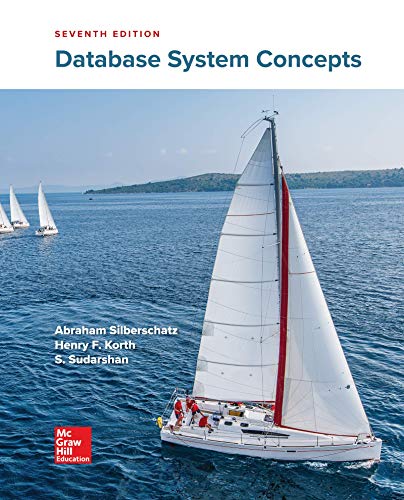
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Task 2: Command-Line Arguments and String Copying
#include <string.h>
#include <stdio.h>
int main(int argc, char* argv[]) {
char str[10];
char *arg1 = argv[1];
int i = 0;
int j = 0;
while (arg1[i] != '\0') {
str[i] = arg1[i];
i++;
}
while (str[j] != '\0') {
printf("%c", str[j]);
j++;
}
return 0;
}
#include <string.h>
#include <stdio.h>
int main(int argc, char* argv[]) {
char str[10];
char *arg1 = argv[1];
int i = 0;
int j = 0;
while (arg1[i] != '\0') {
str[i] = arg1[i];
i++;
}
while (str[j] != '\0') {
printf("%c", str[j]);
j++;
}
return 0;
}
a. Execute the program with 012345678 as the command-line argument. Observe the output.
b. Execute the program with 01. as the command-line argument. Observe the output. Explain what you see and
why.
c. Execute the program with 01234567890123 as the command-line argument. Observe the output. Explain
what you see and why.
d. Fix the condition inside the while loop on line 12 to always print the right amount of characters and never
read past the end of the str array.
b. Execute the program with 01. as the command-line argument. Observe the output. Explain what you see and
why.
c. Execute the program with 01234567890123 as the command-line argument. Observe the output. Explain
what you see and why.
d. Fix the condition inside the while loop on line 12 to always print the right amount of characters and never
read past the end of the str array.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- #include <iostream> #include <string> using namespace std; int main() { // Declare a named constant for array size here // Declare array here // Use this integer variable as your loop index int loopIndex; // Use this variable to store the batting average input by user double battingAverage; // Use these variables to store the minimim and maximum values double min, max; // Use these variables to store the total and the average double total, average; // Write a loop to get batting averages from user and assign to array cout << "Enter a batting average: "; cin >> battingAverage; // Assign value to array // Assign the first element in the array to be the minimum and the maximum min = averages[0]; max = averages[0]; // Start out your total with the value of the first element in the array total = averages[0]; // Write a loop here to access array values starting with…arrow_forward#include <iostream>using namespace std; char* duplicateWithoutBlanks(char *word){int len = sizeof(word); char *new_str = new char[len + 1]; int k = 0; for (int i = 0; i < len; ++i){if (word[i] != ' ')new_str[k++] = word[i];} new_str[k] = '\0'; return new_str;} int main(){// Testing code char word[] = "Hello, World!"; char* result = duplicateWithoutBlanks(word); cout<< "word: "<< word<< "\nResult: "<< result; ; return 0;} What to change to out put the same word without blank?arrow_forward5) Which of the following is a legal way to declare and instantiate an array of 10 Strings?a) String s = new String(10);b) String[10] s = new String;c) String[ ] s = new String[10];d) String s = new String[10];e) String[ ] s = new String;arrow_forward
- 3. int count(10); while(count >= 0) { count -= 2; std::cout << count << endl;arrow_forward23. In the C++ language write a program to find the product of all the elements present in the array of integers given below. int numArr[5] = {16,4, 8, 7, 12};arrow_forwardI need the pseudocode or flowchart for the following code: #include <stdio.h>#include <stdlib.h>#include <string.h>#define array_Length 5#define BUFSIZE 40//Calculate the average int getmean (int *arr, int length) {int sum = 0;for (int i=0; i<length; i++){sum += arr[i];}//end for loopreturn sum/length;}//Output the Maximum int find_Maximum(int *arr,int length) {int max=0;for(int i=1;i<length;++i)if (arr[i]>arr[max])max=i;return arr[max];}//end for loopint main() {//Intialize and Declare Variables int values[array_Length] = {0};char buffer[BUFSIZE];int index = 0;int x = 0;while(x<5) {//Ask user to input integer printf ("Type a number:");x++;if (fgets(buffer, BUFSIZE, stdin) == NULL)break;//Save new int in the array values[index] = atoi(buffer);//Move the index to the next element, wrap around if needed index = (index + 1) % array_Length;//Display the array, maximum number and the average of its valueprintf("[");for (int i= 0; i < array_Length; i++)…arrow_forward
