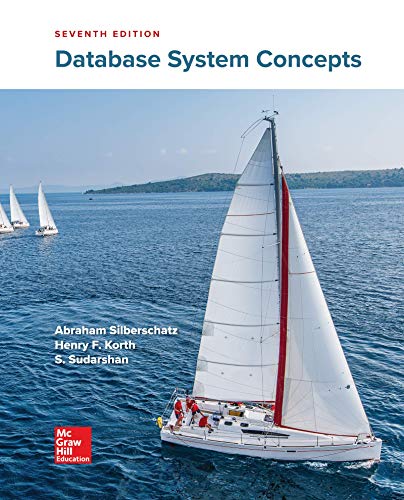
Concept explainers
You are to use the started code provided with QUEUE Container Adapter methods and provide the implementation of a requested functionality outlined below.
The following program has to be in c++, and have to use the already started code that is provided below.
Scenario:
A local restaurant has hired you to develop an application that will manage customer orders. Each order will be put in the queue and will be called on a first come first served bases.
Develop the menu driven application with the following menu items:
- Add order
- Next order
- Previous order
- Delete order
- Order Size
- View order list
- View current order
Order management will be resolved by utilization of an STL-queue container’s functionalities and use of the following Queue container adapter functions:
- enQueue: Adds the order in the queue
- DeQueue: Deletes the order from the queue
- Peek: Returns the order that is top in the queue without removing it
- IsEmpty: checks do we have any orders in the queue
- Size: returns the number of orders that are in the queue
While adding a new order in the queue, the program will be capable of collecting the following order information:
- Name on order
- Order description
- Order total
- Order tip
- Date of order
The started code that you have to use to implement the above requirements:
#include <iostream>
#include <queue>
#include <string>
using namespace std;
class patient {
/ /Creates class for patient variable
public:
string firstName;
string insuranceType;
string lastName;
string ssn;
string address;
string visitDate;
};
queue <patient> p;
void enqueue()
//Enqueues patient details from console input
{
patient pa;
cout << "Please enter Patient First Name:";
cin >> pa.firstName;
cout << "Please enter Patient Last Name:";
cin >> pa.lastName;
cout << "Please enter Patient insurance type:";
cin >> pa.insuranceType;
cout << "Please enter Patient SSN:";
cin >> pa.ssn;
cout << "Please enter Patient Address:";
cin.ignore();
getline(cin, pa.address);
cout << "Please enter Patient Date of Visit:";
cin >> pa.visitDate; p.push(pa);
}
patient dequeue()
//Removes patient from queue
{
patient pa;
if (!p.empty()) {
pa = p.front();
p.pop();
}
return pa;
}
void peek()
//Returns current patient at the front of the queue
{
if (!p.empty()) {
patient tmp = p.front();
cout << "Patient Name is " << tmp.firstName<< endl;
}
}
int main() {
int x = 0;
int n;
patient last;
while (x == 0) {
//Prompts for Menu Selection while selection = 0, which is defaulted to 0
cout << "Welcome to Dental Associates of Kansas City" << endl; cout << "**************************************************" << endl;
cout << "To get started, please select an option from the menu below:" << endl;
cout << "1. Add patient" << endl;
cout << "2. Next patient" << endl;
cout << "3. Previous patient" << endl;
cout << "4. Delete patient" << endl;
cout << "5. View current" << endl;
cout << "6. Exit this program" << endl; cout << "Enter the number of the action you would like to perform: ";
cin >> n; if (n == 1) {
enqueue();
} else if (n == 2) {
last = dequeue();
peek();
} else if (n == 3) {
cout << last.firstName << endl;
} else if (n == 4) {
last = dequeue();
} else if (n == 5) {
peek();
} else if (n == 6) {
exit(0);
}
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

- You are to use the started code provided with QUEUE Container Adapter methods and provide the implementation of a requested functionality outlined below. The program has to be in c++, and have to use the already started code below. Please make sure the code is able to add order, next order, previous order, delete order, order size, view order list, view current order, and exit program. Scenario: A local restaurant has hired you to develop an application that will manage customer orders. Each order will be put in the queue and will be called on a first come first served bases. Develop the menu driven application with the following menu items: Add order Next order Previous order Delete order Order Size View order list View current order Order management will be resolved by utilization of an STL-queue container’s functionalities and use of the following Queue container adapter functions: enQueue: Adds the order in the queue DeQueue: Deletes the order from the queue Peek:…arrow_forwardYou are working with a team creating a grocery shopping app. The app keeps the information of the items to buy in the list grocery list, and the