maze
.h
keyboard_arrow_up
School
Stanford University *
*We aren’t endorsed by this school
Course
120
Subject
Computer Science
Date
Oct 30, 2023
Type
h
Pages
4
Uploaded by JusticeKouprey15684
/**
* @file maze.h
* Square Maze implementation.
* @date 12/07/2009
* @date 12/08/2009
*/
#pragma once
#include <vector>
#include "cs225/PNG.h"
#include "cs225/HSLAPixel.h"
#include "dsets.h"
#include <utility>
#include <random>
#include <iostream>
/**
* Each SquareMaze object represents a randomly-generated square maze and its
*
solution.
*
* (Note that by "square maze" we mean a maze in which each cell is a square;
*
the maze itself need not be a square.)
*/
class SquareMaze
{
private:
int size_;
std::mt19937 gen; // Defining Gen as private member var
int mazeWidth;
int mazeHeight;
DisjointSets cellData;
std::vector<std::vector<std::pair<bool, bool>>> wallData;
// For backtracking & maze solution
std::vector<std::vector<bool>> isVisited;
// cs225::PNG mazeImage;
public:
// My Own
//bool checkPoint(int neighborX, int neighborY) const;
bool hasNoCycle(int x, int y) ;
int calculateIndex(int current, int peek) const;
/**
* No-parameter constructor.
* Creates an empty maze.
*/
SquareMaze();
/**
* Makes a new SquareMaze of the given height and width.
*
* If this object already represents a maze it will clear all the
* existing data before doing so. You will start with a square grid (like
* graph paper) with the specified height and width. You will select
* random walls to delete without creating a cycle, until there are no
* more walls that could be deleted without creating a cycle. Do not
* delete walls on the perimeter of the grid.
*
* Hints: You only need to store 2 bits per square: the "down" and
* "right" walls. The finished maze is always a tree of corridors.)
*
* @param width The width of the SquareMaze (number of cells)
* @param height The height of the SquareMaze (number of cells)
*/
void makeMaze(int width, int height);
/**
*
* This uses your representation of the maze to determine whether it is
* possible to travel in the given direction from the square at
* coordinates (x,y).
*
* For example, after makeMaze(2,2), the possible input coordinates will
* be (0,0), (0,1), (1,0), and (1,1).
*
* - dir = 0 represents a rightward step (+1 to the x coordinate)
* - dir = 1 represents a downward step (+1 to the y coordinate)
* - dir = 2 represents a leftward step (-1 to the x coordinate)
* - dir = 3 represents an upward step (-1 to the y coordinate)
*
* You can not step off of the maze or through a wall.
*
* This function will be very helpful in solving the maze. It will also
* be used by the grading program to verify that your maze is a tree that
* occupies the whole grid, and to verify your maze solution. So make
* sure that this function works!
*
* @param x The x coordinate of the current cell
* @param y The y coordinate of the current cell
* @param dir The desired direction to move from the current cell
* @return whether you can travel in the specified direction
*/
bool canTravel(int x, int y, int dir) const;
/**
* Sets whether or not the specified wall exists.
*
* This function should be fast (constant time). You can assume that in
* grading we will not make your maze a non-tree and then call one of the
* other member functions. setWall should not prevent cycles from
* occurring, but should simply set a wall to be present or not present.
* Our tests will call setWall to copy a specific maze into your
* implementation.
*
* @param x The x coordinate of the current cell
* @param y The y coordinate of the current cell
* @param dir Either 0 (right) or 1 (down), which specifies which wall to
* set (same as the encoding explained in canTravel). You only need to
* support setting the bottom and right walls of every square in the grid.
* @param exists true if setting the wall to exist, false otherwise
*/
void setWall(int x, int y, int dir, bool exists);
/**
* Solves this SquareMaze.
*
* For each square on the bottom row (maximum y coordinate), there is a
* distance from the origin (i.e. the top-left cell), which is defined as
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
please help debuging...
// Fix the bugs so that the report of 5 students grades// is correct. There should be no memory leaks.#include <stdio.h>#include <string.h>#include <stdlib.h>#include <assert.h>
typedef struct student{ int grade; char* nameID;}student_t;
void CreateStudent(student_t* s, int grade, char* nameID){ s->grade = grade; s->nameID = strdup(nameID); }
//float ComputeAverageGrade(student_t* class, int sizeOfClass){ assert(sizeOfClass > 0 && "Must have at least 1 student"); float total=0.0f; for(int i=0; i <= 5; i++){ total+= class[i].grade; } return total / sizeOfClass;}
int main(){
// Create our classroom of 5 students student_t* class = (student_t*)malloc(sizeof(student_t)*5);
for(int i=0; i <5 ; i++){ // Assume all nameID's are "temporary" CreateStudent(&class[i],90,"temporary"); }
// Storage for students grades float averageGrade =…
arrow_forward
SKELETON CODE IS PROVIDED ALONG WITH C AND H FILES.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdbool.h>
#include "node.h"
#include "stack_functions.h"
#define NUM_VERTICES 10
/** This function takes a pointer to the
adjacency matrix of a Graph and the
size of this matrix as arguments and
prints the matrix
*/
void print_graph(int * graph, int size);
/** This function takes a pointer to the
adjacency matrix of a Graph, the size
of this matrix, the source and dest
node numbers along with the weight or
cost of the edge and fills the adjacency
matrix accordingly.
