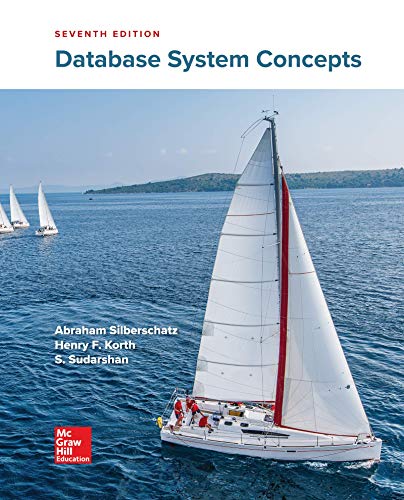
Concept explainers
Fix error codes in "the largest file" include comments
DirectoryController.java:15: error
DirectoryController.java:25
- modify code to sucessfully compile
- Include GUI components to display user input errors
- show local time for the "Last Modified" time stamp.
"LargestFileA7.java"
public class LargestFileA7 {
public static void main(String[] args) {
// Create instances of the model, view, and controller
DirectoryModel model = new DirectoryModel();
DirectoryView view = new DirectoryView();
DirectoryController controller = new DirectoryController(model, view);
}
}
"DirectoryView.java"
import javax.swing.*;
import javax.swing.border.EmptyBorder;
import java.awt.*;
import java.awt.event.*;
import java.io.IOException;
import java.nio.file.*;
import java.text.SimpleDateFormat;
import java.util.Date;
public class DirectoryView extends JFrame {
private JTextField directoryField;
private JTextArea resultArea;
private JButton browseButton;
public DirectoryView() {
setTitle("Directory Explorer");
setSize(400, 300);
setDefaultCloseOperation(EXIT_ON_CLOSE);
JPanel panel = new JPanel();
panel.setLayout(new BorderLayout());
JLabel directoryLabel = new JLabel("Enter Directory Path:");
directoryField = new JTextField(20);
browseButton = new JButton("Browse");
browseButton.addActionListener(new BrowseListener());
resultArea = new JTextArea(10, 30);
resultArea.setEditable(false);
JPanel inputPanel = new JPanel(new BorderLayout());
inputPanel.add(directoryLabel, BorderLayout.WEST);
inputPanel.add(directoryField, BorderLayout.CENTER);
inputPanel.add(browseButton, BorderLayout.EAST);
panel.add(inputPanel, BorderLayout.NORTH);
JScrollPane scrollPane = new JScrollPane(resultArea);
panel.add(scrollPane, BorderLayout.CENTER);
add(panel);
setVisible(true);
}
// Method to get the directory path entered by the user
public String getDirectoryPath() {
return directoryField.getText();
}
// Method to display the result in the text area
public void displayResult(String result) {
resultArea.setText(result);
}
// Method to display error message in a dialog box
public void displayErrorMessage(String message) {
JOptionPane.showMessageDialog(this, message, "Error", JOptionPane.ERROR_MESSAGE);
}
// Method to disable the Browse button during file traversal
public void disableBrowseButton() {
browseButton.setEnabled(false);
}
// Method to enable the Browse button after file traversal
public void enableBrowseButton() {
browseButton.setEnabled(true);
}
// ActionListener for Browse button
class BrowseListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
// Action to perform when Browse button is clicked
JFileChooser fileChooser = new JFileChooser();
fileChooser.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);
int returnValue = fileChooser.showOpenDialog(null);
if (returnValue == JFileChooser.APPROVE_OPTION) {
directoryField.setText(fileChooser.getSelectedFile().getAbsolutePath());
}
}
}
}
Please complete "DirectoryModel.Java"
import java.nio.file.*;
import java.nio.file.attribute.BasicFileAttributes;
import java.io.IOException;
public class DirectoryModel {
private Path largestFilePath;
private long largestFileSize;
public DirectoryModel() {
largestFilePath = null;
largestFileSize = 0;
}
public void findLargestFile(Path directoryPath) {
try {
Files.walkFileTree(directoryPath, new SimpleFileVisitor<Path>() {
@Override
public FileVisitResult visitFile(Path file, BasicFileAttributes attrs) throws IOException {
if (attrs.size() > largestFileSize) {
largestFileSize = attrs.size();
largestFilePath = file;
}
return FileVisitResult.CONTINUE;
Please include the "DirectoryController.java" file

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Write the code in java and please don't plagiarize or copy from other sources write it on your own. Read carefully and follow the instructions in the question. Thank you.arrow_forwardWrite the code in java and understand what the question says and give me the code and don't copy or plagiarize pleasearrow_forwardJava, the user selects an item from the text files ranging 1-15, repeats the loop until 'q'. the program provides a list of what they ordered including discounts, then calculates the total bill and how much they saved. The bottom is my the code I'm working on class BillProcessor {public static void prepareBill(LinkedHashMap<String, Integer> purchases, LinkedHashMap<String,Double>items,LinkedHashMap<String,String> sales, ArrayList<String> itemNames){double totalCost, totalDiscount, actualCost, discount; //note actualCost is the price of a single itemtotalCost = 0.00;totalDiscount = 0.00;actualCost = 0.00;discount = 0.00;for(Map.Entry<String,Integer>set: purchases.entrySet()) {if (sales.get(set.getKey()) != null) {if (sales.get(set.getKey()).equals("bogo") == true) {actualCost = set.getValue() * items.get(set.getKey());totalCost += actualCost;System.out.println("Item Name : " + set.getKey() + "\tItem Quantity(bogo) : " + (set.getValue() * 2 + "\tItem Cost :…arrow_forward
