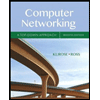
How do I change the error to a success?
import java.lang.Math;
import java.util.Scanner;
public class MidtermProblems
{
public static String odds(int a)
{
int odds;
odds = a;
String s;
if(odds > 0)
{
//s = odds;
return "" + odds;
}
else if(odds < 0)
{
s = "None";
}
else
{
s = "";
}
return s;
}
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
int a=0;
a = in.nextInt();
//Add Loop to get odd Numbers
if(a<0) {
System.out.println(odds(a));
}
else {
for(int i=1;i<=a;i=i+2){
System.out.print(odds(i)+" ");
}
}
//String result = odds(a);Not Required
//result = Main.odds(a); Not Required
}
}


Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Create a Flowchart //Java Source Code :- import java.util.Scanner; public class bExpert { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.println("\nVALUES"); System.out.print("Value 1: "); int v1 = sc.nextInt(); System.out.print("Value 2: "); int v2 = sc.nextInt(); System.out.print("Value 3: "); int v3 = sc.nextInt(); System.out.println("\nPROCESS"); System.out.println("\n1. Summation"); System.out.println("2. Product"); System.out.println("3. Min / Max"); System.out.println("4. Odd / Even"); char st = 'y'; while(st != 'n'){ System.out.print("\nCHOICE: "); int ch = sc.nextInt(); switch(ch) { case 1: int sum = v1 + v2 + v3; System.out.println("\nSum = "+sum); break; case 2: int prod =…arrow_forwardJAVA CODE: check outputarrow_forwardCreate the UML Diagram for this java code import java.util.Scanner; interface Positive{ void Number();} class Square implements Positive{ public void Number() { Scanner in=new Scanner(System.in); System.out.println("Enter a number: "); int a = in.nextInt(); if(a>0) { System.out.println("Positive number"); } else { System.out.println("Negative number"); } System.out.println("\nThe square of " + a +" is " + a*a); System.out.println("\nThe cubic of "+ a + " is "+ a*a*a); }} class Sum implements Positive{ public void Number() { Scanner in = new Scanner(System.in); System.out.println("\nEnter the value for a: "); int a = in.nextInt(); System.out.println("Enter the value for b" ); int b= in.nextInt(); System.out.printf("The Difference of two numbers: %d\n", a-b); System.out.printf("The…arrow_forward
- import java.util.Scanner; public class StateInfo { /* Your code goes here */ public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String stateCode; String stateName; stateCode = scnr.next(); stateName = scnr.next(); printStateInfo(stateCode, stateName); }}arrow_forwardimport java.util.Scanner; public class AverageWithSentinel{ public static final int END_OF_INPUT = -500; public static void main(String[] args) { Scanner in = new Scanner(System.in); // Step 2: Declare an int variable with an initial value // as the count of input integers // Step 3: Declare a double variable with an initial value // as the total of all input integers // Step 4: Display an input prompt // "Enter an integer, -500 to stop: " // Step 5: Read an integer and store it in an int variable // Step 6: Use a while loop to update count and total as long as // the input value is not -500. // Then display the same prompt and read the next integer // Step 7: If count is zero // Display the following message // "No integers were…arrow_forwardimport java.util.Scanner; public class leapYearLab { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int userYear; boolean LeapYear; System.out.println("Enter the year"); userYear = scnr.nextInt(); isLeapYear(userYear); //if leap year if (isLeapYear){ System.out.println(userYear + " - leap year"); } else{ System.out.println(userYear + " - not a leap year"); } scnr.close(); } public static boolean isLeapYear(int userYear){ boolean LeapYear; /* Type your code here. */ if ( userYear % 4 == 0) { // checking if year is divisible by 100 if ( userYear % 100 == 0) { // checking if year is divisible by 400 // then it is a leap year if ( userYear % 400 == 0) LeapYear = true; else LeapYear = false; } // if the year is not divisible by 100 else…arrow_forward
- import java.util.Scanner; public class CharMatch { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String userString; char charToFind; int strIndex; userString = scnr.nextLine(); charToFind = scnr.next().charAt(0); strIndex = scnr.nextInt(); /* Your code goes here */ }}arrow_forwardThis is my program. I need help with the math. import java.util.*;import javax.swing.JOptionPane;public class Craps { public static void main(String[] args) { int Bet, Die; int WinC = 0, LossC = 0, GameC = 0, GameN = 1; double startingBal = 0, curBal = 0; double balD, balDe, balDec, balI, balIn, balInc; char Answer = '\0'; Scanner crapsGame = new Scanner(System.in); System.out.println("Welcome to the Craps Game"); curBal = StartingBal(startingBal); System.out.println("Your starting balance is: $" + curBal); do { System.out.println("Please input your bet for the game>> "); Bet = crapsGame.nextInt(); System.out.println("Game #" + GameN + " Starting with bet of: $" + Bet ); if (Bet < 1 || Bet > curBal) { System.out.println("Invalid bet – Your balance is only: $" + curBal); while (Bet < 1 || Bet > curBal)…arrow_forwardimport java.util.Scanner; public class RomanNumerals { public static void main(String[] args) { Scanner in = new Scanner("I C X D M L"); char romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 1") ; romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 100") ; romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 10") ; romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 500") ; romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 1000") ; romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 50") ; } /** Gives the value…arrow_forward
- import java.util.Scanner; public class CircleAndSphereWhileLoop{ public static final double MAX_RADIUS = 500.0; public static void main(String[] args) { Scanner in = new Scanner(System.in); // Step 2: Read a double value as radius using prompt // "Enter the radius (between 0.0 and 500.0, exclusive): " // Step 3: While the input radius is not in the ragne (0.0, 500.0) // Display a message on one line (ssuming input value -1) // "The input number -1.00 is out of range." // Read a double value as radius using the same promt double circumference = 2 * Math.PI * radius; double area = Math.PI * radius * radius; double surfaceArea = 4 * Math.PI * Math.pow(radius, 2); double volume = (4 / 3.0) * Math.PI * Math.pow(radius, 3); // Step 4: Display the radius, circle circumference, circle area, // sphere surface area, and…arrow_forwardStringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forwardimport java.util.Scanner; public class LabProgram { /* Define your method here */ public static void main(String[] args) { Scanner scnr = new Scanner(System.in); /* Type your code here. */ }}arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
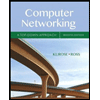
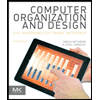
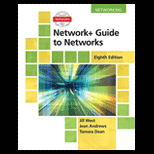
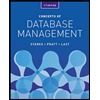
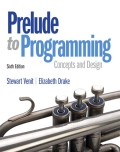
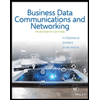