*/
void add_edge(int * graph, int size, int src, int dst, int cost);
/** This function takes a pointer to the adjacency matrix of
a graph, the size of this matrix, source and destination
vertex numbers as inputs and prints out the path from the
source vertex to the destination vertex. It also prints
the total cost of this…
arrow_forward
public class Facility implements Iterable<String> { protected String name;
}
protected class StorageUnit { public String unitId; public ArrayList<String> items; public StorageUnit next; public StorageUnit thread_next; public StorageUnit(String unitId) { this.unitId = unitId; this.items = new ArrayList<String>(); next = null;
}
public void addItem(String singleItem) { items.add(singleItem);
} }
protected StorageUnit head; protected int totalUnits; protected StorageUnit thread_head;
}
Please provide implementation for the setThread method to accompany the above code.
Method description:
public void setThread(int minimumItemsInUnit) - RECURSIVE METHOD - This method initializes thread_head and thread_next to form a list with those nodes from the original list that have at least a number of items that corresponds to the parameter. This method must be recursive. The instance variable thread_head must point to the first node with at least a number of items that correspond…
arrow_forward
OCaml Code: The goal of this project is to understand and build an interpreter for a small, OCaml-like, stackbased bytecode language. Make sure that the code compiles correctly and provide the code with the screenshot of the output. The code must have a function that takes a pair of strings (tuple) and returns a unit. You must avoid this error that is attached as an image. Make sure to have the following methods below:
-Push integers, strings, and names on the stack
-Push booleans
-Pushing an error literal or unit literal will push :error: or :unit:onto the stack, respectively
-Command pop removes the top value from the stack
-The command add refers to integer addition. Since this is a binary operator, it consumes the toptwo values in the stack, calculates the sum and pushes the result back to the stack
- Command sub refers to integer subtraction
-Command mul refers to integer multiplication
-Command div refers to integer division
-Command rem refers to the remainder of integer…
arrow_forward
void getInput(){for(int i =0; i < studentName.length; i++){System.out.print("Student name: ");studentName[i] = keyboard.nextLine();System.out.print("Studnet ID: ");midTerm1[i] = keyboard.nextInt();}keyboard.close();}
Can't put full student name becuase nextLine();
arrow_forward
Find logic errors:
#include <Adafruit_NeoPixel.h>#include <string.h>
#define NEOS 8#define NPX 10#define AVGS 100
int runsamps[AVGS];double curavg;int count;
Adafruit_NeoPixel pixels(NPX, NEOS, NEO_GRB+NEO_KHZ800);
void setup() {// put your setup code here, to run once:pixels.begin();memset(runsamps, 0, AVGS*sizeof(int));count = 0;curavg = 50;}
void loop() {// put your main code here, to run repeatedly:int newdata;int scaleavg;pixels.clear();newdata = analogRead(8);curavg += (double)runsamps[count]/AVGS;runsamps[count] = newdata;curavg -= (double)newdata/AVGS;scaleavg = round(map(curavg, 0, 1023, 0, 10));for (int n = 0; n < scaleavg; n++){pixels.setPixelColor(n, pixels.Color(82, 35, 152));pixels.show();}delay(10);count++;}
arrow_forward
Build a software system that tracks time in individual disciplines while training for a triathlon, and allows the user to add or modify entries. The user interface should look like this:
•You will need:
•Vectors
•Menus (switch statements)
•Loops
•Functions
•IO formatting
•First phase
•Read in a vector of swim times
•Call a function to print the vector of swim times
•Hints: use while (in >>) loop, use vector.push_back();
•Second phase
•Finish this in lab Friday
•Read in a vector of swim times
•Call the display function
•Ask the user for a time to add and add it
•Ask the user for a time to modify and change it
•Call the display function
•Write out the modified vector to a file
•Hints: use push_back() to add a time, use [] to change a time
•Third phase
•Read in a vector of swim times
•Build a loop, until quit is true:
•Display the vector
•Ask the user for an action (add, modify, quit)
•Use a switch to select the action
•Execute the action
•Write out the changed vector…
arrow_forward
//import java.util.List;
import javax.swing.*;
import javax.swing.event.*;
import java.awt.*;
/** * JList basic tutorial and example * * @author wwww.codejava.net */
public class JListTask1_Student_Version extends JFrame {
//define a Jlist Component
// Define a String array that use to create the list
private JPanel ContryPanel;
// To hold components private JPanel selectedcontryPanel; // To hold components
// define a TextFiles to show the result
private JLabel label; // A message
/** Constructor */
public JListTask1_Student_Version(){
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setTitle("JList Task1");
setSize(200, 200);
setLayout(new BorderLayout());
// Build the month and selectedMonth panels. // Add the panels to the content pane. //
add(new JScrollPane(countryList)); setLocationRelativeTo(null);
setVisible(true); }
public void buildcontryPanel() {
// Create a panel to hold the list.
ContryPanel = new JPanel();
//create the list // Set the selection mode to single…
arrow_forward
Computer Science
Write a program in the Java language that includes: 1. A class for books, taking into account the encapsulation and getter, setter. 2. Entering books by the user using Scanner 3. LinkedList class for: (Adding a new book - Inserting a book- Searching for a book using the ISBN Number - Viewing all books,delete book). 4. Serial number increases automatically with each book that is entered. 5. Printing the names of books is as follows: Serial - ISBN - Name - PubYear - Price - Notes. 6-Design a main menu that includes: • Add Book • Insert Book • Delete Book • Search . Display . Exit
arrow_forward
Deliverable #2: How many operations in nested for loops?
Implement the following psuedocode as the function two_dimensional_loop (n) in the cell below:
count = 0
for i = 0 to n-1 :
for j = i to n-1 :
count = count + 1
return count
► def two_dimensional_loop(n) : # complete to satisfy the instructions and implement the pseudocode
||
return 0
expectEqual (two_dimensional_loop (520),135460)
expectEqual (two_dimensional_loop (1234), int(1234* (1234+1)/2))
个
↓
arrow_forward
Jordan University of Science and TechnologyCS415 Contemporary Programming TechniquesMidterm Exam – Second semester 2021Name: ID: Section:Important note: your code must compile correctly and run without errors.Otherwise your code will get the grade zero.Question 1: Implement a java class called ParsingCustomerRecords that has thefollowing data members and functions:ParsingCustomerRecods- customerRecords: array of strings- result: string variable- recordsNumber: int variable-fileName: string variable+ Constructor(String filename, int recordsNumber)+ void Reader()+ void Executor()+ void showResult()+ String toString()All of the data members are private, and all of the methods are public.Constructor has two parameters which are (1) the file name in which Customers’ recordsare stored, and (2) the number of the records in the file. The constructor functionality is toassign suitable initial values to the fileName and recordsNumber data members. Theconstructor also should instantiate the…
arrow_forward
API documentation link ------> https://docs.oracle.com/en/java/javase/11/docs/api/java.base/java/util/ArrayList.html
PREVIOUS CODE -
import java.util.ArrayList;public class BasicArrayList { // main method
public static void main(String[] args) {
// create an arraylist of type Integer
ArrayList<Integer> basic = new ArrayList<Integer>();
// using loop add elements
for(int i=0;i<4;i++){
basic.add(i+1);
}
// using loop set elements
for(int i=0;i<basic.size();i++){
basic.set(i,(basic.get(i)*5));
}
// using loop print elements
for(int i=0;i<basic.size();i++){
System.out.println(basic.get(i));
}
}}
Q1)
a)Consider how to remove elements from the ArrayList. Give two different lines of code that would both remove 5 from the ArrayList.
b)What do you think the contents of the ArrayList will look like after the 5 has been removed? (Sketch or list)
c)Now update the BasicArrayList program to remove the 5 (index 1) from the ArrayList. Add this step before the last loop that…
arrow_forward
C CodeApproved Libraries:<stdio.h><math.h><stdlib.h><time.h> (for srand(time(0)) only)
arrow_forward
********Answer question below:*******
Create UML Class Diagram and java source file.
+add //addLast
+add(index) //addFirst
+remove //removeLast
+remove(key) //removeFirst
+search(key)
+print()
//removeDuplicate
arrow_forward
Step1- Write a Java program in Netbeans that implements parallel programming using the Fork/Join framework. The program should initially generate 15 million random numbers. This should be read into an array of doubles.
Step 2- Then it should make use of a method that uses this array to calculate the sum of these doubles.
Step 3-This program should make use of the subclasses in the Fork/Join Framework abd should also output the number of processors available.
Step 4 - Have a clear screenshoot of code in the IDE and a screenshot of the code running.
arrow_forward
C CodeApproved Libraries:
<stdio.h><math.h><stdlib.h><time.h> (for srand(time(0)) only)
arrow_forward
PLEASE HELP, PYTHON THANK YOU
class Graph(object): def __init__(self, graph_dict=None): ################# # Problem 1: # Check to see if the graph_dict is none # if so, create an empty dictionary and store it in graph_dict ################## #DELETE AND PUT IN THE IF AND ASSIGNMENT STATEMENTS self.__graph_dict = graph_dict ################# # Problem 2: # Create a method called vertices and pass in self as the parameter ################## #DELETE AND PUT IN THE METHOD DEFINITION """ returns the vertices of a graph """ return list(self.__graph_dict.keys()) def edges(self): """ returns the edges of a graph """ ################# # Problem 3: # Return the results of the __generate_edges ################## #DELETE AND PUT IN THE RETURN STATEMENTS
arrow_forward
in python
class name: Runner
function within a class:
def __getitem__(self, lhs: Union[int. list[bool]]) -> Union[float, Runner]:
The function should take a list[bool] and will return a new Runner object containing only the filtered values
Example :
c = Runner([1.0, 2.0, 3.0, 4.0, 2.0, 1.0])
filter = c > 2.0
print(filter) # Output: [False, True, True, False, False])
d = c[filter]
print(d) # Output: Runner([3.0, 4.0])
arrow_forward
Design and implement a service that simulates PHP loops. Each of the three loop variants should be encapsulated in an object. The service can be controlled via a parameter and execute three different simulations. The result is returned as JSON.
