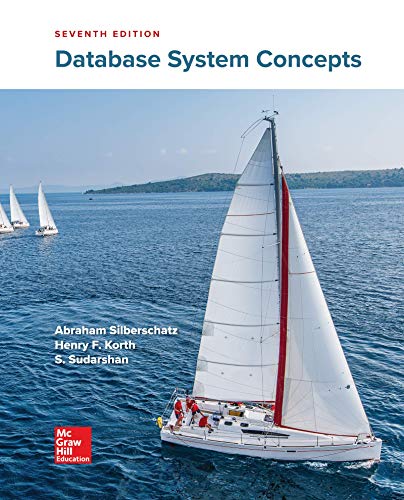
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
thumb_up100%
import javax.swing.*;
import java.awt.*;
import java.util.LinkedList;
class GraphPanel extends JPanel {
private LinkedList<Integer> sensorValues;
public GraphPanel(LinkedList<Integer> sensorValues) {
this.sensorValues = sensorValues;
}
public void updateValues(LinkedList<Integer> values) {
this.sensorValues = values;
repaint();
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
int xOffset = 50;
int yOffset = 50;
int xSpacing = (getWidth() - 2 * xOffset) / sensorValues.size();
g.setColor(Color.BLACK);
g.drawLine(xOffset, getHeight() - yOffset, getWidth() - xOffset, getHeight() - yOffset); // X-axis
g.drawLine(xOffset, getHeight() - yOffset, xOffset, yOffset); // Y-axis
g.setColor(Color.BLUE);
int prevX = xOffset;
int prevY = getHeight() - yOffset - (sensorValues.get(0) * (getHeight() - 2 * yOffset) / 1000);
for (int i = 1; i < sensorValues.size(); i++) {
int value = sensorValues.get(i);
int x = xOffset + i * xSpacing;
int y = getHeight() - yOffset - (value * (getHeight() - 2 * yOffset) / 1000); // Scale for display
g.drawLine(prevX, prevY, x, y); // Draw line between previous and current points
prevX = x;
prevY = y;
}
// Label the x-axis
for (int i = 0; i < sensorValues.size(); i++) {
int xLabel = xOffset + i * xSpacing;
int yLabel = getHeight() - yOffset + 20;
g.drawString(Integer.toString(i), xLabel, yLabel);
}
// Label the y-axis
for (int i = 0; i <= 1000; i += 100) {
int xLabel = xOffset - 30;
int yLabel = getHeight() - yOffset - (i * (getHeight() - 2 * yOffset) / 1000);
g.drawString(Integer.toString(i), xLabel, yLabel);
}
}
import java.awt.*;
import java.util.LinkedList;
class GraphPanel extends JPanel {
private LinkedList<Integer> sensorValues;
public GraphPanel(LinkedList<Integer> sensorValues) {
this.sensorValues = sensorValues;
}
public void updateValues(LinkedList<Integer> values) {
this.sensorValues = values;
repaint();
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
int xOffset = 50;
int yOffset = 50;
int xSpacing = (getWidth() - 2 * xOffset) / sensorValues.size();
g.setColor(Color.BLACK);
g.drawLine(xOffset, getHeight() - yOffset, getWidth() - xOffset, getHeight() - yOffset); // X-axis
g.drawLine(xOffset, getHeight() - yOffset, xOffset, yOffset); // Y-axis
g.setColor(Color.BLUE);
int prevX = xOffset;
int prevY = getHeight() - yOffset - (sensorValues.get(0) * (getHeight() - 2 * yOffset) / 1000);
for (int i = 1; i < sensorValues.size(); i++) {
int value = sensorValues.get(i);
int x = xOffset + i * xSpacing;
int y = getHeight() - yOffset - (value * (getHeight() - 2 * yOffset) / 1000); // Scale for display
g.drawLine(prevX, prevY, x, y); // Draw line between previous and current points
prevX = x;
prevY = y;
}
// Label the x-axis
for (int i = 0; i < sensorValues.size(); i++) {
int xLabel = xOffset + i * xSpacing;
int yLabel = getHeight() - yOffset + 20;
g.drawString(Integer.toString(i), xLabel, yLabel);
}
// Label the y-axis
for (int i = 0; i <= 1000; i += 100) {
int xLabel = xOffset - 30;
int yLabel = getHeight() - yOffset - (i * (getHeight() - 2 * yOffset) / 1000);
g.drawString(Integer.toString(i), xLabel, yLabel);
}
}
update this code so that the y axis is labelled "sensor values" and x axis is labelled "time in seconds"