items that were purchased in purchased list. You need to create a function that returns the items that were purchased, but that were not in the grocery list. For example, if the grocery list is milk, eggs, bacon, and flour; and we purchased milk, chocolate, and muffins, the function should return a list with chocolate and muffins. Drag and drop the expressions needed to implement the required function. def get_extra_items(grocery_list, purchased_list): list3 [] for i in range( ) : if not in list3.append( return list3 len(grocery_list) grocery_list grocery_list[i] purchased_list[i] purchased_list len(purchased_list)arrow_forwardThe code given below represents a saveTransaction() method which is used to save data to a database from the Java program. Given the classes in the image as well as an image of the screen which will call the function, modify the given code so that it loops through the items again, this time as it loops through you are to insert the data into the salesdetails table, note that the SalesNumber from the AUTO-INCREMENT field from above is to be inserted here with each record being placed into the salesdetails table. Finally, as you loop through the items the product table must be update because as products are sold the onhand field in the products table must be updated. When multiple tables are to be updated with related data, you should wrap it into a DMBS transaction. The schema for the database is also depicted. public class PosDAO { private Connection connection; public PosDAO(Connection connection) { this.connection = connection; } public void…arrow_forward
- In C++ language. Please look at the instructions and help with program. This is for intermediate not advanced. so help me understand pleasearrow_forwardElevator (or Lift as referred to by the English folks) Simulator in C language program.Simulate an Elevator-Controller Program. The Elevator should be able to travel between five floorsnamely Ground, 1st, 2nd, 3rd, 4th and 5th floors. The elevator can travel going up (unless they areat the 5th floor) or going down (unless they are at the ground floor). The elevator can only containone passenger (which is the current user of the program). Initially the elevator begins and loads atthe Ground Floor. If the user wants to travel to the 5th floor, the elevator shall go up to the saidfloor. It is the option of the user if they want to alight the elevator or not on the 5th floor or anyfloor on the way. Realistic rules should apply: If the user alights at the 4th floor, when they ride the elevator again, itshould begin traveling from the 4th floor and from there choose to go up or down. Your programshould have some validation: meaning, only the valid characters should be received by the…arrow_forwardLanguage is C++ Lab14A: The Architect. Buildings can be built in many ways. Usually, the architect of the building draws up maps and schematics of a building specifying the building’s characteristics such as how tall it is, how many stories it has etc. Then the actual building itself is built based on the schematics (also known as blueprints). Now it is safe to assume that the actual building is based off the blueprint but is not the blueprint itself and vice versa. The idea of a classes and objects follows a similar ideology. The class file can be considered the blueprint and the object is the building following the analogy mentioned above. The class file contains the details of the object i.e., the object’s attributes (variables) and behavior (methods). Please keep in mind that a class is a template of an eventual object. Although the class has variables, these variables lack an assigned value since each object will have a unique value for that variable. Think of a form that you…arrow_forward
- Write your program in C# with Visual Studio, or an online compiler - compile, and execute it. Upload the file with the .cs extension to Canvas for grading. Canvas will not accept any other file type. • There will be a 10% deduction for each weekly last submission. You only get a maximum of two weeks to make up for any late work; Ex: late two weeks = 20% deductions Programming Assignment 3: Chapter 4 Write, compile, and test a program called Credit Test. Write a program that asks a user to enter their Credit Score and Down payment for a loan approval process. Display credit accepted message if the user meets either of the following requirements: A minimum credit score of 675, and a down payment of $35000 or above. A credit score below 675, and a down payment of 85000 and above. A credit rejected message should display if the user doesn't meet any of the qualification criteria.arrow_forwardUse the started code provided with QUEUE Container Adapter methods and provide the implementation of a requested functionality outlined below. This program has to be in c++, and have to use the already started code below. Scenario: A local restaurant has hired you to develop an application that will manage customer orders. Each order will be put in the queue and will be called on a first come first served bases. Develop the menu driven application with the following menu items: Add order Next order Previous order Delete order Order Size View order list View current order Order management will be resolved by utilization of an STL-queue container’s functionalities and use of the following Queue container adapter functions: enQueue: Adds the order in the queue DeQueue: Deletes the order from the queue Peek: Returns the order that is top in the queue without removing it IsEmpty: checks do we have any orders in the queue Size: returns the number of orders that are in the queue…arrow_forwardYou are to use the started code provided with QUEUE Container Adapter methods and provide the implementation of a requested functionality outlined below. The program has to be in c++, and have to use the already started code below. Scenario: A local restaurant has hired you to develop an application that will manage customer orders. Each order will be put in the queue and will be called on a first come first served bases. Develop the menu driven application with the following menu items: Add order Next order Previous order Delete order Order Size View order list View current order Order management will be resolved by utilization of an STL-queue container’s functionalities and use of the following Queue container adapter functions: enQueue: Adds the order in the queue DeQueue: Deletes the order from the queue Peek: Returns the order that is top in the queue without removing it IsEmpty: checks do we have any orders in the queue Size: returns the number of orders that are in…arrow_forward
- In C++ Create a new project named lab8_1. You will be implementing two classes: A Book class, and a Bookshelf class. The Bookshelf has a Book object (actually 3 of them). You will be able to choose what Book to place in each Bookshelf. Here are their UML diagrams Book class UML Book - author : string- title : string- id : int- count : static int + Book()+ Book(string, string)+ setAuthor(string) : void+ setTitle(string) : void+ print() : void+ setID() : void And the Bookshelf class UML Bookshelf - book1 : Book- book2 : Book- book3 : Book + Bookshelf()+ Bookshelf(Book, Book, Book)+ setBook1(Book) : void+ setBook2(Book) : void+ setBook3(Book) : void+ print() : void Some additional information: The Book class also has two constructors, which again offer the option of declaring a Book object with an author and title, or using the default constructor to set the author and title later, via the setters . The Book class will have a static member variable…arrow_forwardin c++ codearrow_forwardCreate a new C++ project in Visual Studio (or an IDE of your choice). Add the files listed below to your project: BinaryTree.h Download BinaryTree.h BinarySearchTree.h Download BinarySearchTree.h main.cpp Download main.cpp Build and run your project. The program should compile without any errors. Note that function main() creates a new BST of int and inserts the nodes 12, 38, 25, 5, 15, 8, 55 (in that order) and displays them using pre-order traversal. Verify that the insertions are done correctly. You may verify it manually or use the visualization tool at https://www.cs.usfca.edu/~galles/visualization/BST.html. (Links to an external site.) Do the following: 1. add code to test the deleteNode and search member functions. Save screenshot of your test program run. 2. add code to implement the nodeCount member function of class BinaryTree. Test it and save screenshot of your test program run. 3. add code to implement the leafCount member function of class BinaryTree. Test it and…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
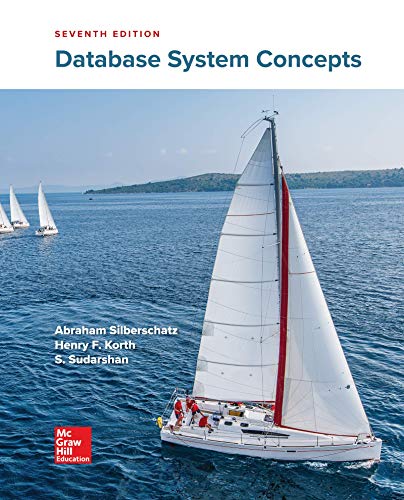
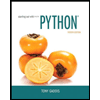
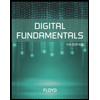
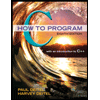
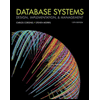
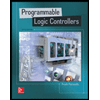