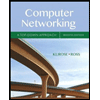
Java programming
I have to do the "Resize method" which I believe if the array is full, it should resize so there is space.
Ill paste the code below:
package circularQueue;
/*
* Design the class that would implement the interfaceiQueue.
*/
public class cirQueue <T> implements iQueue <T> {
//Array of T objects
private T[] Ar; //reference to array of objects
//int size keeps track of how many elements in the queue
int size = 0;
//first the index to the head of the queue
int first = -1;
//last the index the last element of the queue
int last = -1;
public cirQueue() {
Ar = (T[]) new Object[5];
size = 0;
first = last = -1;
}
public int size() {
return size;
}
public boolean empty() {
return (size == 0);
}
private void resize() {
//HW TODO
}
//inserts element e at the back of the queue
public void insert(T e) {
if(this.empty()) {
first = last = 0;
//insert e at indx 0;
Ar[first] = e;
size = 1;
return;
}
if(size == Ar.length) {
//resize HW complete this one.
this.resize();
}
//increase last by one, make sure it circulate back to 0 if it reaches capacity-1
last = (last + 1) % Ar.length;
//insert e at index last
Ar[last] = e;
//increase the size
size++;
}
public T remove() {
if(this.empty()) {
System.out.println("Error empty queue");
return null;
}
T save = Ar[first];
first = (first + 1) % Ar.length;
size--;
return save;
}
public String toString() {
if(this.empty())
return "[]";
String st = "[";
//build your string [-1, -3, -9]
int temp = first;
for(int i = 0; i < size-1; i++) {
//in the loop your are always dealing with element i
//v element i is Ar[i]
//append Ar[i], to the string
st += Ar[temp].toString() + ", ";
temp = (temp + 1) % Ar.length;
}
//close the bracket
st += Ar[temp].toString() + "]";
return st;
}
}
![import java.util.ArrayList;I
public class Tester {
public static void main(String [] args) {
Stack<String> st = new Stack<String>();
st.push("st");
st.push ("sfsf");
st.push("hi");
System.out.println("size:
System.out.println("removing:
System.out.println("size:
System.out.println("the top is: " + st.peek ());
System.out.println("size: " + st.size()+
+ st.size() +
+ st.pop());
+ st.size()+
St:
+ st.toString());
%3D
" St: " + st.toString());
St:
+ st.toString());
Queue<Integer> Q = new LinkedList<Integer>();
Q.add(-4);
}
}](https://content.bartleby.com/qna-images/question/33eba9e5-eb7d-44a1-93e3-ed0ee897e17f/3473cca1-3b98-4f9d-a978-c23da3b9a0de/kjk14xh_thumbnail.png)
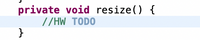

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- In java ****arrow_forwardLab 10 Using an interface to share methods It is often the case that two or more classes share a common set of methods. For programming purposes we might wish to treat the objects of those classes in a similar way by invoking some of their common routines.For example, the Dog and Cat classes listed below agree on the void method speak. Because Dog and Cat objects have the ability to “speak,” it is natural to think of putting both types of objects in an ArrayList and invoking speak on every object in the list. Is this possible? Certainly we could create an ArrayList of Dog that would hold all the Dog objects, but can we then add a Cat object to an ArrayList of Dog?Try running the main program below as it is written. Run it a second time after uncommenting the line that instantiates a Cat object and tries to add it to the ArrayList. import java.util.*;public class AnimalRunner{ public static void main(String[] args) { ArrayList<Dog> dogcatList = new ArrayList<Dog>();…arrow_forwardpackage lab1; /** * A utility class containing several recursive methods * * <pre> * * For all methods in this API, you are forbidden to use any loops, * String or List based methods such as "contains", or methods that use regular expressions * </pre> * * */ public final class Lab1 { /** * This is empty by design, Lab class cannot be instantiated */ privateLab1(){ // empty by design } /** * Returns the product of a consecutive set of numbers from <code> start </code> * to <code> end </code>. * * @param start is an integer number * @param end is an integer number * @return the product of start * (start + 1) * ..*. + end * @pre. * <code> start </code> and <code> end </code> are small enough to let * this method return an int. This means the return value at most * requires 4 bytes and no overflow would happen. */ publicstaticintproduct(ints,inte){ if(s==e){ returne; }else{ returns*product(s+1,e); } }…arrow_forward
- 1). Create the class called ArrayOperations2D and include the method findRowMax below public class ArrayOperations2D { public static intl] findRowMax (int [][] anArray) int [] max = new int [anArray. length]; //for each row for (int i=0; i< anArray. length; i++) int largest = anArray [1][0]; for (int i=0; < anArray [i].langthi i++) if (anArray[i](j] > largest) largest = anArray[illjl: max [i]=largest; } return max; 2). Include the method called findRowSums below public static int[] findRowSums (int [] [] anArray) int [] rowSums = new int [anArray.length]; for (int i=0; i< anArray.length; i++) int sum = 0; for (int j=0; j<anArray(jl.length; J++) sum+=anArray [i][jl; rowSums [1] =sum; } return rowSums; 3). Add a method called findColumnMax to the class. This method should find and return the maximum value in each column of the two dimensional array 4). Add a method called findColumnSum that calculates and returns the sum of each column. 5). Add a method called…arrow_forwardActivity: Create a collection of objects using Java generics. use java to write code. Create a Month class that can accept integer or String data type as an argument. The parameter type will be decided at run time. For this exercise, we will use Month class to store integer and string values that contains 12 months. For example, the integer value of 12 corresponds to string value of “December.” Use the following minimal class definition for Month class. public class Month<T> { private T month; public void setMonth(T month); public T getMonth(); }; Create a new class, MonthApp. This new class will have main method, and will instantiate Month objects to store month indices and names. Here is an example using arrays. // defineMonth<Integer>[] monthsByNumber = new Month<>[12];Month<String>[] monthsByName = new Month<>[12];// adding valuesmonthsByNumber[0] = new Month<Integer>();monthsByNumber[0].setMonth(1);monthsByName[0] = new…arrow_forwardHelparrow_forward
