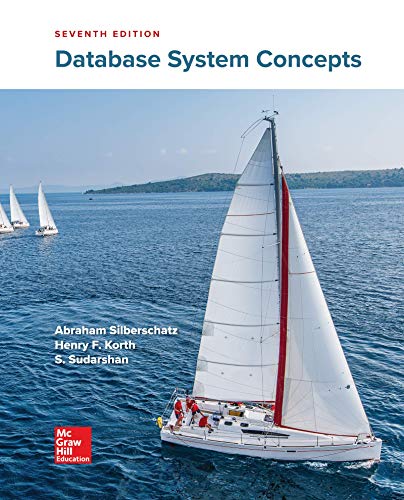
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print(" ");
String s1 = sc.nextLine();
System.out.print("");
String s2 = sc.nextLine();
int minLen = Math.min(s1.length(), s2.length());
int matchCount = 0;
for (int i = 0; i < minLen; i++) {
if (s1.charAt(i) == s2.charAt(i)) {
matchCount++;
}
}
if (matchCount == 1) {
System.out.println("1 character matches");
} else {
System.out.println(matchCount + " characters match");
}
sc.close();
}
}
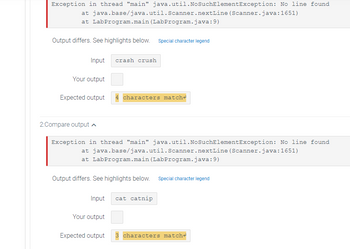

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- public class main { public static void main(String [] args) { Dog dog1=new Dog(“Spark”,2),dog2=new Dog(“Sammy”,3); swap(dog1, dog2); System.out.println(“dog1 is ”+ dog1); System.out.println(“dog2 is ”+ dog2); } public static void swap(Dog a, Dog b) { String nameA = a.getName(); String nameB = b.getName(); a.setName(nameB); b.setName(nameA); } What is the output of the main()?arrow_forwardpublic class Animal { public static int population; private int age; } public Animal (int age) { this.age = age; population++; } + msg); public void say (String msg) { System.out.print("Saying: " System.out.print(" for "); System.out.print(getHuman Years()); System.out.println(" years."); } public int getHuman Years() { return age; } public class Mammal extends Animal { private String species; public Mammal(String species, int age) { super (age); this. species species; } } } = public int getHuman Years() { if (species.equals("dog")) 1 else return super.getHumanYears() * 7; return super.getHumanYears(); €arrow_forwardStringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forward
- import java.util.Scanner; public class Inventory { public static void main (String[] args) { Scanner scnr = new Scanner(System.in); InventoryNode headNode; InventoryNode currNode; InventoryNode lastNode; String item; int numberOfItems; int i; // Front of nodes list headNode = new InventoryNode(); lastNode = headNode; int input = scnr.nextInt(); for(i = 0; i < input; i++ ) { item = scnr.next(); numberOfItems = scnr.nextInt(); currNode = new InventoryNode(item, numberOfItems); currNode.insertAtFront(headNode, currNode); lastNode = currNode; } // Print linked list currNode = headNode.getNext(); while (currNode != null) { currNode.printNodeData(); currNode…arrow_forwardimport java.util.Scanner;public class MP2{public static void main (String[] args){//initialization and declarationScanner input = new Scanner (System.in);String message;int Option1;int Option2;int Quantity;int TotalBill;int Cash;int Change;final int SPECIALS = 1;final int BREAKFAST = 2;final int LUNCH = 3;final int SANDWICHES = 4;final int DRINKS = 5;final int DESSERTS = 6;//For the Special, Breakfast, Lunch Optionsfinal int Meals1 = 11;final int Meals2 = 12;final int Meals3 = 13;final int Meals4 = 14;//First menu and First OptionSystem.out.println("\t Welcome to My Kitchen ");System.out.println("\t\t SPECIALS");System.out.println("\t\t [2] BREAKFAST MEALS");System.out.println("\t\t [3] LUNCH MEALS");System.out.println("\t\t [4] SANDWICHES");System.out.println("\t\t [5] DRINKS");System.out.println("\t\t [6] DESSERTS");System.out.println("\t\t [0] EXIT");System.out.print("Choose an option ");Option1 = input.nextInt();//first switch case statementsswitch(Option1){case (SPECIALS):message…arrow_forward8) Now use the Rectangle class to complete the following tasks: - Create another object of the Rectangle class named box2 with a width of 100 and height of 50. Note that we are not specifying the x and y position for this Rectangle object. Hint: look at the different constructors) Display the properties of box2 (same as step 7 above). - Call the proper method to move box1 to a new location with x of 20, and y of 20. Call the proper method to change box2's dimension to have a width of 50 and a height of 30. Display the properties of box1 and box2. - Call the proper method to find the smallest intersection of box1 and box2 and store it in reference variable box3. - Calculate and display the area of box3. Hint: call proper methods to get the values of width and height of box3 before calculating the area. Display the properties of box3. 9) Sample output of the program is as follow: Output - 1213 Module2 (run) x run: box1: java.awt. Rectangle [x=10, y=10,width=40,height=30] box2: java.awt.…arrow_forward
