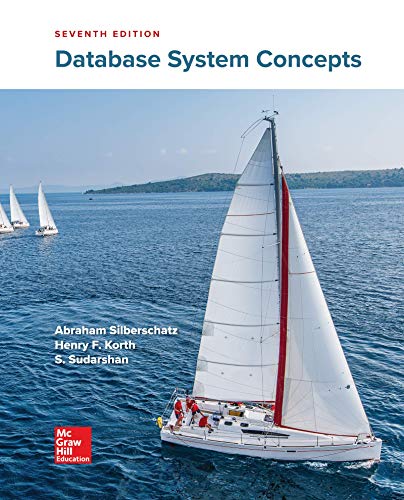
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
How can I make the do-while loop include user-friendly behavior through the addition of input validation to the code to make sure the user's color selections are within the permitted range (0 to 2) and to prompt them to re-enter if necessary?
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
/*
the function 'mixColors' takes the 'color' variable prompted from the 'getUserInput'
function then uses a series of if statements to determine what mixed color two colors ('color1'
and 'color2') create: 'purple', 'orange', or 'green' with 'No mix' elsewise. 'mixedColor'
is returned as a result
*/
string mixColors(int color1, int color2) {
string mixedColor;
if ((color1 == 0 && color2 == 1) || (color1 == 1 && color2 == 0)) {
mixedColor = "purple";
} else if ((color1 == 0 && color2 == 2) || (color1 == 2 && color2 == 0)) {
mixedColor = "orange";
} else if ((color1 == 1 && color2 == 2) || (color1 == 2 && color2 == 1)) {
mixedColor = "green";
} else {
mixedColor = "No Mix";
}
return mixedColor;
}
/*
the 'getUserInput' function is called before the 'mixColors' function to retrieve
user input storing data from user into the color 'variable' using 'main' functions array
*/
int getUserInput() {
int color;
cout << "Enter a prime color: " << endl;
cout << "'0' for red, '1' for blue', and '2' for yellow: ";
cin >> color;
return color;
}
/*
the 'main' function is the basis of the program!
the 'main' function contains: an array of prime colors used in the 'getUserInput' function;
int variables 'color1' and 'color2'; a string varible 'mixedColor' from the 'mixColors'
function; a vairable 'choice' for the do-while loop inside this 'main' function; an ofstream
that creates and opens a file named 'color_mixer_results.txt' with an 'outputFile'
created/opened to truncate user results until user prompts exit with the do while loop set
to mix again if answer is 'y' or 'Y' (exits with all other entries)
*/
int main() {
string arr[] = {"red", "blue", "yellow"};
int color1, color2;
string mixedColor;
char choice;
ofstream outputFile("color_mixer_results.txt"); // opens file that truncates
do {
color1 = getUserInput();
color2 = getUserInput();
mixedColor = mixColors(color1, color2);
// below is where input inside do while loop is writ to .txt file
outputFile << "Mixed color for your selection (" << color1 << " and " << color2 << ") is " << mixedColor << endl;
cout << "Mixed color for your selection (" << color1 << " and " << color2 << ") is " << mixedColor << endl;
cout << "Would you like to mix again? (Y/N): ";
cin >> choice;
} while (toupper(choice) == 'Y');
outputFile.close(); // closing output file
cout << "Results have been saved in 'color_mix_results.txt'. Goodbye!" << endl;
return 0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- create a new file in c++. In this lab, you will add some more functionality to the program. Use loops to keep running the program until the user chooses the exit condition (X or x). When dividing, use a loop to validate user input, making sure the denominator (second number) is not zero. If the user enters a 0 for the second number, display an error message and ask them again. A) Add two numbersB) Subtract two numbersC) Multiply two numbersD) Divide two numbersX) Exit program The program should: display a "hello" message before presenting the menu display the menu prompt user for selection accept either uppercase or lowercase for selection display error message if invalid selection is made (example: E) display the selection back to the user prompt for two numbers perform the operation and display the results use loop for input validation of second number during division display error message for division when second number is 0 after displaying result, use loop to…arrow_forwardThe class I'm taking is assembly programming. I am completely stuck. I have attached the problem. Please view attachment before answering. Thank you so much for your help! Any help is greatly appreciated.arrow_forwardThe language is Java. The method to use is listed in the picture.arrow_forward
- Can you help me write the following C++ program: Add a search command to the file viewer created. Thiscommand asks the user for a string and finds the first line that containsthat string. The search starts at the first line that is currently displayedand stops at the end of the file. If the string is found, then the file willbe scrolled down so that when it is redisplayed, the line that contains thestring is the first line at the top of the window.If the string is not found, the program prints the following error messageat the top of the screen:ERROR: string X was not foundwhere X is replaced by the string entered by the user. In that case, theprogram redisplays the lines that the user was viewing before the searchcommand was executed.Modify the program as little as possible. Make sure you assign new responsibilities to the most appropriate components. Use the C++ string operation find. The file viewer code is given below: // FileViewer.cpp #include "FileViewer.h" using namespace…arrow_forwardCreate a new file (in Dev C++) and save it as lab7_XYZ.cpp (replace XYZ with your initials). In Lab 2, you created a menu for a simple calculator program. In Lab 6, you added some functionality based on the user selection. In this lab, you will add some more functionality to the program. Use loops to keep running the program until the user chooses the exit condition (9). When dividing, use a loop to validate user input, making sure the denominator (second number) is not zero. If the user enters a 0 for the second number, display an error message and keep prompting until a non-zero number is entered. 