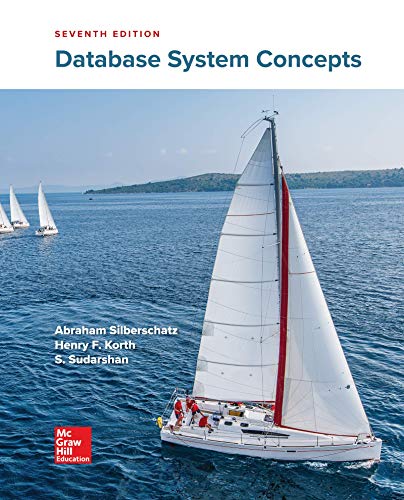
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
import java.util.Map;
import java.util.TreeMap;
public class Maps
{
/**
Returns a new map with common key-value pairs.
@param map1 the first map to compare
@param map2 the second map to compare
@return a map containing entries common to both maps
*/
public static Map<String, Integer> getCommon(Map<String, Integer> map1,
Map<String, Integer> map2)
{
Map<String, Integer> common = new TreeMap<>();
/* code goes here */
return common;
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 3. Define a Pair class using Java Generics framework a. Properties: first, second b. Constructors: Create 2 different constructor with different initialization parameters c. Methods: setFirst(), setSecond(), getFirst(), getSecond(); d. Test Pair class print out the value of Pair instance: i. Define a Pair instance and assign (10,10.1) to the pair; ii. Define a Pair instance and assign (8.2, "ABC") to the pair; iii. Define an array holding[] of Pair class. 1. holding has 100 elements; 2. Assign (int, double) to array by using loop -> (0, 100.0), (1, 99.0), (2, 98.0), ..., (99, 1.0).arrow_forwardimport java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set<Integer> set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set<Integer> numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set…arrow_forwardimport java.util.HashMap; import java.util.Map; public class LinearSearchMap { // Define a method that takes in a map and a target value as parameters public static boolean linearSearch(Map<String, Integer> map, int target) { // Iterate through each entry in the map for () { // Check if the value of the current entry is equal to the target if () { // If the value is equal to the target, return true } } // If no entry with the target value is found, return false } public static void main(String[] args) { // Create a HashMap of strings and integers Map<String, Integer> numbers = new HashMap<>(); // Populate the HashMap with key-value pairs numbers.put("One", 1); numbers.put("Two", 2); numbers.put("Three", 3); numbers.put("Four", 4); numbers.put("Five", 5); // Set the target value to search for…arrow_forward
- Java Code: Create a Parser class. Much like the Lexer, it has a constructor that accepts a LinkedList of Token and creates a TokenManager that is a private member. The next thing that we will build is a helper method – boolean AcceptSeperators(). One thing that is always tricky in parsing languages is that people can put empty lines anywhere they want in their code. Since the parser expects specific tokens in specific places, it happens frequently that we want to say, “there HAS to be a “;” or a new line, but there can be more than one”. That’s what this function does – it accepts any number of separators (newline or semi-colon) and returns true if it finds at least one. Create a Parse method that returns a ProgramNode. While there are more tokens in the TokenManager, it should loop calling two other methods – ParseFunction() and ParseAction(). If neither one is true, it should throw an exception. bool ParseFunction(ProgramNode) bool ParseAction(ProgramNode) -Creates ProgramNode,…arrow_forward/** * This class will use Nodes to form a linked list. It implements the LIFO * (Last In First Out) methodology to reverse the input string. * **/ public class LLStack { private Node head; // Constructor with no parameters for outer class public LLStack( ) { // to do } // This is an inner class specifically utilized for LLStack class, // thus no setter or getters are needed private class Node { private Object data; private Node next; // Constructor with no parameters for inner class public Node(){ // to do // to do } // Parametrized constructor for inner class public Node (Object newData, Node nextLink) { // to do: Data part of Node is an Object // to do: Link to next node is a type Node } } // Adds a node as the first node element at the start of the list with the specified…arrow_forwardImplement a Single linked list to store a set of Integer numbers (no duplicate) • Instance variable• Constructor• Accessor and Update methods 2.) Define SLinkedList Classa. Instance Variables: # Node head # Node tail # int sizeb. Constructorc. Methods # int getSize() //Return the number of nodes of the list. # boolean isEmpty() //Return true if the list is empty, and false otherwise. # int getFirst() //Return the value of the first node of the list. # int getLast()/ /Return the value of the Last node of the list. # Node getHead()/ /Return the head # setHead(Node h)//Set the head # Node getTail()/ /Return the tail # setTail(Node t)//Set the tail # addFirst(E e) //add a new element to the front of the list # addLast(E e) // add new element to the end of the list # E removeFirst()//Return the value of the first node of the list # display()/ /print out values of all the nodes of the list # Node search(E key)//check if a given…arrow_forward
