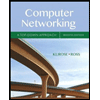
below is my code, need help with this javafx code
package com.example.demo1;
import javafx.application.Application;
import javafx.application.Platform;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.fxml.FXMLLoader;
import javafx.geometry.HPos;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.GridPane;
import javafx.scene.layout.HBox;
import javafx.scene.layout.VBox;
import javafx.scene.paint.Color;
import javafx.scene.text.Font;
import javafx.scene.text.FontWeight;
import javafx.stage.Stage;
import java.io.IOException;
import java.lang.reflect.Field;
import java.util.Objects;
import java.util.Optional;
public class HelloApplication extends Application {
public boolean inputValidation(String s){
try {
int a = Integer.parseInt(s);
return true;
}catch (Exception e ){
return false;
}
}
@Override
public void start(Stage stage) throws IOException {
GridPane grid= new GridPane();
grid.setVgap(10);
grid.setHgap(10);
//Component
RadioButton opt1 = new RadioButton("*");
RadioButton opt2 = new RadioButton("+");
RadioButton opt3 = new RadioButton("-");
RadioButton opt4 = new RadioButton("/");
Label weightL = new Label("Enter first number(x)");
Label heightL = new Label("Enter second number(y)");
Label bmiL = new Label("GUI");
TextField hText = new TextField();
TextField wText = new TextField();
TextField bText = new TextField();
Button btn = new Button("Calculate!");
HBox toggle = new HBox();
ToggleGroup tg = new ToggleGroup();
opt1.setToggleGroup(tg);
opt2.setToggleGroup(tg);
opt3.setToggleGroup(tg);
opt4.setToggleGroup(tg);
toggle.getChildren().addAll(opt1,opt2,opt3,opt4);
grid.add(weightL,0,0);
grid.add(wText,1,0);
grid.add(heightL,0,1);
grid.add(hText,1,1);
grid.add(toggle,0,2);
grid.add(btn,0,3);
grid.add(bmiL,0,4);
grid.add(bText,1,4);
btn.setOnAction(event -> {
if(inputValidation(hText.getText())&&inputValidation(wText.getText())) {
RadioButton gg = (RadioButton) tg.getSelectedToggle();
if (gg == null) {
Alert a = new Alert(Alert.AlertType.ERROR);
a.setTitle("Error");
a.setHeaderText("Nothing is Selected");
a.setContentText("Please select the option out of English or Metric");
a.show();
} else {
double weight = Double.parseDouble(wText.getText());
double height = Double.parseDouble(hText.getText());
if (gg.getText() == "*") {
double BMI = (weight / Math.pow(height,2)) * 703;
bText.setText(Double.toString(BMI));
} else if (gg.getText() == "+") {
double BMI = ((weight) / Math.pow(height,2))*10000;
bText.setText(Double.toString(BMI));
}
}
}else {
Alert a = new Alert(Alert.AlertType.ERROR);
a.setTitle("Error");
a.setHeaderText("Invalid Input");
a.setContentText("Please check Your input maybe br it is invalid");
a.show();
}
});
Scene scene = new Scene(grid);
stage.setWidth(400);
stage.setHeight(400);
stage.setTitle("BMI Calculator");
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch();
}
}
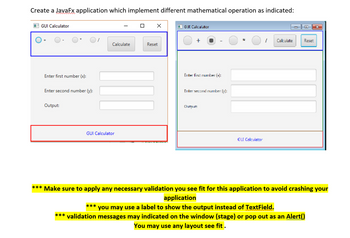

Step by stepSolved in 3 steps with 2 images

- Question & instructions can be found in images please see images first Starter code import javax.swing.*;import java.awt.*;import java.awt.event.*;import java.text.DecimalFormat; public class GPACalculator extends JFrame{private JLabel gradeL, unitsL, emptyL; private JTextField gradeTF, unitsTF; private JButton addB, gpaB, resetB, exitB; private AddButtonHandler abHandler;private GPAButtonHandler cbHandler;private ResetButtonHandler rbHandler;private ExitButtonHandler ebHandler; private static final int WIDTH = 400;private static final int HEIGHT = 150; private static double totalUnits = 0.0; // total units takenprivate static double gradePoints = 0.0; // total grade points from those unitsprivate static double totalGPA = 0.0; // total GPA (gradePoints / totalUnits) //Constructorpublic GPACalculator(){//Create labelsgradeL = new JLabel("Enter your grade: ", SwingConstants.RIGHT);unitsL = new JLabel("Enter number of units: ", SwingConstants.RIGHT);emptyL = new…arrow_forwardWhat is interesting and difficult about GUI concepts in Java? For instance event handlers, layout managers, frames, panels. polymorphism, JavaFX.arrow_forwardPlease help me fix the java program below. I keep on getting hello world when I run it AnimatedBall.java import javax.swing.*;import java.awt.