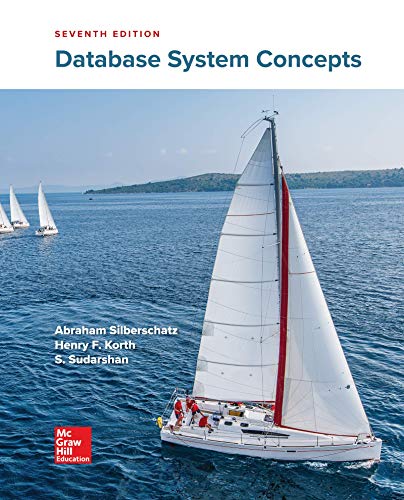
import java.util.Scanner;
public class PalindromeChecker {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print("Please enter a string to test for palindrome or type QUIT to exit:\n");
String input = scanner.nextLine().toLowerCase();
if (input.equals("quit")) {
break;
}
if (isPalindrome(input)) {
System.out.println("The input is a palindrome.");
} else {
System.out.println("The input is not a palindrome.");
}
}
}
private static boolean isPalindrome(String str) {
str = str.replaceAll("[^a-zA-Z0-9]", "");
int left = 0;
int right = str.length() - 1;
while (left < right) {
if (str.charAt(left) != str.charAt(right)) {
return false;
}
left++;
right--;
}
return true;
}
}
Test Case 1
Desserts, I stressedENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
KayakENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quitENTER
Test Case 2
dadENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quitENTER
Test Case 3
momENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
QuitENTER
Test Case 4
helloENTER
The input is not a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quiTENTER
b,a,dENTER
The input is not a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quitENTER
Test Case 6
Able was I, ere I saw ElbaENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quitENTER
Test Case 7
A man, a plan, a canal, PanamaENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quitENTER
Test Case 8
@abENTER
The input is not a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
ab@ENTER
The input is not a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
@aaENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
aa@ENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quitENTER
Test Case 9
abbaENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
abcaENTER
The input is not a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
aabcaaENTER
The input is not a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
abaceedabaENTER
The input is not a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quitENTER

Step by stepSolved in 3 steps with 9 images

- StringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forwardJava Questions - (Has 2 Parts). Based on each code, which answer out of the choices "A, B, C, D, E" is correct. Each question has one correct answer. Thank you. Part 1 - Given the following code, the output is __. class Sample{ public static void main (String[] args){ try{ System.out.println(5/0);}catch(ArithmeticException e){ System.out.print("Divide by zero????");}finally{ System.out.println("5/0"); } }} A. Divide by zero????B. 5/0C. Divide by zero????5/0D. 5/0Divide by zero????E. "5/0" Part 2 - Given the following code, the output is __. try{ Integer number = new Integer("1"); System.out.println("An Integer instance.");}catch (Exception e){ System.out.println("An Exception.");} A. An Integer instance.B. An Exception.C. 1D. Error: ExceptionE. None of the optionsarrow_forwardimport java.util.Scanner; public class LabProgram { /* Define your method here */ public static void main(String[] args) { Scanner scnr = new Scanner(System.in); /* Type your code here. */ }}arrow_forward
- import java.util.Scanner; public class AutoBidder { public static void main (String [] args) { Scanner scnr = new Scanner (System.in); char keepGoing; int nextBid; nextBid = 0; keepGoing 'y'; * Your solution goes here */ while { nextBid = nextBid + 3; System.out.println ("I'll bid $" + nextBid + "!") ; System.out.print ("Continue bidding? (y/n) "); keepGoing = scnr.next ().charAt (0); } System.out.println (""); }arrow_forwardJAVA Language: Transpose Rotate. Question: Modify Transpose.encode() so that it uses a rotation instead of a reversal. That is, a word like “hello” should be encoded as “ohell” with a rotation of one character. (Hint: use a loop to append the letters into a new string) import java.util.*; public class TestTranspose { public static void main(String[] args) { String plain = "this is the secret message"; // Here's the message Transpose transpose = new Transpose(); String secret = transpose.encrypt(plain); System.out.println("\n ********* Transpose Cipher Encryption *********"); System.out.println("PlainText: " + plain); // Display the results System.out.println("Encrypted: " + secret); System.out.println("Decrypted: " + transpose.decrypt(secret));// Decrypt } } abstract class Cipher { public String encrypt(String s) { StringBuffer result = new…arrow_forwardimport java.util.Scanner;public class main{public static void main(String[] args){Scanner sc = new Scanner(System.in):int year, day, weekday;String month;String outday = "";System.out.printf("Enter the month%n");month = sc.nextLine();System.out.printf("Enter the day%n");month = sc.nextInt();weekay = find_day(month, day);outday = out_weekday(weekday);System.out.printf("The day is: %s%n", outday);}} Change the program from the previous example to take command line arguments instead of using the Scanner. For example, running the program like this:> java OutDays March 14will output the weekday string (which is Thursday) on the console for March 14, 2019). public static void main(String[] args){ int year, day, weekday; String month; String outday = ""; // #### your code here for accessing the command line arguments weekday = find_day(month, day); outday = out_weekday(weekday); System.out.printf("The day is: %s%n", outday);}arrow_forward
- Convert the following java code to C++ //LabProgram.javaimport java.util.Scanner;public class LabProgram {public static void main(String[] args) {//defining a Scanner to read input from the userScanner input = new Scanner(System.in);//reading the value for Nint N = input.nextInt();//creating a 1xN matrixint[] m1 = new int[N];//creating an NxN matrixint[][] m2 = new int[N][N];//creating a 1xN matrix to store the resultint[] result = new int[N];//looping and reading N integers into m1for (int i = 0; i < N; i++) {m1[i] = input.nextInt();}//looping from 0 to N-1for (int i = 0; i < N; i++) {//looping from 0 to N-1for (int j = 0; j < N; j++) {//reading an integer and storing it into m2 at position i,jm2[i][j] = input.nextInt();}}//multiply m1 and m2, store result in result//looping from 0 to N-1for (int i = 0; i < N; i++) {//looping from 0 to N-1for (int j = 0; j < N; j++) {//multiplying value at index j in m1 with value at j,i in m2, adding to current value at index i// on…arrow_forwardimport javax.swing.JOptionPane; public class Addition{public static void main( String args[] ){for (int i = 0; i < 10; i++) {String Number = JOptionPane.showInputDialog( "Enter a number" );int number = Integer.parseInt( Number );int sum = number;JOptionPane.showMessageDialog( null, "The sum is " + sum,"Sum of 10 numbers", JOptionPane.PLAIN_MESSAGE );}}} I want to add the number that was entered but I don't know how to do that. Do you know how to add the entered number?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
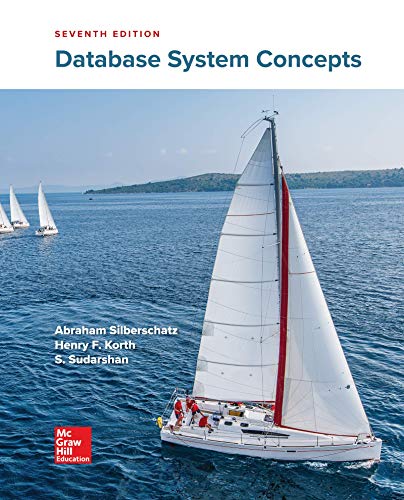
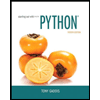
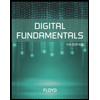
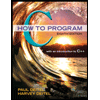
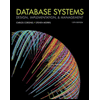
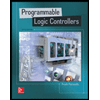