The input is an array consisting of the letters $characters = [A-Z].
-The For loop should store all even letters in an array.-The Foreach loop simulation is to create a backward sorted array by For loop, i.e. [Z-A] .-The While loop should write all characters into an array until the desired character is found.
Interface:-GET Parameter String: loopType ( possible values: REVERSE, EVEN, UNTIL )-GET parameter String: until (up to which character)
Output:JSON Object: {loopName: <string>, result: <array> }
arrow_forward
Dijkstra's algorithm Weight Class Implementationlanguage : C++
Note : Skelton Files Provided
Please check the linksproject Details:https://ece.uwaterloo.ca/~dwharder/aads/Projects/5/Dijkstra/
Files:https://ece.uwaterloo.ca/~dwharder/aads/Projects/5/Dijkstra/src/
Please provide clear and complete solution for thumps up.
arrow_forward
public class CustomLinkedList { public static int findMax(IntNode headObj) { /* Type your code here */ } public static void main(String[] args) { IntNode headObj; IntNode currObj; IntNode lastObj; int i; int max; // Create head node headObj = new IntNode(-1); lastObj = headObj; // Add nodes to the list for (i = 0; i < 20; ++i) { currObj = new IntNode(i); lastObj.insertAfter(currObj); lastObj = currObj; } max = findMax(headObj); System.out.println(max); }}
arrow_forward
Turtle Hare Race - Multithreading
Use Java multithreading to implement the turtle hare race program. You are not allowed to use Timer events. Your program should have one thread for turtle, one thread for hare, and one dedicated thread for periodic painting.
arrow_forward
C++
A robot is initially located at position (0; 0) in a grid [?5; 5] [?5; 5]. The robot can move randomly in any of the directions: up, down, left, right. The robot can only move one step at a time. For each move, print the direction of the move and the current position of the robot. If the robot makes a circle, which means it moves back to the original place, print "Back to the origin!" to the console and stop the program. If it reaches the boundary of the grid, print \Hit the boundary!" to the console and stop the program.
A successful run of your code may look like:Down (0,-1)Down (0,-2)Up (0,-1)Left (-1,-1)Left (-2,-1)Up (-2,0)Left (-3,0)Left (-4,0)Left (-5,0)Hit the boundary!
or
Left (-1,0)Down (-1,-1)Right (0,-1)Up (0,0)Back to the origin!
About: This program is to give you practice using the control ow, the random number generator, and output formatting. You may use <iomanip> to format your output. You may NOT use #include "stdafx.h".
arrow_forward
C++ in visual studio:
Implement it completely with copy constructor, overloaded assignment operator and destructor. Then, in main, type an application that creates 2 numbers, sums them, and assigns the result to a new number.
for example ;
Number 1 (18,32)
Number 2 (15.09)
- - - - - - - - - - - +
Number 3 ( 33,41)
arrow_forward
import ADTs.QueueADT;import DataStructures.ArrayStack;import ADTs.StackADT;import DataStructures.LinkedQueue;import java.util.Scanner;
/**** @author Qiong*/public class RepeatStrings {public static void main(String[] argv) throws Exception{final int SIZE = 3;Scanner keyboard = new Scanner(System.in);QueueADT<String> stringQueue;//stringQueue = new CircularArrayQueue<String>(SIZE);stringQueue = new LinkedQueue<String>();StackADT<String> stringStack;stringStack = new ArrayStack<String>(SIZE);String line;for (int i = 0; i < SIZE; i++){System.out.print("Enter a line of text which includes only 3 words > ");line = keyboard.nextLine();//TODO enque the new element//TODO push the new element}System.out.println("\nOrder is: ");for (int i = 0; i < SIZE; i++){// TODO Remove an element in the order in which we input strings// Save it to the String variable, named lineSystem.out.println(line);}System.out.println("\nOpposite order is: ");for (int i = 0; i <…
arrow_forward
def apply_gaussian_noise(X, sigma=0.1):
"""
adds noise from standard normal distribution with standard deviation sigma
:param X: image tensor of shape [batch, 3, height, width]
Returns X + noise.
"""
### YOUR CODE HERE ###
# noise tests
theoretical_std = (X_train[:100].std() ** 2 + 0.5 ** 2) ** .5
our_std = apply_gaussian_noise(X_train[:100], sigma=0.5).std()
assert abs(theoretical_std - our_std) < 0.01, \
"Standard deviation does not match it's required value. Make sure you use sigma as std."
assert abs(apply_gaussian_noise(X_train[:100], sigma=0.5).mean() - X_train[:100].mean()) < 0.01, \
"Mean has changed. Please add zero-mean noise"
arrow_forward
NOTE: DO NOT COPY FROM ANOTHER QUESTION
Directions:
1. Show the ArrayStackADT interface
2. Create the ArrayStackDataStrucClass<T> with the following methods: default constructor, overloaded constructor, copy constructor, initializeStack, isEmptyStack, isFullStack, push, peek, void pop
3. Create the PrimeFactorizationDemoClass: instantiate an ArrayStackDataStrucClass<Integer> object with 50 elements. Use a try-catch block in the main( ) using pushes/pops.
