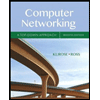
Study the Table class and write a JUnit test class TableTest.java to test the Table class as
follows. Submit the test class and screen captures showing pass of all the tests. Either JUnit4
or JUnit 5 in any IDE may be used.
a. Create a table and put 10 entries of your choice (e.g., testTable.put(1, “testA”)).
Below is the code for pbulic class Table :
public class Table
{
private int manyItems;
private Object[] keys;
private Object[] data;
private boolean[] hasBeenUsed;
public Table(int capacity){
if (capacity <= 0)
throw new IllegalArgumentException("Capacity is negative");
keys=new Object[capacity];
data=new Object[capacity];
hasBeenUsed=new boolean[capacity];
}
public boolean containsKey(Object key){
return findIndex(key)!=-1;
}
public int findIndex(Object key){
int count=0;
int i=hash(key);
while (count <= data.length && hasBeenUsed[i]){
if (key.equals(keys[i]))
return i;
count++;
i=nextIndex(i);
}
return -1;
}
public Object get(Object key){
int index=findIndex(key);
if (index==-1)
return null;
else
return data[index];
}
private int hash(Object key){
return Math.abs(key.hashCode())%data.length;
}
private int nextIndex(int i){
if (i+1 == data.length)
return 0;
else
return i+1;
}
public Object put(Object key, Object element){
int index = findIndex(key);
Object answer;
if (index!=-1){ // The key is already in the table.
answer = data[index];
data[index] = element;
return answer;
}
else if (manyItems<data.length){ // The key is not yet in this Table.
index=hash(key);
while (keys[index]!=null)
index = nextIndex(index);
keys[index] = key;
data[index] = element;
hasBeenUsed[index] = true;
manyItems++;
return null;
}
else{ // The table is full.
throw new IllegalStateException("Table is full.");
}
}
public Object remove(Object key){
int index = findIndex(key);
Object answer = null;
if (index!=-1) // The key exists in the table.
{
answer=data[index];
keys[index]=null;
data[index]=null;
manyItems--;
}
return answer;
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Study the Table class and write a JUnit test class TableTest.java to test the Table class as follows. Submit the test class and screen captures showing pass of all the tests. Either JUnit4 or JUnit 5 in any IDE may be used.
>>Table class code is in previous question
a. Test findIndex() for an non-existing entry.
b. Test findIndex() for an existing entry.
c. Test put() for an non-existing entry.
d. Test put() for an existing entry
e. Test put() for a new entry putting into the full table.
Study the Table class and write a JUnit test class TableTest.java to test the Table class as follows. Submit the test class and screen captures showing pass of all the tests. Either JUnit4 or JUnit 5 in any IDE may be used.
>>Table class code is in previous question
a. Test findIndex() for an non-existing entry.
b. Test findIndex() for an existing entry.
c. Test put() for an non-existing entry.
d. Test put() for an existing entry
e. Test put() for a new entry putting into the full table.
- Update it to create a class Student that includes four properties: an id (type Integer), a name (type String), a Major (type String) and a Grade (type Double). Use class Student to create objects (using buttonAdd) that will be read from the TextFields then save it into an ArrayList. Perform the following queries on the ArrayList of Student objects and show the results on the listViewStudents (Hint: add buttons as needed): d) Use lambdas and streams to calculate the total average of all Students grades, then show the result. e) Use lambdas and streams to group Students by major, then show the results. 1. Ch3HW; import javafx.application.Application; import javafx.fxml.FXMLLoader; import javafx.scene.Parent; import javafx.scene.Scene; import javafx.stage.Stage; public class MainApp extends Application{ @Override publicvoidstart(StageprimaryStage) throwsException { FXMLLoader loader…arrow_forwardWrite the code in java and please dont plagarise and dont copy from other sources or use anything. Do what in the question says. Thank you.arrow_forwardUsing comments within the code itself, can you provide an line by line explanation of the below JavaScript file? The file itself deals with WebGl and if that helps you. Please and thank you JavaScript file: function mat2() { var out = new Array(2); out[0] = new Array(2); out[1] = new Array(2); switch ( arguments.length ) { case 0: out[0][0]=out[3]=1.0; out[1]=out[2]=0.0; break; case 1: if(arguments[0].type == 'mat2') { out[0][0] = arguments[0][0][0]; out[0][1] = arguments[0][0][1]; out[1][0] = arguments[0][1][0]; out[1][1] = arguments[0][1][1]; break; } case 4: out[0][0] = arguments[0]; out[0][1] = arguments[1]; out[1][0] = arguments[2]; out[1][1] = arguments[3]; break; default: throw "mat2: wrong arguments"; } out.type = 'mat2'; return out; } //---------------------------------------------------------------------------- function mat3() { // v = _argumentsToArray( arguments ); var out = new Array(3); out[0] = new Array(3); out[1] = new Array(3); out[2] = new Array(3);…arrow_forward
