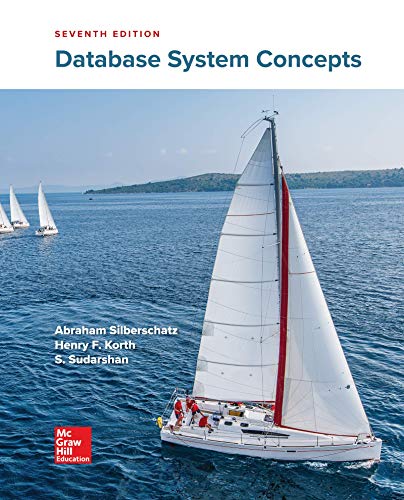
COSC 1336 –
Fundamentals I Python Programming
Write a class named RetaiI_Item that holds data about an item in a retail store.
The class should store the following data in attributes:
• Item Number
• Item Description
• Units in Inventory
• Price
Create another class named Cash_Register that can be used with the Retail_Item class. The Cash_Register class should be able to internally keep a list of Retail_Item objects. The class should include the following methods:
• A method named purchase_item that accepts a Retail_Item object as an
argument. Each time the purchase_item method is called, the Retail_Item
that is passed as an argument should be added to the list.
• A method named get_total that returns the total price of all the
Retail_Items objects stored in the Cash_Register’s internal list.
• A method named show_items that displays data about the Retail_Item
objects stored in the Cash_Register object’s internal list.
• A method named clear_items that should clear the Cash_Register
object’s internal list.
Write a main function that uses the Cash_Register class to allow the user to
select several items for purchase. When the user is ready to check out, the
program should display a list of all the items he or she has selected for purchase, as well as the total price, taxes, and the final price.
Run your program, with your own data, and copy and paste the output to a file

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- c++ class runner with constructor and COPY CONSTRUCTORarrow_forwardB. Pet Class Using Python Program, Write a class named Pet, which should have the following data attributes: • _ _name (for the name of a pet) •__animal_type (for the type of animal that a pet is. Example values are 'Dog', 'Cat', and `Bird')' __age (for the pet's age) init The Pet class should have an method that creates these attributes. It should also have the following methods: set_name() This method assigns a value to the __name field. set_animal_type() This method assigns a value to the __animal_type field. • set_age() This method assigns a value to the • get_name() This method returns the value of the get_animal_type() This method returns the value of the __animal_type field. • get_age() This method returns the value of the _age field. Once you have written the class, write a program that creates an object of the class and prompts the user to enter the name, type, and age of his or her pet. This data should be stored as the object's attributes. Use the object's accessor methods…arrow_forwardJAVA Programming Problem 3 - Book A Book has such properties as title, author, and numberOfPages. A Volume will have properties such as volumeName, numberOfBooks, and an array of book objects (Book [ ]). You are required to develop the Book and Volume classes, then write an application (DemoVolume) to test your classes. The directions below will give assistance. Create a class called Book with the following properties using appropriate data types: Title, Author, numberOfPages, Create a second class called Volume with the following properties using appropriate data types: volumeName, numberOfBooks and Book [ ]. The Book [ ] contains an array of book objects. Directions Create a class called Book with the following properties using appropriate data types: Title, Author, numberOfPages, The only methods necessary in the Book class, for this exercise, are the constructor and a toString(). Create a second class called Volume with the following properties using appropriate data types:…arrow_forward
