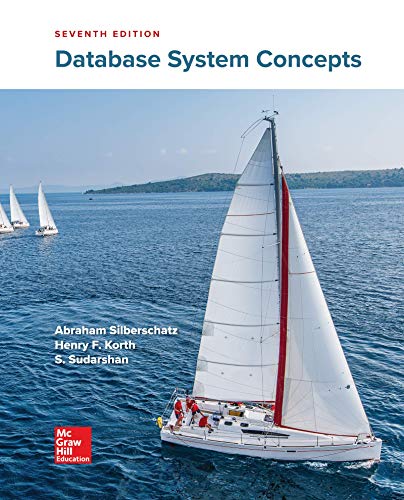
Correct the indentation errors in this code for
import java.util. Scanner;
public class GATest
public static void main(String|] arg)
{
boolean repeat = true;
while(repeat)
{
Scanner myScanner = new Scanner (System. in);
System.out.print("\nEnter string to guess--»");
String goal = myScanner.nextLine);
System.out.print(Enter number of organisms per generation--»");
int popSize = Integer.parseInt (myScanner.next));
System.out.print("Enter number
of generations-->");
int generations =
Integer. parseInt (myScanner. next));
System.out.print (Enter
mutation probability-->"):
double mutateProb = Double.parseDouble (myScanner.next ());
System. out.println();
Population aPopulation = new
Population(goal, popSize, generations.
mutateProb) :
aPopulation. iterate();
System.out.println("Repeat? y/n");
String answer = myScanner.next();
if (answer. toUpperCase() .equals (“Y”);
repeat = true;
else
repeat = false;
}
}
{
import java.util. Random;
public class Organism implements Comparable«Organism»
{
String value, goalString;
double fitness;
int n;
Random myRandom = new Random();
public Organism(String goalString)
{
value=“”;
this.goalString=goalString;
this.n= goalString.length();
for(int i=0; i<n; i++);
{
int j = myRandom. nextInt(27); //formerly 27
1f(j==26)
value=value+" “;
//else if (j==27) value = value+"”;
//else if (j= 28) value=value+".";
int which = myRandom. nextInt(2);
if(which==0)
j=j+65;
else
j=j+97;
value=value+ (char)j;
{
{
{
public Organism(String goalString, String value, int n)
{
this.goalString=goalString;
this.value = value;
this.n=n;
{
public Organism()
{
}
public String getValue()
{
return this.value;
}
public void
setValue (String value)
{
this.value = value;
{
public String toString()
{
return value +” “+ goalString+” "+getFitness(goalString);
{
public int getFitness(String aString)
int count =0;
for(int i=0; i< this.n; i++)
if(this.value.charAt(1)== aString.charAt(1))
count++;
return count;
}
public int compareTo(Organism other)
{
int thisCount, otherCount; thisCount=getFitness (goalString); otherCount=other. getFitness (goalString);
if (thisCount == otherCount)
return 0;
else if (thisCount < otherCount)
return 1;
else
return-1;
}
public Organism[] mate(Organism other)
{
Random aRandom = new Random();
int crossOver = aRandom.nextInt(n);
String child1=“”, child2="”;
for (int i=0; i< crossOver; i++)
{
child1=child1+this.value.charAt(i);
child2 = child2+other .value.charAt(i);
}
for (int 1= crossOver; i<n; i++)
{
child1=child1+other .value.charAt (1);
child2=child2+this.value.charAt (1);
}
Organism[] children= new Organism[2];
children[0] = new Organism(goalString, child1,n);
children[1] = new Organism(goalString, child2, n);
//System.out.println(“In mate"+children[0].getFitness(goalString));
return children;
}
public void mutate(double mutateProb)
{
String newString="”;
for (Int i=0; i< n; i++)
{
int k = myRandom. nextInt (100) ;
if (k/100.0 >mutateProb)
newString = neString+value.charAt(i);
else
{
int j = myRandom. nextInt (27);
if (j==26)
newString=newString+" “;
else
{
int which = myRandom. nextInt (2);
if (which ==0)
j=j +65;
else
j=j+97;
newString = newString+
(char)j;
}
}
}
this.setValue(newString);
}
}
to generate a solution
a solution
- import java.util.Scanner; public class AverageWithSentinel{ public static final int END_OF_INPUT = -500; public static void main(String[] args) { Scanner in = new Scanner(System.in); // Step 2: Declare an int variable with an initial value // as the count of input integers // Step 3: Declare a double variable with an initial value // as the total of all input integers // Step 4: Display an input prompt // "Enter an integer, -500 to stop: " // Step 5: Read an integer and store it in an int variable // Step 6: Use a while loop to update count and total as long as // the input value is not -500. // Then display the same prompt and read the next integer // Step 7: If count is zero // Display the following message // "No integers were…arrow_forwardimport java.util.Scanner; public class leapYearLab { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int userYear; boolean LeapYear; System.out.println("Enter the year"); userYear = scnr.nextInt(); isLeapYear(userYear); //if leap year if (isLeapYear){ System.out.println(userYear + " - leap year"); } else{ System.out.println(userYear + " - not a leap year"); } scnr.close(); } public static boolean isLeapYear(int userYear){ boolean LeapYear; /* Type your code here. */ if ( userYear % 4 == 0) { // checking if year is divisible by 100 if ( userYear % 100 == 0) { // checking if year is divisible by 400 // then it is a leap year if ( userYear % 400 == 0) LeapYear = true; else LeapYear = false; } // if the year is not divisible by 100 else…arrow_forwardimport java.util.Scanner; public class CharMatch { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String userString; char charToFind; int strIndex; userString = scnr.nextLine(); charToFind = scnr.next().charAt(0); strIndex = scnr.nextInt(); /* Your code goes here */ }}arrow_forward