1) Add two numbers2) Subtract two numbers3) Multiply two numbers4) Divide two numbers 9) Exit program The program should: contain header comments as shown in class display a hello message before presenting the menu display the menu prompt user for selection echo the selection back to the user if the selection was invalid, display error message to user and then loop back to menu…arrow_forward(16) 6. You want to write a Java application that creates a while loop that runs until the user enters a 0 as input. Each time the while loop runs, it displays a menu of three choices as will be shown in the sample run below. When the program reads in the user's choice, that choice should be read in as a String called choiceStr. Then, choiceStr should be converted into an int called choice by using the code below: int choice Integer.parseInt(choiceStr); If the user enters a 1 for choice == 1, the program will print "Choice 1" to the screen. If the user enters a 2 for choice == 2, the program will print "Choice 2" to the screen. Here is a sample run: 0 - to quit 1 to print Choice 1 2 to print Choice 2 Enter choice: 1 Choice 1 0 - to quit 1 to print Choice 1 2 to print Choice 2 Enter choice: 1 Choice 1 0 to quit 1 to print Choice 1 2 to print Choice 2 Enter choice: 2 Choice 2 0 - to quit 1 to print Choice 1 2 to print Choice 2 Enter choice: 0arrow_forward
- Summary In this lab, you write a while loop that uses a sentinel value to control a loop in a Python program. You also write the statements that make up the body of the loop. Each theater patron enters a value from 0 to 4 indicating the number of stars that the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. Instructions Make sure the file MovieGuide.py is selected and open. Write thewhile loop using a sentinel value to control the loop, and also write the statements that make up the body of the loop. Execute the program by clicking the Run button at the bottom of the screen. Input the following as star ratings: 0, 3, 4, 4, 1, 1, 2, -1 must be written with this code: """ MovieGuide.py This program allows each theater patron to enter a value from 0 to 4 indicating the number of stars that the patron awards to the Guide's featured movie of the week. The program executes…arrow_forwardAgain, modify the while loop to utilize tolower() or toupper(). Create two more functions (options #3 and #4 in your menu) by taking the to_kilograms() and to_pounds() functions and modifying them to use reference variables instead of normal pass by value variables. Name them: to_kilograms_ref() to_pounds_ref() Create another two functions (options #5 and #6 in your menu) by taking the to_kilograms() and to_pounds() functions and modifying them to use pointers instead of normal pass by value variables. Name them: to_kilograms_ptr() to_pounds_ptr() Your new Menu should look like this, which includes what type of variables are being used: MENU 1. Kilograms to Pounds (pass by value) 2. Pounds to Kilograms (pass by value) 3. Kilograms to Pounds (pass by reference) 4. Pounds to Kilograms (pass by reference) 5. Kilograms to Pounds (using pointers) 6. Pounds to Kilograms (using pointers) Example: #include <iostream> #include <cmath>…arrow_forwardIn this lab, you write a while loop that uses a sentinel value to control a loop in a Python program. You also write the statements that make up the body of the loop. The source code file already contains the necessary assignment and output statements. Each theater patron enters a value from 0 to 4 indicating the number of stars that the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. At the end of the program, you should display the average star rating for the movie.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
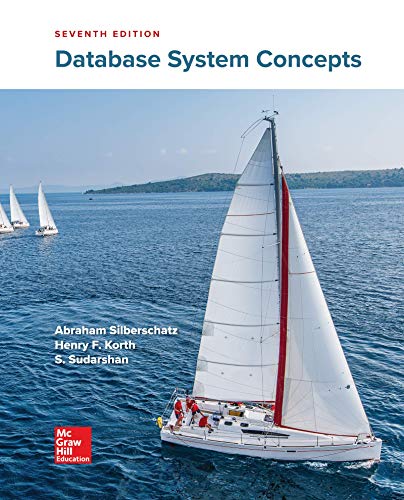
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
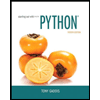
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
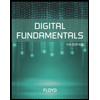
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
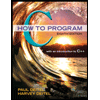
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
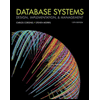
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
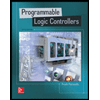
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education