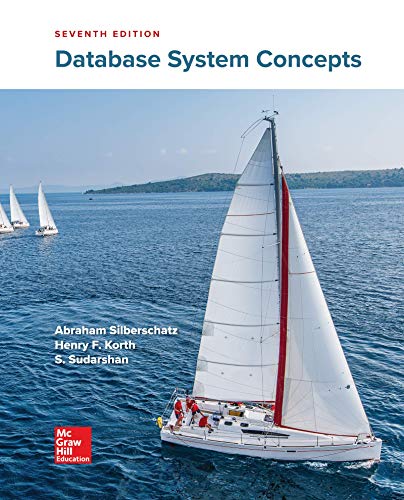
please can you help fix my code
TypeError: 'NoneType' object is not callable
import timeit
import numpy as np
#Defining input as a Numpy array of positive Integers
x = np.array([1, 2, 3, 4])
#defining function to calculate cube of an element
def cube_array(x):
return np.power(x,3) #function using unfunc power
#test function
cube_x = cube_array(x)
#calling function
print("Numpy array of intergers are: ", *x, sep= " ")
print('\n')
print("Cube of array elements is: ", *cube_x, sep=" ")
print('\n')
import math as m
#Defining input as a sequence range of positive integer numbers
i = range(1,5)
#defining function to calculate cube of an element
def cube_pow(x):
cubeA=[pow(x, 3) for x in i] #function using math.pow
return cubeA
#test function
cube_A=cube_pow(x)
#calling function
print("Sequence of intergers are: ", *i, sep=" ")
print('\n')
print("Cube of elements in the range is: ", *cube_A, sep=" ")
print('\n')
print("Time taken to execute cube_array(x) is: ")
array= %timeit cube_array(x)
print('\n')
print("Time taken to execute cube_pow(x) is: ")
pow=%timeit cube_pow(x)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- write this using java script arrow functions -thank you :)arrow_forwardc++ language using addition, not get_sum function with this pseudocode: Function Main Declare Integer Array ages [ ] Declare integer total assign numbers = [ ] assign total = sum(ages) output "Sum: " & total end fucntion sum(Integer Array array) declare integer total declare integer index assign total = 0 for index = 0 to size(array) -1 assign total = total + array[ ] end return integer totalarrow_forwardProgramming language: Processing from Java Question attached as photo Topic: Use of Patial- Full Arraysarrow_forward
- //Required Functionvoid func(int array_1[],int array_2[],int size){ for(int i=0;i<size;i++){ if(array_2[i]>array_1[i]){ array_1[i]=array_2[i]; } } return;} //main function to test the function.int main(){ int size=6; int a[]={1,5,9,8,7,6}; int b[]={1,4,10,12,7,15}; printf("First array before function call: "); for(int i=0;i<size;i++){ printf("%d ",a[i]); } func(a,b,size); //call to the created function printf("\nFirst array after function call: "); for(int i=0;i<size;i++){ printf("%d ",a[i]); } return 0;} 1. Write the statements to do the following: write a prototype for your function in the previous problem write a main function which includes the following steps declare two arrays of ints with 25 elements each prompt the user and read values into both arrays call your function from the previous problem print both arraysarrow_forward1- Write a user-defined function that accepts an array of integers. The function should generate the percentage each value in the array is of the total of all array values. Store the % value in another array. That array should also be declared as a formal parameter of the function. 2- In the main function, create a prompt that asks the user for inputs to the array. Ask the user to enter up to 20 values, and type -1 when done. (-1 is the sentinel value). It marks the end of the array. A user can put in any number of variables up to 20 (20 is the size of the array, it could be partially filled). 3- Display a table like the following example, showing each data value and what percentage each value is of the total of all array values. Do this by invoking the function in part 1.arrow_forward1. Display Function Implement a function to display the contents of the patient_list array. Add code to call this function. Note that there are multiple places where this function needs to be called. Look for the // TODO comments to find the correct locations. 2. Sorting the array by Age Implement the code to sort the contents of the patient_list array based on the value stored in the age field. To do this you will need to implement code that relies on the qsort function from the C Standard library A function that compares two patient elements, based on the value stored in the age field. A call to the qsort function, which includes the array to be sorted, the number of elements in the array, the size of each array element and the function used to compare the array elements. Add code to call the qsort function, using the age comparison function that you implemented. This code should be placed just under the appropriate // TODO comment in main(). Sorting the array by…arrow_forward