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- import java.util.LinkedList; public class IntTree { private Node root; private static class Node { public int key; public Node left, right; public Node(int key) { this.key = key; } } public void printInOrder() { printInOrder(root); } private void printInOrder(Node n) { if (n == null) return; printInOrder(n.left); System.out.println(n.key); printInOrder(n.right); } /* * Returns true if this tree and that tree "look the same." (i.e. They have the * same keys in the same locations in the tree.) Note that just having the same * keys is NOT enough. They must also be in the same positions in the tree. */ public boolean treeEquals(IntTree that) { // TODO throw new RuntimeException("Not implemented"); } • You are not allowed to use any kind of loop in your solutions. • You may not modify the Node class in any way• You may not modify the function…arrow_forwardpackage hw5; public class LinkedIntSet {private static class Node {private int data;private Node next; public Node(int data, Node next) {this.data = data;this.next = next;}} private Node first; // Always points to the first node of the list.// THE LIST IS ALWAYS IN SORTED ORDER!private int size; // Always equal to the number of elements in the set. /*** Construts an empty set.*/public LinkedIntSet() {throw new RuntimeException("Not implemented");} /*** Returns the number of elements in the set.* * @return the number of elements in the set.*/public int size() {throw new RuntimeException("Not implemented");} /*** Tests if the set contains a number* * @param i the number to check* @return <code>true</code> if the number is in the set and <code>false</code>* otherwise.*/public boolean contains(int i) {throw new RuntimeException("Not implemented");} /*** Adds <code>element</code> to this set if it is not already present and* returns…arrow_forwardHi i need an implementation on the LinkListDriver and the LinkListOrdered for this output : Enter your choice: 1 Enter element: Mary List Menu Selections 1. add element 2_remove element 3.head element 4. display 5.Exit Enter your choice: 3 Element @ head: Mary List Menu Selections 1-add element 2-remove element 3_head element 4.display 5-Exit Enter your choice: 4 1. Mary List Menu Selections 1. add element 2. remove element 3.head element 4. display 5. Exit Enter your choice: LinkedListDriver package jsjf; import java.util.LinkedList; import java.util.Scanner; public class LinkedListDriver { public static void main(String [] args) { Scanner input = new Scanner(System.in); LinkedList list = new LinkedList(); int menu = 0; do { System.out.println("\nList menu selection\n1.Add element\n2.Remove element\n3.Head\n4.Display\n5.Exit"); System.out.println();…arrow_forward
- import java.util.*;public class MyLinkedListQueue{ class Node { public Object data; public Node next; private Node first; public MyLinkedListQueue() { first = null; } public Object peek() { if(first==null) { throw new NoSuchElementException(); } return first.data; } public void enqueue(Object element) { Node newNode = new newNode(); newNode.data = element; newNode.next = first; first = newNode; } public boolean isEmpty() { return(first==null); } public int size() { int count = 0; Node p = first; while(p ==! null) { count++; p = p.next; } return count; } } } Hello, I am getting an error message when I execute this program. This is what I get: MyLinkedListQueue.java:11: error: invalid method declaration; return type required public MyLinkedListQueue() ^1 error I also need to have a dequeue method in my program. It is very similar to the enqueue method. I'll…arrow_forwardComplete public class Solution { public static LinkedListNode<Integer> findPrevNode(LinkedListNode<Integer> head, int count) { for (int i=0;i<count-1;i++) { head=head.next; } return head; } public static LinkedListNode<Integer> swapNodes(LinkedListNode<Integer> head, int