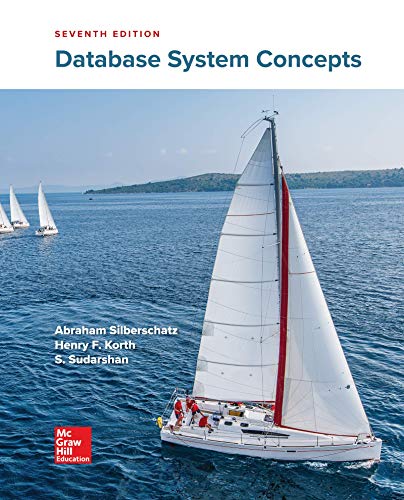
Complete the implementations of
- the abstract class LinkedList.java, which implements the List.java interface and
- the class LinkedOrderedList.java, which extends the abstract class LinkedList.java and implements theOrderedList.java interface.
LinkedList methods to implement:
- removeFirst
- removeLast
- remove
- contains
LinkedOrderedList method to implement:
- add
Recommended order of development:
- add
- to empty list
- to end of list
- to front of list
- to middle of list
- contains
- removeFirst
- removeLast
- remove
Write a test class that tests all of the methods you implemented. Note: You only need to test LinkedOrderedList, because all of the methods are implemented in that class or inherited from LinkedList.
Although you are not required to submit the test class, you might be asked to do so if you are having difficulties getting your implementation to work.
Submit the completed LinkedList.java and LinkedOrderedList.java.
LinkedList.java code
import java.util.NoSuchElementException;
import java.util.StringJoiner;
public abstract class LinkedList<E> implements List<E> {
protected class Node {
E element;
Node next;
Node(E element) {
this(element, null);
}
Node(E element, Node next) {
this.element = element;
this.next = next;
}
}
protected int count;
protected Node head;
protected Node tail;
/**
* Creates an empty list.
*/
public LinkedList() {
count = 0;
head = null;
tail = null;
}
/**
* Removes and returns the first element from the list.
*
* @return the first element of the list
* @throws IllegalStateException if the list is empty
*/
@Override
public E removeFirst() {
if (isEmpty()) {
throw new IllegalStateException("The LinkedList is empty.");
}
return null;
}
/**
* Removes and returns the last element from the list.
*
* @return the last element in the list
* @throws IllegalStateException if the list is empty
*/
@Override
public E removeLast() {
if (isEmpty()) {
throw new IllegalStateException("The LinkedList is empty.");
}
return null;
}
/**
* Removes and returns the specified element from the list.
*
* @param targetElement the element to be removed from the list
* @return the removed element
* @throws IllegalStateException if the list is empty
* @throws NoSuchElementException if the target element is not found
*/
@Override
public E remove(E targetElement) {
if (isEmpty()) {
throw new IllegalStateException("The LinkedList is empty.");
}
return null;
}
/**
* Returns the first element in the list without removing it.
*
* @return the first element in the list
* @throws IllegalStateException if the list is empty
*/
@Override
public E first() {
if (isEmpty()) {
throw new IllegalStateException("The LinkedList is empty.");
}
return head.element;
}
/**
* Returns the last element in the list without removing it.
*
* @return the last element in the list
* @throws IllegalStateException if the list is empty
*/
@Override
public E last() {
if (isEmpty()) {
throw new IllegalStateException("The LinkedList is empty.");
}
return tail.element;
}
/**
* Returns true if the specified element is found in the list.
*
* @param targetElement the element that is sought in the list
* @return true if the element is found in the list
* @throws IllegalStateException if the list is empty
*/
@Override
public boolean contains(E targetElement) {
if (isEmpty()) {
throw new IllegalStateException("The LinkedList is empty.");
}
return false;
}
/**
* Returns true if the list contains no elements.
*
* @return true if the list contains no elements
*/
@Override
public boolean isEmpty() {
return (count == 0);
}
/**
* Returns the number of elements in the list.
*
* @return the number of elements in the list
*/
@Override
public int size() {
return count;
}
/**
* Returns a string representation of the list.
*
* @return a string representation of the list
*/
@Override
public String toString() {
StringJoiner result = new StringJoiner(", ", "[", "]");
Node current = head;
while (current != null) {
result.add(current.element.toString());
current = current.next;
}
return result.toString();
}
}
Thank you for any help/suggestions!
