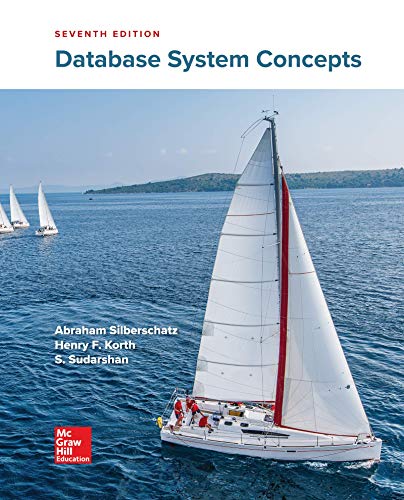
Please I need to complete the requirements in the code
import java.util.Scanner;
public class Palindrome {
public static void main(String[] args) {
String readUserSequence;
int Lenght =20;
boolean flag=true;
Scanner sc=new Scanner(System.in);
System.out.println("Please enter the Sequence of numbers:");
readUserSequence=sc.nextLine();
int len=readUserSequence.length();
for(int i=0;i<len/2;i++)
{
char f=readUserSequence.charAt(i);
char e=readUserSequence.charAt(len-i-1);
if(f!=e)
{
flag=false;
}
}
if(flag==true)
{
System.out.println("Your Sequence is palindrome");
}
else
{
System.out.println("Your Sequence is not palindrome");
}
}
}
Method displayArray(int[] arrayToDisplay)
This method accepts an array and displays it.
Write a test program that do the following:
- Create two arrays of size 30.
- Call readUserSequence(…) method to read data from the user and fill the arrays
- Call displayArray(…)to print the arrays.
- Pass the arrays to the isPalindrome(…) If the user’s sequence is palindrome then display "Your array is palindrome.", if not then display "Your array is not palindrome."
the sample output
Please enter the 5 elements of the first array:
2
4
0
4
2
Your array is: [2, 4, 0, 4, 2]
Your array is palindrome!
Please enter the 5 elements of the second array:
6
2
0
6
2
Your array is: [6, 2, 0, 6, 2]
Your array is not palindrome!

Step by stepSolved in 3 steps with 5 images

- I have this java code " import java.util.Scanner;public class Main3 {public static void main(String[] args) {// Create Scanner input;Scanner scanner = new Scanner(System.in);boolean Continue = true;while (!Continue) {}// Defining variables with input parametersSystem.out.print("Enter the Loan amount: ");double loanAmount = scanner.nextDouble();System.out.print("Enter the number of years: ");int n = scanner.nextInt();System.out.print("Enter the annual interest rate: ");float a = scanner.nextFloat();// Equations for Variables// Calculate monthly interest ratefloat i = a / 12;// Calculate total monthsint m = n * 12;// Calculate payment monthlyfloat monthlyPayment = (float) ((loanAmount * i * Math.pow(1 + i, n)) / (Math.pow(1 + i, n) - 1));// Calculate Total Paymentfloat totalPayment = monthlyPayment * 12;// Printing the results for VariablesSystem.out.printf("Monthly payment is $%.2f\n", monthlyPayment);System.out.printf("Total payment is $%.2f\n", totalPayment);}}"How do I turn single…arrow_forwardimport java.util.Scanner; public class DebugSix4 { public static void main(String[] args) { int high, low, count = 0; final int NUM = 5; Scanner input = new Scanner(System.in); System.out.print("This application displays " + NUM + " random numbers" + "\nbetween the low and high values you enter" + "\nEnter low value now... "); low = input.nextInt(); // Inserted the missing semicolon System.out.print("Enter high value... "); high = input.nextInt(); // Added the missing dot to the method call. while (low >= high) { // For proper input validation, changed "" to ">=" System.out.println("The number you entered for a high number, " + high + ", is not more than " + low); System.out.print("Enter a number higher than " + low + "... "); high =…arrow_forwardHelp me fix the code make it run out like the EXPEarrow_forward
- Complete the code and make it run sucessful by fixing errors//MainValidatorA3 public class MainA3 { public static void main(String[] args) { System.out.println("Welcome to the Validation Tester application"); // Int Test System.out.println("Int Test"); ValidatorNumeric intValidator = new ValidatorNumeric("Enter an integer between -100 and 100: ", -100, 100); int num = intValidator.getIntWithinRange(); System.out.println("You entered: " + num + "\n"); // Double Test System.out.println("Double Test"); ValidatorNumeric doubleValidator = new ValidatorNumeric("Enter a double value: "); double dbl = doubleValidator.getDoubleWithinRange(); System.out.println("You entered: " + dbl + "\n"); // Required String Test System.out.println("Required String Test:"); ValidatorString stringValidator = new ValidatorString("Enter a