MyFrame Demo Left Middle - Right X Implement Java code in the constructor of MyFrame below to create a GUI like an image above. No event listeners and handlers are required. Class MyFrame extends JFrame { private JButton] buttons;
MyFrame Demo Left Middle - Right X Implement Java code in the constructor of MyFrame below to create a GUI like an image above. No event listeners and handlers are required. Class MyFrame extends JFrame { private JButton] buttons;
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
![MyFrame Demo
Left
Middle
public MyFrame() {
-
☐ X
Right
Implement Java code in the constructor of MyFrame below to create a GUI like an image above.
No event listeners and handlers are required.
Class MyFrame extends JFrame {
private JButton[] buttons;
super("MyFrame Demo");
//Add the implementation for the GUI here](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F03256047-c4d6-4acb-b3bb-18185f2c131a%2F5908976b-914d-4664-bd25-a5cf6230623d%2F729s9y8_processed.png&w=3840&q=75)
Transcribed Image Text:MyFrame Demo
Left
Middle
public MyFrame() {
-
☐ X
Right
Implement Java code in the constructor of MyFrame below to create a GUI like an image above.
No event listeners and handlers are required.
Class MyFrame extends JFrame {
private JButton[] buttons;
super("MyFrame Demo");
//Add the implementation for the GUI here
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Recommended textbooks for you
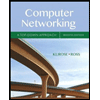
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
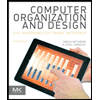
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
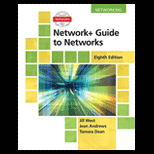
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
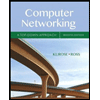
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
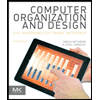
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
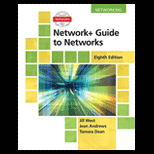
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
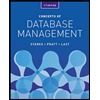
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
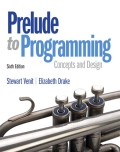
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
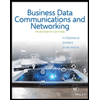
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY