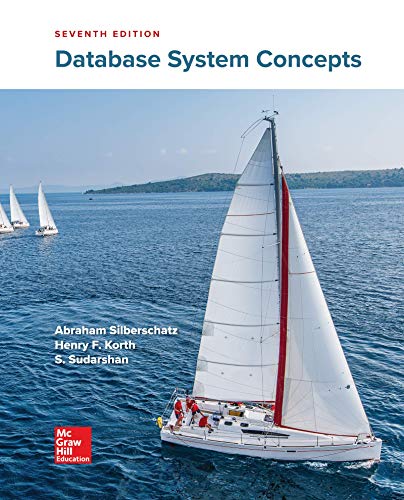
Concept explainers
please build up the java code
complete the code and fill up missing codes
// = needs input for the code
thank you
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import java.util.ArrayList;
public class GUI extends JFrame implements ActionListener {
JMenuItem resetItem, startItem;
JTextField ansTextField;
JLabel resultLabel, scoreLabel, timeLabel, operationLabel;
Timer timer;
DoubleDigitOp op;
final int maxTime = 6;
final int runTime = 100;
double score = 0;
int newTime = maxTime*runTime;
String[] status = new String[]{"Score: ","Timer: "};
String[] reset = new String[]{"Score: 0.00","Timer: "+((double)newTime/100),
"00 + 00 = ","Game Message"};
public GUI(String title){
setTitle(title);
//Create a Menu names "Game" (1)
//Add two menus on "Game": the menus named "Start" and "Reset" (6) -- both execute an action
JMenuBar menuBar = new JMenuBar();
menuBar.add(gameMenu);
setJMenuBar(menuBar);
JPanel content = new JPanel();
content.setLayout(new BorderLayout());
JPanel statusPanel = new JPanel();
statusPanel.setLayout(new FlowLayout());
scoreLabel = new JLabel(reset[0]); //contains the current score
statusPanel.add(scoreLabel);
timeLabel = new JLabel(reset[1]); //contains the remaining time
statusPanel.add(timeLabel);
content.add(statusPanel,BorderLayout.NORTH);
JPanel opsPanel = new JPanel();
opsPanel.setLayout(new FlowLayout());
operationLabel = new JLabel(reset[2]); //contains the operation, e.g. x + y
opsPanel.add(operationLabel);
ansTextField = new JTextField(10); //a text field where thew user orplayer enter the answer
ansTextField.addActionListener(this);
ansTextField.requestFocus();
ansTextField.setBackground(Color.LIGHT_GRAY);
opsPanel.add(ansTextField);
content.add(opsPanel,BorderLayout.CENTER);
//the resulLabel contains the current situation in the game
resultLabel = new JLabel(reset[3],SwingConstants.CENTER);
content.add(resultLabel,BorderLayout.SOUTH);
setContentPane(content);
pack();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocation(30,10);
setVisible(true);
}
public static void main(String[] args){
//write the appropriate command here (1)
}
public void actionPerformed(ActionEvent e){
if(){ //chooses to reset the game, write the correct condition (1)
//resets the answer, score, timer, operations, and result (1)
} else if(){ //chooses to start the game, write the correct condition (1)
start();
} else if(e.getSource()==ansTextField){ //when the user presses enter on the answer text field
if(){ //this is where the answer is correct, write the correct condition (1)
//print "Correct" on the result (1)
score += (double)newTime/runTime;
scoreLabel.setText(status[0]+score);
start();
} else {
resetMany(1,5);
//print "Incorrect" on the result (1)
}
} else if(e.getSource()==timer){
newTime--;
timeLabel.setText(status[1]+((double)newTime/runTime));
if(newTime==0){
resetMany(1,5);
resultLabel.setText("Time's Up!");
}
} else;
}
public void resetMany(int ... choices){
for(int choice: choices){
resetOne(choice);
}
}
public void resetOne(int choice){
if(choice == 4){
ansTextField.setText("");
} else if(choice == 0){
scoreLabel.setText(reset[0]);
score = 0;
} else if(choice == 1){
timeLabel.setText(reset[1]);
newTime = maxTime*runTime;
} else if(choice == 2){
operationLabel.setText(reset[2]);
} else if(choice == 3){
resultLabel.setText(reset[3]);
} else {
if(timer!=null){
timer.stop();
}
}
}
public void resetAll(){
ansTextField.setText("");
scoreLabel.setText(reset[0]);
score = 0;
timeLabel.setText(reset[1]);
newTime = maxTime*runTime;
operationLabel.setText(reset[2]);
resultLabel.setText(reset[3]);
if(timer!=null){
timer.stop();
}
}
public void start(){
//reset time and answer textfield (1)
//print the operation to the operationLLabel (1) -- e.g. x + y =
timer = new Timer(10,this);
timer.start();
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Chapter 18 Problem 38.PE Textbook: Introduction to Java programming and datastructure. 11th Edition Y. Daniel Liang Publisher: PEARSON ISBN: 9780134670942 This code is taken from your website does not work. package e38; import javafx.application.Application;import javafx.event.ActionEvent;import javafx.event.EventHandler;import javafx.scene.Scene;import javafx.scene.control.Button; import javafx.stage.Stage;import javafx.geometry.Pos;import javafx.scene.control.Label;import javafx.scene.control.TextField;import javafx.scene.control.Textfield;import javafx.scene.layout.BorderPane;import javafx.scene.layout.HBox;import javafx.scene.layout.Pane;import javafx.scene.shape.Line;import javafx.stage.Stage; public class E38 extends Application { @Override public void start(Stage primaryStage) { // Create a pane TreePane tp = new TreePane(); // Create a textfield TextField tfOrder = new TextField(); // Set the Depth…arrow_forwardYou have to use comment function to describe what each line does import java.io.BufferedReader; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.IOException; import java.util.ArrayList; import java.util.Arrays; import java.util.List; public class PreferenceData { private final List<Student> students; private final List<Project> projects; private int[][] preferences; private static enum ReadState { STUDENT_MODE, PROJECT_MODE, PREFERENCE_MODE, UNKNOWN; }; public PreferenceData() { super(); this.students = new ArrayList<Student>(); this.projects = new ArrayList<Project>(); } public void addStudent(Student s) { this.students.add(s); } public void addStudent(String s) { this.addStudent(Student.createStudent(s)); } public void addProject(Project p) { this.projects.add(p); } public void addProject(String p) { this.addProject(Project.createProject(p)); } public void createPreferenceMatrix() { this.preferences = new…arrow_forwardJava Given main(), complete the SongNode class to include the printSongInfo() method. Then write the Playlist class' printPlaylist() method to print all songs in the playlist. DO NOT print the dummy head node.arrow_forward
- Please write the code in Java usign this UML diagram. Add Comments.arrow_forwardIn Java intellej Correct this code and add the following 1.Correct: (The error shows a problem under () next to "firstBeachShoot.printPhotoDetails") 2.Add the following to the second package (screenshot): 1.for or for...each or while loops. 2.Arrays 3.default, no-args and parameterized constructors. Here's the first package : import java.util.Scanner;public class Image {int numberOfPhotos; // photos on rolldouble fStop; // light let it 1.4,2.0,2.8 ... 16.0int iso; // sensativity to light 100,200, 600int filterNumber; // 1-6String subjectMatter;String color; // black and white or colorString location;boolean isblurry;public String looksBlurry(boolean key) {if (key == true) {return "Photo is Blurry";} else {return "Photo is Clear";}}public void printPhotoDetails(String s1) {Scanner br = new Scanner(System.in);String subjectMatter = s1;System.out.println("Data of Nature photos:");System.out.println("Enter number of photos:");numberOfPhotos = br.nextInt();int i = 1;while…arrow_forwardPlease help me fix the errors in the java program below. There are two class AnimatedBall and BouncingBall import javax.swing.*;import java.awt.*;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;public class AnimatedBall extends JFrame implements ActionListener{private Button btnBounce; // declare a button to playprivate TextField txtSpeed; // declare a text field to enterprivate Label lblDelay;private Panel controls; // generic panel for controlsprivate BouncingBall display; // drawing panel for ballContainer frame;public AnimatedBall (){// Set up the controls on the appletframe = getContentPane();btnBounce = new Button ("Bounce Ball");//create the objectstxtSpeed = new TextField("10000", 10);lblDelay = new Label("Enter Delay");display = new BouncingBall();//create the panel objectscontrols = new Panel();setLayout(new BorderLayout()); // set the frame layoutcontrols.add (btnBounce); // add controls to panelcontrols.add (lblDelay);controls.add…arrow_forward
- Can you please help me with this, its in java. Thank you. Write classes in an inheritance hierarchy Implement a polymorphic method Create an ArrayList object Use ArrayList methods For this, please design and write a Java program to keep track of various menu items. Your program will store, display and modify salads, sandwiches and frozen yogurts. Present the user with the following menu options: Actions: 1) Add a salad2) Add a sandwich3) Add a frozen yogurt4) Display an item5) Display all items6) Add a topping to an item9) QuitIf the user does not enter one of these options, then display:Sorry, <NUMBER> is not a valid option.Where <NUMBER> is the user's input. All menu items have the following: Name (String) Price (double) Topping (StringBuilder or StringBuffer of comma separated values) In addition to everything that a menu item has, a main dish (salad or sandwich) also has: Side (String) A salad also has: Dressing (String) A sandwich also has: Bread type…arrow_forwardPlease help me with this using java. A starters code is provided (I created a starters code). For more info refer to the image provided. Please ensure tge code works Starter code:import javax.swing.*; import java.applet.*; import java.awt.event.*; import java.awt.*;public class halloween extends Applet implements ActionListener{ int hallow[] [] = ? int rows = 4; int cols = 3; JButton pics[] = new JButton [rows * cols]; public void init () { Panel grid = new Panel (new GridLayout (rows, cols)); int m = 0; for (int i = 0 ; i < rows ; i++) { for (int j = 0 ; j < cols ; j++) { pics [m] = new JButton (createImageIcon ("back.png")); pics [m].addActionListener (this); pics [m].setActionCommand (m + ""); pics [m].setPreferredSize (new Dimension (128, 128)); grid.add (pics [m]); } }m++; }}add (grid); }public void actionPerformed (ActionEvent…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
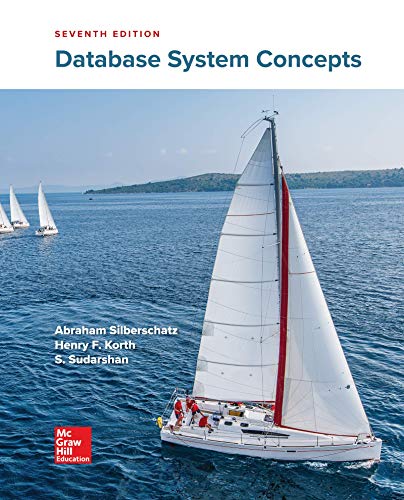
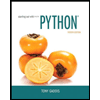
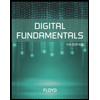
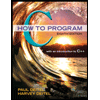
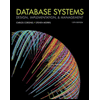
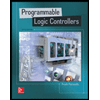