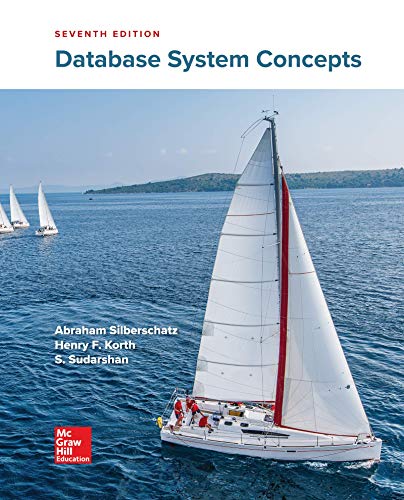
Help
/**
* Implement the method below, which takes a two-dimensional array of integers
* as the input argument and returns the total number of <strong>distinct
* pairs</strong> (a, b) where both a and b are even numbers and a is less than
* b ({@code a < b} ). Your code should use nested for loops.
*
* <p>
* For example:
* </p>
*
* <pre>
* if inputarray = { {9, 10, 3, 4, 3}, {1, 6, 2}, {4, 24, 46, 0}, {15, 9, 45, 57} } then return 21
* note we have 21 distinct pairs within this 2D array as follows
* [0, 2],[0, 4],[0, 6],[0, 10],[0, 24],[0, 46],[2, 4],[2, 6],
* [2, 10],[2, 24],[2, 46],[4, 6],[4, 10],[4, 24],[4, 46],[6, 10],
* [6, 24],[6, 46],[10, 24],[10, 46],[24, 46]
* if inputarray = { {1, 6, 1}, {5, 9, 15, 20}, {23, 33, 99} } then return 1
* if inputarray = { {4, 21, 43, 1}, {22, 32, 42, 52} } then return 10
* if inputarray = { {15, 9, 45, 57}, {13, 17, 23} }then return 0
* </pre>
*
* @param inputarray 2D int input array
* @return the number of distinct pairs (a, b) where both a and b are even
* numbers and {@code a < b}.
*/
publicstaticintcountEvenPairs2D(int[][]inputarray){
/*
* Your implementation of this method starts here. Recall that : 1. No
* System.out.println statements should appear here. Instead, you need to return
* the result. 2. No Scanner operations should appear here (e.g.,
* input.nextInt()). Instead, refer to the input parameters of this method.
*/

Step by stepSolved in 2 steps

- The code in the picture takes two sorted arrays of sizes m and n, then it merges and sorts them. Whats the best-case and worst-case for this alogrithm?arrow_forwardGiven the Array declaration below, determine the output: int T[8] = {2, 3, 7, 9, -1, 2, 4, 5}; int x = 5; cout << T[x-2] / T[12-2*x]; int y = -3; cout << pow(T[y+4],T[3-y]);arrow_forward8arrow_forward
- Python Please Recap of two-dimensional arrays and their numpy implementation ** uploaded image ^ 1) In this problem, implement a TwoDArray class that is meant to mimic (some of) the functionality of a two-dimensional numpy array. DO NOT use numpy at any point. 1A) Give the class an __init__ method Make sure that the variable array is a valid input. This means you should a) Make sure that array is a list of lists. and b) Make sure that each of the inner lists has the same length. You do not need to check for anything else. If these conditions are not met you should raise the appropriate error(s). It is up for you to decide if you should raise a TypeError, KeyError, IndexError, ValueError, ZeroDivisionError, etc. When raising the error, you should also give an informative error message. With actual numpy arrays, all numbers are required to be the same datatype. You don't have to worry about that here. You can also assume that all numbers are ints or floats so you only have to worry…arrow_forwardin C++ Write a method ‘void addBack(double x)’ that adds value x to the back of a linked list. Assume you have access to a ‘head’ pointer pointing to the first node in the list. Please note that you do NOT have a tail pointer. Be sure to check for any special cases.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
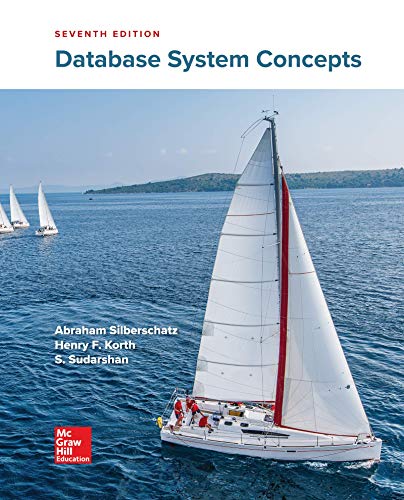
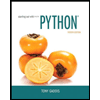
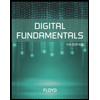
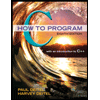
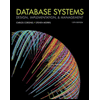
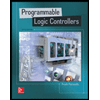