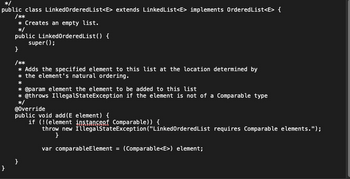
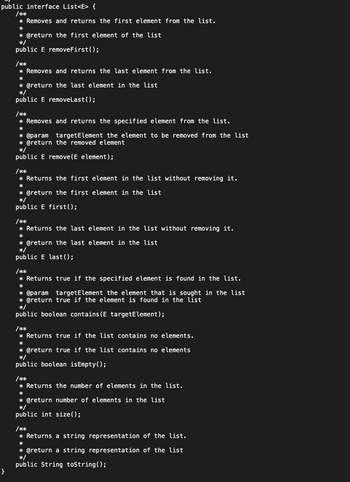

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps

- Implement a Single linked list to store a set of Integer numbers (no duplicate), Using Java Language.• Instance variable• Constructor• Accessor and Update methods 1. Define a Node Class.a. Instance Variables # E element- (generics framework) # Node Next (pointer) - refer to the next node (Self-referential)b. Constructorc. Methods # E getElement() //Return the value of this node. # setElement(E e) // Set value to this node. # Node getNext() //Return the pointer of this node. # setNext(Node n) //Set pointer to this node. # displayNode() //Display information of this node.arrow_forwardI need help with getting started for this in Java. Codes listed below. Complete the CircularArrayQueue.java class implementation of the Queue.java interface so that it does not use a count field. This implementation must have only the following fields: DEFAULT_CAPACITY front rear queue Implement the methods enter leave front isEmpty isFull expandCapacity Write a test class to test each of the methods you implement. Queue.java public interface Queue<E> { /** * The element enters the queue at the rear. */ public void enter(E element); /** * The front element leaves the queue and is returned. * @throws java.util.NoSuchElementException if queue is empty. */ public E leave(); /** * Returns True if the queue is empty. */ public boolean isEmpty(); /** * Returns the front element without removing it. * @throws java.util.NoSuchElementException if queue is empty. */ public E front();}…arrow_forwardCode In Java Using Array or doubly Linklist instead of linklist COVID-19 has effect whole world. People are interested in knowing current situation of COVID-19. You are required to write JAVA program for storing information in a Array Following JAVA classes are provided. // Node class public class COVIDDetail { public String country; public int activecases; public COVIDDetail next; } // linked List class public class COVIDList { COVIDDetail start=null; } You are required to add following methods to COVIDList class void add(COVIDDetail n) this method receives a node as argument and adds it to given list. Nodes are arranged in descending order with respect to number of cases. Note that you are not required to sort the list in fact while adding first find its appropriate position and then add given node to that position. void updateCases(String country_name , int cases) this method receives two argument country name and cases (can have…arrow_forward
- add public method size() to the class Stack so the output will be pop is: 4 peek is: 8 size is: 2 false public class Stack<T> { private LinkedList<T> list; public Stack() { list = new LinkedList<>(); } public boolean isEmpty() { return list.isEmpty(); } public void push(T data) { list.prepend(data); } public T pop() { if (isEmpty()) { throw new EmptyStackException(); } return list.removeHead(); } public T peek() { if (isEmpty()) { throw new EmptyStackException(); } return list.getHead(); } } public class Main { public static void main(String[] args) { Stack<Integer> stack = new Stack<>(); stack.push(6); stack.push(8); stack.push(4); System.out.println("pop is: " + stack.pop()); System.out.println("peek is: " + stack.peek()); System.out.println("size is: " +…arrow_forwardFor the Queue use the java interface Queue with the ArrayDeque classFor the Stack use the java Deque interface with the ArrayDeque classFor the LinkedList, use the java LinkedList class Also, when you are asked to create and use a Queue, you can use only those methods pertaining to the general operations of a queue (Enqueue: addLast, Dequeue: removeFirst, and peek() Similarly, when you are asked to create and/or use a stack, you can use only those methods pertaining to the general operations of a Stack (push: addFirst, pop:removeFirst, and peek() ) Your code should not only do the job, but also it should be done as efficiently as possible: fast and uses no additional memory if at all possible Questions Write a method “int GetSecondMax(int[] array)” . this method takes an array of integers and returns the second max value Example: if the array contains: 7, 2, 9, 5, 4, 15, 6, 1}. It should return the value: 9arrow_forwardimport java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set<Integer> set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set<Integer> numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set…arrow_forward
