JAVA PROGRAM Lab #2. Chapter 7. PC #11. Array Operations (Page 491) Write a program that accepts a file name from command line, then initializes an array with test data using that text file as an input. The file should contain floating point numbers (use double data type). The program should also have the following methods: * getTotal. This method should accept a one-dimensional array as its argument and return the total of the values in the array. * getAverage. This method should accept a one-dimensional array as its argument and return the average of the values in the array. * getHighest. This method should accept a one-dimensional array as its argument and return the highest value in the array. * getLowest. This method should accept a one-dimensional array as its argument and return the lowest value in the array. double_input1.txt double_input2.txt PLEASE MODIFY THIS CODE, SO WHEN I UPLOAD IT TO HYPERGRADE IT PASSES ALL THE TEST CASES, BECAUSE WHEN I UPLOAD IT TO HYPERGRADE IT DOES NOT PASS THE TEST CASES. IT HAS TO PASS ALL THE TEST CASES. I PROVIDED THE CORRECT OUTPUT AS A SCREENSHOT AS A REFERRENCE. import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; public class Main { public static void main(String[] args) { if (args.length != 1) { System.out.println("Usage: java ArrayStatistics "); System.exit(1); } String fileName = args[0]; double[] data = readDataFromFile(fileName); if (data != null) { double total = getTotal(data); double average = getAverage(data); double highest = getHighest(data); double lowest = getLowest(data); System.out.println("Total: " + total); System.out.println("Average: " + average); System.out.println("Highest: " + highest); System.out.println("Lowest: " + lowest); } } public static double[] readDataFromFile(String fileName) { try (BufferedReader br = new BufferedReader(new FileReader(fileName))) { String line; String[] tokens; int count = 0; while ((line = br.readLine()) != null) { count++; } double[] data = new double[count]; int index = 0; br.close(); BufferedReader br1 = new BufferedReader(new FileReader(fileName)); while ((line = br1.readLine()) != null) { tokens = line.trim().split("\\s+"); if (tokens.length > 0) { data[index++] = Double.parseDouble(tokens[0]); } } return data; } catch (IOException e) { System.err.println("Error reading from file: " + e.getMessage()); return null; } catch (NumberFormatException e) { System.err.println("Invalid data format in the file: " + e.getMessage()); return null; } } public static double getTotal(double[] arr) { double total = 0; for (double num : arr) { total += num; } return total; } public static double getAverage(double[] arr) { if (arr.length == 0) { return 0; } double total = getTotal(arr); return total / arr.length; } public static double getHighest(double[] arr) { if (arr.length == 0) { return Double.NaN; } double highest = arr[0]; for (double num : arr) { if (num > highest) { highest = num; } } return highest; } public static double getLowest(double[] arr) { if (arr.length == 0) { return Double.NaN; } double lowest = arr[0]; for (double num : arr) { if (num < lowest) { lowest = num; } } return lowest; } }
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
if (args.length != 1) {
System.out.println("Usage: java ArrayStatistics <input_file>");
System.exit(1);
}
String fileName = args[0];
double[] data = readDataFromFile(fileName);
if (data != null) {
double total = getTotal(data);
double average = getAverage(data);
double highest = getHighest(data);
double lowest = getLowest(data);
System.out.println("Total: " + total);
System.out.println("Average: " + average);
System.out.println("Highest: " + highest);
System.out.println("Lowest: " + lowest);
}
}
public static double[] readDataFromFile(String fileName) {
try (BufferedReader br = new BufferedReader(new FileReader(fileName))) {
String line;
String[] tokens;
int count = 0;
while ((line = br.readLine()) != null) {
count++;
}
double[] data = new double[count];
int index = 0;
br.close();
BufferedReader br1 = new BufferedReader(new FileReader(fileName));
while ((line = br1.readLine()) != null) {
tokens = line.trim().split("\\s+");
if (tokens.length > 0) {
data[index++] = Double.parseDouble(tokens[0]);
}
}
return data;
} catch (IOException e) {
System.err.println("Error reading from file: " + e.getMessage());
return null;
} catch (NumberFormatException e) {
System.err.println("Invalid data format in the file: " + e.getMessage());
return null;
}
}
public static double getTotal(double[] arr) {
double total = 0;
for (double num : arr) {
total += num;
}
return total;
}
public static double getAverage(double[] arr) {
if (arr.length == 0) {
return 0;
}
double total = getTotal(arr);
return total / arr.length;
}
public static double getHighest(double[] arr) {
if (arr.length == 0) {
return Double.NaN;
}
double highest = arr[0];
for (double num : arr) {
if (num > highest) {
highest = num;
}
}
return highest;
}
public static double getLowest(double[] arr) {
if (arr.length == 0) {
return Double.NaN;
}
double lowest = arr[0];
for (double num : arr) {
if (num < lowest) {
lowest = num;
}
}
return lowest;
}
}
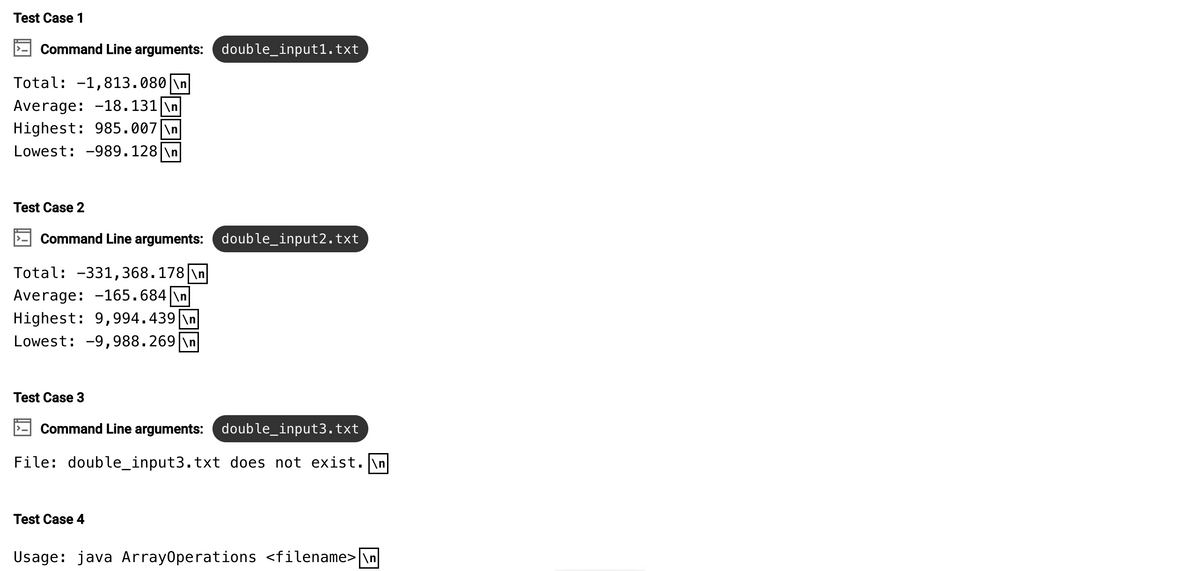
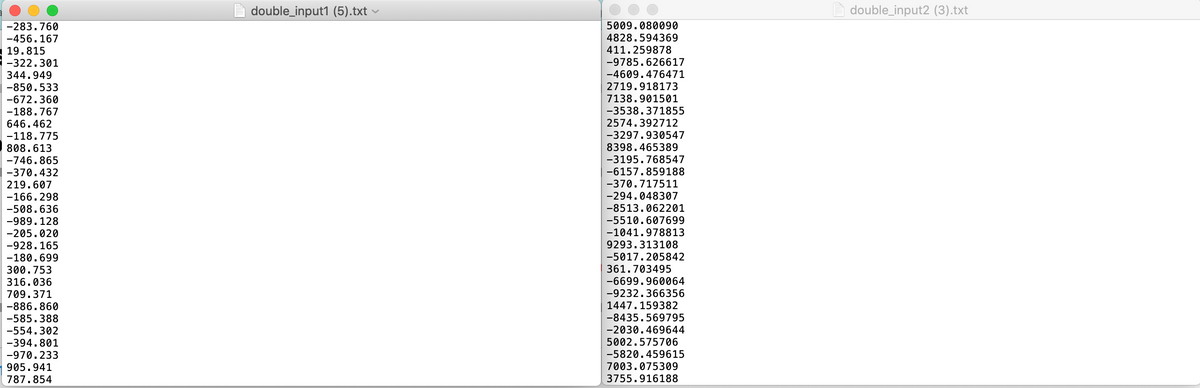

Step by step
Solved in 3 steps with 2 images

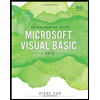
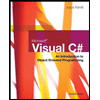
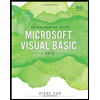
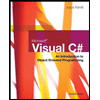