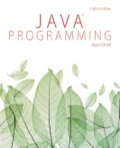
Concept explainers
Explanation of Solution
Program:
File name: “Purchase.java”
//Define a class named Purchase
public class Purchase
{
//Declare the private variables
private int invoiceNumber;
private double saleAmount;
private double tax;
private static final double RATE = 0.05;
//Define a set method that takes the invoice number
public void setInvoiceNumber(int num)
{
//Assign the value
invoiceNumber = num;
}
//Define a set method that takes the amount of sale
public void setSaleAmount(double amt)
{
//Assign the value
saleAmount = amt;
//Compute the sales tax
tax = saleAmount * RATE;
}
//Define a get method that returns the amount of sale
public double getSaleAmount()
{
//Return the value
return saleAmount;
}
//Define a get method that returns the invoice number
public int getInvoiceNumber()
{
//Return the value
return invoiceNumber;
}
/*Define a method to display the invoice number,
amount of sale, and amount of sales tax*/
public void display()
{
//Print the result
System.out.println("Invoice #" + invoiceNumber +
" Amount of sale: $" + saleAmount + " Tax: $" + tax);
}
}
File name: “SortPurchasesArray.java”
//Import necessary header files
import java.util.Scanner;
//Define a class named SortPurchasesArray
public class SortPurchasesArray
{
//Define a main method
public static void main(String[] args)
{
//Declare an array of five Purchase objects
Purchase[] purchases = new Purchase[5];
//Declare the variables
int i;
String message;
char choice;
final char QUIT = 'Z';
int number;
double price;
//Create an object for Scanner class
Scanner keyboard = new Scanner(System.in);
//For loop to be executed until i exceeds 5
for(i = 0; i < purchases.length; ++i)
{
//Prompt the user to enter the invoice number
System.out.print("Enter invoice number >> ");
number = keyboard.nextInt();
//Prompt the user to enter the amount of sale
System.out.print("Enter sale amount >> ");
price = keyboard.nextDouble();
purchases[i] = new Purchase();
//Function call
purchases[i].setInvoiceNumber(number);
purchases[i].setSaleAmount(price);
}
keyboard.nextLine();
/*Prompt the user to enter whether the Purchase objects
should be sorted and displayed in invoice number
order or sale amount order*/
System.out.print("\nSort Purchases by (I)nvoice number, or (S)ale amount? ");
choice = keyboard.nextLine()...

Want to see the full answer?
Check out a sample textbook solution
- I got part of this code right but the other part I dont this is the question - In the exercises in Chapter 6, you created a class named Purchase. Each Purchase contains an invoice number, amount of sale, amount of sales tax, and several methods. Now, write a program that declares an array of five Purchase objects and prompt a user for their values. As each Purchase object is created, continuously prompt until the user enters an invoice number between 1000 and 8000 inclusive and a non-negative sale amount. Prompt the user for values for each object and then display all the values. This is the code I have - public class Purchase { private int invoiceNumber; private double saleAmount; private double tax; private static final double RATE = 0.05; public void setInvoiceNumber(int num) { invoiceNumber = num; } public void setSaleAmount(double amt) { saleAmount = amt; tax = saleAmount * RATE; } public double getSaleAmount() { return…arrow_forwardWrite a class called AnalyzeNumbers which asks the user to enter the number of inputs and stores them in array, then prints the total numbers greater than the first inputted number: please show your code and console. Also refer to the pic.arrow_forwardDefine an array to be a 121 array if all elements are either 1 or 2 and it begins with one or more 1s followed by a one or more 2s and then ends with the same number of 1s that it begins with. Write a method named is121Array that returns 1 if its array argument is a 121 array, otherwise, it returns 0. There is one additional requirement. You should return 0 as soon as it is known that the array is not a 121 array; continuing to analyze the array would be a waste of CPU cycles. If you are programming in Java or C#, the function signature isint is121Array(int[ ] a) If you are programming in C or C++, the function signature isint is121Array(int a[ ], int len) where len is the number of elements in the array a. Examples a is then function returns reason {1, 2, 1} 1 because the same number of 1s are at the beginning and end of the array and there is at least one 2 in between them. {1, 1, 2, 2, 2, 1, 1} 1 because the same number of 1s are at the beginning and end of…arrow_forward
- please use the name, phonenumber and phonebookentry to build this. The three parallel arrays are gone — replaced with a single array of type PhonebookEntry. You should be reading in the entries using the read method of your PhonebookEntry class (which in turn uses the read methods of the Name and PhoneNumber classes). Use the equals methods of the Name and PhoneNumber classes in your lookup and reverseLookup methods. Use the toString methods to print out information. Make 100 the capacity of your Phonebook array Throw an exception (of class Exception) if the capacity of the Phonebook array is exceeded. Place a try/catch around your entire main and catch both FileNotFoundExceptions and Exceptions (remember, the order of appearance of the exception types in the catch blocks can make a difference). Do not use BufferedReader while(true) breaks The name of your application class should be Phonebook. Also, you should submit ALL your classes (i.e., Name, Strip off public from all your class…arrow_forwardIn the Utils class provided to you in this exercise, please fill in the details for a method called swapArray(). You can either upload a file or use the Edit tab to submit your work. The VPL tester will pass an arbitrary array of type integer