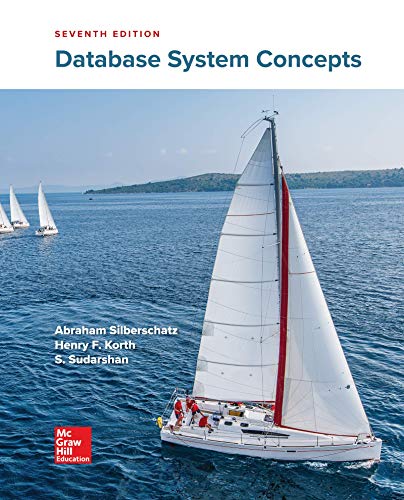
import java.awt.*;
import javax.swing.*;
import java.util.*;
// Model class
class FrogModel {
private int xc;
private int yc;
public FrogModel() {
// Initialize frog's coordinates randomly
Random rng = new Random(); // Creating an instance of Random
xc = rng.nextInt(200);
yc = rng.nextInt(200);
}
public int getX() {
return xc;
}
public int getY() {
return yc;
}
public void leap() {
// Update frog's coordinates randomly
Random rng = new Random(); // Creating an instance of Random
xc = rng.nextInt(200);
yc = rng.nextInt(200);
}
}
// View class
class FrogView extends JPanel {
private FrogModel model;
public FrogView(FrogModel model) {
this.model = model;
setBackground(Color.BLUE);
}
@Override
protected void paintComponent(Graphics gc) {
super.paintComponent(gc);
gc.setColor(Color.GREEN);
gc.fillOval(model.getX(), model.getY(), 10, 10); // Adjust size as needed
}
}
// Controller class
class FrogController {
private FrogModel model;
private FrogView view;
public FrogController(FrogModel model, FrogView view) {
this.model = model;
this.view = view;
}
public void leap() {
model.leap();
view.repaint();
}
}
// Main class
public class FroggerMVC {
public static void main(String[] args) {
FrogModel model = new FrogModel();
FrogView view = new FrogView(model);
FrogController controller = new FrogController(model, view);
// Set up GUI
JFrame frame = new JFrame("Frogger");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().add(view);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
// Leap button setup
JButton leapButton = new JButton("Leap");
leapButton.addActionListener(e -> controller.leap());
frame.getContentPane().add(leapButton, BorderLayout.SOUTH);
}
}
i'm trying to run the code in replit but the code is not running. can you check from your side if this code correct and what i do to run this code.

Step by stepSolved in 1 steps

- import bridges.base.ColorGrid;import bridges.base.Color;public class Scene {/* Creates a Scene with a maximum capacity of Marks andwith a background color.maxMarks: the maximum capacity of MarksbackgroundColor: the background color of this Scene*/public Scene(int maxMarks, Color backgroundColor) {}// returns true if the Scene has no room for additional Marksprivate boolean isFull() {return false;}/* Adds a Mark to this Scene. When drawn, the Markwill appear on top of the background and previously added Marksm: the Mark to add*/public void addMark(Mark m) {if (isFull()) throw new IllegalStateException("No room to add more Marks");}/*Helper method: deletes the Mark at an index.If no Marks have been previously deleted, the methoddeletes the ith Mark that was added (0 based).i: the index*/protected void deleteMark(int i) {}/*Deletes all Marks from this Scene thathave a given Colorc: the Color*/public void deleteMarksByColor(Color c) {}/* draws the Marks in this Scene over a background…arrow_forwardHow do I remove the space at the end of the result? Code: import java.util.Scanner; public class FinalExamAnswers{ public static void main(String [] args) { manipulateString(); //calls function } //your code here public static void manipulateString() { Scanner sc=new Scanner(System.in); //create Scanner instance System.out.println("Enter a sentence"); String sentence=sc.nextLine(); //input a sentence String[] words=sentence.split(" "); //split the sentence at space and store it in array for(int i=0;i<words.length;i++) //i from 0 to last index { if(i%2==0) //if even index words[i]=words[i].toUpperCase(); //converted to upper case else //if odd index words[i]=words[i].toLowerCase(); //converted to lower case } for(int i=words.length-1;i>=0;i--) //i from last index to 0 {…arrow_forwardImplement move the following way. public