*;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;public class AnimatedBall extends JFrame implements ActionListener{private Button btnBounce; // declare a button to playprivate TextField txtSpeed; // declare a text field to enterprivate Label lblDelay;private Panel controls; // generic panel for controlsprivate BouncingBall display; // drawing panel for ballContainer frame;public AnimatedBall (){// Set up the controls on the appletframe = getContentPane();btnBounce = new Button ("Bounce Ball");//create the objectstxtSpeed = new TextField("10000", 10);lblDelay = new Label("Enter Delay");display = new BouncingBall();//create the panel objectscontrols = new Panel();setLayout(new BorderLayout()); // set the frame layoutcontrols.add (btnBounce); // add controls to panelcontrols.add (lblDelay);controls.add…arrow_forward
- Please help me fix the errors in this java program. I can’t get the program to work Class: AnimatedBall Import java.swing.*; import java.awt.*; import java.awt.event.*; public class AnimatedBall extends JFrame implements ActionListener { private Button btnBounce; // declare a button to play private TextField txtSpeed; // declare a text field to enter number private Label lblDelay; private Panel controls; // generic panel for controls private BouncingBall display; // drawing panel for ball Container frame; public AnimatedBall () { // Set up the controls on the applet frame = getContentPane(); btnBounce = new Button ("Bounce Ball"); //create the objects txtSpeed = new TextField("10000", 10); lblDelay = new Label("Enter Delay"); display = new BouncingBall();//create the panel objects controls = new Panel(); setLayout(new BorderLayout()); // set the frame layout controls.add (btnBounce); // add controls to panel controls.add (lblDelay); controls.add (txtSpeed); frame.add(controls,…arrow_forwardRecreate the following GUI in JavaFX: 2. In JavaFX, implement the functionality of making a popup appear on the screen when the 'Addworkshop' button is pressed. The popup should contain the message: 'Maximum number of workshopsreached.'arrow_forwardCreate a class RectangleExample in eclipse. Copy the following code into the class. RectangleExample.java package mypackage; import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.stage.Stage; import javafx.scene.shape.Rectangle; public class RectangleExample extends Application { Rectangle rect1, rect2; @Override public void start(Stage stage) { Group root = new Group(); //Drawing a Rectangle rect1 = new Rectangle(); //Setting the properties of the rectangle rect1.setX(20.0f); rect1.setY(20.0f); rect1.setWidth(300.0f); rect1.setHeight(10.0f); rect1.setFill(javafx.scene.paint.Color.RED); //Add to a Group object root.getChildren().add(rect1); //Drawing a Rectangle rect2 = new Rectangle(); //Setting the properties of the rectangle rect2.setX(20.0f); rect2.setY(40.0f); rect2.setWidth(200.0f); rect2.setHeight(10.0f); rect2.setFill(javafx.scene.paint.Color.GREEN); //Add to a Group object root.getChildren().add(rect2); //Creating a scene…arrow_forward
- How to create an AnimationGUI in java?arrow_forwardPlease help me create a matching game using java and 2D arrays. Create a reset button and titlearrow_forwardPlease help me fix the errors in the java program below. There are two class AnimatedBall and BouncingBall import javax.swing.*;import java.awt.*;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;public class AnimatedBall extends JFrame implements ActionListener{private Button btnBounce; // declare a button to playprivate TextField txtSpeed; // declare a text field to enterprivate Label lblDelay;private Panel controls; // generic panel for controlsprivate BouncingBall display; // drawing panel for ballContainer frame;public AnimatedBall (){// Set up the controls on the appletframe = getContentPane();btnBounce = new Button ("Bounce Ball");//create the objectstxtSpeed = new TextField("10000", 10);lblDelay = new Label("Enter Delay");display = new BouncingBall();//create the panel objectscontrols = new Panel();setLayout(new BorderLayout()); // set the frame layoutcontrols.add (btnBounce); // add controls to panelcontrols.add (lblDelay);controls.add…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
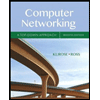
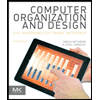
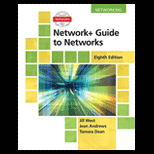
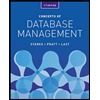
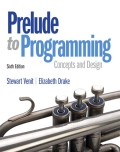
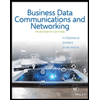