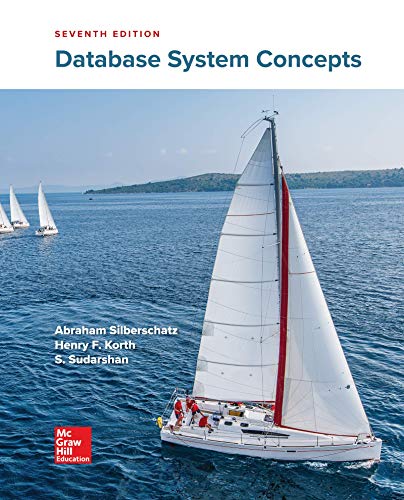
Concept explainers
Don't copy from otherwbsite i will rate
Create an appropriate Java Postfix calculator class using th ealgorithm below. Your program should accept at least five elements (data1, data2, data3, data4, data5) and will perform an appropriate postfix operation given the following scenarios:
- data1 data2 + data3 * data4 -
- data1 data2 * data3 data1 - / data4 data5 * +
- data1data3 - data2^ data4+
Use the following values for each input:
data1 = 1;
data2 = 2;
data3 = 4
data4 = 5
data5 = 3
Ensure that your program has the required class and a test class.
Algorithm evaluatePostfix(postfix)
// Evaluates postfix expression.
valueStack = a new empty stack
while (postfix has characters left to parse)
{
nextCharacter = next nonblank character of postfix
switch (nextCharacter)
{
case variable:
valueStack.push(value of the variable nextCharacter)
break
case '+' : case '-' : case '*' : case '/' : case '^' :
operabdTwo = valueStack.pop()
operandOne = valueStack.pop()
result = the result if the operation in nextCharacter and its operands
operandOne and operandTwo
valueStack.push(result)
break
default : break //Ignore unexpected characters
}
}
return valueStack.peek()

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- What am I doing wrong on this and how can I fix it? #include <iostream> #include <string> using namespace std; //declare Student Class class Student { //private member of class private: string Full_Name; float Student_GPA; int Student_Rank; //public class members public: //function to get student data void setStudentData(string studentName,float studentGpa,int studentRank) { this->Full_Name = studentName;this->Student_GPA = studentGpa;this->Student_Rank = studentRank; } //return student data function string getStudentData(string &studentName, float &studentGpa, int &StudentRank) { return Full_Name;return Student_GPA;return Student_Rank; } }; int main() { int Number_Student; //prompt user to enter total number of student cout << "Please input the total students: "; cin >> Number_Student; Student Info[Number_Student]; //variable declaration string studentName[20]; float studentGpa; int studentRank; //prompt user to enter name GPA and…arrow_forward*C Programming This exercise is to help you learn how to debug compiler warnings/errors and other common errors in your code. For each part labeled P(n), there is a warning/error/problem that goes with it. Write down what the issue was in the `Error:` section of each problem. Work on `segfault.c` along with your fixes and error comments. segfault.c file // P0#include <stdio.h>#include <stdlib.h>/* Error: */ void fib(int* A, int n); intmain(int argc, char *argv[]) {int buf[10];unsigned int i;char *str;char *printThisOne;char *word;int *integers;int foo;int *bar;char *someText; // P1for (i = 0; i <= 10; ++i) { buf[i] = i;}for (i = 0; i <= 10; ++i) { printf("Index %s = %s\n", i, buf[i]);}/* Error: */ // P2str = malloc(sizeof(char) * 10);strcpy(str, "Something is wrong");printf("%s\n", printThisOne);/* Error: */ // P3word = "Part 3";*(word + 4) = '-';printf("%s\n", word);/* Error: */ // P4*(integers + 10) = 10;printf("Part 4: %d\n", *(integers +…arrow_forwardWritten in Python It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method. Docstrings for modules, functions, classes, and methodsarrow_forward
- Write a C# program that uses a class called ClassRegistration as outlined below: The ClassRegistration class is responsible for keeping track of the student id numbers for students that register for a particular class. Each class has a maximum number of students that it can accommodate. Responsibilities of the ClassRegistration class: It is responsible for storing the student id numbers for a particular class (in an array of integers) It is responsible for adding new student id numbers to this list (returns boolean) It is responsible for checking if a student id is in the list (returns a boolean) It is responsible for getting a list of all students in the class (returns a string). It is responsible for returning the number of students registered for the class (returns an integer) It is responsible for returning the maximum number of students the class is allowed (returns an integer) It is responsible for returning the name of the class. (returns a string)arrow_forwardc++ A FastCritter moves twice as fast as a regular critter. When asked to move by n steps, it actually moves by 2 * n steps. Implement a FastCritter class derived from Critter whose move function behaves as described. Complete the following file: fastcritter_Tester.cpp #include <iostream>using namespace std; #include "critter.h" /**A FastCritter moves twice as fast as a regular critter.*/class . . .{public:void move(int steps);}; . . . int main(){FastCritter speedy;speedy.move(10);cout << speedy.get_history() << endl;cout << "Expected: [move to 20]" << endl;speedy.move(-1);cout << speedy.get_history() << endl;cout << "Expected: [move to 20, move to 18]" << endl;return 0;} Use the following file: critter.h #ifndef CRITTER_H #define CRITTER_H #include <string> #include <vector> using namespace std; /** A simulated critter. */ class Critter { public: /** Constructs a critter at position 0 with blank history. */ Critter();…arrow_forwardPLEASE ANSWER IN C++ Begin by writing a Student class. The public section has the following methods:Student::Student()Constructor. Initializes all data elements: name to empty string(s), numeric variables to 0.bool Student::ReadData(istream& in)Data input. The istream should already be open. Reads the following data, in this order:• First name (a string, 1 word)• Last name (also a string, also 1 word)• 5 quiz scores (integers, range 0-20)• 3 exam scores (integers, range 0-100)Assumes that all data is separated by whitespace. The method returns true if reading all data wassuccessful, otherwise returns false. Does not need to validate or range-check data; if one of the quiz orexam scores is out of range, just keep going.bool Student::WriteData(ostream& out) constOutput function. Writes data in the following format. Each student’s data is on one line.• First name (left justified, 20 characters)• Last name (left justified, 20 characters)• 5 quiz scores (each right justified in a…arrow_forward