- import java.util.Scanner; public class ParkingFinder {/* Your code goes here */ public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int numVisits; int duration; numVisits = scnr.nextInt(); duration = scnr.nextInt(); System.out.println(findParkingPrice(numVisits, duration)); }}arrow_forwardimport java.util.Scanner; public class LabProgram { // Recursive method to draw the triangle public static void drawTriangle(int baseLength, int currentLength) { if (currentLength <= 0) { return; // Base case: stop when currentLength is 0 or negative } // Calculate the number of spaces needed for formatting int spaces = (baseLength - currentLength) / 2; if (currentLength == baseLength) { // If it's the first line, don't output spaces before the first '*' System.out.println("*".repeat(currentLength) + " "); } else { // Output spaces and asterisks System.out.println(" ".repeat(spaces) + "*".repeat(currentLength) + " "); } // Recursively call drawTriangle with the reduced currentLength drawTriangle(baseLength, currentLength - 2); } public static void drawTriangle(int baseLength) { drawTriangle(baseLength, baseLength); } public static…arrow_forwardPlease help fastarrow_forward
- StringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forward8) Now use the Rectangle class to complete the following tasks: - Create another object of the Rectangle class named box2 with a width of 100 and height of 50. Note that we are not specifying the x and y position for this Rectangle object. Hint: look at the different constructors) Display the properties of box2 (same as step 7 above). - Call the proper method to move box1 to a new location with x of 20, and y of 20. Call the proper method to change box2's dimension to have a width of 50 and a height of 30. Display the properties of box1 and box2. - Call the proper method to find the smallest intersection of box1 and box2 and store it in reference variable box3. - Calculate and display the area of box3. Hint: call proper methods to get the values of width and height of box3 before calculating the area. Display the properties of box3. 9) Sample output of the program is as follow: Output - 1213 Module2 (run) x run: box1: java.awt. Rectangle [x=10, y=10,width=40,height=30] box2: java.awt.…arrow_forwardimport java.util.Scanner; public class LabProgram { /* Define your method here */ public static void main(String[] args) { Scanner scnr = new Scanner(System.in); /* Type your code here. */ }}arrow_forward
- import java.util.Scanner; public class AutoBidder { public static void main (String [] args) { Scanner scnr = new Scanner (System.in); char keepGoing; int nextBid; nextBid = 0; keepGoing 'y'; * Your solution goes here */ while { nextBid = nextBid + 3; System.out.println ("I'll bid $" + nextBid + "!") ; System.out.print ("Continue bidding? (y/n) "); keepGoing = scnr.next ().charAt (0); } System.out.println (""); }arrow_forwardpackage lab06;;public class gradereport { public static void main(String[] args) { Scanner in = new Scanner(System.in);double[] Scores = new double[10]; for(int i=0;i<10;i++){ System.out.println("Enter score " + (i+1));scores[i]=in.nextdouble(); } for(int i=0;i<10;i++){ if (scores[i] >=80) System.out.println("Score " + (i+1) + " receives a grade of HD"); else if (scores[i]>=70) System.out.println("Score " + (i+1) + " receives a grade of D"); else if (scores[i] >=60) System.out.println("Score "+ (i+1) + " receives a grade of C"); else if (scores[i] >=50) System.out.println("Score " + (i+1) + " receives a grade of P"); else if (scores[i] >=40) System.out.println("Score " + (i+1) + " receives a grade of MF"); else if (scores[i] >=0) System.out.println("Score " +…arrow_forwardConvert the following java code to C++ //LabProgram.javaimport java.util.Scanner;public class LabProgram {public static void main(String[] args) {//defining a Scanner to read input from the userScanner input = new Scanner(System.in);//reading the value for Nint N = input.nextInt();//creating a 1xN matrixint[] m1 = new int[N];//creating an NxN matrixint[][] m2 = new int[N][N];//creating a 1xN matrix to store the resultint[] result = new int[N];//looping and reading N integers into m1for (int i = 0; i < N; i++) {m1[i] = input.nextInt();}//looping from 0 to N-1for (int i = 0; i < N; i++) {//looping from 0 to N-1for (int j = 0; j < N; j++) {//reading an integer and storing it into m2 at position i,jm2[i][j] = input.nextInt();}}//multiply m1 and m2, store result in result//looping from 0 to N-1for (int i = 0; i < N; i++) {//looping from 0 to N-1for (int j = 0; j < N; j++) {//multiplying value at index j in m1 with value at j,i in m2, adding to current value at index i// on…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
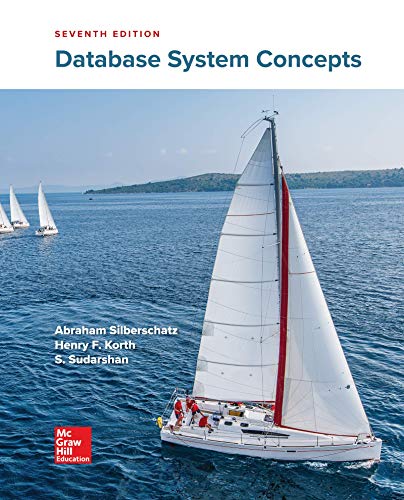
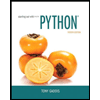
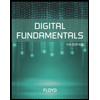
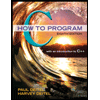
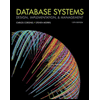
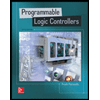