4. Exception classes: StackException, StackUnderflowException, StackOverflowException5. Show the 4 outputs for the following: 3,960 1,234 222,22213,780
arrow_forward
GuitarString.java
Create a data type to model a vibrating guitar string. Write a class named GuitarString that
implements the following API:
public class GuitarString
// Creates a guitar string of the specified frequency,
// using a sampling rate of 44,100.
public GuitarString(double frequency)
// Creates a guitar string whose length and initial values
// are given by the specified array.
public GuitarString(double[] init)
// Returns the number of samples in the ring buffer.
public int length()
// Plucks this guitar string by replacing the ring buffer with white noise.
public void pluck()
// Advances the Karplus-Strong simulation one time step.
public void tic()
// Returns the current sample.
public double sample()
// Tests this class by directly calling both constructors
// and all instance methods.
public static void main(String[] args)
arrow_forward
/** To change this license header, choose License Headers in Project Properties.* To change this template file, choose Tools | Templates* and open the template in the editor.*/
package Linked_List;
import java.util.Scanner;
/**** @author lab1257-15*/public class Demo {
public static boolean has42 (RefUnsortedList<Integer> t){t.reset();for (int i=1; i<=t.size();i++)if (t.getNext()==42)return true;return false;}public static void RemoveAll(RefUnsortedList<Integer> t, int v){while(t.contains(v)){t.remove(v); } }public static void main(String[] args) {}}
/** To change this license header, choose License Headers in Project Properties.* To change this template file, choose Tools | Templates* and open the template in the editor.*/
package Linked_List;/**** @author lab1257-15*/public class LLNode<T>{private LLNode<T> link;private T info;public LLNode(T info){this.info = info;link = null;}public void setInfo(T info)// Sets info of this LLNode.{this.info =…
arrow_forward
• Do not add statements that call print, input , or open , or add an import statement.
• Do not use any break or continue statements. We are imposing this restriction (and we have not even taught you
these statements) because they are very easy to abuse, resulting in terrible, hard to read code.
• Do not modify or add to the import statements provided in the starter code.
import math
from typing import List, TextIO
# For simplicity, we'll use "Station" in our type contracts to indicate that
# we mean a list containing station data.
# You can read "Station" in a type contract as:
List[int, str, float, float, int, int, int]
23
# where the values at each index represent the station data as described in the
# handout on Quercus.
# A set of constants, each representing a list index for station information.
ID - 0
NAME - 1
LATITUDE - 2
LONGITUDE - 3
CAPACITY - 4
BIKES_AVAILABLE - 5
DOCKS_AVAILABLE - 6
NO_KIOSK - 'SMART"
# For use in the get_lat_lon_distance helper function
EARTH RADIUS -…
arrow_forward
FIX ERRORS GIVEN IN IMAGE
#include<iostream>
#include<math.h>
#include<assert.h>
using namespace std;
typedef float f;
class vector3d
{
public:
f x,y,z;
vector3d() {
x=0;
y=0;
z=0;
}
vector3d(f x1,f y1,f z1=0) {
x=x1;
y=y1;
z=z1;
}
vector3d(const vector3d &vec);
vector3d operator+(const vector3d &vec);
vector3d &operator+=(const vector3d &vec);
vector3d operator-(const vector3d &vec);
vector3d &operator-=(const vector3d &vec);
vector3d operator*(f value); //multiplication
vector3d &operator*=(f value); //assigning new result to the vector.
vector3d &operator/=(f value);
vector3d &operator=(const vector3d &vec);
bool coplanar(vector3d p,vector3d q,vector3d r,vector3d s);
f dot_product(const vector3d &vec);
vector3d cross_product(const vector3d &vec);
f magnitude();
vector3d…
arrow_forward
package filterSort;import java.io.File;import java.io.FileNotFoundException;import java.util.Arrays;import java.util.Scanner;import java.util.*;
public class FilterSort {
/*** Allocates and returns an integer array twice the size of the one* supplied as a parameter. The first half of the new array will* be a copy of the supplied array and the second half of the new* array will be zeros.** @param arr the array to be copied* @return array twice the size of <tt>arr</tt> with its initial* elements copied from <tt>arr</tt>* @throws NullPointerException if <tt>arr</tt> is null.*/public static int[] doubleArrayAndCopy(int[] arr) {int len = arr.length;int max = arr.length * 2;int[] copyArr = Arrays.copyOf(arr,max);int length = copyArr.length-1;Arrays.sort(copyArr, 0, length);return copyArr;
}
public static void main(String[] args) {int[] data = new int[8];try {File file = new File("data.txt");Scanner myReader = new Scanner(file);for(int i = 0;…
arrow_forward
1 import java.util.Random;
2
3
4
5
6-
7
8
9
10
11
12
▼
import java.util.StringJoiner;
public class preLabC {
public static MathVector myMethod(int[] testValues) {
// Create an object of type "MathVector".
