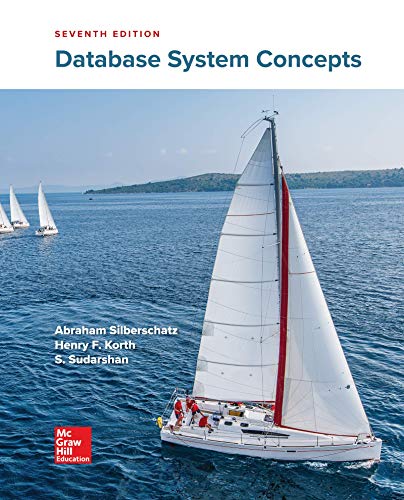
Explain the functionality of each line of code in the provided C# scripts-
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class Healthbar : MonoBehaviour
{
[SerializeField] private Health playerHealth;
[SerializeField] private Image totalHealthbar;
[SerializeField] private Image currentHealthbar;
private void Start()
{
totalHealthbar.fillAmount = playerHealth.currentHealth / 10;
}
private void Update()
{
currentHealthbar.fillAmount = playerHealth.currentHealth / 10;
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class Health : MonoBehaviour
{
[SerializeField] private float startingHealth;
public float currentHealth { get; private set; }
private Animator anim;
private void Awake()
{
currentHealth = startingHealth;
anim = GetComponent<Animator>();
}
public void TakeDamage(float _damage)
{
currentHealth = Mathf.Clamp(currentHealth - _damage, 0, startingHealth);
if (currentHealth <= 0)
{
anim.SetTrigger("death");
GetComponent<Rigidbody2D>().bodyType = RigidbodyType2D.Static;
}
}
private void OnCollisionEnter2D(Collision2D collision)
{
if (collision.gameObject.CompareTag("Spike Trap"))
{
TakeDamage(1);
}
}
private void RestartLevel()
{
SceneManager.LoadScene(SceneManager.GetActiveScene().name);
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class GroundCheck : MonoBehaviour
{
GameObject Player;
private void Start()
{
Player = gameObject.transform.parent.gameObject;
}
private void OnCollisionEnter2D(Collision2D collision)
{
if (collision.collider.tag == "Ground" ||
collision.collider.tag == "Platform")
{
Player.GetComponent<Movement2D>().isGrounded = true;
}
}
private void OnCollisionExit2D(Collision2D collision)
{
if(collision.collider.tag == "Ground"||
collision.collider.tag == "Platform")
{
Player.GetComponent<Movement2D>().isGrounded = false;
}
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlatformManager : MonoBehaviour
{
GameObject[] platforms;
GameObject currentPlatform;
int index;
public GameObject coin;
private void Start()
{
NewPlatform();
}
// Start is called before the first frame update
public void NewPlatform()
{
platforms = GameObject.FindGameObjectsWithTag("Platform");
index = Random.Range(0, platforms.Length);
currentPlatform = platforms[index];
coin.transform.position = new Vector2(currentPlatform.transform.position.x, currentPlatform.transform.position.y + 2f);
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps

- StockReader Class • This class will contain the method necessary to read and parse the .csv file of stock information. • This class shall contain a default constructor with no parameters. The constructor should be made private. . This is to prevent the class from being instantiated since the class will only contain static methods. • This class shall contain no data fields. • This class shall have a method called readStockData: . This method shall be a public, static, method. . This method shall return a StockList object once all data has been processed. . This method will take a File object as a parameter. This File object should link to the stock market data in your files folder created previously. . This method must validate that the given File object is a .csv file. If it is not, then this method shall throw an IllegalArgumentException. . This method shall read the File object and process all of the stock data into TeslaStock objects and store each object in a StockList. NOTE: The…arrow_forwardA class object can encapsulate more than one [answer].arrow_forwardDescribe the process of doing interface tests.arrow_forward
- Please fill in all the code gaps if possible: (java) public class LinkedListNode { private Object date; private LinkedListNode next; // Constructor: public LinkedListNode (Object data) // You also need to define the getter and setter: public LinkedListNode getNext() public void setNext (LinkedListNode next) public Object getData() public void setData(Object data) }arrow_forwardDraw Design Layout References Mailings Review View Help Create a Java program and name your file: FIRSTNAME. java (for example, lohn.java). Work on the following: Create three interfaces with the names "InterfaceOne," "IrnterfaceTwo," and "Interfacelhree" In the first interface, declare a method (signature only) with a name "updateGear()." In the second interface, dedare a method (signature only) with a name "accelerate()." In the third interface, dedare a method (signature only) with a name "pusherake()." Create two classes Car and Truck that implement these three interfaces at one time. Define a new method "currentSpeed()" in both the classes to find the current speed after the brake. • Define all the three methods inside each class. The data to these methods will be provided during the object creation. Invoke the two objects with a name c1 of class Car and t1 of class Truck. • After creating the objects, call all the three methods defined above in both the classes. Pass any of the…arrow_forwardRules: Corner cases. By convention, the row and column indices are integers between 0 and n − 1, where (0, 0) is the upper-left site. Throw an IllegalArgumentException if any argument to open(), isOpen(), or isFull() is outside its prescribed range. Throw an IllegalArgumentException in the constructor if n ≤ 0. Unit testing. Your main() method must call each public constructor and method directly and help verify that they work as prescribed (e.g., by printing results to standard output). Performance requirements. The constructor must take Θ(n^2) time; all instance methods must take Θ(1)Θ(1) time plus Θ(1)Θ(1) calls to union() and find().arrow_forward
