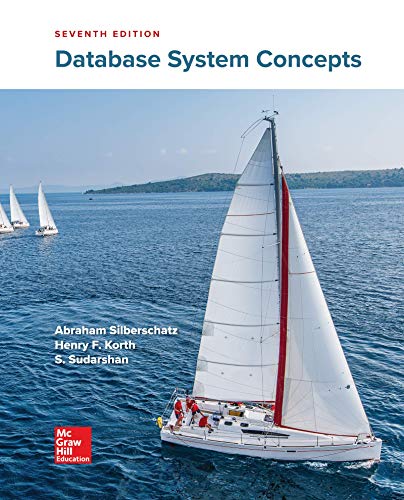
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Implement a Single linked list to store a set of Integer numbers (no duplicate)
• Instance variable
• Constructor
• Accessor and Update methods
1. Define a Node Class.
a. Instance Variables
# E element- (generics framework)
# Node Next (pointer) - refer to the next node (Self-referential)
b. Constructor
c. Methods
# E getElement() //Return the value of this node.
# setElement(E e) // Set value to this node.
# Node getNext() //Return the pointer of this node.
# setNext(Node n) //Set pointer to this node.
# displayNode() //Display information of this node.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Tree Define a class called TreeNode containing three data fields: element, left and right. The element is a generic type. Create constructors, setters and getters as appropriate. Define a class called BinaryTree containing two data fields: root and numberElement. Create constructors, setters and getters as appropriate. Define a method balanceCheck to check if a tree is balanced. A tree being balanced means that the balance factor is -1, 0, or 1.arrow_forwardimport java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set<Integer> set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set<Integer> numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set…arrow_forward1. Complete the function evaluate_postfix(String exp): Input: "10 23 - 5 15 + +" Retur: 7 Node.java public class Node {Object info;Node next;Node(Object info, Node next){this.info=info;this.next=next;}}// "((1+2)"// ['(','(','1','+','2',')']// stack : ')' -> '2' -> '1' -> '(' -> '(' -> null Stack.java public class Stack {private Node top;public Stack() {top = null;}public boolean isEmpty() {return (top == null);}public void push(Object newItem) {top = new Node(newItem, top);}public Object pop() {if (isEmpty()) {System.out.println("Trying to pop when stack is empty");return null;} else {Node temp = top;top = top.next;return temp.info;}}void popAll() {top = null;}public Object peek() {if (isEmpty()) {System.out.println("Trying to peek when stack is empty");return null;} else {return top.info;}}} Runner.java public class Runner {public static void main(String[] args) {String expression1 = "((1+2)))))+(2+2))(1/2)";boolean res =…arrow_forward
- /** * This class will use Nodes to form a linked list. It implements the LIFO * (Last In First Out) methodology to reverse the input string. * **/ public class LLStack { private Node head; // Constructor with no parameters for outer class public LLStack( ) { // to do } // This is an inner class specifically utilized for LLStack class, // thus no setter or getters are needed private class Node { private Object data; private Node next; // Constructor with no parameters for inner class public Node(){ // to do // to do } // Parametrized constructor for inner class public Node (Object newData, Node nextLink) { // to do: Data part of Node is an Object // to do: Link to next node is a type Node } } // Adds a node as the first node element at the start of the list with the specified…arrow_forwardImplement a Single linked list to store a set of Integer numbers (no duplicate) • Instance variable• Constructor• Accessor and Update methods 2.) Define SLinkedList Classa. Instance Variables: # Node head # Node tail # int sizeb. Constructorc. Methods # int getSize() //Return the number of nodes of the list. # boolean isEmpty() //Return true if the list is empty, and false otherwise. # int getFirst() //Return the value of the first node of the list. # int getLast()/ /Return the value of the Last node of the list. # Node getHead()/ /Return the head # setHead(Node h)//Set the head # Node getTail()/ /Return the tail # setTail(Node t)//Set the tail # addFirst(E e) //add a new element to the front of the list # addLast(E e) // add new element to the end of the list # E removeFirst()//Return the value of the first node of the list # display()/ /print out values of all the nodes of the list # Node search(E key)//check if a given…arrow_forward1. Write code to define LinkedQueue<T> and CircularArrayQueue<T> classes which willimplement QueueADT<T> interface. Note that LinkedQueue<T> and CircularArrayQueue<T>will use linked structure and circular array structure, respectively, to define the followingmethods.(i) public void enqueue(T element); //Add an element at the rear of a queue(ii) public T dequeue(); //Remove and return the front element of the queue(iii) public T first(); //return the front element of the queue without removing it(iv) public boolean isEmpty(); //Return True if the queue is empty(v) public int size(); //Return the number of elements in the queue(vi) public String toString();//Print all the elements of the queuea. Create an object of LinkedQueue<String> class and then add some names of your friendsin the queue and perform some dequeue operations. At the end, check the queue sizeand print the entire queue.b. Create an object from CircularArrayQueue<T> class and…arrow_forward