int[] walk(int... stepCounts) { // Implement the logic for walking the players returnnewint[0]; } public class WalkingBoardWithPlayers extends WalkingBoard{ privatePlayer[] players; privateintround; publicstaticfinalintSCORE_EACH_STEP=13; publicWalkingBoardWithPlayers(int[][] board, intplayerCount) { super(board); initPlayers(playerCount); } publicWalkingBoardWithPlayers(intsize, intplayerCount) { super(size); initPlayers(playerCount); } privatevoidinitPlayers(intplayerCount) { if(playerCount <2){ thrownewIllegalArgumentException("Player count must be at least 2"); } else { this.players=newPlayer[playerCount]; this.players[0] =newMadlyRotatingBuccaneer(); for (inti=1; i < playerCount; i++) { this.players[i] =newPlayer(); } } } package walking.game.player; import walking.game.util.Direction; public class Player{ privateintscore; protectedDirectiondirection=Direction.UP; publicPlayer() {} publicintgetScore() { return score; }…arrow_forward
- What is the output of running class Test? public class Test{ public static void main(String[] args) { new Circle9(); } } public abstract class GeometricObject { protected GeometricObject() { } protected GeometricObject(String color, boolean filled) { System.out.print("A"); } } System.out.print("B"); public class Circle9 extends GeometricObject { /** No-arg constructor */ public Circle9() { this(1.0); } } /** Construct circle with a specified radius */ public Circle9(double radius) { this(radius, "white", false); System.out.print("D"); } System.out.print("C"); } /** Construct a circle with specified radius, filled, and color */ public Circle9(double radius, String color, boolean filled) { super(color, filled); System.out.print("E"); ABCD BACD CBAE AEDC BEDCarrow_forwardCreate the UML Diagram for this java code import java.util.Scanner; interface Positive{ void Number();} class Square implements Positive{ public void Number() { Scanner in=new Scanner(System.in); System.out.println("Enter a number: "); int a = in.nextInt(); if(a>0) { System.out.println("Positive number"); } else { System.out.println("Negative number"); } System.out.println("\nThe square of " + a +" is " + a*a); System.out.println("\nThe cubic of "+ a + " is "+ a*a*a); }} class Sum implements Positive{ public void Number() { Scanner in = new Scanner(System.in); System.out.println("\nEnter the value for a: "); int a = in.nextInt(); System.out.println("Enter the value for b" ); int b= in.nextInt(); System.out.printf("The Difference of two numbers: %d\n", a-b); System.out.printf("The…arrow_forwardimport java.util.Scanner; public class LabProgram { public static Roster getInput(){ /* Reads course title, creates a roster object with the input title. Note that */ /* the course title might have spaces as in "COP 3804" (i.e. use nextLine) */ /* reads input student information one by one, creates a student object */ /* with each input student and adds the student object to the roster object */ /* the input is formatted as in the sample input and is terminated with a "q" */ /* returns the created roster */ /* Type your code here */ } public static void main(String[] args) { Roster course = getInput(); course.display(); course.dislayScores(); }} public class Student { String id; int score; public Student(String id, int score) { /* Student Employee */ /* Type your code here */ } public String getID() { /* returns student's id */…arrow_forward
- Consider the GameOfLife class public class GameOfLife { private BooleanProperty[][] cells; public GameOfLife() { cells = new BooleanProperty[10][10]; for (int x = 0; x < 10; x++) { for (int y = 0; y < 10; y++) { cells[x][y] = new SimpleBooleanProperty(); public void ensureAlive(int x, int y) { cells[x][y].set(true); public void ensureDead(int x, int y) { cells[x][y]•set(false); public boolean isAlive(int x, int y) { return cells[x][y]•get(); } a. Identify one code smell in this program. b. Identify and explain the most pertinent (problematic) design smell in this program.arrow_forwardCreate a UML class diagram of the application illustrating class hierarchy, collaboration, and the content of each class. There is only one class. There is a main method that calls four methods. I am not sure if I made the UML Class diagram correct. import java.util.Random; // import Random packageimport java.util.Scanner; // import Scanner Package public class SortArray{ // class name public static void main(String[] args) { //main method Scanner scanner = new Scanner(System.in); // creates object of the Scanner classint[] array = initializeArray(scanner); System.out.print("Array of randomly generated values: ");displayArray(array); // Prints unsorted array sortDescending(array); // sort the array in descending orderSystem.out.print("Values in desending order: ");displayArray(array); // prints the sorted array, should be in descending ordersortAscending(array); // sort the array in ascending orderSystem.out.print("Values in asending order: ");displayArray(array); // prints the…arrow_forwardGiven the following class definition: class Point2d { private int x, y; public Point2d () { X = 0; y = 0; } public Point2d (int i, int j) { X = i; y = j; } public void SetX (int i) { x = i; } public void SetY (int j) { y = j; } public int GetX () { return x; public int GetŸ () { return y; } public String toString() { return '[' + x + ", } + y + ']'; } } Use inheritance to create a class, Point3d, with any additional appropriate data and/or functions.arrow_forward
- StringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forwardimport javax.swing.*; import java.util.ArrayList; import java.util.Collections; import java.util.Random; import java.awt.*; import java.awt.event.*; public class memory extends JFrame implements ActionListener { private JButton[] cards; private ImageIcon[] icons; private int[] iconIDs; private JButton firstButton; private ImageIcon firstIcon; private int numMatches; public memory() { setTitle("Memory Matching Game"); setSize(800, 600); setLayout(new BorderLayout()); JPanel boardPanel = new JPanel(new GridLayout(4, 4)); add(boardPanel, BorderLayout.CENTER); icons = new ImageIcon[8]; for (int i = 1; i <= 8; i++) { icons[i-1] = new ImageIcon("image" + i + ".png"); } iconIDs = new int[16]; for (int i = 0; i < 8; i++) { iconIDs[2*i] = i; iconIDs[2*i+1] = i; } Random rand = new Random(); for (int i = 0; i < 16;…arrow_forwardPRACTICE CODE import java.util.TimerTask;import org.firmata4j.ssd1306.MonochromeCanvas;import org.firmata4j.ssd1306.SSD1306;public class CountTask extends TimerTask {private int countValue = 10;private final SSD1306 theOledObject;public CountTask(SSD1306 aDisplayObject) {theOledObject = aDisplayObject;}@Overridepublic void run() {for (int j = 0; j <= 3; j++) {theOledObject.getCanvas().clear();theOledObject.getCanvas().setTextsize(1);theOledObject.getCanvas().drawString(0, 0, "Hello");theOledObject.display();try {Thread.sleep(2000);} catch (InterruptedException e) {throw new RuntimeException(e);}theOledObject.clear();theOledObject.getCanvas().setTextsize(1);theOledObject.getCanvas().drawString(0, 0, "My name is ");theOledObject.display();try {Thread.sleep(2000);} catch (InterruptedException e) {throw new RuntimeException(e);}while (true) {for (int i = 10; i >= 0; i--)…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
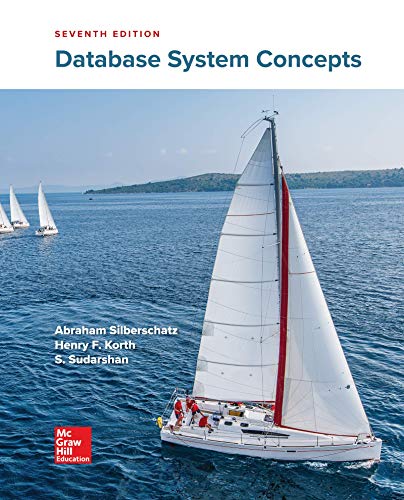
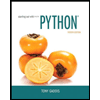
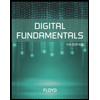
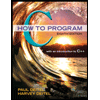
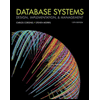
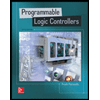