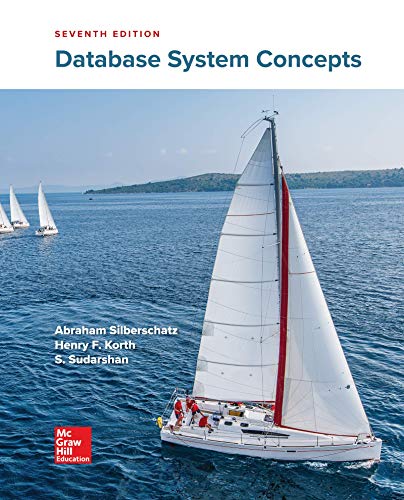
Concept explainers
Is it possible to demonstrate the program with the text file and the output printed in the text files created using class and dict.
example
def canConstruct(ransomNote: str, magazine: str) -> bool:
if len(ransomNote) > len(magazine):
return False
magazinedict = dict()
for letter in magazine:
if letter not in magazinedict:
magazinedict[letter] = 1
else:
magazinedict[letter] += 1
for letter in ransomNote:
if letter not in magazinedict:
return False
if letter in magazinedict and magazinedict[letter] <= 0:
return False
magazinedict[letter] -= 1
return True
with open('lab1_input.txt') as text:
for line in text.readlines():
strings = list(map(str,line.split()))
print(strings, end="\t")
ransomNote = strings[0]
magazine = strings[1]
output = canConstruct(ransomNote,magazine)
print('-\t'+str(output))
outfile = open('lab1_output.txt', 'a')
outfile.write(str(output)+'\n')
outfile.close()

This is very simple.
This is how we can demonstrate the program with the text file and output printed in the text files.
----------------------------------------------------- Python ---------------------------------------------------------
def canConstruct(ransomNote: str, magazine: str) -> bool:
if len(ransomNote) > len(magazine):
return False
magazinedict = dict()
for letter in magazine:
if letter not in magazinedict:
magazinedict[letter] = 1
else:
magazinedict[letter] += 1
for letter in ransomNote:
if letter not in magazinedict:
return False
if letter in magazinedict and magazinedict[letter] <= 0:
return False
magazinedict[letter] -= 1
return True
with open('lab1_input.txt', 'r') as text:
for line in text.readlines():
strings = list(map(str, line.split()))
print(strings, end="\t")
ransomNote = strings[0]
magazine = strings[1]
output = canConstruct(ransomNote, magazine)
print('-\t'+str(output))
for line in text :
outfile = open('lab1_output.txt', 'a')
outfile.writelines(str(output)+'\n')
outfile.close()
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- public class utils { * Modify the method below. The method below, myMethod, will be called from a testing function in VPL. * write one line of Java code inside the method that adds one string * to another. It will look something like this: * Assume that 1. String theInput is 2. string mystring is ceorge неllo, пу nane is * we want to update mystring so that it is неllo, пу nane is Ceorge */ public static string mymethod(string theInput){ System.out.println("This method combines two strings."); Systen.out.println("we combined two strings to make mystring); return mystring;arrow_forwardThe following code implements a Java Pasta timer that looks like the application below.• Once the start button is pressed the timer will count to two minutes.• The timer can be stopped by pressing stop.• When the timer is finished, it should pop-up an appropriate dialog box that states the timeis up.You are given a partial implementation of program. Fill out the missing code to complete theprogram import javax.swing.*;import java.awt.event.*;import javax.swing.border.*;public class PastaTimerApp extends implements { private JProgressBar progrBar; private JButton startButton, stopButton; private JLabel textLabel; private PastaTimer PastaTimer; public PastaTimerApp() { JPanel p = new JPanel(); p.setLayout(new BoxLayout(p, BoxLayout.Y_AXIS)); p.setBorder(new TitledBorder("Pasta Timer:")); progrBar = new JProgressBar(JProgressBar.HORIZONTAL, 0, 540); textLabel = new JLabel(" 0 Seconds "); p.add(progrBar); p.add(textLabel); // Initialize buttons and add action listeners JPanel controls =…arrow_forwardimport java.util.*; public class Budgeter {public static void main(String[] args) {Scanner console = new Scanner(System.in); please use java and start the code the way provided and do NOT use \n or printf in the code please.arrow_forward
- Write a Fraction class that works with the FractionMath program above. It should use your Fraction.java file to run.Main Program:public class FractionMath{public static void main(String[] arguments){// create four fractions accountsFraction w = new Fraction();Fraction x = new Fraction(3,4);Fraction y = new Fraction(2,5);Fraction z = new Fraction(6,0);System.out.println("*** Does the toStringMethod work?"); System.out.println("First fraction : " + w); System.out.println("Second fraction : " + x); System.out.println("Third fraction : " + y); System.out.println("Forth fraction : " + z); System.out.println(); // Does the get() method work? System.out.println("*** Does the get() methods work?"); System.out.println("w = " + w.getNumerator() + " over " + w.getDenominator()); System.out.println();// Can we change the values correctly nameSystem.out.println("*** Does the set() methods work?");System.out.println("w fraction Before : " + w); w.setNumerator(22);System.out.println("w fraction After…arrow_forwardThe files provided in the code editor to the right contain syntax and/or logic errors. In each case, determine and fix the problem, remove all syntax and coding errors, and run the program to ensure it works properly. // This class uses a DebugBox class to instantiate two Box objects public class DebugFour3 { public static void main(String args[]) { int width = 12, length = 10, height = 8; DebugBox box1 = new DebugBox(); debugBox box2 = new DebugBox(width, length, height); System.out.println("The dimensions of the first box are"); box1.showData; System.out.print(" The volume of the first box is "); showVolume(box1); System.out.println(The dimensions of the second box are"); box2.showData(); System.out.print(" The volume of the second box is "); showVolume(box2); } public static void showVolume(DebugBox aBox) { double vol = aBox.getVolume(); System.out.println(volume); } }arrow_forwardin c++ codearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
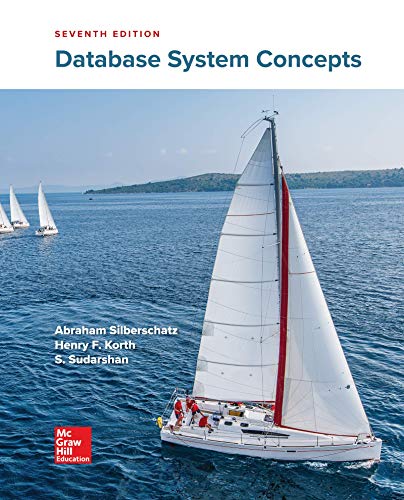
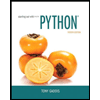
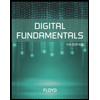
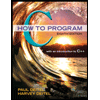
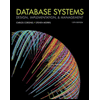
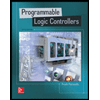