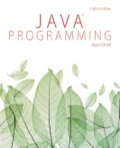
Explanation of Solution
Program:
File name: “SalespersonDatabase.java”
//Import necessary header files
import java.util.*;
//Define a class named SalespersonDatabase
public class SalespersonDatabase
{
//Define main method
public static void main(String[] args)
{
//Create an array of objects
SalesPerson[] salespeople = new SalesPerson[20];
//Declare variables
int x;
int id;
int count = 0;
double sales;
final int QUIT = 999;
char option;
String message = "";
//Create an object for Scanner class
Scanner keyboard = new Scanner(System.in);
//Prompt the user to enter an option
System.out.print("Do you want to (A)dd, (D)elete, or (C)hange a record or (Q)uit >> ");
option = keyboard.nextLine().charAt(0);
//While condition exists if option entered by the user is not equal to Quit
while(option != 'Q')
{
//If option is to Add a record
if(option == 'A')
count = addOption(salespeople, count);
//If option is to Delete a record
else
if(option == 'D')
count = deleteOption(salespeople, count);
//If option is to Change a record
else
if(option == 'C')
changeOption(salespeople, count);
//Invalid
else
//Print the result
System.out.println("Invalid entry");
//Prompt the user to enter an option
System.out.print("Do you want to (A)dd, (D)elete, or (C)hange a record or (Q)uit >> ");
option = keyboard.nextLine().charAt(0);
}
}
//Define a method for add option
public static int addOption(SalesPerson[] array, int count)
{
//Create an object for Scanner class
Scanner keyboard = new Scanner(System.in);
//Declare variables
int id;
double sales;
boolean alreadyEntered;
//Check if
if(count == array.length)
//Print the result
System.out.println("Sorry - array is full -- cannot add a record");
//if database is not full
else
{
//Prompt the user to enter the ID of the salesperson
System.out.print("Enter salesperson ID >> ");
id = keyboard.nextInt();
alreadyEntered = false;
for(int x = 0; x < count; ++x)
//Check if ID number already exists
if(array[x].getId() == id)
{
//Print the result
System.out.println("Sorry -- ID number already exists");
alreadyEntered = true;
}
//Check if ID number does not exists
if(!alreadyEntered)
{
//Prompt the user to enter the sales
System.out.print("Enter sales >> ");
sales = keyboard.nextDouble();
array[count] = new SalesPerson(id, sales);
//Increment the count
++count;
}
}
//Display the database values
display(array, count);
keyboard.nextLine();
//Return the value
return count;
}
//Define a method for delete option
public static int deleteOption(SalesPerson[] array, int count)
{
//Create an object for Scanner class
Scanner keyboard = new Scanner(System.in);
//Declare variables
int id;
int position = 0;
//Check if database is empty
if(count == 0)
//Print the result
System.out.println("Cannot delete - no records in database");
//if database is not empty
else
{
//Prompt the user to enter the ID of the salesperson
System.out.print("Enter ID to delete >> ");
id = keyboard.nextInt();
boolean exists = false;
for(int x = 0; x < count; ++x)
//Check if ID number already exists
if(array[x].getId() == id)
{
exists = true;
position = x;
}
//if ID number does not exists
if(!exists)
//Print the result
System...

Trending nowThis is a popular solution!

- In Chapter 11, you created the most recent version of the MarshallsRevenue program, which prompts the user for customer data for scheduled mural painting. Now, save all the entered data to a file that is closed when data entry is complete and then reopened and read in, allowing the user to view lists of customer orders for mural types.arrow_forward1. In this lab, you will read two files. Firstly, the ‘AccountData.csv’ file has the name and account number.Use each account number to scan the ‘BankData.csv’ file for a matching account number to get a ‘balance’from the account transactions. You must repeatedly open, read the ‘BankData.csv’ file and close it for eachaccount number in the ‘AccountData.csv’ file unless you wish to store its entire contents in a static array.(More code and may not be enough time to complete.)2. So, for this lab, read my pseudocode in the given java files FIRST!!3. ANALYZE my code carefully.4. Then start coding the solution. I have given the solution to each lab instructor to help you during the timefor your specific lab.5. You will submit in iCollege, ONLY one file: BankDataProcessing.java6. No need to submit the data files or the BankAccount ‘object’ file.7. Do NOT MODIFY the ‘BankAccount.java’ file. PLEASE COMPLETE THE JAVA CODE BELOW AND MAKE SURE ITS LiKE THE OUTPUTimport java.io.*;import…arrow_forward1. In this lab, you will read two files. Firstly, the ‘AccountData.csv’ file has the name and account number.Use each account number to scan the ‘BankData.csv’ file for a matching account number to get a ‘balance’from the account transactions. You must repeatedly open, read the ‘BankData.csv’ file and close it for eachaccount number in the ‘AccountData.csv’ file unless you wish to store its entire contents in a static array.(More code and may not be enough time to complete.)2. So, for this lab, read my pseudocode in the given java files FIRST!!3. ANALYZE my code carefully.4. Then start coding the solution. I have given the solution to each lab instructor to help you during the timefor your specific lab.5. You will submit in iCollege, ONLY one file: BankDataProcessing.java6. No need to submit the data files or the BankAccount ‘object’ file.7. Do NOT MODIFY the ‘BankAccount.java’ file. import java.io.*;import java.nio.file.Files;import java.nio.file.Path;import java.nio.file.Paths;import…arrow_forward