- Task 3: Implement method setup in PE1 class. The description of what is wanted is given in the starter code. public class PE1 { MazedogMaze; /** * This method sets up the maze using the given input argument * @param maze is a maze that is used to construct the dogMaze */ publicvoidsetup(String[][]maze){ /* insert your code here to create the dogMaze * using the input argument. */ } /** * This method returns true if the number of * gates in dogMaze >= 2. * @return it returns true, if enough gate exists (at least 2), otherwise false. */ publicbooleanenoughGate(){ // insert your code here. Change the return value to fit your purpose. returntrue; } /** * This method finds a path from the entrance gate to * the exit gate. * @param row is the index of the row, where the entrance is. * @param column is the index of the column, where the entrance is. * @return it returns a string that contains the path from the start to the end. * The return value should…arrow_forwardTask 3: Implement method setup in PE1 class. The description of what is wanted is given in the starter code. TASK 1 and 2 are done in class maze. Please use PE1 class. public class PE1 { MazedogMaze; /** * This method sets up the maze using the given input argument * @param maze is a maze that is used to construct the dogMaze */ publicvoidsetup(String[][]maze){ /* insert your code here to create the dogMaze * using the input argument. */ } /** * This method returns true if the number of * gates in dogMaze >= 2. * @return it returns true, if enough gate exists (at least 2), otherwise false. */ publicbooleanenoughGate(){ // insert your code here. Change the return value to fit your purpose. if() returntrue; } /** * This method finds a path from the entrance gate to * the exit gate. * @param row is the index of the row, where the entrance is. * @param column is the index of the column, where the entrance is. * @return it returns a string that contains the…arrow_forwardIn C# Use a one-dimensional array to solve the following problem. Write a class called ScoreFinder. This class receives a single dimensional integer array representing passer ratings for NFL players as an argument for its constructor. The test class will provides this argument. The test class then calls upon a method from the ScoreFinder class to start the process of finding a specific rating, which will be provided by the user. The method will iterate through the array and find any matching values. For all the matches that are found, the method will note the index of the cell along with its value. At completion, the method will print a list of all the indices and the associated scores along with a count of all the matching values.arrow_forward
- Matrix Multiplication Write a method called multiply in the Matrix class that takes an instance of Matrix as a parameter and returns the result of matrix multiplication. Complete the definition of the class Matrix and method definition multiply shown here and in the starter code. public class Matrix { public Matrix multiply(Matrix other) { // fill in code here } } For purposes of this exercise, you should assume (without checking) that the matrices to be multiplied have the correct shapes, ie, that this.numCols() is equivalent to other.numRows(). To multiply matrices, the number of columns in the first matrix must equal the number of rows in the second matrix. In general, if we multiply an m x n matrix A (ie, having m rows and n columns) by a n x p matrix B (ie, having n rows and p columns), the product will have size m x p (ie, m rows and p columns). Here is a simple illustration of the computation for two 2 x 2 matrices: Examples Example 1 If A and B were both instances of the…arrow_forwardInstructions-Java Assignment is to define a class named Address. The Address class will have three private instance variables: an int named street_number a String named street_name and a String named state. Write three constructors for the Address class: an empty constructor (no input parameters) that initializes the three instance variables with default values of your choice, a constructor that takes the street values as input but defaults the state to "Arizona", and a constructor that takes all three pieces of information as input Next create a driver class named Main.java. Put public static void main here and test out your class by creating three instances of Address, one using each of the constructors. You can choose the particular address values that are used. I recommend you make them up and do not use actual addresses. Run your code to make sure it works. Next add the following public methods to the Address class and test them from main as you go: Write getters and…arrow_forwardCan you please help me with this, its in java. Thank you. Write classes in an inheritance hierarchy Implement a polymorphic method Create an ArrayList object Use ArrayList methods For this, please design and write a Java program to keep track of various menu items. Your program will store, display and modify salads, sandwiches and frozen yogurts. Present the user with the following menu options: Actions: 1) Add a salad2) Add a sandwich3) Add a frozen yogurt4) Display an item5) Display all items6) Add a topping to an item9) QuitIf the user does not enter one of these options, then display:Sorry, <NUMBER> is not a valid option.Where <NUMBER> is the user's input. All menu items have the following: Name (String) Price (double) Topping (StringBuilder or StringBuffer of comma separated values) In addition to everything that a menu item has, a main dish (salad or sandwich) also has: Side (String) A salad also has: Dressing (String) A sandwich also has: Bread type…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
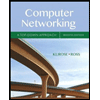
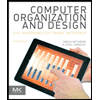
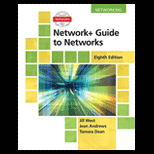
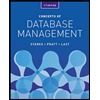
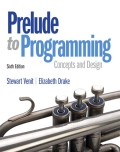
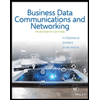