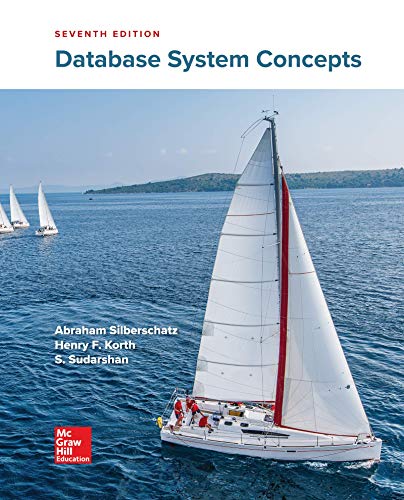
I need to write a header file for my C++ code:
#include <iostream>
using namespace std;
class Fraction
{
private:
int numerator, denominator;
public:
Fraction()
{
numerator = 1;
denominator = 1;
}
Fraction(int n, int d)
{
numerator = n;
if (d==0) {
cout << "ERROR: ATTEMPTING TO DIVIDE BY ZERO" << endl;
exit(0);
}
else
denominator = d;
}
Fraction Sum(Fraction otherFraction)
{
int n = numerator*otherFraction.denominator+otherFraction.numerator*denominator;
int d = denominator*otherFraction.denominator;
return Fraction(n/gcd(n,d),d/gcd(n,d));
}
int gcd(int n, int d)
{
int remainder;
while (d != 0)
{
remainder = n % d;
n = d;
d = remainder;
}
return n;
}
void show()
{
if (denominator == 1)
cout << numerator << endl;
else
cout << numerator << "/" << denominator << endl;;
}
};
int main()
{
Fraction a(1,2);
Fraction b(1,4);
Fraction c;
c = a.Sum(b);
c.show();
return 0;
}

Header files also known as library files is a file with extension .h which contains C++ function declarations and macro definitions.
Uses of header files in C++ programming:
It declares the interfaces which are going to be used in the program.
Each time when such declarations are needed in several different source files, it is a good idea to create a header file for them. It prevents the re-declaration again and again.
It eliminates the labor of finding errors in code in each source file.
We can create a customized header file.Create a file with .h extension. In main include the header file along with system defined header file.
Syntax:
#include "filename.h"
Step by stepSolved in 5 steps with 1 images

- this code is in c++ and i having trouble making It run this is my first time working with classes and header files main.cpp #include <iostream>#include<iomanip>#include <string>#include"Pet.h"#include "Pet.cpp" using namespace std; int main(){Pet* myPets[2];myPets[0] = new Pet("Fluffy", 2);myPets[1] = new Pet("Frisky", 4);for (int day = 1; day <= 9; day++){cout << "======= Day " << day << endl;for (int k = 0; k < 2; k++) {careFor(myPets[k], day);}}cout << "=======" << endl;for (int k = 0; k < 2; k++){if (myPets[k]->alive()) {cout << "Animal Control has come to rescue "<< myPets[k]->name() << endl;}delete myPets[k];}return 0;} pet.cpp #include<iostream>#include "Pet.h" Pet::Pet(std::string nm, int initialHealth){m_name = nm;m_health = initialHealth;} void Pet::eat(int amt){if (amt < 0)return;m_health += amt;// Increase the pets health by the amount} void Pet::play(){m_health--;// Decrease pets…arrow_forwardC++ Define an "Expression" class that manages expression info: operand1 (integer), operand2 (integer), and the expression operator (char). It must provide at least the following method:- toString() to return a string containing all the expression info such as 50 + 50 = 100 Write a command-driven program named "ListExpressions" that will accept the following commands:add, listall, listbyoperator, listsummary, exit "add" command will read the expression and save it away in the list "listall" command will display all the expressions "listbyoperator" command will read in the operator and display only expressions with that given operator "listsummary" command will display the total number of expressions, the number of expressions for each operator, the largest and smallest expression values "exit" command will exit the program Requirements:- It must be using the array of pointers (not vector) to Expression objects to manage the list of Expression…arrow_forwardHello, I was making a hangman simulation in C++. The code runs, but not fully. Could you identify the error and fix it? #include<iostream>#include<cstring> // for string class functions#include<fstream>#include <cctype>using namespace std; int main(){// define variable to get the response from user "Yes" or "No"string response;// Define index variableint w = 0;// define number of words that need to be guessed by the user assume 4const int WORDS = 4;// loopdo{// we will define the hangman bodyconst char body[] = "o/|\\|\\";// here we define the wordsstring words[WORDS] = {"MACAW", "SADDLE", "TOASTER", "XENOICIDE"};// fetch size or lengthstring xword(words[w].length(),'*');// define iterator to fetch the wordsstring::iterator i, ix = xword.begin();// define number of words to prompt for the userchar letters[26]={"\0"};// Now we define the variables which will be used in the simulationint n =0, xcount = xword.length();bool found = false, solved = false, hung =…arrow_forward
- #include <iostream> #include <string> #include <cstdlib> using namespace std; template<class T> class A { public: T func(T a, T b){ return a/b; } }; int main(int argc, char const *argv[]) { A <float>a1; cout<<a1.func(3,2)<<endl; cout<<a1.func(3.0,2.0)<<endl; return 0; } Give output for this code.arrow_forward#include <iostream>using namespace std; struct Person{ int weight ; int height;}; int main(){ Person fred; Person* ptr = &fred; fred.weight=190; fred.height=70; return 0;} What is a correct way to subtract 5 from fred's weight using the pointer variable 'ptr'?arrow_forward#include <iostream> #include <iomanip> #include <string> #include <vector> using namespace std; class Movie { private: string title = ""; int year = 0; public: void set_title(string title_param); string get_title() const; // "const" safeguards class variable changes within function string get_title_upper() const; void set_year(int year_param); int get_year() const; }; // NOTICE: Class declaration ends with semicolon! void Movie::set_title(string title_param) { title = title_param; } string Movie::get_title() const { return title; } string Movie::get_title_upper() const { string title_upper; for (char c : title) { title_upper.push_back(toupper(c)); } return title_upper; } void Movie::set_year(int year_param) { year = year_param; } int Movie::get_year() const { return year; } int main() { cout << "The Movie List program\n\n"…arrow_forward
- Please answer in C++ / Text book question / C++ for scientist and Engineers 3rd edition Construct a class named Fractions containing two integer data members named num and denom, used to store the numerator and denominator of a fraction having the form num/denom. Your class should include a default constructor that initializes num and denom to 1 and four operator functions for adding, subtracting, multiplying, and dividing the two fractions, as follows: Addition: a/b + c/d = (a * d + b * c) / (b * d)Subtraction: a/b - c/d = (a * d - b * c) / (b * d)Multiplication: a/b * c/d = (a* c) / (b * d)Division: (a/b) / (c/d) = (a * d) / (b * c) Finally, your class should have a member function that reduces each fraction to its terms (refer to Exercise 15 in Programming Projects for Chapter 6 for how to do this) as well as input and output functions for entering and displaying a fraction. thanksarrow_forwardIn C++ language only !!arrow_forwardshould write in c language (not c++ or not c#)arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
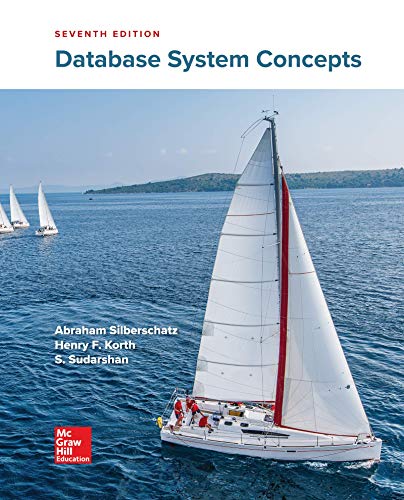
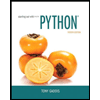
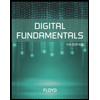
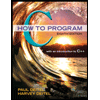
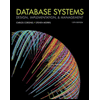
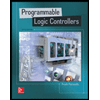