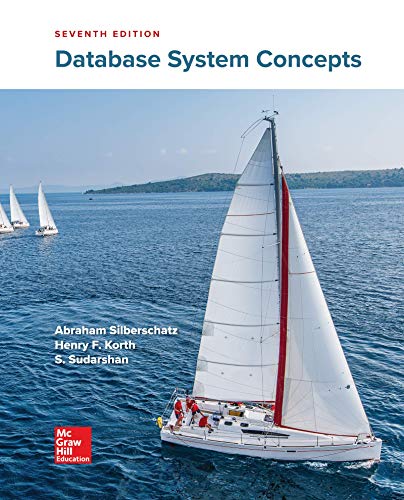
Please help me with this using java:
/**
* Implement the method below, which takes a two-dimensional array of integers
* as the input argument and returns the total number of <strong>distinct
* pairs</strong> (a, b) where both a and b are even numbers and a is less than
* b ({@code a < b} ). Your code should use nested for loops.
*
* <p>
* For example:
* </p>
*
* <pre>
* if inputarray = { {9, 10, 3, 4, 3}, {1, 6, 2}, {4, 24, 46, 0}, {15, 9, 45, 57} } then return 21
* note we have 21 distinct pairs within this 2D array as follows
* [0, 2],[0, 4],[0, 6],[0, 10],[0, 24],[0, 46],[2, 4],[2, 6],
* [2, 10],[2, 24],[2, 46],[4, 6],[4, 10],[4, 24],[4, 46],[6, 10],
* [6, 24],[6, 46],[10, 24],[10, 46],[24, 46]
* if inputarray = { {1, 6, 1}, {5, 9, 15, 20}, {23, 33, 99} } then return 1
* if inputarray = { {4, 21, 43, 1}, {22, 32, 42, 52} } then return 10
* if inputarray = { {15, 9, 45, 57}, {13, 17, 23} }then return 0
* </pre>
*
* @param inputarray 2D int input array
* @return the number of distinct pairs (a, b) where both a and b are even
* numbers and {@code a < b}.
*/
publicstaticintcountEvenPairs2D(int[][]inputarray){
/*
* Your implementation of this method starts here. Recall that : 1. No
* System.out.println statements should appear here. Instead, you need to return
* the result. 2. No Scanner operations should appear here (e.g.,
* input.nextInt()). Instead, refer to the input parameters of this method.
*/

Step by stepSolved in 2 steps with 1 images

- assignment is to complete the starter code:arrow_forwardPLEASE TYPE ONLY*** JAVA PROGRAMMING Task: a program called ArrayList_Practice. In this program, please do the following operations. Complete a method called RedundantCharacterMatch(ArrayList<Character> YourFirstName): the parameter of this method is an ArrayList<Character> whose elements are the characters in your first name (they should be in the order appear in your first name, e.g., if your first name is bob, then the ArrayList<Char> includes ‘b’, ‘o’, ‘b’.). The method will check whether there exists duplicate characters in your name and return the index of those duplicate characters. For example, when using bob as first name, it will return b: 0, 2. Create ArrayList<Character> NameExample. All the characters of your first name will appear twice in this ArrayList. For example, if your first name is bob, then NameExample will include the following element {b,o,b,b,o,b}. Then, please use NameExample as parameter for the method RedundantCharacterMatch().…arrow_forwardPlease write it in c# program. Problem: BigNumberInteger numbers in programming limit how large numbers can be processed, so double types come tothe rescue. Sometimes we need to implement bigger values. Create a class BigNumber that uses a 40-element array of digits to store integers as large as 40 digits each. Provide methods Input, ToString, Addand Subtract. For comparing BigNumber objects, provide the following methods: IsEqualTo,IsNotEqualTo, IsGreaterThan, IsLessThan, IsGreaterThanOrEqualTo and IsLessThanOrEqualTo. Each ofthese is a method that returns true if the relationship holds between the two BigNumber objects andreturns false if the relationship does not hold. Provide method IsZero. In the Input method, use thestring method ToCharArray to convert the input string into an array of characters, then iterate throughthese characters to create your BigNumber. (Add Multiply and Divide methods For extra five points innext exam)Hint: use char –‘0’ to convert character to…arrow_forward
- The code in the picture takes two sorted arrays of sizes m and n, then it merges and sorts them. Whats the best-case and worst-case for this alogrithm?arrow_forwardpublic static void reverse(ArrayList<Integer> alist){}public static ArrayList<Integer> reverseList(ArrayList<Integer> alist){} How to use these two headers method and a main method to create the output of [1,2,3] [3,2,1]arrow_forwardPlease help with the following in JAVA: Question 1 Could you minimize the total number of operations done for the previous problem? Previous Question > Given an integer list nums, move all 0's to the end of it while maintaining the relative order of the non-zero elements. Note that you must do this in-place without making a copy of the array. Code public static void main(String[] args) {ArrayList<Integer> mylist = new ArrayList<Integer>();Scanner sc = new Scanner(System.in);System.out.println("Enter the list with last a add : ");while (sc.hasNextInt()) {//TAKING INPUT UNTILL THE USER ENTERS ANY CHARACTER OTHER THAN INTint i = sc.nextInt();mylist.add(i);}int count=0;for(int i=0;i<mylist.size();i++){if(mylist.get(i)==0){count++; //COUNTING NUMBER OF ZEROES}}while(count!=0){for(int i=0;i<mylist.size()-1;i++){if(mylist.get(i)==0){Collections.swap(mylist, i, i+1);}}count--;}for(int i=0;i<mylist.size();i++){System.out.print(mylist.get(i)+ " "); //PRINTING THE…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
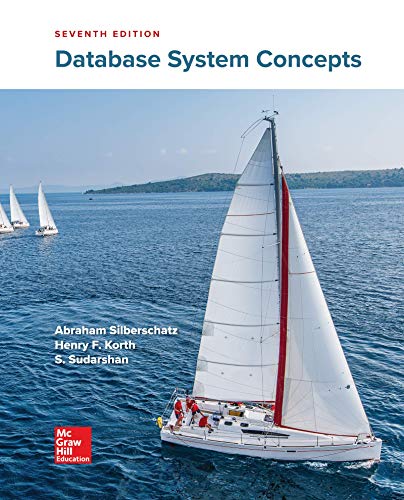
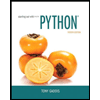
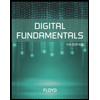
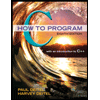
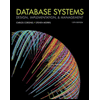
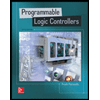