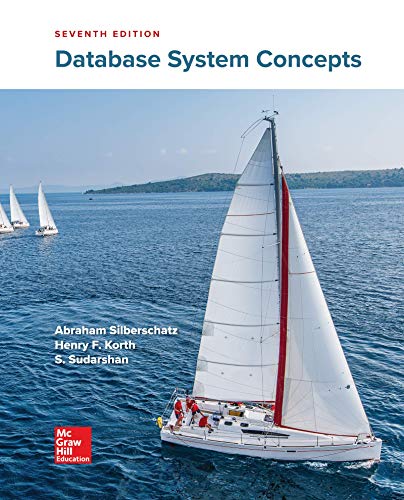
Java Code: Below is Parser.java and there are errors. Look at the errors carefully and please fix those errors and show the fixed code.
Parser.java
import java.text.ParseException;
import java.util.LinkedList;
import java.util.List;
import mypack.Token.TokenType;
public class Parser {
private TokenHandler tokenHandler;
private LinkedList<Token> tokens;
public Parser(LinkedList<Token> tokens) {
this.tokenHandler = new TokenHandler(tokens);
this.tokens = tokens;
}
public boolean AcceptSeparators() {
boolean foundSeparator = false;
while (tokenHandler.MoreTokens()) {
Token currentToken = tokenHandler.getCurrentToken();
if (currentToken.getType() == Token.TokenType.NEWLINE || currentToken.getType() == Token.TokenType.SEMICOLON) {
tokenHandler.consumeMatchedToken();
foundSeparator = true;
} else {
break;
}
}
return foundSeparator;
}
public ProgramNode Parse() throws Exception {
ProgramNode program = new ProgramNode();
while (tokenHandler.MoreTokens()) {
if (!parseFunction(programNode) && !parseAction(programNode)) {
throw new ParseException("Unexpected token: " + tokenHandler.getCurrentToken());
}
}
return programNode;
}
private boolean ParseFunction(ProgramNode programNode) {
Token functionNameToken = tokenHandler.getCurrentToken();
if (tokenHandler.MatchAndRemove(Token.TokenType.IDENTIFIER) != null) {
FunctionDefinitionNode functionNode = new FunctionDefinitionNode(functionNameToken.getType());
programNode.addNode(functionNode);
if (tokenHandler.MatchAndRemove(Token.TokenType.LPAREN) != null) {
if (tokenHandler.MatchAndRemove(Token.TokenType.RPAREN) != null) {
AcceptSeparators();
return true;
}
}
}
return false;
}
private boolean parseAction(ProgramNode programNode) {
Token functionNameToken = tokenHandler.getCurrentToken();
if (tokenHandler.MatchAndRemove(Token.TokenType.IDENTIFIER) != null) {
ActionNode actionNode = new ActionNode(tokenHandler.getLastMatchedToken().getType());
programNode.addNode(actionNode);
AcceptSeparators();
return true;
}
return false;
}
}

There are some mistakes in the sample Java code provided for Parser.java that need to be fixed. It's possible that these mistakes are keeping the code from working properly. In order to guarantee that the code functions as intended, we will thoroughly evaluate the code, identify any errors, and then make a step-by-step repair.
Please refer to the following steps for the complete solution to the problem above.
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

You copied the same code that has errors. Please paste the code on eclipse and take a look at each error. There are red squiggles. I need an error free code for parser.java. Please fix those
You copied the same code that has errors. Please paste the code on eclipse and take a look at each error. There are red squiggles. I need an error free code for parser.java. Please fix those
- Explore the role of generics in conjunction with the Comparable interface. How do generics enhance type safety when sorting collections of objects?arrow_forwardI need a test harness for the following code: LinkedListImpl.java package linkedList; public abstract class LinkedListImpl implements LinkedList { private ListItem firstItem; public LinkedListImpl() { this.firstItem = null; } @Override public Boolean isItemInList(String thisItem) { ListItem currentItem = firstItem; while (currentItem != null) { if (currentItem.data.equals(thisItem)) { return true; } currentItem = currentItem.next; } return false; } @Override public Boolean addItem(String thisItem) { if (isItemInList(thisItem)) { return false; } else { ListItem newItem = new ListItem(thisItem); if (firstItem == null) { firstItem = newItem; } else { ListItem currentItem = firstItem; while (currentItem.next != null) { currentItem = currentItem.next; } currentItem.next = newItem; } return true; } } @Override public Integer itemCount() { Integer count = 0; ListItem currentItem = firstItem; while (currentItem != null) { count++; currentItem = currentItem.next; } return count; }…arrow_forwardPlease help answer this Java multiple choice question. Assume you are a developer working on a class as part of a software package for running artificial neural networks. The network is made of Nodes (objects that implement a Node interface) and Connections (objects that implement a Connection interface). To create a new connection you call the constructor for a concrete class that implements the Connection interface, passing it three parameters: Node origin, Node target, and double weight. The weight is a double that is greater than or equal to 0, and represents how strongly the target node should weigh the input from the origin node. So with a weight of 0.1 the target node will only slightly weight the input from the origin, but with a weight of 725.67 the target node will strongly weight the input. The method throws an IllegalArgumentException. Which of the following are true? A. All of the above are true. B. The code:Connection c = new ConvLayerConnection(origin, target,…arrow_forward
- import java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set<Integer> set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set<Integer> numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set…arrow_forwardJava Code: Create a Parser class. Much like the Lexer, it has a constructor that accepts a LinkedList of Token and creates a TokenManager that is a private member. The next thing that we will build is a helper method – boolean AcceptSeperators(). One thing that is always tricky in parsing languages is that people can put empty lines anywhere they want in their code. Since the parser expects specific tokens in specific places, it happens frequently that we want to say, “there HAS to be a “;” or a new line, but there can be more than one”. That’s what this function does – it accepts any number of separators (newline or semi-colon) and returns true if it finds at least one. Create a Parse method that returns a ProgramNode. While there are more tokens in the TokenManager, it should loop calling two other methods – ParseFunction() and ParseAction(). If neither one is true, it should throw an exception. bool ParseFunction(ProgramNode) bool ParseAction(ProgramNode) -Creates ProgramNode,…arrow_forwardHi, I am a bit lost on how to do this coding assignment. It would be great if someone could help me fill in the MyStack.java file with the given information. All the information is in the pictures. Thank you!arrow_forward
- VariableReferenceNode.java, OperationNode.java, ConstantNode.java, and PatternNode.java must have their own java classes with the correct methods implemented: OperationNode: Has enum, left and Optional right members, good constructors and ToString is good VariableReferenceNode: Has name and Optional index, good constructors and ToString is good Constant & Node Pattern: Have name, good constructor and ToString is good Make sure to include the screenshot of the output of Parser.java as well.arrow_forwardJava Code: Below is Parser.java and there are errors. getType() and getStart() is undefined for the type Optional<Token> and there is an error in addNode(). Make sure to get rid of all the errors in the code. Attached is images of the errors. Parser.java import java.text.ParseException;import java.util.LinkedList;import java.util.List;import java.util.Optional; import javax.swing.ActionMap; public class Parser { private TokenHandler tokenHandler; private LinkedList<Token> tokens; public Parser(LinkedList<Token> tokens) { this.tokenHandler = new TokenHandler(tokens); this.tokens = tokens; } public boolean AcceptSeparators() { boolean foundSeparator = false; while (tokenHandler.MoreTokens()) { Optional<Token> currentToken = tokenHandler.getCurrentToken(); if (currentToken.getType() == Token.TokenType.NEWLINE || currentToken.getType() == Token.TokenType.SEMICOLON) {…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
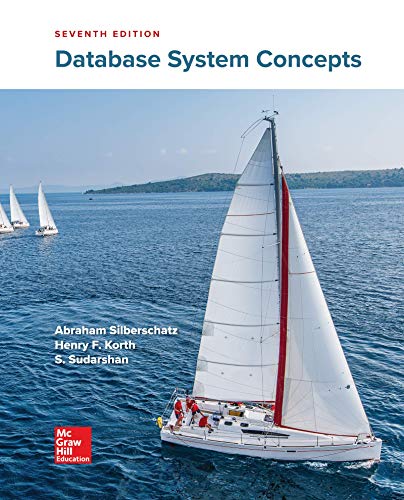
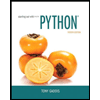
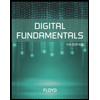
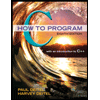
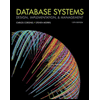
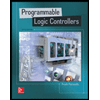