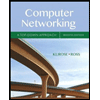
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
#include<iostream> using namespace std; class Base { public: virtual void f() { cout<<"Base\n"; } }; class Derived:public Base { public: void f() { cout<<"Derived\n"; } }; main() { Base *p = new Derived(); p->f(); }
A - Base
B - Derived
C - Compile error
D - None of the above.
c++
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Similar questions
- Define a header file named BST.h. In this header file, declare your BST class./*** header file BST.h*/class BST {private:struct TreeNode {int val;TreeNode* left;TreeNode* right;};TreeNode* root;// private function declarations here....public:// public function declarations here...} (C++)arrow_forward#include <iostream>using namespace std;class st{private:int arr[100];int top;public:st(){top=-1;}void push(int ItEM) {top++;arr[top]=ItEM; }bool ise() {return top<0;} int pop(){int Pop;if (ise()) cout<<"Stack is emptye ";else{Pop=arr[top];top--;return Pop;}}int Top(){int TOP;if (ise()) cout<<" empty ";else {TOP=arr[top];return TOP;}}void screen(){ for (int i = 0; i <top+1 ; ++i) {cout<<arr[i];} }};int main() {st c;c.push(1);c.push(2);c.push(3);c.push(4);c.pop();c.push(5);c.screen();return 0;} alternative to this code??arrow_forwardC++ Data Structure:Create an AVL Tree C++ class that works similarly to std::map, but does NOT use std::map. In the PRIVATE section, any members or methods may be modified in any way. Standard pointers or unique pointers may be used.** MUST use given Template below: #ifndef avltree_h#define avltree_h #include <memory> template <typename Key, typename Value=Key> class AVL_Tree { public: classNode { private: Key k; Value v; int bf; //balace factor std::unique_ptr<Node> left_, right_; Node(const Key& key) : k(key), bf(0) {} Node(const Key& key, const Value& value) : k(key), v(value), bf(0) {} public: Node *left() { return left_.get(); } Node *right() { return right_.get(); } const Key& key() const { return k; } const Value& value() const { return v; } const int balance_factor() const {…arrow_forward
- C++ language (Composition problem) Given the following two classes A and B, where all member functions have been fully implemented inline. class A { public: void f(int arg) { data = arg; } int g() { return data; } private: int data; }; class B { public: A x; }; int main() { B obj; // ... write code here return 0; } a. Insert the code in the main() function that assigns integer value 99 to the “data” member of obj, which is a B class object). b.Write the code to display the value (i.e., 99) that has just been assigned to the obj. c. Note that only a single object obj of B class is declared. How many times are constructors called? If more than one constructor is called, in what order are they called?arrow_forwardvoid fun(int i) { do { if (i % 2 != 0) cout =1); cout << endl; } int main() { int i = 1; while (i <= 8) { fun(i); it; } cout <arrow_forwardC:/Users/r1821655/CLionProjects/untitled/sequence.cpp:48:5: error: return type specification for constructor invalidtemplate <class Item>class sequence{public:// TYPEDEFS and MEMBER SP2020typedef Item value_type;typedef std::size_t size_type;static const size_type CAPACITY = 10;// CONSTRUCTORsequence();// MODIFICATION MEMBER FUNCTIONSvoid start();void end();void advance();void move_back();void add(const value_type& entry);void remove_current();// CONSTANT MEMBER FUNCTIONSsize_type size() const;bool is_item() const;value_type current() const;private:value_type data[CAPACITY];size_type used;size_type current_index;};} 48 | void sequence<Item>::sequence() : used(0), current_index(0) { } | ^~~~ line 47 template<class Item> line 48 void sequence<Item>::sequence() : used(0), current_index(0) { }arrow_forward
- 6. Given: class Pet { public: virtual void eat() { cout eat(); } What is the result of compiling and running the above program? m. The program compiles and crashes when it runs. i. The program compiles and runs, printing "Pet::eat" j. The program compiles and runs, printing "Cat::eat" The program compiles and runs to completion without printing anything. n. o. None of the above. k. The program fails to compile because the method eat is not marked virtual in Cat. I. The program fails to compile for some other reason.arrow_forward#include <iostream> #include <string> #include <cstdlib> using namespace std; template<class T> class A { public: T func(T a, T b){ return a/b; } }; int main(int argc, char const *argv[]) { A <float>a1; cout<<a1.func(3,2)<<endl; cout<<a1.func(3.0,2.0)<<endl; return 0; } Give output for this code.arrow_forwardclass implementation file -- Rectangle.cpp class Rectangle { #include #include "Rectangle.h" using namespace std; private: double width; double length; public: void setWidth (double); void setLength (double) ; double getWidth() const; double getLength() const; double getArea () const; } ; // set the width of the rectangle void Rectangle::setWidth (double w) { width = w; } // set the length of the rectangle void Rectangle::setLength (double l) { length l; //get the width of the rectangle double Rectangle::getWidth() const { return width; // more member functions herearrow_forward
- C++ Given code #include <iostream>using namespace std; class Node {public:int data;Node *pNext;}; void displayNumberValues( Node *pHead){while( pHead != NULL) {cout << pHead->data << " ";pHead = pHead->pNext;}cout << endl;} //Option 1: Search the list// TODO: complete the function below to search for a given value in linked lsit// return true if value exists in the list, return false otherwise. ?? linkedlistSearch( ???){ } //Option 2: get sum of all values// TODO: complete the function below to return the sum of all elements in the linked list. ??? getSumOfAllNumbers( ???){ } int main(){int userInput;Node *pHead = NULL;Node *pTemp;cout<<"Enter list numbers separated by space, followed by -1: "; cin >> userInput;// Keep looping until end of input flag of -1 is givenwhile( userInput != -1) {// Store this number on the listpTemp = new Node;pTemp->data = userInput;pTemp->pNext = pHead;pHead = pTemp;cin >> userInput;}cout <<"…arrow_forwardCourse: Data Structure and Algorithims Language: Java Kindly make the program in 2 hours. Task is well explained. You have to make the proogram properly in Java: Restriction: Prototype cannot be change you have to make program by using given prototype. TAsk: Create a class Node having two data members int data; Node next; Write the parametrized constructor of the class Node which contain one parameter int value assign this value to data and assign next to null Create class LinkList having one data members of type Node. Node head Write the following function in the LinkList class publicvoidinsertAtLast(int data);//this function add node at the end of the list publicvoid insertAthead(int data);//this function add node at the head of the list publicvoid deleteNode(int key);//this function find a node containing "key" and delete it publicvoid printLinkList();//this function print all the values in the Linklist public LinkListmergeList(LinkList l1,LinkList l2);// this function…arrow_forwardDesign a struct with following members: p_num_lots, int* type pa_lots, int [] type (dynamically allocated) Implement following methods: parameterized constructor which takes an int for num of lots copy constructor copy assignment operator destructorarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
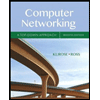
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
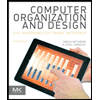
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
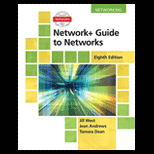
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
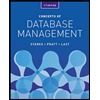
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
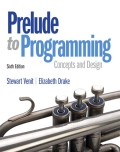
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
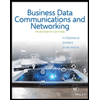
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY