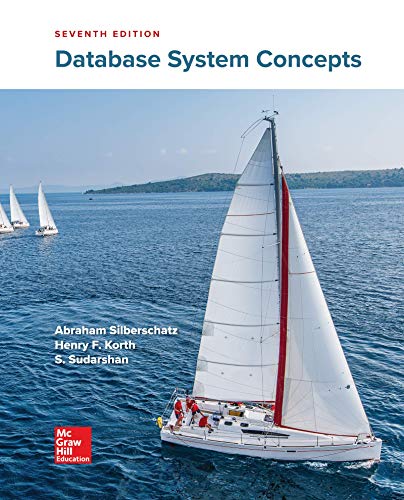
Provided Code:
BST.Java
import java.util.NoSuchElementException;
/**
* Your implementation of a BST.
*/
public class BST<T extends Comparable<? super T>> {
/*
* Do not add new instance variables or modify existing ones.
*/
private BSTNode<T> root;
private int size;
/*
* Do not add a constructor.
*/
/**
* Adds the data to the tree.
*
* This must be done recursively.
*
* The new data should become a leaf in the tree.
*
* Traverse the tree to find the appropriate location. If the data is
* already in the tree, then nothing should be done (the duplicate
* shouldn't get added, and size should not be incremented).
*
* Should be O(log n) for best and average cases and O(n) for worst case.
*
* @param data The data to add to the tree.
* @throws java.lang.IllegalArgumentException If data is null.
*/
public void add(T data) {
// WRITE YOUR CODE HERE (DO NOT MODIFY METHOD HEADER)!
}
/**
* Removes and returns the data from the tree matching the given parameter.
*
* This must be done recursively.
*
* There are 3 cases to consider:
* 1: The node containing the data is a leaf (no children). In this case,
* simply remove it.
* 2: The node containing the data has one child. In this case, simply
* replace it with its child.
* 3: The node containing the data has 2 children. Use the SUCCESSOR to
* replace the data. You should use recursion to find and remove the
* successor (you will likely need an additional helper method to
* handle this case efficiently).
*
* Do NOT return the same data that was passed in. Return the data that
* was stored in the tree.
*
* Hint: Should you use value equality or reference equality?
*
* Must be O(log n) for best and average cases and O(n) for worst case.
*
* @param data The data to remove.
* @return The data that was removed.
* @throws java.lang.IllegalArgumentException If data is null.
* @throws java.util.NoSuchElementException If the data is not in the tree.
*/
public T remove(T data) {
// WRITE YOUR CODE HERE (DO NOT MODIFY METHOD HEADER)!
}
/**
* Returns the root of the tree.
*
* For grading purposes only. You shouldn't need to use this method since
* you have direct access to the variable.
*
* @return The root of the tree
*/
public BSTNode<T> getRoot() {
// DO NOT MODIFY THIS METHOD!
return root;
}
/**
* Returns the size of the tree.
*
* For grading purposes only. You shouldn't need to use this method since
* you have direct access to the variable.
*
* @return The size of the tree
*/
public int size() {
// DO NOT MODIFY THIS METHOD!
return size;
}
}
BSTNode.Java
/**
* Node class used for implementing the BST.
*
* DO NOT MODIFY THIS FILE!!
*
* @author CS 1332 TAs
* @version 1.0
*/
public class BSTNode<T extends Comparable<? super T>> {
private T data;
private BSTNode<T> left;
private BSTNode<T> right;
/**
* Constructs a BSTNode with the given data.
*
* @param data the data stored in the new node
*/
BSTNode(T data) {
this.data = data;
}
/**
* Gets the data.
*
* @return the data
*/
T getData() {
return data;
}
/**
* Gets the left child.
*
* @return the left child
*/
BSTNode<T> getLeft() {
return left;
}
/**
* Gets the right child.
*
* @return the right child
*/
BSTNode<T> getRight() {
return right;
}
/**
* Sets the data.
*
* @param data the new data
*/
void setData(T data) {
this.data = data;
}
/**
* Sets the left child.
*
* @param left the new left child
*/
void setLeft(BSTNode<T> left) {
this.left = left;
}
/**
* Sets the right child.