- #include <iostream> usingnamespace std; class Queue { int size; int* queue; public: Queue(){ size = 0; queue = new int[100]; } void add(int data){ queue[size]= data; size++; } void remove(){ if(size ==0){ cout <<"Queue is empty"<<endl; return; } else{ for(int i =0; i < size -1; i++){ queue[i]= queue[i +1]; } size--; } } void print(){ if(size ==0){ cout <<"Queue is empty"<<endl; return; } for(int i =0; i < size; i++){ cout<<queue[i]<<" <- "; } cout << endl; } //your code goes here }; int main(){ Queue q1; q1.add(42); q1.add(2); q1.add(8); q1.add(1); Queue q2; q2.add(3); q2.add(66); q2.add(128); q2.add(5); Queue q3 = q1+q2; q3.print();…arrow_forwardA map is a container that stores a collection of ordered pairs, each pair consists of a key and a value, <key, value>. Keys must be unique. Values need not be unique, so several keys can map to the same values. The pairs in the map are sorted based on keys. Group of answer choices True Falsearrow_forwardDesign an implementation of an Abstract Data Type consisting of a set with the following operations: insert(S, x) Insert x into the set S. delete(S, x) Delete x fromthe set S. member(S, x) Return true if x ∈ S, false otherwise. position(S, x) Return the number of elements of S less than x. concatenate(S, T) Set S to the union of S and T, assuming every element in S is smaller than every element of T. All operations involving n-element sets are to take time O(log n).arrow_forward
- Please fill in the code gaps if possible. This problem has been giving me trouble. Any help is appreciated. public class Node { private String element; // we assume elements are character strings private Node next; /* Creates a node with the given element and next node. */ public Node(String s, Node n) { //fill in body } /* Returns the element of this node. */ public String getElement() { //fill in body } /* Returns the next node of this node. */ public Node getNext() { //fill in body } // Modifier methods: /* Sets the element of this node. */ public void setElement(String newElem) { //fill in body } /* Sets the next node of this node. */ public void setNext(Node newNext) { //fill in body } }arrow_forwardComputer Science PLEASE HELP TO CONVERT THIS FOR LOOP TO FOREACH LOOP using System;using System.Collections.Generic; namespace SwinAdventure{public class IdentifierableObject{//field ,datapublic List<string> Identifier = new List<string>(); //constructorpublic IdentifierableObject(string[] ident){for (int i = 0; i < ident.Length; i++){Identifier.Add(ident[i]);}} // Methodpublic void AddIdentifier(string id){Identifier.Add(id.ToLower());} // propertiespublic string FirstID{get{return Identifier[0];}} public bool AreYou(string id){for (int i = 0; i < Identifier.Count; i++){if (Identifier[i] == id.ToLower()){return true;} }return false;}}}arrow_forwardclass Queue { private static int front, rear, capacity; private static int queue[]; Queue(int c) { front = rear = 0; capacity = c; queue = new int[capacity]; } static void queueEnqueue(int data) { if (capacity == rear) { System.out.printf("\nQueue is full\n"); return; } else { queue[rear] = data; rear++; } return; } static void queueDequeue() { if (front == rear) { System.out.printf("\nQueue is empty\n"); return; } else { for (int i = 0; i < rear - 1; i++) { queue[i] = queue[i + 1]; } if (rear < capacity) queue[rear] = 0; rear--; } return; } static void queueDisplay() { int i; if (front == rear) { System.out.printf("\nQueue is Empty\n"); return; } for (i = front; i < rear; i++) { System.out.printf(" %d <-- ", queue[i]); } return; } static void queueFront() { if (front == rear) { System.out.printf("\nQueue is Empty\n"); return; } System.out.printf("\nFront Element is: %d", queue[front]);…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
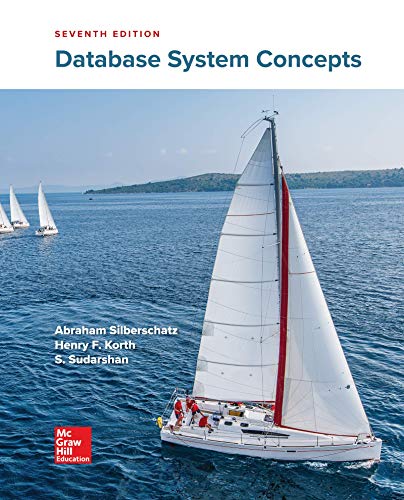
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
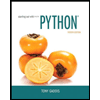
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
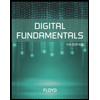
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
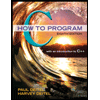
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
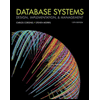
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
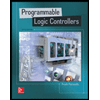
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education