Objects (6th Edition)
C++ How to Program (10th Edition)
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Programming in C
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
- Assume that you have created a class named MyClass. The header of the MyClass constructor can be ____________. public MyClass() public MyClass (double d) Either of these can be the constructor header. Neither of these can be the constructor header.arrow_forward# Car Class CompositionCreate a class called `Car` that will utilize other objects. ## Car ### Car member variablesCreate two data members that are: (1) an instance of the `VehicleId` class called `id_` and (2) an instance of the `Date` class called `release_date_`. *NOTE*: `VehicleId` and `Date` are classes that have been provided to you. You **DO NOT** need to create them. ### Default ConstructorThe default constructor will be **EMPTY**, so you do not have to initialize anything. `VehicleId` and `Date`'s respective constructors will initialize their default values. ### Non-Default Constructors1. Create a non-default constructor that takes in a `VehicleId` object. This will assign the parameter to the `id_` member variable.2. Create a non-default constructor that takes in a `Date` object. This will assign the parameter to the `release_date_` member variable.3. Create a non-default constructor that takes in a `VehicleId` and a `Date` object (in this order). This will assign the…arrow_forwardconsole application named SalesTransactionDemo that declares several SalesTransaction objects and dispplays their values and their sum. The SalesTransaction class contains fields for a salesperson name, sales amount, and comission rate. Include three constructors for the class. Once constructor accepts values for the name, sales amount, and rate, and when the sales value is set, the constructor computes the commission as sales value time commission rate. The second constructor accepts a name and sales amount, but sets the commission rate to 0. The third constructor accepts a name and sets all other fields to 0. An overloaded + operator add the sales values for two SalesTransaction objects.arrow_forward
- Employee and ProductionWorker ClassesCreate an Employee class that has properties for the following data:Employee nameEmployee numberNext, create a class named ProductionWorker that is derived from the Employee class. The ProductionWorker class should have properties to hold the following data:Shift number (an integer, such as 1, 2, or 3)Hourly pay rateThe workday is divided into two shifts: day and night. The Shift property will hold an integer value representing the shift that the employee works. The day shift is shift 1 and the night shift is shift 2.Create an application that creates an object of the ProductionWorker class and lets the user enter data for each of the object’s properties. Retrieve the object’s properties and display their values. this.ReportViewer1.Employee(); cannot reference?arrow_forwardClass object method This program has three methods and two constructorsone constructor has one parameter and the other none The class has two primitive variables one static and the other notThe static member is initialized to greater than 0 the non-static to 0 Variables in two methods are assigned with the static member main() has an initialized variable with a value not the same as others in the program; it is used as the argument to one of the constructors; this value is used to assign a value to the static class variable The constructor that takes no argument has a variable declared with the same name as the static variable, it is initialize by the non-static variable A.Demonstrate and explain the displayed results of objects created in the following order Constructor(arg)Constructor() B.Create a second program to Demonstrate and explain Constructor()Constructor(arg) Iolecnotes Assignment 4. Write a program to create two files with the names file4 and file4a two 10 element int…arrow_forward2. Car Class Write a class named car that has the following fields: ▪ yearModel. The year Model field is an int that holds the car's year model. ▪ make. The make field is a String object that holds the make of the car, such as "Ford", "Chevrolet", "Honda", etc. ▪ speed. The speed field is an int that holds the car's current speed. In addition, the class should have the following methods. ■ Constructor. The constructor should accept the car's year model and make as arguments. These values should be assigned to the object's year Model and make fields. The constructor should also assign 0 to the speed field.arrow_forward
- (Date Class) Create a class called Date that includes three pieces of information as auto-implemented properties—a month (type int), a day (type int) and a year (type int). Your class should have a constructor that initializes the three automatic properties and assumes that the values provided are correct. Provide a method DisplayDate that displays the month, day and year separated by forward slashes (/). Write a test app named DateTest that demonstrates class Date’s capabilities.arrow_forward(Invoice Class) Create a class called Invoice that a hardware store might use to representan invoice for an item sold at the store. An Invoice should include four data members—a part number (type string), a part description (type string), a quantity of the item being purchased (typeint) and a price per item (type int). Your class should have a constructor that initializes the fourdata members. A constructor that receives multiple arguments is defined with the form:ClassName( TypeName1 parameterName1, TypeName2 parameterName2, ... )Provide a set and a get function for each data member. In addition, provide a member functionnamed getInvoiceAmount that calculates the invoice amount (i.e., multiplies the quantity by theprice per item), then returns the amount as an int value. If the quantity is not positive, it should beset to 0. If the price per item is not positive, it should be set to 0. Write a test program that demonstrates class Invoice’s capabilitiesarrow_forwardDesign a Class You’ll design a class named Car that has the following fields: yearModel—An Integer that holds the car’s model year make—A String that holds the make of the car speed—An Integer that holds the car’s current speed The class should have the following constructor and other methods: The constructor should accept the car’s model year and make as arguments. These values should be assigned to the object’s yearModel and make fields. The constructor should also assign 0 to the speed field. Design appropriate accessor methods to get the values stored in an object’s yearModel, make, and speed fields. The accelerate method should add 5 to the speed field each time it’s called. The brake method should subtract 5 from the speed field each time it’s called. Part 2: Design a Program You’ll create both pseudocode and a flowchart to design a program that creates a Car object and then calls the accelerate method five times. Then, call the brake method five times. After each call to the…arrow_forward
- JAVA PROGRAMMING: Lesson – Overloading Constructors Create a class named House that includes data fields for the number of occupants and the annual income as well as methods named setOccupants(), setIncome(), getOccupants() and getIncome() that set and return those values respectively. Additionally, create a constructor that requires no arguments and automatically sets the occupants field to 1 and income field to 0. Create an additional overloaded constructor . This constructor receives an integer argument and assigns the value to the occupants field. Create a third overloaded constructor this time, the constructor receives 2 arguments, the values of which are assigned to the occupants and income fields respectively. Create another class named I_house that instantiates the House class and see if the constructors work correctly.arrow_forwardDesign a class named Month. The class should have the following private members: • name - A string object that holds the name of a month, such as "January", "February", etc. • monthNumber - An integer variable that holds the number of the month. For example, January would be 1, February would be 2, etc. Valid values for this variable are 1 through 12. In addition, provide the following member functions: • A default constructor that sets monthNumber to 1 and name to "January." • A constructor that accepts the name of the month as an argument. It should set name to the value passed as the argument and set monthNumber to the correct value. • A constructor that accepts the number of the month as an argument. It should set monthNumber to the value passed as the argument and set name to the correct month name. • Appropriate set and get functions for the name and monthNumber member variables. • Prefix and postfix overloaded ++ operator functions that increment…arrow_forwardCIST 1305 UNIT 10 DROP BOX ASSIGNMENT Create the class diagram and write the pseudocode for a class named Computer that holds the make, model, and amount of memory of a computer. Include methods to set the values for each data field, and include a method that displays all the values for each field.arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
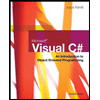
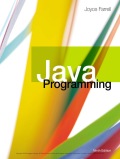