// return the Math Vector Object back to the testing script.
return VectorObject;
13
14
15 }
}
▸ Comments
Description
The MathVector.java file contains a class (Math Vector). This class allows you to create objects in your program that
can contain vectors (a 1D array) and perform actions on those vectors. This is useful for doing math operations like
you would in your linear algebra class.
Your method should evaluate against two tests.
What do you need to do in this exercise? You need to create an "instance" of the MathVector object, VectorObject,
in your method. If you do this correctly, your method will accept a 1D array of integers, testValues, feed it into the
VectorObject and then return the VectorObject.
If you look in the file MathVector.java you'll see that this is one way in…
arrow_forward
void getVectorSize(int& size); void readData(vector<Highscore>& scores); void sortData(vector<Highscore>& scores); vector<Highscore>::iterator findLocationOfLargest( const vector<Highscore>::iterator startingLocation, const vector<Highscore>::iterator endingLocation); void displayData(const vector<Highscore>& scores);
The size parameter from the given code won't be needed now, since a vector knows its own size.
Notice that the findLocationOfLargest() function does not need the vector itself as a parameter, since you can access the vector using the provided iterator parameters.
The name field in the struct must still be a c-string
The focus of this assignment is to use iterators. You must use iterators wherever possible to access the vector. As a result, you must not use square brackets, the push_back() function, the at() function, etc. Also, the word "index" shouldn't appear in your code anywhere. You won't get full credit if…
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
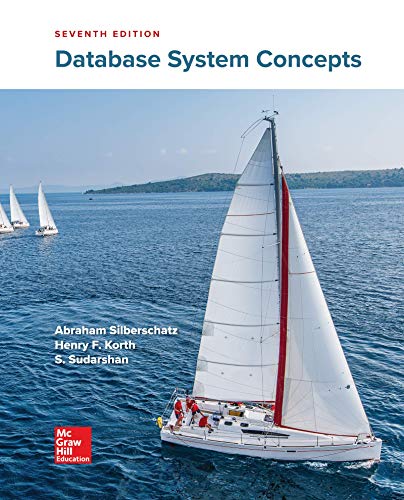
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
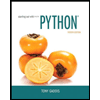
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
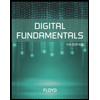
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
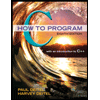
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
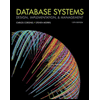
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
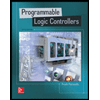
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- please help debuging... // Fix the bugs so that the report of 5 students grades// is correct. There should be no memory leaks.#include <stdio.h>#include <string.h>#include <stdlib.h>#include <assert.h> typedef struct student{ int grade; char* nameID;}student_t; void CreateStudent(student_t* s, int grade, char* nameID){ s->grade = grade; s->nameID = strdup(nameID); } //float ComputeAverageGrade(student_t* class, int sizeOfClass){ assert(sizeOfClass > 0 && "Must have at least 1 student"); float total=0.0f; for(int i=0; i <= 5; i++){ total+= class[i].grade; } return total / sizeOfClass;} int main(){ // Create our classroom of 5 students student_t* class = (student_t*)malloc(sizeof(student_t)*5); for(int i=0; i <5 ; i++){ // Assume all nameID's are "temporary" CreateStudent(&class[i],90,"temporary"); } // Storage for students grades float averageGrade =…arrow_forwardSKELETON CODE IS PROVIDED ALONG WITH C AND H FILES. #include <stdio.h> #include <stdlib.h> #include <string.h> #include <stdbool.h> #include "node.h" #include "stack_functions.h" #define NUM_VERTICES 10 /** This function takes a pointer to the adjacency matrix of a Graph and the size of this matrix as arguments and prints the matrix */ void print_graph(int * graph, int size); /** This function takes a pointer to the adjacency matrix of a Graph, the size of this matrix, the source and dest node numbers along with the weight or cost of the edge and fills the adjacency matrix accordingly. */ void add_edge(int * graph, int size, int src, int dst, int cost); /** This function takes a pointer to the adjacency matrix of a graph, the size of this matrix, source and destination vertex numbers as inputs and prints out the path from the source vertex to the destination vertex. It also prints the total cost of this…arrow_forwardpublic class Facility implements Iterable<String> { protected String name; } protected class StorageUnit { public String unitId; public ArrayList<String> items; public StorageUnit next; public StorageUnit thread_next; public StorageUnit(String unitId) { this.unitId = unitId; this.items = new ArrayList<String>(); next = null; } public void addItem(String singleItem) { items.add(singleItem); } } protected StorageUnit head; protected int totalUnits; protected StorageUnit thread_head; } Please provide implementation for the setThread method to accompany the above code. Method description: public void setThread(int minimumItemsInUnit) - RECURSIVE METHOD - This method initializes thread_head and thread_next to form a list with those nodes from the original list that have at least a number of items that corresponds to the parameter. This method must be recursive. The instance variable thread_head must point to the first node with at least a number of items that correspond…arrow_forward