- Prompt: In Python language, write a function that applies the logistic sigmoid function to all elements of a NumPy array. Code: import numpy as np import math import matplotlib.pyplot as plt import pandas as pd def sigmoid(inputArray): modifiedArray = np.zeros(len(inputArray)) #YOUR CODE HERE: return(modifiedArray) def test(): inputs = np.arange(-100, 100, 0.5) outputs = sigmoid(inputs) plt.figure(1) plt.plot(inputs) plt.title('Input') plt.xlabel('Index') plt.ylabel('Value') plt.show() plt.figure(2) plt.plot(outputs,'Black') plt.title('Output') plt.xlabel('Index') plt.ylabel('Value') plt.show() test()arrow_forwardC++arrow_forward/* (name header) #include // Function declaration int main() { int al[] int a2[] = { 7, 2, 10, 9 }; int a3[] = { 2, 10, 7, 2 }; int a4[] = { 2, 10 }; int a5[] = { 10, 2 }; int a6[] = { 2, 3 }; int a7[] = { 2, 2 }; int a8[] = { 2 }; int a9[] % { 5, 1, б, 1, 9, 9 }; int al0[] = { 7, 6, 8, 5 }; int all[] = { 7, 7, 6, 8, 5, 5, 6 }; int al2[] = { 10, 0 }; { 10, 3, 5, 6 }; %3D std::cout « ((difference (al, 4) « ((difference(a2, 4) <« ((difference (a3, 4) « ((difference(a4, 2) <« ((difference (a5, 2) <« ((difference (a6, 2) <« ((difference (a7, 2) <« ((difference(a8, 1) « ((difference (a9, 6) « ((difference(a10, 4) " <« ((difference(al1, 7) « ((difference (al2, 2) == 10) ? "OK\n" : "X\n"); << "al: << "a2: %3D 7)? "OK" : "X") << std::endl == 8) ? "OK\n" : "X\n") 8) ? "OK\n" : "X\n") == 8) ? "OK\n" : "X\n") == 8) ? "OK\n" : "X\n") == 1) ? "OK\n" : "X\n") == 0) ? "OK\n" : "X\n") 0) ? "OK\n" : "X\n") == 8) ? "OK\n" : "X\n") == 3) ? "OK\n" : "X\n") == 3) ? "OK\n" : "X\n") == << "a3: == << "a4: <<…arrow_forward
- use c++ Programming language Write a program that creates a two dimensional array initialized with test data. Use any data type you wish . The program should have following functions: .getAverage: This function should accept a two dimensional array as its argument and return the average of each row (each student have their average) and each column (class test average) all the values in the array. .getRowTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The function should return the total of the values in the specified row. .getColumnTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a column in the array. The function should return the total of the values in the specified column. .getHighestInRow: This function should accept a two…arrow_forwardc++ programming Write a function named maxCols which stores the maximum value in each row of a 3x3 2D array into a 1D array. This function should accept a 3x3 2D array, a 1D array and the size of each dimension as parameters. Example Calling maxCols with this 2D array:3 7 2 9 3 6 8 5 6 Results in the 1D array storing the values 7,9,8.arrow_forwardCreate the following functions makeBoard Accepts two parameters, integers mm and nn, and creates an mm x nn numpy array of zeros Returns the created array makeMove Accepts two parameters, a 2D array (such as created by makeBoard), and an integer xx Checks if the value at position xx in the array is 0. If it is, changes it to 1 and returns True If it isn't, does not change it and returns False Position xx in the array is given by reading the array top-down and left-to right. A simple 2x3 array is seen below with each value representing its position You may assume without checking that the value of xx provided is valid, that is it is within the array. Hint: Arrays have a property you can use to determine their shape. Hint: This function may (and indeed should) contain if statements, but no loops. Sample array with positions: [[0 1 2][ 3 4 5]] For testing purposes, you may copy the function provided here into your code def playGame(mm,nn): board = makeBoard(mm,nn)…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
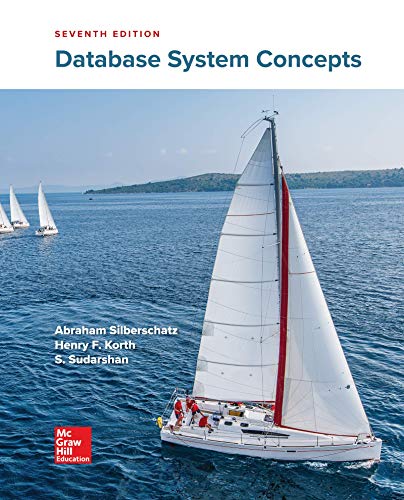
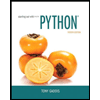
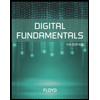
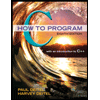
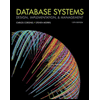
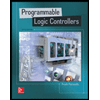