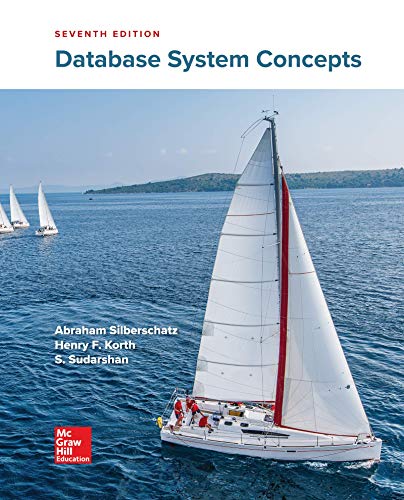
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
![Part 2: binary search. When binary searching a sorted array that contains more than one key equal to the search key, the client may want to know the index of either the first or the last such key.
Accordingly, implement the following API:
public class BinarySearchDeluxe {
// Returns the index of the first key in the sorted array a[]
// that is equal to the search key, or -1 if no such key.
public static <Key> int firstIndexof (Key[] a, Key key, Comparator<Key> comparator)
// Returns the index of the last key in the sorted array a[]
// that is equal to the search key, or -1 if no such key.
public static <Key> int lastIndexOf (Key[] a, Key key, Comparator<Key> comparator)
// unit testing (required)
public static void main (String [] args)
}
Corner cases. Throw an 1llegalArgumentException if any argument to either firstIndexOf() or lastIndexOf ( ) is null.
Preconditions. Assume that the argument array is in sorted order (with respect to the supplied comparator).
Unit testing. Your main () method must call each public method directly and help verify that they work as prescribed (e.g., by printing results to standard output).
Performance requirements. The firstIndexof () and lastIndexof () methods must make at most 1 + [log, n] compares in the worst case, where n is the length of the array. In this context, a
compare is one call to comparator.compare().](https://content.bartleby.com/qna-images/question/a76acd85-1038-470b-93a5-cc8f3268b5fe/0b68ba1b-4f90-4540-a55e-20285f0d3e90/83pdop5_thumbnail.png)
Transcribed Image Text:Part 2: binary search. When binary searching a sorted array that contains more than one key equal to the search key, the client may want to know the index of either the first or the last such key.
Accordingly, implement the following API:
public class BinarySearchDeluxe {
// Returns the index of the first key in the sorted array a[]
// that is equal to the search key, or -1 if no such key.
public static <Key> int firstIndexof (Key[] a, Key key, Comparator<Key> comparator)
// Returns the index of the last key in the sorted array a[]
// that is equal to the search key, or -1 if no such key.
public static <Key> int lastIndexOf (Key[] a, Key key, Comparator<Key> comparator)
// unit testing (required)
public static void main (String [] args)
}
Corner cases. Throw an 1llegalArgumentException if any argument to either firstIndexOf() or lastIndexOf ( ) is null.
Preconditions. Assume that the argument array is in sorted order (with respect to the supplied comparator).
Unit testing. Your main () method must call each public method directly and help verify that they work as prescribed (e.g., by printing results to standard output).
Performance requirements. The firstIndexof () and lastIndexof () methods must make at most 1 + [log, n] compares in the worst case, where n is the length of the array. In this context, a
compare is one call to comparator.compare().