- OCaml Code: The goal of this project is to understand and build an interpreter for a small, OCaml-like, stackbased bytecode language. Make sure that the code compiles correctly and provide the code with the screenshot of the output. The code must have a function that takes a pair of strings (tuple) and returns a unit. You must avoid this error that is attached as an image. Make sure to have the following methods below: -Push integers, strings, and names on the stack -Push booleans -Pushing an error literal or unit literal will push :error: or :unit:onto the stack, respectively -Command pop removes the top value from the stack -The command add refers to integer addition. Since this is a binary operator, it consumes the toptwo values in the stack, calculates the sum and pushes the result back to the stack - Command sub refers to integer subtraction -Command mul refers to integer multiplication -Command div refers to integer division -Command rem refers to the remainder of integer…arrow_forwardvoid getInput(){for(int i =0; i < studentName.length; i++){System.out.print("Student name: ");studentName[i] = keyboard.nextLine();System.out.print("Studnet ID: ");midTerm1[i] = keyboard.nextInt();}keyboard.close();} Can't put full student name becuase nextLine();arrow_forwardFind logic errors: #include <Adafruit_NeoPixel.h>#include <string.h> #define NEOS 8#define NPX 10#define AVGS 100 int runsamps[AVGS];double curavg;int count; Adafruit_NeoPixel pixels(NPX, NEOS, NEO_GRB+NEO_KHZ800); void setup() {// put your setup code here, to run once:pixels.begin();memset(runsamps, 0, AVGS*sizeof(int));count = 0;curavg = 50;} void loop() {// put your main code here, to run repeatedly:int newdata;int scaleavg;pixels.clear();newdata = analogRead(8);curavg += (double)runsamps[count]/AVGS;runsamps[count] = newdata;curavg -= (double)newdata/AVGS;scaleavg = round(map(curavg, 0, 1023, 0, 10));for (int n = 0; n < scaleavg; n++){pixels.setPixelColor(n, pixels.Color(82, 35, 152));pixels.show();}delay(10);count++;}arrow_forward
- Build a software system that tracks time in individual disciplines while training for a triathlon, and allows the user to add or modify entries. The user interface should look like this: •You will need: •Vectors •Menus (switch statements) •Loops •Functions •IO formatting •First phase •Read in a vector of swim times •Call a function to print the vector of swim times •Hints: use while (in >>) loop, use vector.push_back(); •Second phase •Finish this in lab Friday •Read in a vector of swim times •Call the display function •Ask the user for a time to add and add it •Ask the user for a time to modify and change it •Call the display function •Write out the modified vector to a file •Hints: use push_back() to add a time, use [] to change a time •Third phase •Read in a vector of swim times •Build a loop, until quit is true: •Display the vector •Ask the user for an action (add, modify, quit) •Use a switch to select the action •Execute the action •Write out the changed vector…arrow_forward//import java.util.List; import javax.swing.*; import javax.swing.event.*; import java.awt.*; /** * JList basic tutorial and example * * @author wwww.codejava.net */ public class JListTask1_Student_Version extends JFrame { //define a Jlist Component // Define a String array that use to create the list private JPanel ContryPanel; // To hold components private JPanel selectedcontryPanel; // To hold components // define a TextFiles to show the result private JLabel label; // A message /** Constructor */ public JListTask1_Student_Version(){ setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setTitle("JList Task1"); setSize(200, 200); setLayout(new BorderLayout()); // Build the month and selectedMonth panels. // Add the panels to the content pane. // add(new JScrollPane(countryList)); setLocationRelativeTo(null); setVisible(true); } public void buildcontryPanel() { // Create a panel to hold the list. ContryPanel = new JPanel(); //create the list // Set the selection mode to single…arrow_forwardComputer Science Write a program in the Java language that includes: 1. A class for books, taking into account the encapsulation and getter, setter. 2. Entering books by the user using Scanner 3. LinkedList class for: (Adding a new book - Inserting a book- Searching for a book using the ISBN Number - Viewing all books,delete book). 4. Serial number increases automatically with each book that is entered. 5. Printing the names of books is as follows: Serial - ISBN - Name - PubYear - Price - Notes. 6-Design a main menu that includes: • Add Book • Insert Book • Delete Book • Search . Display . Exitarrow_forward
