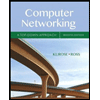
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Im trying ro read a csv file and store it into a 2d array but im getting an error when I run my java code. my csv file contains 69 lines of data
Below is my code:
import java.util.Scanner;
import java.util.Arrays;
import java.util.Random;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FilenameFilter;
public class CompLab2 {
public static String [][] getEarthquakeDatabase (String Filename) { //will read the csv file and convert it to a string 2-d array
String [][] Fileinfo = new String [69][22];
int counter = 0;
File file = new File(Filename);
try {
Scanner scnr = new Scanner(file);
scnr.nextLine(); //skips the label in the first row of the file
while (scnr.hasNextLine()) { // this while loop will count the number of values in the usgs file
counter += 1; // increases by one each time a line is read
scnr.nextLine();
}
while (scnr.hasNext()) { // reads through csv file while there is a line of data
String data = scnr.nextLine();
String[] values = data.split(","); // splits the data at the commas
double closingValue = Double.parseDouble(values[4]);
System.out.println(values[4]); // prints the data at element 5
}
scnr.close(); // closes the scanner
} catch (FileNotFoundException e) { //throws exception if file is not found
e.printStackTrace();
}
return Fileinfo;
}
public static void main(String[] args) {
String nameOfFile = "query(2).csv"; //stores the csv file in a string
String [][] earthquakeDatabase = getEarthquakeDatabase (nameOfFile); // creates 2d string array thats equal to method getEarthquakeDatabase
System.out.println("Expected number of rows: 69");
System.out.println("Number of rows from the array: " +earthquakeDatabase);
double [] earthquakeValues = getEarthquakeValues(earthquakeDatabase);
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Similar questions
- Java Question- Convert this source code into a GUI application using JOptionPane. Make sure the output looks similar to the following picture. Thank you. import java.util.Calendar;import java.util.TimeZone;public class lab11_5{public static void main(String[] args){//menuSystem.out.println("-----------------");System.out.println("(A)laska Time");System.out.println("(C)entral Time");System.out.println("(E)astern Time");System.out.println("(H)awaii Time");System.out.println("(M)ountain Time");System.out.println("(P)acific Time");System.out.println("-----------------"); System.out.print("Enter the time zone option [A-P]: "); //inputString tz = System.console().readLine();tz = tz.toUpperCase(); //change to uppercase //get current date and timeCalendar cal = Calendar.getInstance();TimeZone.setDefault(TimeZone.getTimeZone("GMT"));System.out.println("GMT/UTC:\t" + cal.getTime()); switch (tz){case "A":tz =…arrow_forwardImplement the Board class. Make sure to read through those comments so that you know what is required. import java.util.Arrays; import java.util.Random; public class Board { // You don't have to use these constants, but they do make your code easier to read public static final byte UR = 0; public static final byte R = 1; public static final byte DR = 2; public static final byte DL = 3; public static final byte L = 4; public static final byte UL = 5; // You need a random number generator in order to make random moves. Use rand below private static final Random rand = new Random(); private byte[][] board; /** * Construct a puzzle board by beginning with a solved board and then * making a number of random moves. Note that making random moves * could result in the board being solved. * * @param moves the number of moves to make when generating the board. */ public Board(int moves) { // TODO } /** * Construct a puzzle board using a 2D array of bytes to indicate the contents *…arrow_forwardPlease help and thank you in advance. The language is Java. I have to write a program that takes and validates a date but it won't output the full date. Here is my current code: import java.util.Scanner; public class P1 { publicstaticvoidmain(String[]args) { // project 1 // date input // date output // Create a Scanner object to read from the keyboard. Scannerkeyboard=newScanner(System.in); // Identifier declarations charmonth; intday; intyear; booleanisLeapyear; StringmonthName; intmaxDay; // Asks user to input date System.out.println("Please enter month: "); month=(char)keyboard.nextInt(); System.out.println("Please enter day: "); day=keyboard.nextInt(); System.out.println("Please enter year: "); year=keyboard.nextInt(); // Formating months switch(month){ case1: monthName="January"; break; case2: monthName="February"; break; case3: monthName="March"; break; case4: monthName="April"; break; case5: monthName="May"; break; case6: monthName="June"; break; case7:…arrow_forward
- I need help with a Java program described below: import java.util.Scanner;import java.io.FileInputStream;import java.io.IOException; public class LabProgram { public static void main(String[] args) throws IOException { Scanner scnr = new Scanner(System.in); /* Type your code here. */ }}arrow_forwardimport java.util.Scanner;import java.io.FileInputStream;import java.io.IOException; public class LabProgram { public static void main(String[] args) throws IOException { Scanner scnr = new Scanner(System.in); /* Type your code here. */ }}arrow_forwardStringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forward
- need help finishing/fixing this java code so it prints out all the movie names that start with the first 2 characters the user inputs the code is as follows: Movie.java: import java.io.File;import java.io.FileNotFoundException;import java.util.ArrayList;import java.util.Scanner;public class Movie{public String name;public int year;public String genre;public static ArrayList<Movie> loadDatabase() throws FileNotFoundException {ArrayList<Movie> result=new ArrayList<>();File f=new File("db.txt");Scanner inputFile=new Scanner(f);while(inputFile.hasNext()){String name= inputFile.nextLine();int year=inputFile.nextInt();inputFile.nextLine();String genre= inputFile.nextLine();Movie m=new Movie(name, year, genre);//System.out.println(m);result.add(m);}return result;}public Movie(String name, int year, String genre){this.name=name;this.year=year;this.genre=genre;}public boolean equals(int year, String genre){return this.year==year&&this.genre.equals(genre);}public String…arrow_forwardSubmit the code (copy&paste) with a screen shot of the same sample run as below. 1. Write a class ArrayMethods, where the main method asks the user to enter the num ber of inputs and stores them in an array called myList. Create a method printArray that prints the array myList. Create another method copyArray that copies the array using System.arraycopy, and multiplies each element in the copied array by 2, then finally returns the array to the main method. Invoke the printarray again to print the array returned by copyArray. In summary, see below. main method does: asks user for inputs and stores in array. Invoke printArray. Invoke copyArray. Invoke printArray again. printArray: void method, parameters passed is an array, prints an array copyArray: array-returningmethod, parameters passedis an array, copies array, multiplies 2 to each element in the copied array, returnsthe copied arrayarrow_forwardModify this code to make a moving animated house. import java.awt.Color;import java.awt.Font;import java.awt.Graphics;import java.awt.Graphics2D; /**** @author**/public class MyHouse { public static void main(String[] args) { DrawingPanel panel = new DrawingPanel(750, 500); panel.setBackground (new Color(65,105,225)); Graphics g = panel.getGraphics(); background(g); house (g); houseRoof (g); lawnRoof (g); windows (g); windowsframes (g); chimney(g); } /** * * @param g */ static public void background(Graphics g) { g.setColor (new Color (225,225,225));//clouds g.fillOval (14,37,170,55); g.fillOval (21,21,160,50); g.fillOval (351,51,170,55); g.fillOval (356,36,160,50); } static public void house (Graphics g){g.setColor (new Color(139,69,19)); //houseg.fillRect (100,250,400,200);g.fillRect (499,320,200,130);g.setColor(new Color(190,190,190)); //chimney and doorsg.fillRect (160,150,60,90);g.fillRect…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
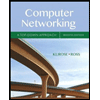
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
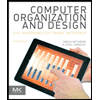
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
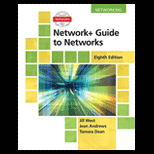
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
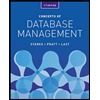
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
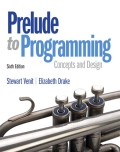
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
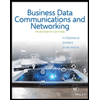
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY