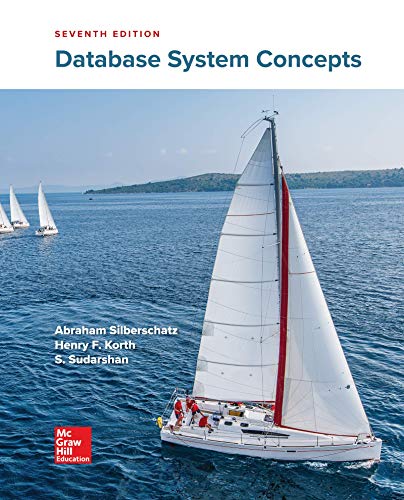
Using this starter code:
#include <stdio.h>
//function prototypes
void PrintLine(int length, char theChar);
void PrintRectangle(int width, int height, char theChar);
void PrintTriangle(int baseLength, char theChar);
void PrintInvertedTriangle(int height, char theChar);
int main(void)
{
char choice;
int a, b;
char character;
//asking for user input
printf("Which shape (L-line, T-triangle, R-rectangle, I-inverted triangle): \n");
scanf(" %c", &choice);
printf("Which character: \n");
scanf(" %c", &character);
switch (choice)
{
//if user input is for a triangle
case 'T':
case 't':
printf("Enter an integer base length between 3 and 25: \n");
scanf("%d", &a);
if (a >= 3 && a <= 25)
PrintTriangle(a, character);
else
printf("Length not in range.");
break;
//if user input is for a rectangle
case 'R':
case 'r':
printf("Enter an integer width and height between 2 and 25: \n");
scanf("%d%d",&a, &b);
if((a<2 || a>25 )){
printf("Width not in range.");
break;
}
if((b<2||b>25)){
printf("Height not in range.");
break;
}
if(a >= 2&&a<=25 && b>=2&&b<=25)
PrintRectangle(a, b, character);
break;
//if user input is a line
case 'L':
case 'l':
printf("Enter an integer length between 1 and 25: \n");
scanf("%d", &a);
if (a >= 1 && a <= 25)
PrintLine(a, character);
else
printf("Length not in range.");
break;
//if user input is an inverted triangle
case 'i':
case 'I':
printf("Enter an integer base length between 2 and 25: \n");
scanf("%d", &a);
if (a >= 2 && a <= 25)
PrintInvertedTriangle(a, character);
else
printf("Height not in range.");
break;
//if user input isn't a line, rectagnle, triangle or inverted triangle
default:
printf("Unknown shape.\n");
break;
}
}
//line function
void PrintLine(int length, char theChar)
{
int i;
for (i = 0; i < length; i++)
printf("%c", theChar);
printf("\n");
}
//triangle function
void PrintTriangle(int baseLength, char theChar)
{
int i, j;
for (i = 1; i <= baseLength; i++)
{
for (j = 0; j < i; j++)
printf("%c", theChar);
printf("\n");
}
}
//rectangle function
void PrintRectangle(int width, int height, char theChar)
{
int i, j;
for (i = 0; i < height; i++)
{
for (j = 0; j < width; j++)
printf("%c", theChar);
printf("\n");
}
}
//inverted triangle function
void PrintInvertedTriangle(int height, char theChar)
{
int i;
for (i = height; i > 0; i--)
{
PrintLine(i, theChar);
}
}
Answer this prompt in C programming:
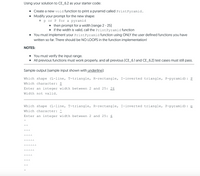

Step by stepSolved in 2 steps

- // // main.c // Assignment1 // // Created by Hassan omer on 15/10/21. // #include <stdio.h> # include <stdlib.h> int input(); int multiples(); int cions(); void display_change(); int main() { int num; num = input(); multiples(num); cions(); display_change(); return 0; } int input(int num) { printf("enter 5-95 number\n"); scanf("%d",&num); return num; } int multiples(int num) { int sum5 =0; if (sum5 %5 != 0 ||sum5<5|| sum5 >95) { printf("invaild input %d",sum5); } cions(sum5); return sum5; } int cions(int sum05){ int cent50 = 0; int cent20 = 0; int cent10 = 0; int cent05 = 0; if (sum05 > 0) { if (sum05 >= 50){ sum05 -= 50; cent50++; } else if (sum05 >=20){ sum05 -= 20; cent20++; } else if (sum05 >= 10){ sum05 -=10;…arrow_forward>> IN C PROGRAMMING LANGUAGE ONLY << COPY OF DEFAULT CODE, ADD SOLUTION INTO CODE IN C #include <stdio.h>#include <stdlib.h>#include <string.h> #include "GVDie.h" int RollSpecificNumber(GVDie die, int num, int goal) {/* Type your code here. */} int main() {GVDie die = InitGVDie(); // Create a GVDie variabledie = SetSeed(15, die); // Set the GVDie variable with seed value 15int num;int goal;int rolls; scanf("%d", &num);scanf("%d", &goal);rolls = RollSpecificNumber(die, num, goal); // Should return the number of rolls to reach total.printf("It took %d rolls to get a \"%d\" %d times.\n", rolls, num, goal); return 0;}arrow_forward// Add to this partially built code. // fill in code where there is a TODO #include<iostream> using namespace std; int main() { cout.setf(ios::fixed); cout.setf(ios::showpoint); // show decimals even if not needed cout.precision(2); // two places to the right of the decimal // TODO: enter the missing types below int time; float ticketPrice; destination; //'C'=Chicago, 'P'=Portland, 'M'=Miami typeOfDay; //'D'=weekDay 'E'=weekEnd cout << "Welcome to Airlines!" << endl; cout << "What is your destination? ([C]hicago, [M]iami, [P]ortland) "; cin >> destination; cout << "What time will you travel? (Enter time between 0-2359) "; cin >> time; // TODO: set isDayTime to true if time 5AM or later, but before 7PM cout << "What type of day are you traveling? (week[E]nd or week[D]ay) "; cin >> typeOfDay; // TODO: set isWeekend to true if typeOfDay is 'E', otherwise false // Depending upon the destination, and whether…arrow_forward
- Language: C++ Also, create two hurricane objects in the main function and print the average wind speed of them. All the data members can be public.arrow_forwardC++ This program does not compile! Spot the error and give the line number. Presume that the header file is error free and // Implementation file for the Rectangle class.1. #include "Rectangle.h" // Needed for the Rectangle class2. #include <iostream> // Needed for cout3. #include <cstdlib> // Needed for the exit function4. using namespace std; //***********************************************************// setWidth sets the value of the member variable width. *//*********************************************************** 5. void Rectangle::setWidth(double w){6. if (w >= 0)7. width = w;8. else{9. cout << "Invalid width\n";10. exit(EXIT_FAILURE);}} //***********************************************************// setLength sets the value of the member variable length. *//*********************************************************** 11. void Rectangle::setLength(double len){12. if (len >= 0)13. length = len;14. else{15. cout << "Invalid length\n";16.…arrow_forward/* Programming concept: structures Program should: be a C program, define a structure to store the year, month, day, and high temperature. write a function get_data that has a parameter that is a pointer to a struct and reads from the user into the struct pointed to by the parameter. write a function print_data that takes the struct pointer as a parameter and prints the data. in main, declare a struct, call the get_data function, call the print_data function. */ #include <stdio.h> int main(int argc, char *argv[]) { return 0;}arrow_forward
- C PROGRAMMING HELP I need help with an old script of mine. I'm having trouble getting math involving accessing an array to work properly, I'm getting an error message that's puzzling me. I'm pretty sure the solution is simple and right in front of me but it's stumping me. Below is the script: #include <stdio.h>#include <math.h>void value(float x){ printf("what is the value of x?"); scanf("%f",&x); }void power(float i,int p,int x,float arr[100]){ float equation,butt; printf("what is the highest power in the series?"); scanf("%d",&p); for (i = 0; i < p; ++i) equation = pow(x,i); //how the hell do i get the counter to do what i want butt = 1+equation^arr[i];}void shortcut(int x){ shortcut = 1/(1-x)}int main(){ printf("Here is the result!:") printf("%d %f", p, f); printf("If we had used shortcut, value would be: %d", shortcut); int diff; diff = shortcut - butt; printf("The difference is only %0.2f!",diff);…arrow_forwardPlease explain this question void main() {int a =300; char *ptr = (char*) &a ; ptr ++; *ptr =2; printf("%d", a); }arrow_forwardC++ Program: #include <iostream>#include <string> using namespace std; const int AIRPORT_COUNT = 12;string airports[AIRPORT_COUNT] = {"DAL","ABQ","DEN","MSY","HOU","SAT","CRP","MID","OKC","OMA","MDW","LAX"}; int main(){ // define stack (or queue ) here string origin; string dest; string citypair; cout << "Loading the CONTAINER ..." << endl; // LOAD THE STACK ( or queue) HERE // Create all the possible Airport combinations that could exist from the list provided. // i.e DALABQ, DALDEN, ...., ABQDAL, ABQDEN ... // DO NOT Load SameSame - DALDAL, ABQABQ, etc .. cout << "Getting data from the CONTAINER ..." << endl;// Retrieve data from the STACK/QUEUE here } Using the attached program (AirportCombos.cpp), create a list of strings to process and place on a STL STACK container. The provided code is meant to be generic. Using the provided 3 char airport codes, create a 6 character string that is the origin &…arrow_forward
- This assignment is not graded, I just need to understand how to do it. Please help, thank you! Language: C++ Given: Main.cpp #include #include "Shape.h" using namespace std; void main() { /////// Untouchable Block #1 ////////// Shape* shape; /////// End of Untouchable Block #1 ////////// /////// Untouchable Block #2 ////////// if (shape == nullptr) { cout << "What shape is this?! Good bye!"; return; } cout << "The perimeter of your " << shape->getShapeName() << ": " << shape->getPerimeter() << endl; cout << "The area of your " << shape->getShapeName() << ": " << shape->getArea() << endl; /////// End of Untouchable Block #2 //////////} Shape.cpp string Shape::getShapeName() { switch (mShapeType) { case ShapeType::CIRCLE: return "circle"; case ShapeType::SQUARE: return "square"; case ShapeType::RECTANGLE: return "rectangle"; case…arrow_forwardDescribe how to pass an array as a parameter to a function.arrow_forwardC++ printSmaller is a function that accepts two int parameters and returns no value. It will print the value of the smaller one parameters. The function protoype is as follows: void printSmaller(int num1, int num2); write the statments to read two integers and call this function to display the smaller one.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
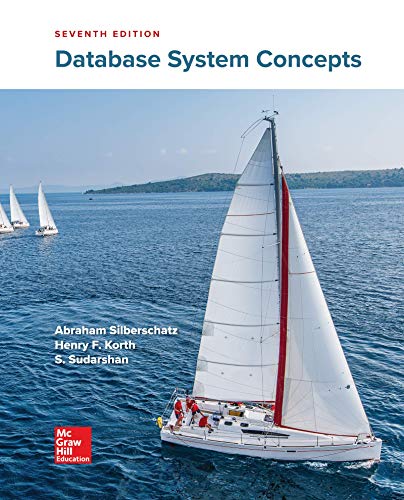
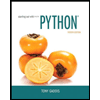
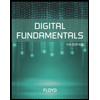
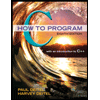
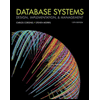
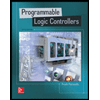