- True or False? When implementing a queue with a linked list, the front of the queue is also the front of the linked list.arrow_forwardModify the Java class for the abstract stack type shown belowto use a linked list representation and test it with the same code thatappears in this chapter. class StackClass {private int [] stackRef;private int maxLen,topIndex;public StackClass() { // A constructorstackRef = new int [100];maxLen = 99;topIndex = -1;}public void push(int number) {if (topIndex == maxLen)System.out.println("Error in push–stack is full");else stackRef[++topIndex] = number;}public void pop() {if (empty())System.out.println("Error in pop–stack is empty"); else --topIndex;}public int top() {if (empty()) {System.out.println("Error in top–stack is empty");return 9999;}elsereturn (stackRef[topIndex]);}public boolean empty() {return (topIndex == -1);}}An example class that uses StackClass follows:public class TstStack {public static void main(String[] args) {StackClass myStack = new StackClass();myStack.push(42);myStack.push(29);System.out.println("29 is: " + myStack.top());myStack.pop();System.out.println("42 is:…arrow_forwardWrite a Java program for a matrix class that can add and multiply arbitrary two dimensional arrays of integers. Textbook Project P-3.36, pp. 147 Implement Singly Linked List - use textbook Chapter 3.2 as an exaple. Write a main driver to test basic list implementations. Textbook reference : Data structures and algorithms in Java Micheal Goodricharrow_forward
- Implement a Single linked list to store a set of Integer numbers (no duplicate) • Instance variable• Constructor• Accessor and Update methods 2.) Define SLinkedList Classa. Instance Variables: # Node head # Node tail # int sizeb. Constructorc. Methods # int getSize() //Return the number of nodes of the list. # boolean isEmpty() //Return true if the list is empty, and false otherwise. # int getFirst() //Return the value of the first node of the list. # int getLast()/ /Return the value of the Last node of the list. # Node getHead()/ /Return the head # setHead(Node h)//Set the head # Node getTail()/ /Return the tail # setTail(Node t)//Set the tail # addFirst(E e) //add a new element to the front of the list # addLast(E e) // add new element to the end of the list # E removeFirst()//Return the value of the first node of the list # display()/ /print out values of all the nodes of the list # Node search(E key)//check if a given…arrow_forwardUsing Java, modify the doubly linked list class presented below so it works with generic types. Add the following methods drawn from the java.util.List interface:• void clear(): remove all elements from the list.• E get(int index): return the element at position index in the list.• E set(int index, E element): replace the element at the specified position with the specified element and return the previous element. Test your generic linked list class by processing a list of numbers of type double. ====================================/**The DLinkedList class implements a doubly Linked list. */class DLinkedList {/**The Node class stores a list element and a reference to the next node.*/private class Node{String value; // Value of a list elementNode next; // Next node in the listNode prev; // Previous element in the list/**Constructor. @param val The element to be stored in the node.@param n The reference to the successor node.@param p The reference to the predecessor node.*/ Node(String…arrow_forwardAssume class MyStack implements the following StackGen interface. For this question, make no assumptions about the implementation of MyStack except that the following interface methods are implemented and work as documented. Write a public instance method for MyStack, called interchange(T element) to replace the bottom "two" items in the stack with element. If there are fewer than two items on the stack, upon return the stack should contain exactly two items that are element.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
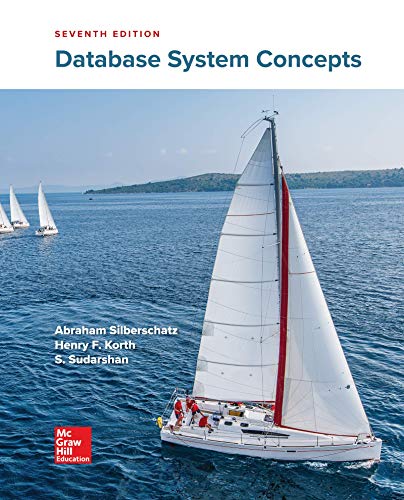
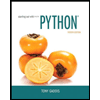
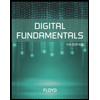
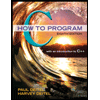
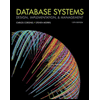
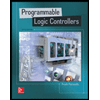