- 7. Given a Queue Q, write a non-generic method that finds the maximum element in the Queue. You may use only queue operations. No other data structure can be used other than queues. The Queue must remain intact after finding the maximum value. Assume the class that has the findMax method implements Comparable. The header of the findMax method: public Comparable findMax(Queue q)arrow_forwardIn Java. The following is a class definition of a linked list Node:class Node{int info;Node next;}Show the instructions required to create a linked list that is referenced by head and stores in order, the int values 13, 6 and 2. Assume that Node's constructor receives no parameters.arrow_forwardImplement a Single linked list to store a set of Integer numbers (no duplicate) • Instance variable• Constructor• Accessor and Update methods 3. Define TestSLinkedList Classa. Declare an instance of the Single List class.b. Test all the methods of the Single List class.arrow_forward
- 1. Goals and Overview The goal of this assignment is to practice creating a hash table. This assignment also has an alternative, described in Section 5. A hash table stores values into a table (meaning an array) at a position determined by a hash function. 2. Hash Function and Values Every Java object has a method int hashCode() which returns an int suitable for use in a hash table. Most classes use the hashCode method they inherit from object, but classes such as string define their own hashCode method, overriding the one in object. For this assignments, your hash table stores values of type public class HashKeyValue { K key; V value; } 3. Hash Table using Chained Hashing Create a hash table class (called HashTable211.java) satisfying the following interface: public interface HashInterface { void add(K key, V value); // always succeeds as long as there is enough memory V find (K key); // returns null if there is no such key } Internally, your hash table is defined as a private…arrow_forwardAdd the following method in the BST class that returns aniterator for traversing the elements in a BST in preorder./** Return an iterator for traversing the elements in preorder */java.util.Iterator<E> preorderIterator()arrow_forwardpackage circularlinkedlist;import java.util.Iterator; public class CircularLinkedList<E> implements Iterable<E> { // Your variablesNode<E> head;Node<E> tail;int size; // BE SURE TO KEEP TRACK OF THE SIZE // implement this constructorpublic CircularLinkedList() {} // I highly recommend using this helper method// Return Node<E> found at the specified index// be sure to handle out of bounds casesprivate Node<E> getNode(int index ) { return null;} // attach a node to the end of the listpublic boolean add(E item) {this.add(size,item);return false; } // Cases to handle// out of bounds// adding to empty list// adding to front// adding to "end"// adding anywhere else// REMEMBER TO INCREMENT THE SIZEpublic void add(int index, E item){ } // remove must handle the following cases// out of bounds// removing the only thing in the list// removing the first thing in the list (need to adjust the last thing in the list to point to the beginning)// removing the last…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
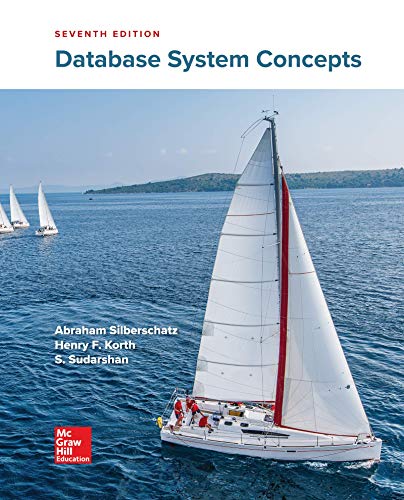
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
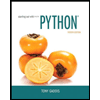
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
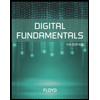
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
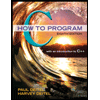
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
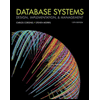
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
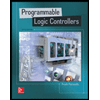
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education