- public class Test { } public static void main(String[] args) { int a = 5; a += 5; } switch(a) { case 5: } System.out.print("5"); break; case 10: System.out.print("10"); System.out.print("0"); default:arrow_forwardimport java.util.Scanner; public class AutoBidder { public static void main (String [] args) { Scanner scnr = new Scanner (System.in); char keepGoing; int nextBid; nextBid = 0; keepGoing 'y'; * Your solution goes here */ while { nextBid = nextBid + 3; System.out.println ("I'll bid $" + nextBid + "!") ; System.out.print ("Continue bidding? (y/n) "); keepGoing = scnr.next ().charAt (0); } System.out.println (""); }arrow_forwardpackage lab06;;public class gradereport { public static void main(String[] args) { Scanner in = new Scanner(System.in);double[] Scores = new double[10]; for(int i=0;i<10;i++){ System.out.println("Enter score " + (i+1));scores[i]=in.nextdouble(); } for(int i=0;i<10;i++){ if (scores[i] >=80) System.out.println("Score " + (i+1) + " receives a grade of HD"); else if (scores[i]>=70) System.out.println("Score " + (i+1) + " receives a grade of D"); else if (scores[i] >=60) System.out.println("Score "+ (i+1) + " receives a grade of C"); else if (scores[i] >=50) System.out.println("Score " + (i+1) + " receives a grade of P"); else if (scores[i] >=40) System.out.println("Score " + (i+1) + " receives a grade of MF"); else if (scores[i] >=0) System.out.println("Score " +…arrow_forward
- import java.util.Scanner;public class main{public static void main(String[] args){Scanner sc = new Scanner(System.in):int year, day, weekday;String month;String outday = "";System.out.printf("Enter the month%n");month = sc.nextLine();System.out.printf("Enter the day%n");month = sc.nextInt();weekay = find_day(month, day);outday = out_weekday(weekday);System.out.printf("The day is: %s%n", outday);}} Change the program from the previous example to take command line arguments instead of using the Scanner. For example, running the program like this:> java OutDays March 14will output the weekday string (which is Thursday) on the console for March 14, 2019). public static void main(String[] args){ int year, day, weekday; String month; String outday = ""; // #### your code here for accessing the command line arguments weekday = find_day(month, day); outday = out_weekday(weekday); System.out.printf("The day is: %s%n", outday);}arrow_forwardimport java.awt.*; public class TestRectangle {public static void main(String[] args) {Rectangle r1 = new Rectangle(5, 4, 10, 17);Rectangle r2 = new Rectangle(10, 10, 20, 3);Rectangle r3 = new Rectangle(0, 1, 12, 15);Rectangle r4 = new Rectangle(10, 10, 20, 3);System.out.println("r1 = " + r1);System.out.println("r2 = " + r2);System.out.println("r3 = " + r3);System.out.println("r2 equals r1? " + r2.equals(r1));System.out.println("r2 equals r2? " + r2.equals(r2));System.out.println("r2 equals r3? " + r2.equals(r3));System.out.println("r2 equals r4? " + r2.equals(r4)); System.out.println("r1 contains (6, 8)? = " + r1.contains(6, 8));System.out.println("r2 contains (6, 8)? = " + r2.contains(6, 8));System.out.println("r3 contains (6, 8)? = " + r3.contains(6, 8));System.out.println("r2 contains (30, 13)? = " + r2.contains(30, 13)); r1.intersect(r3);r2.intersect(r4);System.out.println("r1 intersect r3 = " + r1);System.out.println("r2 intersect r4 = " + r2);…arrow_forwardpublic class worksheet3_1 { public static void main(String[] arg) { ShadowingExample example = new ShadowingExample(); example.x = 99; example.sampleMethod(); }}class ShadowingExample { int x; public void sampleMethod() { int x = 0; System.out.println("the value of local variable x = " + ………………………………………………); System.out.println("the value of instance variable x = " + …………………………………………); }} what should be written in the second print statement so that the output is: the value of instance variable x = 99 what should be written in the first print statement so that the output is: the value of local variable x = 0 options are: samplemethod.x this.x shadowingexample.x x Answer 1 Question 1arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
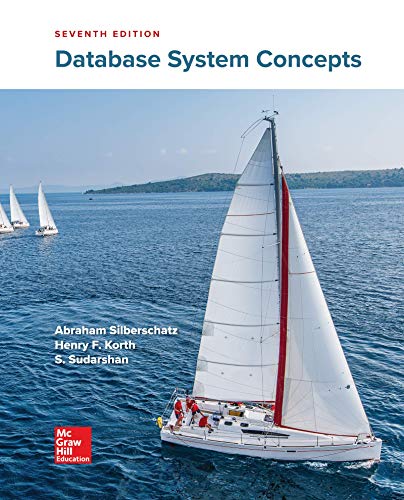
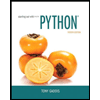
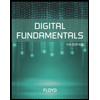
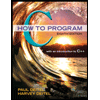
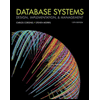
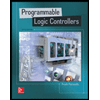