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

Knowledge Booster
Similar questions
- I need some help making this code more simple. 2) AlgorithmtokenList = "{}()[]"initialize stack to emptyGet file name from user: fileNameOpen the file (fileName)read line from fileName into strLinewhile not EOF (end of file)find tokenList items in strLine for each token in strLineif token = "[" thenpush "]" into stackelse if token = "(" thenpush ")" into stackelse if token = "{" thenpush "}" into stackelse if token = "]" OR token = ")" OR token = "}" thenpop stack into poppedTokenif poppedToken not equal tokenprint "Incorrect Grouping Pairs"exitend ifend ifnext tokenend whileif stack is emptyprint "Correct Grouping Pairs"elseprint "Incorrect Grouping Pairs"end ifarrow_forwardPlease answer the below question for operating system using shell script. write a program in bash that has a user_defined function array_sum that takes each element of array as argument andcalculates their sum. At each step the sum will printed and then the final sum will be printed. For example:Input Array:[2 3 7 9]Output: 2 5 12 21final sum: 21arrow_forwardEvaluate the following functions: 1. prune [[[3],[1,2,4]],[[1,3],[3,4]]] 2. fix prune [[[5],[6,8]],[[7],[7,8]]] 3. blocked [[[3,4],[],[3]],[[1],[1,2],[1]]] 4. expand [[[1,2],[3]],[[4),[1,2]]arrow_forward
- fast please c++ Insert the elements of A in hash table H of size 10. H is a vector of int is size 10. Do not allow duplicates. Draw the table. The hash function is the number itself; f(n) = n%10arrow_forwardDesign a class that acquires the JSON string from question #1 and converts it to a class data member dictionary. Your class produces data sorted by key or value but not both. Provide searching by key capabilities to your class. Provide string functionality to convert the dictionary back into a JSON string. question #1: import requestsfrom bs4 import BeautifulSoupimport json class WebScraping: def __init__(self,url): self.url = url self.response = requests.get(self.url) self.soup = BeautifulSoup(self.response.text, 'html.parser') def extract_data(self): data = [] lines = self.response.text.splitlines()[57:] # Skip the first 57 lines for line in lines: if line.startswith('#'): # Skip comment lines continue values = line.split() row = { 'year': int(values[0]), 'month': int(values[1]), 'decimal_date': float(values[2]),…arrow_forwardMASM x86 classarrow_forward
- Mergesort is a method that takes an integer array and returns a sorted copy of the same array. It first sorts the left half of the input array, then the right half of the input array, and finally merges the two sorted halves together into a sorted version of the input array. Implement the mergesort method public static int[] mergesort(int[] arr) { return new int[] {-1}; } *must be in this layout and done in javaarrow_forward1-Write a JAVA program that reads an array from input file and invokes twodifferent methods Sort and Max ,that sorts the elements of the array and findsthe Max element and writes out the resulted array in to output file . Usetwo interfaces for methods and throw Exception Handling for Out Of Boundindex for the arrayarrow_forward1. Goals and Overview The goal of this assignment is to practice creating a hash table. This assignment also has an alternative, described in Section 5. A hash table stores values into a table (meaning an array) at a position determined by a hash function. 2. Hash Function and Values Every Java object has a method int hashCode() which returns an int suitable for use in a hash table. Most classes use the hashCode method they inherit from object, but classes such as string define their own hashCode method, overriding the one in object. For this assignments, your hash table stores values of type public class HashKeyValue { K key; V value; } 3. Hash Table using Chained Hashing Create a hash table class (called HashTable211.java) satisfying the following interface: public interface HashInterface { void add(K key, V value); // always succeeds as long as there is enough memory V find (K key); // returns null if there is no such key } Internally, your hash table is defined as a private…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
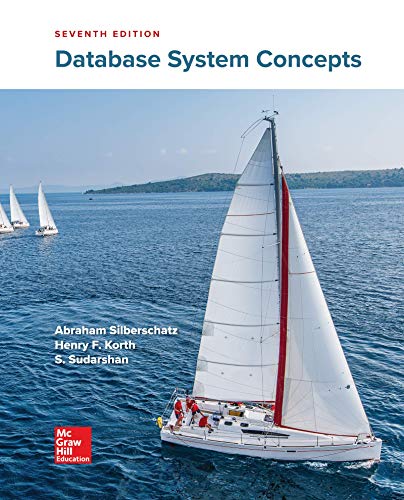
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
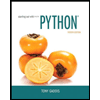
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
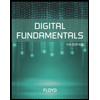
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
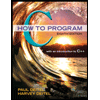
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
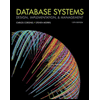
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
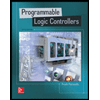
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education