- class Book: book_belongs_to = 'Schulich School of Engineering' def _init_(self, pages = 0, title = 'Unknown', author = 'Unknown', isbn = self.pages = pages self.title = title e): self.author = author self.isbn = isbn book1 = Book(255, 'Black Beauty', 'Anna Sewell', 9780001840423) book2 = Book(208, 'The Chrysalids', 'John Wyndham', 9780140013085) Book.book_belongs_to = 'Emily Marasco' book3 = Book()arrow_forwardQuestion 31 class Person: def __init__(mysillyobject, name, age): = name mysillyobject.name mysillyobject.age = age def myfunc(abc): print("Hello my age is", abc.age) p1 = Person("John", 36) p1.age = 40 p1.myfunc() O 40 Hello my age is 40 36 Hello my age is 36arrow_forwardIN C++ Lab #6: Shapes Create a class named Point. private attributes x and y of integer type. Create a class named Shape. private attributes: Point points[6] int howManyPoints; Create a Main Menu: Add a Triangle shape Add a Rectangle shape Add a Pentagon shape Add a Hexagon shape Exit All class functions should be well defined in the scope of this lab. Use operator overloading for the array in Shape class. Once you ask the points of any shape it will display in the terminal the points added.arrow_forward
- #Python IDLE: #Below is my Pizza class ,how would I write the function described in the attached image,based on this class? # The Pizza class should have two attributes(data items): class Pizza: # The Pizza class should have the following methods/operators): # __init__ - # constructs a Pizza of a given size (defaults to ‘M’) # and with a given set of toppings (defaults to empty set). def __init__(self, size='M', toppings=set()): self.size = size self.toppings = toppings # setSize – set pizza size to one of ‘S’,’M’or ‘L’ def setSize(self, size): self.size = size # getSize – returns size def getSize(self): return self.size # addTopping – adds a topping to the pizza, no duplicates, i.e., adding ‘pepperoni’ twice only adds it once def addTopping(self, topping): self.toppings.add(topping) # removeTopping – removes a topping from the pizza def removeTopping(self, topping):…arrow_forwardPointer and classImplement a class called Team as specified:data members:name - the name of the Team (defined as a dynamic variable)members - a dynamic array of stringsize - number of members in the team.functions:Course(): default constructor set name to “TBD”, and size to 0, members toempty listCourse(string): one argument constructor set name, and size to 0, members toempty listaccessor - an accessor for the name and size variablemutator - an mutator for the name variableUse following main() to test your class.int main() {Team a,b("Mets");cout<<a.getName()<<endl; // print TBDa.setName("Yankee");cout<<a.getName()<<endl; // print Yankeecout<<b.getName()<<endl; // print Metscout<<a.getSize()<<endl; // print 0return 0;}arrow_forwardPersonal Information Class: Design a class called Employee that holds the following data about an employee: name ID number Department Job Title Class. Store your class in a separate file called employee.py. Your class will have an initializer method that will be passed the information entered by the user as arguments. Write appropriate accessor and mutator methods for each data attribute. Write a __str__ method to print the contents of the class (see example of __str__ on p. 523). Main program: Your main program should create three instances of the class. Your program should get the information from the user and pass it as parameters to the initializer method. Using the __str__ method invoked by the print function, the program should display the personal information for the three individuals. Output and Sample Dialog: Enter employee name: Mary Smith Enter employee ID: 123456 Enter department: Accounting Enter position: Accountant Enter employee name: Joe Morales Enter…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
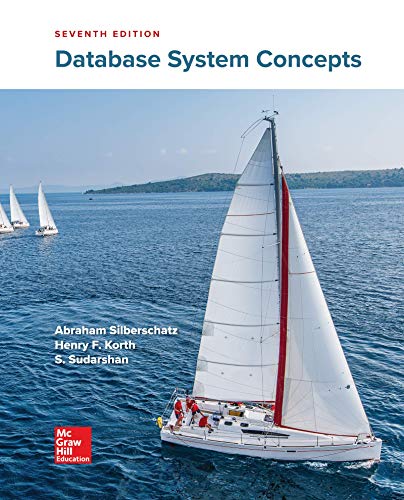
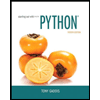
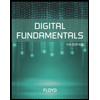
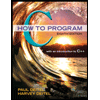
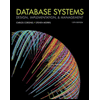
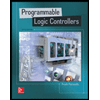