- Please help me using java. I am not understanding why I am getting errorsarrow_forwardJava Program ASAP Modify this program so it passes the test cases in Hypergrade becauses it says 5 out of 7 passed. Also I need one one program and please correct and change the program so that the numbers are in the correct places as shown in the correct test case. import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;import java.util.ArrayList;import java.util.Arrays;import java.util.InputMismatchException;import java.util.Scanner;public class FileSorting { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); while (true) { System.out.println("Please enter the file name or type QUIT to exit:"); String fileName = scanner.nextLine(); if (fileName.equalsIgnoreCase("QUIT")) { break; } try { ArrayList<String> lines = readFile(fileName); if (lines.isEmpty()) { System.out.println("File " +…arrow_forwardIm trying ro read a csv file and store it into a 2d array but im getting an error when I run my java code. my csv file contains 69 lines of data Below is my code: import java.util.Scanner; import java.util.Arrays; import java.util.Random; import java.io.File; import java.io.FileNotFoundException; import java.io.FilenameFilter; public class CompLab2 { public static String [][] getEarthquakeDatabase (String Filename) { //will read the csv file and convert it to a string 2-d array String [][] Fileinfo = new String [69][22]; int counter = 0; File file = new File(Filename); try { Scanner scnr = new Scanner(file); scnr.nextLine(); //skips the label in the first row of the file while (scnr.hasNextLine()) { // this while loop will count the number of values in the usgs file counter += 1; // increases by one each time a line is read scnr.nextLine(); } while…arrow_forward
- Java. Please use the templatearrow_forwardIn Java Assume code that has imported Scanner and instantiated it for keyboard input as stdln. Wtire a code fragment that does the following: 1. Uses a for loop to fill each array element with the square root of that elements index valuearrow_forwardin java Integer numVals is read from input and integer array userCounts is declared with size numVals. Then, numVals integers are read from input and stored into userCounts. If the first element is less than the last element, then assign Boolean firstSmaller with true. Otherwise, assign firstSmaller with false. Ex: If the input is: 5 40 22 41 84 77 then the output is: First element is less than last element 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 import java.util.Scanner; public class UserTracker { publicstaticvoidmain(String[] args) { Scannerscnr=newScanner(System.in); intnumVals; int[] userCounts; inti; booleanfirstSmaller; numVals=scnr.nextInt(); userCounts=newint[numVals]; for (i=0; i<userCounts.length; ++i) { userCounts[i] =scnr.nextInt(); } /* Your code goes here */ if (firstSmaller) { System.out.println("First element is less than last element"); } else { System.out.println("First element is not less…arrow_forward
- Write all the code within the main method in the TestTruck class below. Implement the following functionality. Construct two Truck objects: one with any make and model you choose and the second object with gas tank capacity 10. If an exception occurs, print the stack trace. Print both Truck objects that were constructed. import java.lang.IllegalArgumentException ; public class TestTruck { public static void main( String[] args ) { // write your code herearrow_forwardpackage Q2;import java.io.FileInputStream;import java.io.FileNotFoundException;import java.util.ArrayList;import java.util.Scanner;public class Question2 {public static void main(String[] args) throws FileNotFoundException {/*** Part a* Finish creating an ArrayList called NameList that stores the names in the file Names.txt.*/ArrayList<String> NameList;/*** Part b* Replace null on the right-hand-side of the declaration of the FileInputStream object named inputStream* so that it is initialized correctly to the Names.txt file located in the folder specified in the question description*/FileInputStream inputStream = null;Scanner scnr = new Scanner(inputStream); //Do not modify this line of code/*** Part c* Using a loop and the Scanner object provided, read the names from Names.txt* and store them in NameList created in Part a.*//*** Part d* Reorder the names in the ArrayList so that they appear in reverse alphabetical order.*/// System.out.println("NameList after correct ordering: "…arrow_forwardPlease help me code in java: Write a program that reads two files “Data1.txt”, “Data2.txt”; adding their corresponding elements produces an output file “output.txt”. If the number of elements are not equal, fill the elements of the smaller file up with “0” s. Sample output: Elements of the “Data1.txt”: 3 5 7 8 9 Elements of the “Data2.txt”: 45 11 Elements of the “Output.txt”: 48 16 7 8arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
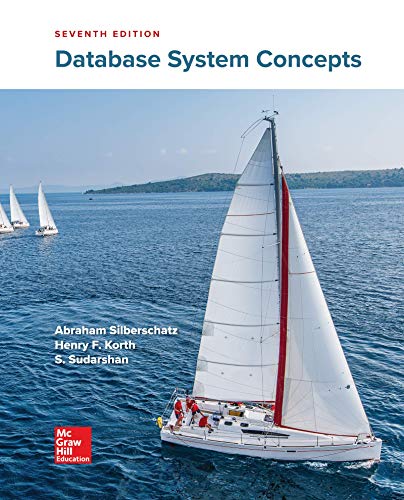
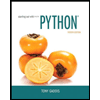
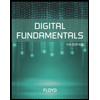
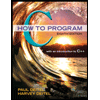
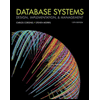
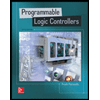