- Write a C++ program, you will create a class called Books to hold the information about the books in a library. The information required for each book is as follows Book Title Author name Publisher Publish year Subject Book ID (identical 5 digits’ number) Implement the following getBookInfo () member function that receives a pointer to a book and fills it up with user data. User input validation is necessary. The user should be properly prompted for each field. void getBookInfo (Books *b) Implement the following printBookInfo () member function. void printBookInfo (Books *b) Your function should print the book in the following format: Book Title : Object Oriented Programming Using C++ Author : Chris M. Szalwinski Publisher : Amazon Year : 2016 Subject : Computer Science Book Id : 00034 Note: You need to add required constructors/destructors, member functions or data members/variables in your program to complete its execution. //Missing lines of codes to be completed by the student }…arrow_forwardQ5: Correct the following code fragment and what will be the final results of the variable a and b class A {protected int x1,y1,z; public: A(a, b,c):x1(a+2),y1 (b-1),z(c+2) { for(i=0; i<5;i++) x1++;y1++;z++;}}; class B {protected: int x,y; public: B(a,b):x(a+1),y(b+2) { for(i=0; i<5;i++) x+=2; y+=1;}}; class D:public B, virtual public A { private: int a,b; public: D(k,m,n): a(k+n), B(k,m),b(n+2),A(k,m,n) { a=a+1;b=b+1;}); int main() {Dob(4,2,5);)arrow_forwardCan I please get help writing this in C++ Write a class called Word that stores a word from a product review in a data member called “word.” The class should also contain an integer variable representing the number of times (i.e. frequency) that the word was found in a product review document. The class should have a one-argument constructor that receives a pointer to a c-string (character array) containing the word as its one parameter. (Note that the output of the strtok_s function described above is a pointer to a c-string containing the word that was parsed. This is what you will pass in to the Word constructor.) The Word constructor should also set the frequency of this Word object to 1. Appropriate set and get functions should be included for both the word and frequency data members. Write a class called Review that contains a vector of objects of the Word class. The class should contain functions to add a new Word object to the vector and to print out all of the Words in…arrow_forward
- please answer with proper explanation and step by step solution. Question: Program in python: Design a simple class called Dealership, that has two functions: buyCar(Car a) and sellCar(Car a). buyCar(Car a): Adds the car to the list of cars already in the dealership. sellCar(Car a): Removes the car from the list of cars already in the dealership. Note: Implementation of the Car class is not important for this questionarrow_forwardInstructions-Java Assignment is to define a class named Address. The Address class will have three private instance variables: an int named street_number a String named street_name and a String named state. Write three constructors for the Address class: an empty constructor (no input parameters) that initializes the three instance variables with default values of your choice, a constructor that takes the street values as input but defaults the state to "Arizona", and a constructor that takes all three pieces of information as input Next create a driver class named Main.java. Put public static void main here and test out your class by creating three instances of Address, one using each of the constructors. You can choose the particular address values that are used. I recommend you make them up and do not use actual addresses. Run your code to make sure it works. Next add the following public methods to the Address class and test them from main as you go: Write getters and…arrow_forwardQ5: Correct the following code fragment and what will be the final results of the variable a and b class A {protected int x1,y1,z; public: A(a, b,c):x1(a+2),y1 (b-1),z(c+2) { for(i=0; i<5;i++) x1++;y1++;z++;}}; class B {protected: int x,y; public: B(a,b):x(a+1),y(b+2) { for(i=0; i<5;i++) x+=2; y+=1;}}; class D:public B, virtual public A { private: int a,b; public: D(k,m,n): a(k+n), B(k,m),b(n+2),A(k,m,n) { a=a+1;b=b+1;}); int main() {Dob(4,2,5);)arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
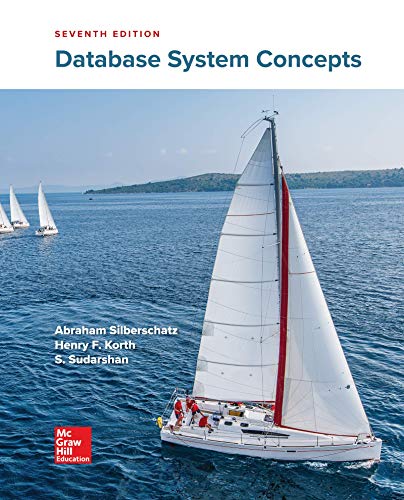
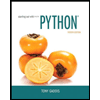
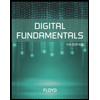
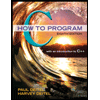
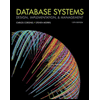
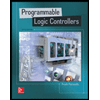