*
* @param right the new right child
*/
void setRight(BSTNode<T> right) {
this.right = right;
}
}
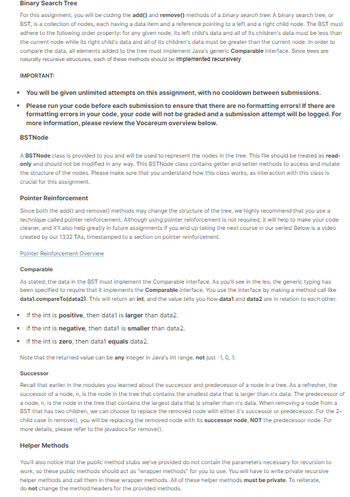
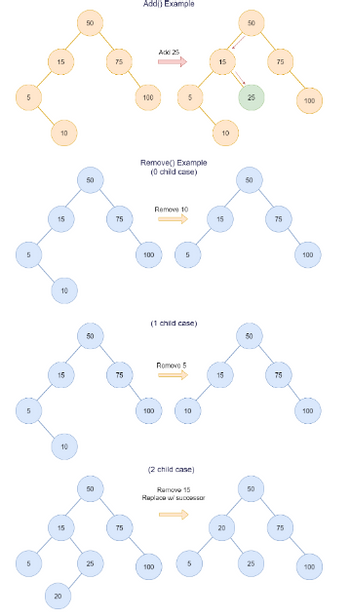

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- Program #11. Show the ArrayStackADT interface 2. Create the ArrayStackDataStrucClass<T> with the following methods: default constructor, overloaded constructor, copy constructor, initializeStack, isEmptyStack, isFullStack, push, peek, void pop 3. Create the PrimeFactorizationDemoClass: instantiate an ArrayStackDataStrucClass<Integer> object with 50 elements. Use a try-catch block in the main( ) using pushes/pops. 4. Exception classes: StackException, StackUnderflowException, StackOverflowException 5. Show the 4 outputs for the following: 3,960 1,234 222,222 13,780arrow_forwardTask 3: Implement method setup in PE1 class. The description of what is wanted is given in the starter code. TASK 1 and 2 are done in class maze. Please use PE1 class. public class PE1 { MazedogMaze; /** * This method sets up the maze using the given input argument * @param maze is a maze that is used to construct the dogMaze */ publicvoidsetup(String[][]maze){ /* insert your code here to create the dogMaze * using the input argument. */ } /** * This method returns true if the number of * gates in dogMaze >= 2. * @return it returns true, if enough gate exists (at least 2), otherwise false. */ publicbooleanenoughGate(){ // insert your code here. Change the return value to fit your purpose. if() returntrue; } /** * This method finds a path from the entrance gate to * the exit gate. * @param row is the index of the row, where the entrance is. * @param column is the index of the column, where the entrance is. * @return it returns a string that contains the…arrow_forwardJAVA programming languagearrow_forward
- Instructions-Java Assignment is to define a class named Address. The Address class will have three private instance variables: an int named street_number a String named street_name and a String named state. Write three constructors for the Address class: an empty constructor (no input parameters) that initializes the three instance variables with default values of your choice, a constructor that takes the street values as input but defaults the state to "Arizona", and a constructor that takes all three pieces of information as input Next create a driver class named Main.java. Put public static void main here and test out your class by creating three instances of Address, one using each of the constructors. You can choose the particular address values that are used. I recommend you make them up and do not use actual addresses. Run your code to make sure it works. Next add the following public methods to the Address class and test them from main as you go: Write getters and…arrow_forwardLab2.java contains the following code: public final class Lab2 { /** * This is empty by design, Lab2 cannot be instantiated */ private Lab2() { // empty by design } /** * Returns the sum of a consecutive set of numbers from <code> start </code> to <code> end </code>. * * @pre <code> start </code> and <code> end </code> are small enough to let this * method return an int. This means the return value at most requires 4 bytes and * no overflow would happen. * * @param start is an integer number * @param end is an integer number * @return the sum of start + (start + 1) + .... + end */ public static int sum(int start, int end) { //Insert your code here and change the return statement to fit your implementation. return 0; } /** * This method creates a string using the given char * by repeating the character <code> n </code> times. * * @param first is…arrow_forwardFix this code for any errorsarrow_forward
- Create a class ATM with the following data members and methods: • int totalCash • default parameterized constructor • void setCash(int) • int getCash() In Javaarrow_forwardJava:arrow_forward3.25 Fixed Sized Deque Your task is to create an implementation of the Java Deque interface that can only hold N items where N is a number passed into the constructor. Note that most (if not all) of the unit tests rely on the method object[] toArray() inherited from Collection. Therefore, you must make sure you implement that method correctly in order to get most of the points. 301664.1524810.gpazay7 LAB АCTIVITY 3.25.1: Fixed Sized Deque 0/ 70 Submission Instructions Compile command javac FixedsizedDeque.java -xlint:all -encoding utf-8 We will use this command to compile your code Upload your files below by dragging and dropping into the area or choosing a file on your hard drive. FixedSizedDeque.java Drag file here or Choose on hard drive.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
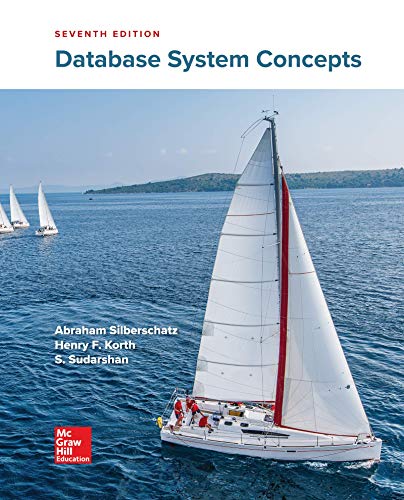
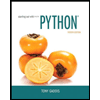
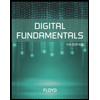
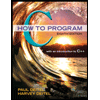
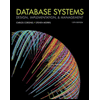
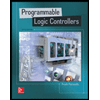