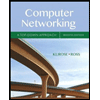
Python help, please have all steps and write down comments
Implement the functions below following the instruction for each problem. No template has been provided for these four problems, but I have included lots of hints. Still testing your wings. Be sure to provide an appropriate docstring for each (Note: the docstring for all four of these problems should cover more than one line) and appropriate comments.
Write a function diffSequence() that takes a list of numbers (int or float) as a parameter and returns True if every trio in the list (from left to right) have the property that the difference of the first one minus the second one equals the third one. The function returns False if there is any trio in the list that does not have this property. For example, diffSequence([12, 8, 4, 4]) would return True because 12 - 8 = 4 and 8 - 4 = 4, but diffSequence([12, 8, 4, 2]) would return False because 12 - 8 = 4 but 8 - 4 != 2. If the list has fewer than three elements, the function returns False. Do not take the absolute value when computing the difference. The function must return a Boolean it does not print a value. A correct solution to this function must use an indexed loop. That is, one that makes a call to the range() function to generate indices into the list which are used to solve the problem (Review lecture notes 8-1). A solution that does not use an indexed loop will not be accepted. Note in particular that you are not allowed to use the index() or find() methods of the list class. Don’t forget to include the docstring and comments. Submissions that don’t include all will lose points. Copy and paste or screen shot the code and the seven test cases shown below in your submission.
How the function should run when running these seven test cases
>>> diffSequence([])
False
>>> diffSequence([47])
False
>>> diffSequence([54,64])
False
>>> diffSequence([12,8,4,4])
True
>>> diffSequence([12,8,4,2])
False
>>> diffSequence([21,13,8,5,3,2,1,1])
True
>>> diffSequence([20,15,5,10,5])
False

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- There are 5 mistakes in the code below, highlighted by the red tilde. Fix each mistake by rewriting the code to the right. Just rewrite the lines that are incorrect. Hint, you might have to change a line that doesn’t have a tilde.arrow_forwardObjectives Practice defining functions with parameters. Practice manipulating strings. Implement your own version of Python string methods The provided file string_functions.py defines several string functions, but does not include the code for them. Add code to implement functions that are equivalent in behavior to the corresponding built-in Python string function. Every function MUST return a value. Use loops to implement your functions. You cannot use built-in string methods, string functions, or string-operators to implement your functions. Each function is worth a maximum of 10 points. 1. cs110_upper: Takes a string as a parameter and returns it as a string in all uppercase. Hint: Use the ord () function to get the ASCI value of a character. For example, ord ('a') returns the integer 97. You will also need to use the chr (value) function that returns a string of one character whose ASCII code is the integer valus. For example, chr (97) returns the string 'a'. You cannot use the…arrow_forwardCan you help me write the code for this MATLAB assignment?arrow_forward
- Add a function to get the CPI values from the user and validate that they are greater than 0. 1. Declare and implement a void function called getCPIValues that takes two float reference parameters for the old_cpi and new_cpi. 2. Move the code that reads in the old_cpi and new_cpi into this function. 3. Add a do-while loop that validates the input, making sure that the old_cpi and new_cpi are valid values. + if there is an input error, print "Error: CPI values must be greater than 0." and try to get data again. 4. Replace the code that was moved with a call to this new function. - Add an array to accumulate the computed inflation rates 1. Declare a constant called MAX_RATES and set it to 20. 2. Declare an array of double values having size MAX_RATES that will be used to accumulate the computed inflation rates. 3. Add code to main that inserts the computed inflation rate into the next position in the array. 4. Be careful to make sure the program does not overflow the array. - Add a…arrow_forwardPython only** define the following function: 1. This function must add a task to a checklist, setting its initial value to False. It will accept two parameters: the checklist object to add to, and the task name to add. In addition to adding the task, it must return the (now modified) checklist object that it was given. There is one issue, however: a task cannot be added to a checklist if the name requested is already being used by another task in that checklist. In that case, this function must print a specific message and must return None Define addTask with 2 parameters Use def to define addTask with 2 parameters Use a return statement Within the definition of addTask with 2 parameters, use return _ in at least one place. Do not use any kind of loop Within the definition of addTask with 2 parameters, do not use any kind of loop.arrow_forwardUse Python for this question. Also, could you please comment/explain what each line of code represents in this question. Write a function piggyBank that accepts a sequence of "coins" and returns counts of the coins and the total value of the bank. the coins will be given by a list of single characters, each one of 'Q','D','N',P' , representing quarter, dime, nickel, penny the function returns a tuple with two items: a dictionary with counts of each denomination. They should always be listed in the order 'Q','D','N',P' the total value in whole cents of the amount in the bank Output for this question is shown in the attached imagearrow_forward
- python only define the following function: This function must reset the value of every task in a checklist to False. It accept just one parameter: the checklist object to reset, and it must return the (now modified) checklist object that it was given. Define resetChecklist with 1 parameter Use def to define resetChecklist with 1 parameter Use a return statement Within the definition of resetChecklist with 1 parameter, use return _in at least one place. Use any kind of loop Within the definition of resetChecklist with 1 parameter, use any kind of loop in at least one place.arrow_forwardI want to know how to write this C++ code with comments to help me understand better.arrow_forwardPlease tell me where I get wrong.arrow_forward
- The input string is provided as a parameter to the compress_string function. The Challenge: You must build a reference to a function that accepts a single integer parameter and returns an array of pointers to functions that accept a single string input and return an integer.Whew! You have to read the specification at least three times before you realise you don't comprehend it.How do you handle something so complicated.arrow_forwardPython question please include all steps and screenshot of code. Also please provide a docstring, and comments throughout the code, and test the given examples below. Thanks. Write a function diceprob() that takes a possible result r of a roll of pair of dice (i.e. aninteger between 2 and 12) and simulates repeated rolls of a pair of dice until 100 rolls of rhave been obtained. Your function should print how many rolls it took to obtain 100 rollsof r.>>> diceprob(2)It took 4007 rolls to get 100 rolls of 2>>> diceprob(3)It took 1762 rolls to get 100 rolls of 3>>> diceprob(4)It took 1058 rolls to get 100 rolls of 4>>> diceprob(5)It took 1075 rolls to get 100 rolls of 5>>> diceprob(6)It took 760 rolls to get 100 rolls of 6>>> diceprob(7)It took 560 rolls to get 100 rolls of 7arrow_forwardPlease use C++ and make sure it's for a sorted array Write a function, removeAll, that takes three parameters: an array of integers,the number of elements in the array, and an integer (say, removeItem). Thefunction should find and delete all of the occurrences of removeItem in thearray. If the value does not exist or the array is empty, output an appropriatemessage. (Note that after deleting the element, the number of elements in thearray is reduced.) Assume that the array is sorted.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
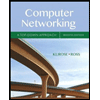
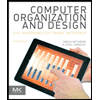
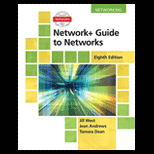
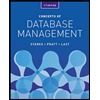
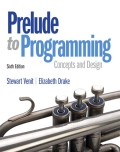
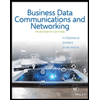