- #include<stdio.h>#include<string.h>struct info{char names[100];int age;float wage;};int main(int argc, char* argv[]) {int line_count = 0;int index, i;struct info hr[10];if (argc != 2)printf("Invalid user_input!\n");else {FILE* contents = fopen (argv[1], "r");struct info in;if (contents != NULL) {printf("File has been opened successfully.\n\n");while (fscanf(contents, "%s %d %f\n",hr[line_count].names, &hr[line_count].age, &hr[line_count].wage) != EOF) {printf("%s %d %f\n", hr[line_count].names, hr[line_count].age, hr[line_count].wage);line_count++;}printf("\nTotal number of lines in document are: %d\n\n", line_count);fclose(contents);printf("Please enter a name: ");char user_input[100];fgets(user_input, 30, stdin);user_input[strlen(user_input)-1] = '\0';index = -1;for(i = 0; i < line_count; i++){if(strcmp(hr[i].names, user_input) == 0){index = i;break;}}if(index == -1)printf("Name %s not found\n", user_input);else{while(fread(&in, sizeof(struct info), 1,…arrow_forward#include using namespace std; void scanPrice (string [], double []); // Do not modify the code double printReceipt(string [], double[]); // Do not modify the code int main () { double sum; string item [3] = {"Vegetable","Egg", "Meat"}; double price [3];| %3D scanPrice (item,price); sum = RrintReceipt (item,price); cout Price: 10 The price of Vegetable is RM 2 The price of Egg is RM 3 The price of Meat is RM 10 Your total expenses is RM 15arrow_forwardhere is a and b part plz solve c part #include <iostream>#include <string.h>#include <stdlib.h>#include <sstream>#include <cmath>using namespace std; int main(){ /*Variable declarations*/int higestDegree,higestDegree1;int diffDegree,smallDegree;std::stringstream polynomial,addpolynomial;/*prompt for degree*/std::cout<<"Please enter higest degree of polynomial"<<std::endl;std::cin>>higestDegree; std::cout<<"Please Enter highest degree of 2nd polynomial"<<std::endl;std::cin>>higestDegree1;int *cofficient=new int[higestDegree+1];//cofficient array creation for 1st polynomailint *cofficient1=new int[higestDegree1+1];//cofficient array creation for 2st polynomail /*taking cofficient for 1st polynomial*/std::cout<<"Please enter cofficient of each term from higest degree to lowest for 1st polynomial"<<std::endl;for(int i=0;i<=higestDegree;i++){std::cin>>cofficient[i];}/*taking cofficient for 2st…arrow_forward
- initial c++ file/starter code: #include <vector>#include <iostream>#include <algorithm> using namespace std; // The puzzle will always have exactly 20 columnsconst int numCols = 20; // Searches the entire puzzle, but may use helper functions to implement logicvoid searchPuzzle(const char puzzle[][numCols], const string wordBank[],vector <string> &discovered, int numRows, int numWords); // Printer function that outputs a vectorvoid printVector(const vector <string> &v);// Example of one potential helper function.// bool searchPuzzleToTheRight(const char puzzle[][numCols], const string &word,// int rowStart, int colStart) int main(){int numRows, numWords; // grab the array row dimension and amount of wordscin >> numRows >> numWords;// declare a 2D arraychar puzzle[numRows][numCols];// TODO: fill the 2D array via input// read the puzzle in from the input file using cin // create a 1D array for wodsstring wordBank[numWords];// TODO:…arrow_forward#include<bits/stdc++.h>using namespace std;bool isPalindrome(string &s){ int start=0; int end=s.length()-1; while(start<=end) { if(s[start]!=s[end]) { return false; } start++; end--; } return true;}int main(){ string s; cout<<"ENTER STRING:"; getline(cin,s); int n=s.length(); bool flag=true; for(int i=1;i<n;i++) { string lowerHalf=s.substr(0,i); string upperHalf=s.substr(i,n-i); if(isPalindrome(lowerHalf) && isPalindrome(upperHalf)) { flag=false; cout<<"String A is:"<<lowerHalf<<"\n"; cout<<"String B is:"<<upperHalf<<"\n"; break; } } if(flag) { cout<<"NO\n"; } return 0;} change to stdio.h string.harrow_forward#include <iostream> #include <string> using namespace std; int main() { // Declare a named constant for array size here // Declare array here // Use this integer variable as your loop index int loopIndex; // Use this variable to store the batting average input by user double battingAverage; // Use these variables to store the minimim and maximum values double min, max; // Use these variables to store the total and the average double total, average; // Write a loop to get batting averages from user and assign to array cout << "Enter a batting average: "; cin >> battingAverage; // Assign value to array // Assign the first element in the array to be the minimum and the maximum min = averages[0]; max = averages[0]; // Start out your total with the value of the first element in the array total = averages[0]; // Write a loop here to access array values starting with…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
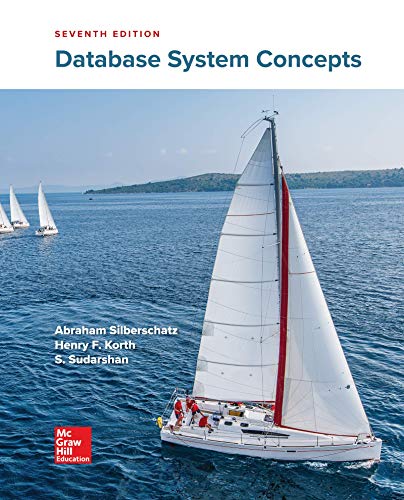
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
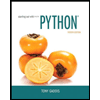
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
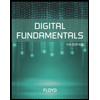
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
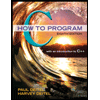
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
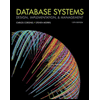
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
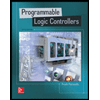
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education