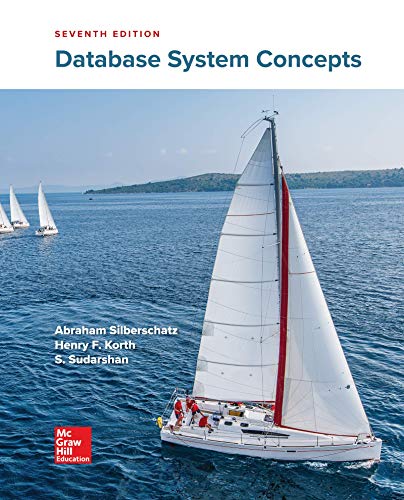
I got errors in parser.java. How to create methods in TokenHandler.java for getCurrentToken(), consumeToken(), getLastMatchedToken()? Please create these methods in TokenHandler.java. Fix the error for programNode that is attached. Below is TokenHandler.java
TokenHandler.java
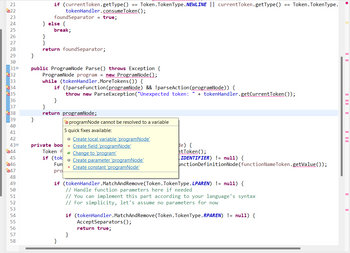

To create the methods getCurrentToken(), consumeToken(), and getLastMatchedToken() in TokenHandler.java, you can modify the class as follows:
import java.util.LinkedList;
import java.util.Optional;
import mypack.Token.TokenType;
public class TokenHandler {
private LinkedList<Token> tokens; // List of tokens
private Token currentToken;
private Token lastMatchedToken;
public TokenHandler(LinkedList<Token> tokens) {
this.tokens = tokens;
this.currentToken = null;
this.lastMatchedToken = null;
}
public Optional<Token> Peek(int j) {
if (j < tokens.size()) {
return Optional.of(tokens.get(j));
} else {
return Optional.empty();
}
}
public boolean MoreTokens() {
return !tokens.isEmpty();
}
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- Java Code: Create a Parser class. Much like the Lexer, it has a constructor that accepts a LinkedList of Token and creates a TokenManager that is a private member. The next thing that we will build is a helper method – boolean AcceptSeperators(). One thing that is always tricky in parsing languages is that people can put empty lines anywhere they want in their code. Since the parser expects specific tokens in specific places, it happens frequently that we want to say, “there HAS to be a “;” or a new line, but there can be more than one”. That’s what this function does – it accepts any number of separators (newline or semi-colon) and returns true if it finds at least one. Create a Parse method that returns a ProgramNode. While there are more tokens in the TokenManager, it should loop calling two other methods – ParseFunction() and ParseAction(). If neither one is true, it should throw an exception. bool ParseFunction(ProgramNode) bool ParseAction(ProgramNode) -Creates ProgramNode,…arrow_forwardThe Java classes GenericServlet and HttpServlet may be differentiated from one another with the assistance of an example.arrow_forwardin java please.Create a method from the implementation perspective. Create a method where it takes in a linked chain of values and adds them in order to the front. method header:public void addToFront(ANode<T> first)arrow_forward
- VariableReferenceNode.java, OperationNode.java, ConstantNode.java, and PatternNode.java must have their own java classes with the correct methods implemented: OperationNode: Has enum, left and Optional right members, good constructors and ToString is good VariableReferenceNode: Has name and Optional index, good constructors and ToString is good Constant & Node Pattern: Have name, good constructor and ToString is good Make sure to include the screenshot of the output of Parser.java as well.arrow_forwardIn our demo program, we compared the efficiency of ArrayList and LinkedList byadding a number of items to beginning of these two kinds of lists. Which kind of list(ArrayList or LinkedList) takes less time to add? Briefly explain why is that? Youmay draw diagrams to explain.arrow_forwardfrom Library.javaQ: Write the class names with extends keyword linkedlist ?arrow_forward
- Java Code: Below is Parser.java and there are errors. getType() and getStart() is undefined for the type Optional<Token> and there is an error in addNode(). Make sure to get rid of all the errors in the code. Attached is images of the errors. Parser.java import java.text.ParseException;import java.util.LinkedList;import java.util.List;import java.util.Optional; import javax.swing.ActionMap; public class Parser { private TokenHandler tokenHandler; private LinkedList<Token> tokens; public Parser(LinkedList<Token> tokens) { this.tokenHandler = new TokenHandler(tokens); this.tokens = tokens; } public boolean AcceptSeparators() { boolean foundSeparator = false; while (tokenHandler.MoreTokens()) { Optional<Token> currentToken = tokenHandler.getCurrentToken(); if (currentToken.getType() == Token.TokenType.NEWLINE || currentToken.getType() == Token.TokenType.SEMICOLON) {…arrow_forwardPlease answer question. This is pertaining to Java programming language 2-14arrow_forwardPlease Code in JAVA. Type the code out neatly. Add comments . (No need for long comments). Dont add any unnecesary code or comments. Do what the attached file says. And call it a day. Thanks !arrow_forward
- The Java classes GenericServlet and HttpServlet may be differentiated from one another with the assistance of an example.arrow_forwardwrite a default constructor for class Table(in java) here's the the method signature public static int ceiling (double num) { return num <=0? (int) num : (int) num +1; }arrow_forwardCode with java please. Critique the following code which is intended to print out whether or not a key value k1 is used in a map named relationships: if (relationships.get(k1) != null) System.out.println("yes it does"); else System.out.println("no it does not"); Now rewrite the code so that it works correctly.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
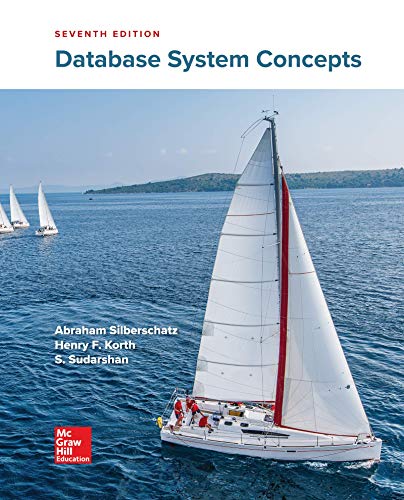
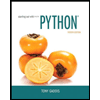
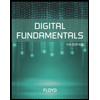
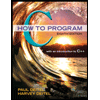
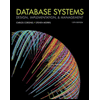
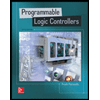