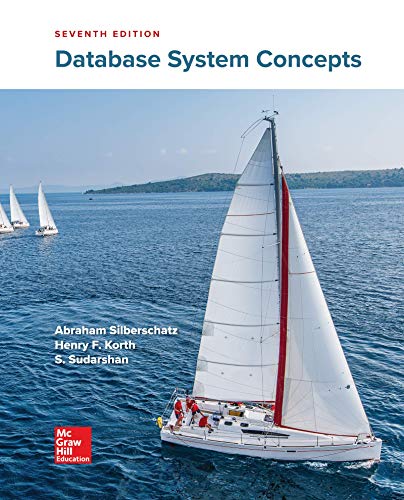
Please finish this problem in OCaml.
Please implement two functions:
string_explode : string -> char list
string_implode : char list -> string
string_explode turns a string into a list of characters and string_implode turns a list of characters back into a string.
To implement these two functions, use a selection of the following higher-order functions: List.map, List.fold_right, List.fold_left and tabulate. tabulate is implemented for you in the prelude.
You may also find the following functions from the OCaml string and char libraries useful:
String.get : string -> int -> char returns the character at index n in string s.
String.length : string -> int returns the length (number of characters) of the given string.
Char.escaped : char -> string returns the string representation of the given character
In order to get full marks for each question, you must use higher-order functions. Solutions using manual recursion will be capped at half marks.
Please follow the follwing format:
let string_explode (s : string) : char list =
raise NotImplemented
let string_implode (l : char list) : string =
raise NotImplemented
tabulate is defined as follow:
let rec tabulate f n =
let rec tab n acc =
if n < 0 then acc
else tab (n - 1) ((f n) :: acc)
in
tab (n - 1) []

Step by stepSolved in 3 steps

- Python Complete the get_2_dimensional_list() function that takes two parameters: 1. An integer num_rows. 2. An integer num_columns. The function should return a 2D list with num_rows rows and num_columns columns. Each element is set to a random value between 1 and 99 included. If both parameters num_rows and num_columns are set 0, the function returns an empty list. You MUST use a for...in.range loop to create the 2D list. You do not need to import the random module as it has already been done for you. Some examples of the function being called are shown below. For example: Test random.seed (15) num_rows - 2 num columns 5 matrix = get_2_dimensional_list(num_rows, num_columns) print(matrix) random.seed (132) num_rows=2 num_columns 2 matrix = get_2_dimensional_list(num_rows, num_columns) print(matrix) Result [[27, 2, 67, 95, 5], [21, 31, 3, 8, 88]] [[53, 20], [53, 8]]arrow_forwardin #lang schemearrow_forwardIn python pleasearrow_forward
- Write a function that concatenates all the strings in a list. The concatenation of all the strings in an empty list is the empty string "". please use Ocaml for the code and make sure the code is completearrow_forwardWrite a function in_list that will take as its parameters an input list called mylist and a number. If the number is in the list the function should retun true, ottherwise, it should retun false. def in_list(mylist,number):arrow_forwardWrite a function called find_duplicates which accepts one list as a parameter. This function needs to sort through the list passed to it and find values that are duplicated in the list. The duplicated values should be compiled into another list which the function will return. No matter how many times a word is duplicated, it should only be added once to the duplicates list. NB: Only write the function. Do not call it. For example: Test Result random_words = ("remember","snakes","nappy","rough","dusty","judicious","brainy","shop","light","straw","quickest", "adventurous","yielding","grandiose","replace","fat","wipe","happy","brainy","shop","light","straw", "quickest","adventurous","yielding","grandiose","motion","gaudy","precede","medical","park","flowers", "noiseless","blade","hanging","whistle","event","slip") print(find_duplicates(sorted(random_words))) ['adventurous', 'brainy', 'grandiose', 'light', 'quickest', 'shop', 'straw', 'yielding']…arrow_forward
- 1.Given the argument passed to the function is a list containing integer values, what does the function return def mystery1(someListOfValues: list):total = 0for value in someListOfValues:total += valuereturn total/len(someListOfValues) 2. The function below is to find the largest value in a list passed by parameter. Does it work as intened (yes or no)? If not give an example of an argument it would not work for. def maxValue(someListOfValues:list):"""returns the largest value the list passed by parameter"""value = 0for num in someListOfValues:if num > value:value = numreturn value 3.Write a function called loadNames() that will open a file called names.txt and build a list of its contents and return it. Function Call: names = loadNames()print(names) Sample ouput: ['Nolan Rafaj\n', 'Brandon Brzuszkiewicz\n', 'Amanda Vozari\n', 'Chase Harper\n', 'Bryce Conner']arrow_forwardPYTHON!!! Write a function that will filter course grades that are stored in a list, creating and returning a new list. Your implementation must have the following rules: - The function must accept two (and only two) parameters: The first is: the maximum integer value for any grade to remain in the list (any value above the maximum will be filtered and removed from the list). The second is: the list of grades (you can assume the list contains only integer values) - The function must return a new list and not modify the existing list. - The function must use a list comprehension to filter the existing list and create the new list that is returned. - DO NOT USE THE: built-in-"filter" function.arrow_forwardComplete the reverse_list() function that returns a new string list containing all contents in the parameter but in reverse order. Ex: If the input list is: ['a', 'b', 'c'] then the returned list will be: ['c', 'b', 'a'] Note: Use a for loop. DO NOT use reverse() or reversed(). This is my code so far: def reverse_list(letters): for x in (letters): letters.sort(reverse=True) return lettersif __name__ == '__main__': ch = ['a', 'b', 'c'] print(reverse_list(ch)) # Should print ['c', 'b', 'a'] When submitting I get 4/10 and it says: Test input list ['a', 'b', 'c'] returns ['c', 'b', 'a'] Your output reverse_list() function reversed array incorrectly: ['c', 'b', 'a'] reverse_list() function should have returned: ['a', 'b', 'c'] Test input list ['a', 'b', 'c', 'd'], first element of returned list is ['d'] Your output First character of returned array should be a instead of d Test empty input array TypeError: object of type 'NoneType' has no len()arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
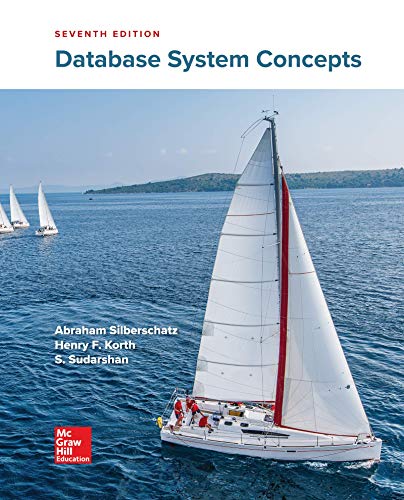
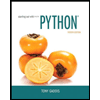
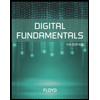
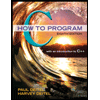
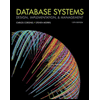
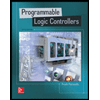