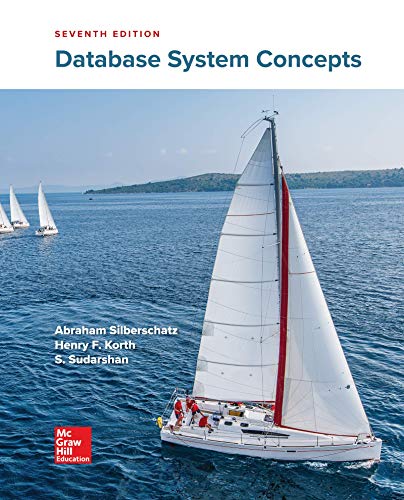
How do I mahe my code output results Java?
Code:
import java.util.Scanner;
class FinalExamAnswer
{
public static void main(String [] args)
{
manipulateString(); //calls function
}
//your code here
public static void manipulateString()
{
Scanner sc=new Scanner(System.in); //create Scanner instance
System.out.print("Enter a sentence: ");
String sentence=sc.nextLine(); //input a sentence
String[] words=sentence.split(" "); //split the sentence at space and store it in array
for(int i=0;i<words.length;i++) //i from 0 to last index
{
if(i%2==0) //if even index
words[i]=words[i].toUpperCase(); //converted to upper case
else //if odd index
words[i]=words[i].toLowerCase(); //converted to lower case
}
for(int i=words.length-1;i>=0;i--) //i from last index to 0
{
System.out.print(words[i]+" "); //print word at index i and space
}
}
}
Input and output:
1.
Now is the time for all good programmers to come to the aid of their country
Enter a sentence country THEIR of AID the TO come TO programmers GOOD all FOR time THE is NOW
2.
How are you doing today?
Enter a sentence TODAY? doing YOU are HOW
3.
I don't always Test My CODE, but when I do, I do it in Production
Enter a sentence PRODUCTION in IT do I do, I when BUT code, MY test ALWAYS don't I
4.
Caterpillars bRing riverS aNd forests surrounding bEARs
Enter a sentence BEARS surrounding FORESTS and RIVERS bring CATERPILLARS
5.
WhaT GoeS Up MusT ComE DowN
Enter a sentence down COME must UP goes WHAT
6.
yoU Were ComInG When i wAs GoinG
Enter a sentence GOING was I when COMING were YOU

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

- 1 import java.util.Scanner; 3 public class TriangleArea { INM&567 000 2 4 8 9 10 11 12 13 14 15 4567890 16 17 18 19 20 21 } public static void main(String[] args) { Scanner scnr = new Scanner(System.in); } Current file: TriangleArea.java = Triangle triangle1 new Triangle(); Triangle triangle2 = new Triangle(); // TODO: Read and set base and height for triangle1 (use setBase() and setHeight()) // TODO: Read and set base and height for triangle2 (use setBase() and setHeight()) System.out.println("Triangle with smaller area:"); // TODO: Determine smaller triangle (use getArea()) // and output smaller triangle's info (use printInfo())arrow_forwardHow do I remove the space at the end of the result? Code: import java.util.Scanner; public class FinalExamAnswers{ public static void main(String [] args) { manipulateString(); //calls function } //your code here public static void manipulateString() { Scanner sc=new Scanner(System.in); //create Scanner instance System.out.println("Enter a sentence"); String sentence=sc.nextLine(); //input a sentence String[] words=sentence.split(" "); //split the sentence at space and store it in array for(int i=0;i<words.length;i++) //i from 0 to last index { if(i%2==0) //if even index words[i]=words[i].toUpperCase(); //converted to upper case else //if odd index words[i]=words[i].toLowerCase(); //converted to lower case } for(int i=words.length-1;i>=0;i--) //i from last index to 0 {…arrow_forwardPrimary U.S. interstate highways are numbered 1-99. Odd numbers (like the 5 or 95) go north/south, and evens (like the 10 or 90) go east/west. Auxiliary highways are numbered 100-999, and service the primary highway indicated by the rightmost two digits. Thus, 1-405 services 1-5, and I-290 services 1-90. Note: 200 is not a valid auxiliary highway because 00 is not a valid primary highway number. Given a highway number, indicate whether it is a primary or auxiliary highway. If auxiliary, indicate what primary highway it serves. Also indicate if the (primary) highway runs north/south or east/west. Ex: If the input is: 90 the output is: I-90 is primary, going east/west. Ex: If the input is: 290 the output is: I-290 is auxiliary, serving I-90, going east/west. Ex: If the input is: 0 or any number not between 1 and 999, the output is: 0 is not a valid interstate highway number. Ex: If the input is: 200 the output is: 200 is not a valid interstate highway number.arrow_forward
- JAVA CODE: check outputarrow_forwardI have the following code: import java.util.*; import java.io.*; public class GradeBook { publicstaticvoidmain(String[] args)throwsIOException{ // TODO Auto-generated method stub File infile =newFile("students.dat"); Scanner in =newScanner(infile); while(in.hasNext()){ // Read information form file and create a student object and print String name = in.nextLine(); Student student =newStudent(name, in.nextLine()); for(int i =1; i <=4;++i){ student.setQuiz(i, in.nextInt()); } student.setMidtrmExm(in.nextInt()); student.setFinalExm(in.nextInt()); in.nextLine(); // Calculate grade and letter grade; double overallQuizScore=0.0,score=0.0; for(int i=1;i<=student.NUM_QUIZZES;i++) { overallQuizScore+=(student.getQuiz(i)/student.QUIZ_MAX_POINTS)*100; } overallQuizScore = (overallQuizScore/4)*0.30; score =…arrow_forwardimport java.util.Scanner; public class TriangleArea { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); Triangle triangle1 = new Triangle(); Triangle triangle2 = new Triangle(); // TODO: Read and set base and height for triangle1 (use setBase() and setHeight()) // TODO: Read and set base and height for triangle2 (use setBase() and setHeight()) System.out.println("Triangle with smaller area:"); // TODO: Determine smaller triangle (use getArea()) // and output smaller triangle's info (use printInfo()) }} public class Triangle { private double base; private double height; public void setBase(double userBase){ base = userBase; } public void setHeight(double userHeight) { height = userHeight; } public double getArea() { double area = 0.5 * base * height; return area; } public void printInfo() { System.out.printf("Base:…arrow_forward
- import java.util.Scanner; public class StateInfo { /* Your code goes here */ public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String stateCode; String stateName; stateCode = scnr.next(); stateName = scnr.next(); printStateInfo(stateCode, stateName); }}arrow_forwardimport java.util.Scanner; public class AverageWithSentinel{ public static final int END_OF_INPUT = -500; public static void main(String[] args) { Scanner in = new Scanner(System.in); // Step 2: Declare an int variable with an initial value // as the count of input integers // Step 3: Declare a double variable with an initial value // as the total of all input integers // Step 4: Display an input prompt // "Enter an integer, -500 to stop: " // Step 5: Read an integer and store it in an int variable // Step 6: Use a while loop to update count and total as long as // the input value is not -500. // Then display the same prompt and read the next integer // Step 7: If count is zero // Display the following message // "No integers were…arrow_forwardimport java.util.Scanner;import java.util.ArrayList; public class LabProgram { public static void main(String[] args) { Course cop3804=new Course(); String fn, ln; int score; String studentType; Scanner kb=new Scanner(System.in); studentType=kb.next(); while (!studentType.equals("q")) { fn = kb.next(); ln = kb.next(); score=kb.nextInt(); if (studentType.equals("R")) cop3804.addStudent(new Student(fn, ln, score)); else cop3804.addStudent(new HonorStudent(fn, ln, score)); studentType = kb.next(); } cop3804.print(); } } import java.util.ArrayList; public class Course { private ArrayList<Student> roster; //collection of Student objects public Course() { roster = new ArrayList<Student>(); } public void addStudent(Student s) { roster.add(s); } public void…arrow_forward
- import java.util.Scanner; public class CharMatch { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String userString; char charToFind; int strIndex; userString = scnr.nextLine(); charToFind = scnr.next().charAt(0); strIndex = scnr.nextInt(); /* Your code goes here */ }}arrow_forwardimport java.util.Scanner; public class ParkingFinder {/* Your code goes here */ public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int numVisits; int duration; numVisits = scnr.nextInt(); duration = scnr.nextInt(); System.out.println(findParkingPrice(numVisits, duration)); }}arrow_forwardPeople find it easier to read time in hours, minutes, and seconds rather than just seconds. Write a program that reads in seconds as input, and outputs the time in hours, minutes, and seconds. Ex: If the input is: 4000 the output is: Hours: 1 Minutes: 6 Seconds: 40arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
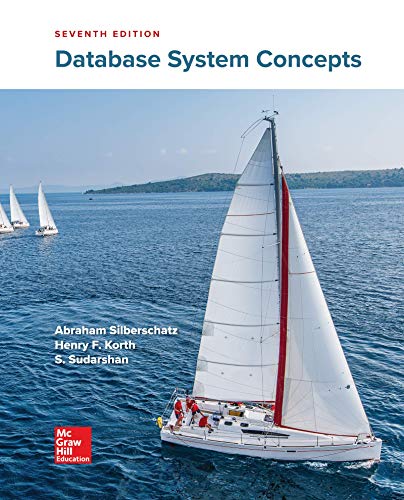
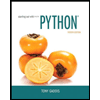
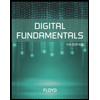
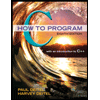
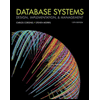
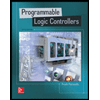