- wordBag is type BagInterface<String>. What is printed? wordBag.add("apple");wordBag.add("frog");wordBag.add("banana");wordBag.add("frog");wordBag.add("elephant"); System.out.println(wordBag.getFrequencyOf("frog"));arrow_forwardSuppose MyBaseType is an interface. Then MyBaseType* x; is a valid declaration.A. True B. Falsearrow_forwardUpdate it to create a class Student that includes four properties: an id (type Integer), a name (type String), a Major (type String) and a Grade (type Double). Use class Student to create objects (using buttonAdd) that will be read from the TextFields then save it into an ArrayList. Perform the following queries on the ArrayList of Student objects and show the results on the listViewStudents (Hint: add buttons as needed): d) Use lambdas and streams to calculate the total average of all Students grades, then show the result. 1. Ch3HW; import javafx.application.Application; import javafx.fxml.FXMLLoader; import javafx.scene.Parent; import javafx.scene.Scene; import javafx.stage.Stage; public class MainApp extends Application{ @Override publicvoidstart(StageprimaryStage) throwsException { FXMLLoader loader =newFXMLLoader(getClass().getResource("StudentScreen.fxml")); Parent parent = loader.load(); Scene scene =newScene(parent);…arrow_forward
- Execute the following statements over initially empty ArrayList myList one after another (cascade). Explain what was changed after each statement (what did it do?) myList.add("z"); ArrayList content: Your explanation (where this output came from): myList.add(0, "a"); ArrayList content: Your explanation (where this output came from): myList.add("t"); ArrayList content: Your explanation (where this output came from): myList.add(2,"w"); ArrayList content: Your explanation (where this output came from): myList.set(0,"b"); ArrayList content: Your explanation (where this output came from):arrow_forwardHelp create object in swiftarrow_forwardimport bridges.base.ColorGrid;import bridges.base.Color;public class Scene {/* Creates a Scene with a maximum capacity of Marks andwith a background color.maxMarks: the maximum capacity of MarksbackgroundColor: the background color of this Scene*/public Scene(int maxMarks, Color backgroundColor) {}// returns true if the Scene has no room for additional Marksprivate boolean isFull() {return false;}/* Adds a Mark to this Scene. When drawn, the Markwill appear on top of the background and previously added Marksm: the Mark to add*/public void addMark(Mark m) {if (isFull()) throw new IllegalStateException("No room to add more Marks");}/*Helper method: deletes the Mark at an index.If no Marks have been previously deleted, the methoddeletes the ith Mark that was added (0 based).i: the index*/protected void deleteMark(int i) {}/*Deletes all Marks from this Scene thathave a given Colorc: the Color*/public void deleteMarksByColor(Color c) {}/* draws the Marks in this Scene over a background…arrow_forward
- Create a UML diagram to document the Cuboid class. You can model this class after the Rectangle class we covered in this chapter. It should have 3 data fields: width, length, height. It should have 3 accessors and 3 mutators. It should have a method that calculates the volume of the cuboid. Save your file in MS Word or PDF format. Hint: In MS Word, you can create a table of 1 column and 3 rows. What is a cuboid? You can consider a cuboid as a cube whose length, width, and height may not be equal to each other.arrow_forwardExercise #2 Implement an instance method belonging to the IntArrayBag class that takes two input parameters, oldVal and newVal. The method replaces each oldVal element in the array with newVal. Make sure to include the method header.arrow_forwardImplement a graphical application that displays a list of courses. Briefly, this GUI will take the inputs (i.e., course information), store them in an array list, and display course information in the output area. Figure 1 shows the layout ofthe CourseDisplay GUI. You should have three Java files: 1. CourseDisplayFrame class that extendsJFrame class (The main part of your program). 2.CourseDisplayViewer class, which contains the main method where a CourseDisplay object will be created, and the GUI will pop up (Suggested frame width of 450, and frame height of 400). 3.Course class, which is the class that models the Course objects (This file will be given to you). In your CourseDisplayFrame class file, you should have the followings: •Input area: –A text label and a text field (suggested width of 30) for course code; –A text label and a text field (suggested width of 30) for course name; –A text label and a text field (suggested width of 30) for course credit; –A text label and a text…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
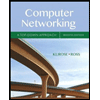
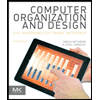
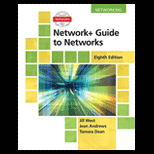
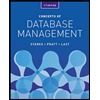
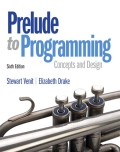
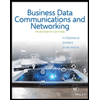