required string: "); String requiredString =…arrow_forwardGiven string inputStr on one line and integers idx1 and idx2 on a second line, output "Match found" if the character at index idx1 of inputStr is equal to the character at index idx2. Otherwise, output "Match not found". End with a newline. Ex: If the input is: eerie 4 1 then the output is: Match found Note: Assume the length of string inputStr is greater than or equal to both idx1 and idx2.arrow_forwardimport java.util.Scanner; public class RomanNumerals { public static void main(String[] args) { Scanner in = new Scanner("I C X D M L"); char romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 1") ; romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 100") ; romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 10") ; romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 500") ; romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 1000") ; romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 50") ; } /** Gives the value…arrow_forward
- import java.util.Scanner; public class CircleAndSphereWhileLoop{ public static final double MAX_RADIUS = 500.0; public static void main(String[] args) { Scanner in = new Scanner(System.in); // Step 2: Read a double value as radius using prompt // "Enter the radius (between 0.0 and 500.0, exclusive): " // Step 3: While the input radius is not in the ragne (0.0, 500.0) // Display a message on one line (ssuming input value -1) // "The input number -1.00 is out of range." // Read a double value as radius using the same promt double circumference = 2 * Math.PI * radius; double area = Math.PI * radius * radius; double surfaceArea = 4 * Math.PI * Math.pow(radius, 2); double volume = (4 / 3.0) * Math.PI * Math.pow(radius, 3); // Step 4: Display the radius, circle circumference, circle area, // sphere surface area, and…arrow_forwardimport java.util.Scanner;import java.util.InputMismatchException; public class LabProgram { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); /* Type your code here. */ }}arrow_forwardJava - How can I output the following statement as a single line with a newline? Program below. import java.util.Scanner; public class LabProgram { public static void main(String[] args) { Scanner sc = new Scanner(System.in); int n = sc.nextInt(); if(n>=11 && n<=100) { while(n>=11 && n<=100) { System.out.print(n+ " "); (the problem line. When I use System.out.println, it makes the line vertical when I want it horizontal.) if(n ==11||n==22||n==33||n==44||n==55||n==66||n==77||n==88||n==99) break; n--; } } else System.out.println("Input must be 11-100"); }}arrow_forward
- 1. What is the output of the following program? import java.util.Scanner; public class Factorial { public static void main(String args[]){ Scanner scanner = new Scanner(System.in); System.out.printIn("Enter the number:"); scanner.nextInt(); int factorial = fact(num); System.out.println("Factorial of int num = entered number is: "+factorial); } static int fact(int n) { int output; if(n==1){ return 1; } output = fact(n-1)* n; return output; } }arrow_forwardUsing Java.arrow_forwardJava - How to make this a horizontal statement with a newline. Whenever I use System.out.println, it makes the output vertical instead of horizontal. What am I doing wrong? Program below. import java.util.Scanner; public class LabProgram { public static void main(String[] args) { int i; String InputString; Scanner sc = new Scanner(System.in); InputString = sc.nextLine(); char[] strArray = new char[InputString.length()]; for(i = 0; i < InputString.length(); i++){ strArray[i] = InputString.charAt(i); } for(i = 0 ; i< InputString.length() ; i++) if(strArray[i] >= 'a' && strArray[i] <= 'z' || strArray[i] >= 'A' && strArray[i] <= 'Z') System.out.print(strArray[i]); (the problem line) }}arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
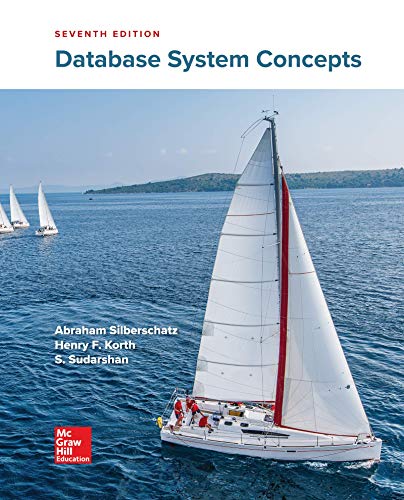
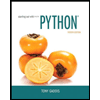
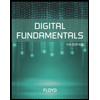
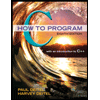
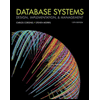
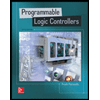