- Create an array of objects of the Person class, of size 4. Create three objects of the Person class, with values, and assign the objects to the array. Loop through the array and print the name, job, and email of all Personobjects. (using the Netbeans application)arrow_forwardPlease allow the buttons to sort properly the sortByname, sortbywaittime and capacity to all sort please // Constructor that takes a Driver object as input public EntryScreen(Driver reservationlist1) { this.reservationlist1 = reservationlist1; // Initialize the necessary components txttableNumber = new JTextField(20); setTitle("Make a Reservation"); pnlCommand = new JPanel(); pnlDisplay = new JPanel(); String[] columnNames = {"ID", "Name", "Capacity", "Date", "Time"}; tableModel = new DefaultTableModel(columnNames, 0); table = new JTable(tableModel); JScrollPane scrollPane = new JScrollPane(table); add(scrollPane, BorderLayout.NORTH); // create the table and set the model // add the table to a scroll pane and add it to the GUI // add labels and text fields to the display panel pnlDisplay.add(new JLabel("Name:")); txtName = new JTextField(20);…arrow_forwardAssuming that M is already a variable in the workspace and M contains numeric data in a matrix format, which of the following lines of code changes the value of M? Write your answer in a comment in your m file. List all correct answers. There could be more than one. M(:) x = M(end,:) M = M’ transpose(M) M = 1:10arrow_forward
- What is printed to the console when the code that follows is executed? ArrayList<String> passwords = new ArrayList<>(); passwords.add("akjdk12"); passwords.add("buujl32"); passwords.add("chrcl92"); passwords.add("nnnii87"); passwords.set(2, "cb2kr45"); passwords.remove("akjdk12"); System.out.println(passwords.get(1));arrow_forwardThe intNums array contains six elements. Which of the following increases the number of elements to eight?a. ReDim Preserve intNums(2)b. ReDim Preserve intNums(7) c. ReDim intNums(2)d. Preserve ReDim intNums(8)arrow_forwardDetermine if the following statement are true or false screen shot shows the text's SortedArrayCollection and ArrayCollection When comparing two objects using the == operator, what is actually compared is the references to the objects. When comparing two objects using the equals method inherited from the Object class, what is actually compared is the references to the objects. The text's sorted array-based collection implementation stores elements in the lowest possible indices of the array. The text's array-based collection implementation stores elements in the lowest possible indices of the array.arrow_forward
- MongoDB 1) The customers collection includes documents that have accounts as a field that contains an array of values. Write a statement to retrieve a cursor containing all documents where there is only one account. 2) Write a statement that returns only the email addresses of all customer documents. 3) Write a statement to retrieve a cursor containing all customer documents where the active field within the main document (i.e., do not worry about nested documents) is set to true. 4) Write a statement to retrieve a cursor containing all customer documents that do not have the active field within the main document (i.e., do not worry about nested documents). (Hint: Take a look at the Query and Projection operators in the MongoDB documentation to find an operator that helps determine whether a field exists within a document.)arrow_forwardRight now we have a CourseListType that will hold a static 50 courses. Now we need to make that a little more flexible. To do that we want to change the static array to a dynamic array. So, what do we need to do? Modify CourseListType so that it contains a pointer to a CourseType rather than an array of CourseType. Modify the CourseListType constructor to create a dynamic array allocating space for a specific number of CourseType elements rather than always using 50. (Note: This is still not totally flexible, but is much better than a static limit.) Finally, we need to create a destructor to for CourseListType to deallocate space whenever a declared object goes out of scope.) //courseListType.h file #ifndef COURSELISTTYPE_H_INCLUDED#define COURSELISTTYPE_H_INCLUDED #include <string>#include "courseType.h" class courseTypeList { public:void print() const;void addCourse(std::string);courseTypeList(); private:static const int NUM_COURSES = 50;int courseCount;courseType…arrow_forwardTrue or FalseAn application works directly with a database, modifying rows, deleting rows, adding new rows, and so forth.arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
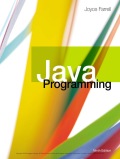
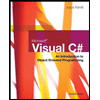
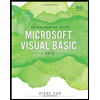