to your method, along with the value of the locations in the array of two values that need to be swapped (exchanged). For instance, in the illustration below, the method swapArray is told to swap the values in positions 0 and 2 of an array made up of {10, 20, 30, 40, 50}. The three input parameters to the method are the array, as well as the two array indices (i and j) that control which elements to swap. Your task is to write the contents of the method that can execute this swap.arrow_forwardNeed help writing the following method: getRegisteredStudents() – returns an array of type Student[], namely the registered Students. The length of the array is the current size (number of students) of the course. public Student[] getRegisteredStudents() { // insert your solution here and modify the return statement return null; } FOR CONTEXT public class Course { public String code; public int capacity; public SLinkedList<Student>[] studentTable; public int size; public SLinkedList<Student> waitlist; public Course(String code) { this.code = code; this.studentTable = new SLinkedList[10]; this.size = 0; this.waitlist = new SLinkedList<Student>(); this.capacity = 10; } public Course(String code, int capacity) { this.code = code; this.studentTable = new SLinkedList[capacity]; this.size = 0; this.waitlist = new SLinkedList<>();…arrow_forward
- Create a program whose Main() method declares an array of 10 integers. Call a method to interactively fill the array with any number of values up to 10 or until a sentinel value is entered. If an entry is not an integer, re-prompt the user. Call a second method that accepts out parameters for the highest value in the array, lowest value in the array, sum of the values in the array, and arithmetic average. In the Main() method, display all the statistics.arrow_forwardUse a two-dimensional, multi-dimensional array and pass it to a method and receive it back. You will create the array, add data, send it to a method to be updated and return it.arrow_forwardIn this exercise you will write a program to compute the distance between any two geo locations. In this program you will ask the user for four numbers. starting latitude starting longitude ending latitude ending longitude Then, using the GeoLocation class and our earlier example as a reference, compute the distance in miles between the two locations. A sample program run should match exactly as below: Enter the latitude of the starting location: 48.8567 Enter the longitude of the starting location: 2.3508 Enter the latitude of the ending location: 51.5072 Enter the longitude of the ending location: 0.1275 The distance is 208.08639358288565 miles. setInputs(String[]) should be included /* * This class stores information about a location on Earth. Locations are * specified using latitude and longitude. The class includes a method for * computing the distance between two locations. * * This implementation is based off of the example from Stuart Reges at * the University of…arrow_forward
- Add a method called multiplesOfFive to the Exercise class. The method must have a void return type and take a single int parameter called limit. In the body of the method, write a while loop that prints out all multiples of 5 between 10 and limit (inclusive) on a single line. For instance, if the value of limit were 15 then the method will print: 10 15 You can use the printEvenNumbers method that is already in the Exercise class as an example to help you work out how to complete this method. In the main method of the Main class, add a call on the Exercise object to your multiplesOfFive method that prints the multiples of 5 between 10 and 25. Add a method called sum to the Exercise class. The method must have a void return type and take no parameters. In the body of the method, write a while loop to sum the values 1 to 10 and print the sum once the loop has finished. In the main method of the Main class, add a call on the Exercise object to your sum method. Check that it prints: 55 Add…arrow_forwardIn java i have some code for a method that finds the index of an integer that is passed in thet int is (target) the method also has the array called (array) and the first and last element of the array. also for this method to work the array has to be in descending order "static int find(int [] array, int first, int last, int target)" thats the method with all the variables that are passed to it my guess is that the first if statement just will have a short line that is "return -1;" because since the array has to be in descending order the first element cant be greater then the last element in the array but im confused on the rest because i cant find a way to make them one line codes with the conditions that i have to meet in the comments any help would be aprreaciatedarrow_forwardAnother way to write this java applacation? Write an application that inputs five numbers, each between 10 and 100, inclusive. As each number is read, display it only if it’s not a duplicate of a number already read. Provide for the “worst case,” in which all five numbers are different. Use the smallest possible array to solve this problem. Display the complete set of unique values input after the user enters each new value. Here is the code I have I just want to see another way it could be written. import java.util.Scanner; public class DuplicateElimination { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); int arr[] = new int[5]; int size = 0, n; for(int i = 0;i<5;i++){ System.out.print("Enter an integer between 10 and 100:"); n = scanner.nextInt(); if(size==0 || elemanVarmı(arr,size,n)){ System.out.println("This is the first time "+n+" has been entered");…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
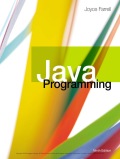
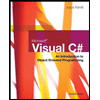
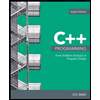