- Deliverable #2: How many operations in nested for loops? Implement the following psuedocode as the function two_dimensional_loop (n) in the cell below: count = 0 for i = 0 to n-1 : for j = i to n-1 : count = count + 1 return count ► def two_dimensional_loop(n) : # complete to satisfy the instructions and implement the pseudocode || return 0 expectEqual (two_dimensional_loop (520),135460) expectEqual (two_dimensional_loop (1234), int(1234* (1234+1)/2)) 个 ↓arrow_forwardJordan University of Science and TechnologyCS415 Contemporary Programming TechniquesMidterm Exam – Second semester 2021Name: ID: Section:Important note: your code must compile correctly and run without errors.Otherwise your code will get the grade zero.Question 1: Implement a java class called ParsingCustomerRecords that has thefollowing data members and functions:ParsingCustomerRecods- customerRecords: array of strings- result: string variable- recordsNumber: int variable-fileName: string variable+ Constructor(String filename, int recordsNumber)+ void Reader()+ void Executor()+ void showResult()+ String toString()All of the data members are private, and all of the methods are public.Constructor has two parameters which are (1) the file name in which Customers’ recordsare stored, and (2) the number of the records in the file. The constructor functionality is toassign suitable initial values to the fileName and recordsNumber data members. Theconstructor also should instantiate the…arrow_forwardAPI documentation link ------> https://docs.oracle.com/en/java/javase/11/docs/api/java.base/java/util/ArrayList.html PREVIOUS CODE - import java.util.ArrayList;public class BasicArrayList { // main method public static void main(String[] args) { // create an arraylist of type Integer ArrayList<Integer> basic = new ArrayList<Integer>(); // using loop add elements for(int i=0;i<4;i++){ basic.add(i+1); } // using loop set elements for(int i=0;i<basic.size();i++){ basic.set(i,(basic.get(i)*5)); } // using loop print elements for(int i=0;i<basic.size();i++){ System.out.println(basic.get(i)); } }} Q1) a)Consider how to remove elements from the ArrayList. Give two different lines of code that would both remove 5 from the ArrayList. b)What do you think the contents of the ArrayList will look like after the 5 has been removed? (Sketch or list) c)Now update the BasicArrayList program to remove the 5 (index 1) from the ArrayList. Add this step before the last loop that…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
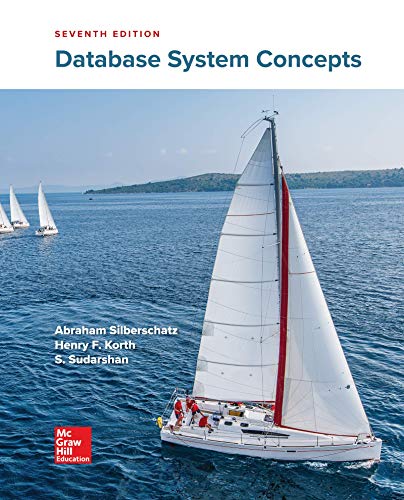
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
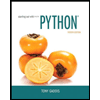
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
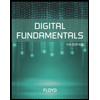
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
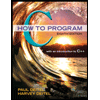
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
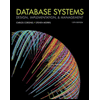
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
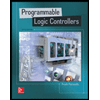
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Browse Popular Homework Q&A
Q: Match the materials to their descriptions below.
Material is made out of iron and carbon and is…
Q: Please show your answer to at least 4 decimal places.
Determine the gradient of f(r, y) = e sin(4y)…
Q: Which set of ordered processes best describes the steps to perform an emergency stop and reset of…
Q: You are the HR consultant to a small business with about 40 employees. At the present time, the firm…
Q: Which of these junctions allows solutes and water to pass directly from one cell to another, without…
Q: Write a Java program, SortedIntegerList.java, that uses a linked list to store 100 random integer…
Q: The mean entry level salary of an employee at a company is $60,000. You believe it is lower for IT…
Q: Suppose a yttrium-88 nuclide transforms into a strontium-88 nuclide by absorbing an electron and…
Q: If 11.2 g of naphthalene, C 10H 8 (Molar mass= 128 g/mol), is dissolved in
107.8 g of chloroform,…
Q: Which of the following depends on temperature?
Group of answer choices
Molarity
molality
percent by…
Q: What are the three major mechanisms by which exercise increases glycolytic rate?
Q: Breslin Incorporated made a capital contribution of investment property to its 100-percent-owned…
Q: Implicit Differentiation using f, fy & f
Let 20x²
1
dy
dr
dy
Use partial derivatives to calculate at…
Q: d. K3PO4
The purple spheres represent particles of solute. Which compounds are represente
(IV)?
I…
Q: The records of Fremont Corporation’s initial and unaudited accounts show the following ending…
Q: b1. What are the tax consequences (amount and character of recognized gain or loss) of the…
Q: what happens when a changing magnetic field through a loop of wire
a potential difference voltage…
Q: 15) What compound is produc
'N
s treated with LiAlH4?
-) CH3CH2CONH2
OCH3CH2CH2NH2
O CH3CH2CH2OH…
Q: If asteroid Toutatis were to pass somewhere within 24 x105 km of planet Syrene, what is the…
Q: What do we expect from schools? How do we know when a school is a "good school?"
Q: The fourth and final phase of the project life cycle.…
Q: Consider the reaction below. If you start with 5.00 moles of C
produced?
C3H8(g) + 5 O₂(g) → 3…
Q: Given: f(x)=-x^2-4x-3 What is the derivative of f(x) at a=-4?
Q: 3. Below is the graph of y=f(x), which is the derivative for some function f. Using the graph of the…
Q: Please
PROBLEMS
help with 7.14
Renal Disease
The mean serum-creatinine level measured in 12 patients…