i, int j) { //Your code goes here if (head==null) { return head; } else if (j==0 || (i-j==-1)) { int temp=i; i=j; j=temp; } LinkedListNode<Integer> swap1=null,swap2=null,p1=null,n1=null,p2=null,n2=null; if (i==0 && i-j==1) { swap1=head; swap2=head.next; swap1=swap1.next; head=swap2; swap2.next=swap1; } else if(i-j==1) { p1=findPrevNode(head,j); swap1=p1.next; swap2=swap1.next; n2=swap2.next; //System.out.println(p1.data); //System.out.println(swap1.data);…arrow_forwardGiven the tollowiıng class template detinition: template class linkedListType { public: const linked ListType& operator=(const linked ListType&); I/Overload the assignment operator. void initializeList(); /Initialize the list to an empty state. //Postcondition: first = nullptr, last = nullptr, count = 0; linked ListType(); Ildefault constructor //Initializes the list to an empty state. /Postcondition: first = nullptr, last = nullptr, count = 0; -linkedListīype(); Ildestructor /Deletes all the nodes from the list. //Postcondition: The list object is destroyed. protected: int count; //variable to store the number of elements in the list nodeType *first; //pointer to the first node of the list nodeType *last; //pointer to the last node of the list 1) Write initializeList() 2) Write linkedListType() 3) Write operator=0 4) Add the following function along with its definition: void rotate(); I/ Remove the first node of a linked list and put it at the end of the linked listarrow_forward
- Redesign LaptopList class from previous project public class LaptopList { private class LaptopNode //inner class { public String brand; public double price; public LaptopNode next; public LaptopNode(String brand, double price) { // add your code } public String toString() { // add your code } } private LaptopNode head; // head of the linked list public LaptopList(String fname) throws IOException { File file = new File(fname); Scanner scan = new Scanner(file); head = null; while(scan.hasNextLine()) { // scan data // create LaptopNode // call addToHead and addToTail alternatively } } private void addToHead(LaptopNode node) { // add your code } private void addToTail(LaptopNode node) { // add your code } private…arrow_forwardBelow you're given a Node class and a LinkedList class. You will implement a method for the LinkedList class named "delete48in148". That is, whenever there is a sequence of nodes with values 1, 4, 8, we delete the 4 and 8. For exCample, Before: 1 -> 4 -> 8 LAfter: 1 Before: 7 -> 1 -> 4 -> 8 -> 9 -> 4 -> 8 After: 7 -> 1 -> 9 -> 4 -> 8 Before: 7 -> 1 -> 4 -> 8 -> 4 -> 8 -> 4 -> 8 -> 9 After: 7 -> 1 -> 9 Note from the above example that, after deleting one instance of 48, there may be new instances of 148 formed. You must delete ALL of them. Requirement: Your implementation must be ITERATIVE (i.e., using a loop). You must NOT use recursion. Recursive solutions will NOT be given marks. import ... # No other import is allowedarrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
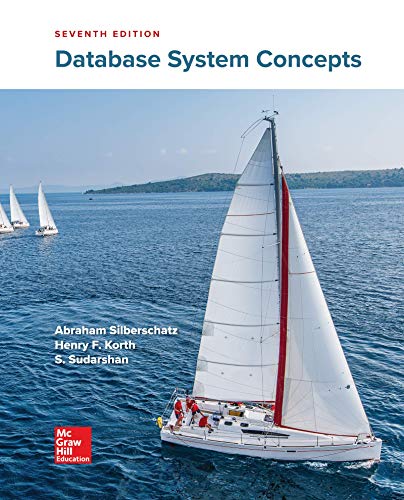
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
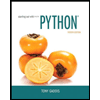
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
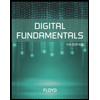
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
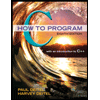
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
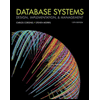
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
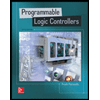
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education