- public class Artwork { // TODO: Declare private fields - title, yearCreated // TODO: Declare private field artist of type Artist // TODO: Define default constructor // TODO: Define get methods: getTitle(), getYearCreated() // TODO: Define second constructor to initialize // private fields (title, yearCreated, artist) // TODO: Define printInfo() method // Call the printInfo() method in Artist.java to print an artist's information }} public class ArtworkLabel { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String userTitle, userArtistName; int yearCreated, userBirthYear, userDeathYear; userArtistName = scnr.nextLine(); userBirthYear = scnr.nextInt(); scnr.nextLine(); userDeathYear = scnr.nextInt(); scnr.nextLine(); userTitle = scnr.nextLine(); yearCreated =…arrow_forwardpublic class VisitorPackage{// Declare data fields: an int named travelerID, // a String named destination, and // a double named totalCost.// Your code here... // Implement the default contructor.// Set the value of destination to N/A, and choose an // appropriate value for the other data fields,// Your code here... // Implement the overloaded constructor that passes new// values to all data fields.// Your code here... // Implement method getTotalCost to return the totalCost.// Your code here... // Implement method applyDiscount.//// This method applies a discount off of the total cost// of the tour. The way the discount is applied depends// on the kind of visitor.//// Child discount: the unit discount is subtracted ONCE// from the total cost of the tour.// Senior discount: the unit discount is subtracted TWICE// from the total cost of the tour.// Parameters:// - a char to indicate the kind of visitor// ('c' for child, 's' for senior)// - a double that indicates the amount of the…arrow_forwardpublic class Plant { protected String plantName; protected String plantCost; public void setPlantName(String userPlantName) { plantName = userPlantName; } public String getPlantName() { return plantName; } public void setPlantCost(String userPlantCost) { plantCost = userPlantCost; } public String getPlantCost() { return plantCost; } public void printInfo() { System.out.println(" Plant name: " + plantName); System.out.println(" Cost: " + plantCost); }} public class Flower extends Plant { private boolean isAnnual; private String colorOfFlowers; public void setPlantType(boolean userIsAnnual) { isAnnual = userIsAnnual; } public boolean getPlantType(){ return isAnnual; } public void setColorOfFlowers(String userColorOfFlowers) { colorOfFlowers = userColorOfFlowers; } public String getColorOfFlowers(){ return colorOfFlowers; } @Override public void printInfo(){…arrow_forward
- Develop a Visual C# .NET solution that provides a login authentication service. This solution will have a class library component and two application components contained within the same solution. The LoginAuthenticator Class Create a class library project named LibLoginAuthenticator that contains a class named LoginAuthenticator. The LoginAuthenticator class must support the following features: •The class defines a public string read/write property named Username which gets/sets the Username for the authenticator. • The class defines a public string property named Password which sets the Password for the authenticator. The get part should be private. •The constructor for the class should set the initial values of Username and Password to null. • The class defines a public method named Authenticate with no parameters which returns a bool? (nullable bool) value. • If either Username or Password is null, the method should return null to indicate that not all fields were provided. • If…arrow_forwardTrue or False An interface must be instantiated before it can be used.arrow_forwardpublic class Course { private String courseNumber, courseTitle; public Course() { } public Course(String courseNumber, String courseTitle) { this.courseNumber = courseNumber; this.courseTitle = courseTitle; } public void setCourseNumber(String courseNumber) { this.courseNumber = courseNumber; } public void setCourseTitle(String courseTitle) { this.courseTitle = courseTitle; } public String getCourseNumber() { return courseNumber; } public String getCourseTitle() { return courseTitle; } public void printInfo() { System.out.println("Course Information: "); System.out.println(" Course Number: " + courseNumber); System.out.println(" Course Title: " +…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
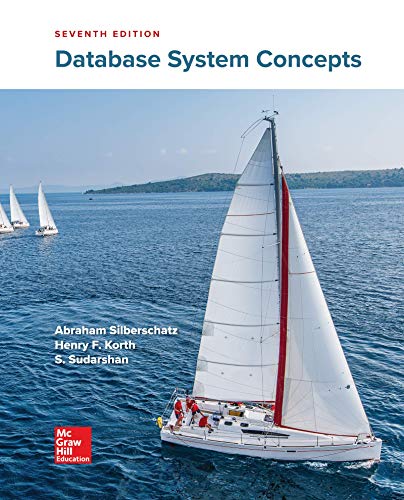
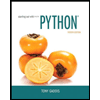
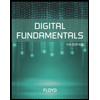
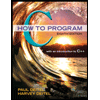
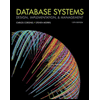
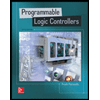