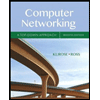
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Write a function, slens, that borrows a
HashMap that maps each string to its length.
fn slens(stuff: &Vec<String>) -> HashMap<String, usize> {
...
}
fn main() {
let stuff = vec![
String::from("A"),
String::from("fine"),
String::from("mess"),
];
println!("{:?}", slens(&stuff));
println!("{:?}", stuff);
}
/* Output:
{"fine": 4, "mess": 4, "A": 1}
["A", "fine", "mess"]
*/
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

Knowledge Booster
Similar questions
- For example: Binary value of character ‘B’ from ASCII table is 01000010Dataword is 1000010 Even Parity Bit is 0 Codeword: 10000100 Even Parity – Complete the following table using Even Parity Character Binary Datawordfrom ASCII table(7-bit value) Parity BitValue TransmittedCodeword ReceivedCodewordat Receiver Check ifthecodewordisrejected t 1010100 10101101 h 1101000 11010000 i 1101001 11010010 s 1110011 11100110arrow_forwardGiven the following function and a Linked List : head->a->b->c->d->e->f->g->null, where a,...g are void fun1(Node head) { if(head == null) return; System.out.printf("%d ", head.data); fun1(head.link.link); } What does the call fun1(head) do? Binary search tree properties.arrow_forwardWrite a function named addStars that accepts as a parameter a reference to a vector of strings, and modifies the vector by placing a "*" element between elements, as well as at the start and end of the vector. For example, if the vector v contains {"the", "quick", "brown", "fox"}, the call of addStars(v); should modify it to store {"*", "the", "*", "quick", "*", "brown", "*", "fox", "*"}.arrow_forward
- In Ocaml Map functions left Write a function map_fun_left : (’a -> ’a) list -> ’a list -> ’a list = that is given a list of functions and a list of elements. For each element apply all functions from left to it and keep the result. Return the results as a list. examplesmap_fun_left [((+) 1) ;( * ) 2; fun x - >x -10] [1;2;3;4];;- : int list = [ -6; -4; -2; 0]map_fun_left [( fun x - > int_of_char x | > (+) 32 | >char_of_int ) ;( fun x - > char_of_int (( int_of_char x )+1) ) ] [ ’A ’; ’B ’; ’C ’; ’D ’];;- : char list = [ ’b ’; ’c ’; ’d ’; ’e ’]arrow_forwardCode to implement a command-line search auto complete interface won't work, whats the problem? #include <iostream> #include <fstream> #include <string> #include <vector> const int ALPHABET_SIZE = 28; class Trie { private: structTrieNode { //Node struct which contains a a child node for each letter of the alphabet TrieNode *children[ALPHABET_SIZE]; boolisEndOfWord;//bool to determine if letter is the last in a word TrieNode() {//constructor to initially set every child node to empty, and bool to false for (int i = 0; i < ALPHABET_SIZE; i++) { children[i] = NULL; } isEndOfWord = false; } ~TrieNode() {//deconstructor for (int i = 0; i < ALPHABET_SIZE; i++) { if (children[i] != NULL) { deletechildren[i]; } } } }; TrieNode *root;//pointer to point to first letter in word public: Trie() { root = newTrieNode(); //new node is created for first letter inserted } ~Trie() { deleteroot; } //this function inserts every word in the dictionary to the…arrow_forwardIn C++ /** * Performs an index-based bubble sort on any indexable container object. * * @tparam IndexedContainer must support `operator[]` and `size()`, e.g. * `std::vector`. Container * elements must support `operator<` and `operator=`. * * @param values the object to sort */ template <typename IndexedContainer> void bubble_sort(IndexedContainer &values){ //TODO } /** * Sorts the elements in the range `[first,last)` into ascending order, using * the bubble-sort * algorithm. The elements are compared using `operator<`. * * @tparam Iterator a position iterator that supports the [standard * bidirectional iterator * operations](http://www.cplusplus.com/reference/iterator/BidirectionalIterator/) * * @param first the initial position in the sequence to be sorted * @param last one element past the final position in the sequence to be sorted */ template <typename Iterator> void bubble_sort(Iterator first, Iterator last) { //TODO }arrow_forward
- In AList class, we have the get function to return an item at a particular position in list indexed by i. For example, let L=[1,2,3]. A call to L.get (1) will return 2. Write in the answer an enhanced get function to accept negative indices. Specifically, the last item has index -1, the second last item has index -2, and the i-th last item has index -i. For example, let L=[1,2,31. A call to L.get (-1) will return 3. A call to L.get (-2) will return 2. A call to L.get (-3) will return 1. Below is a skeleton of the AList class: template class AList { private: /** The underlying array. */ ItemType *items; /** Stores the current size of the list. */ int count; /** Max number of items allowed. */ int maxCnt; public: // Other functions omitted... ItemType get (int i) { // Copy this function in the answer and write code below this line. } ;arrow_forwardImplement a __setitem__ function that also supports negative indices. For example: W = L2(Node(10, Node(20, Node(30))))print W[ 10, 20, 30 ] W[1]=25print W[ 10, 25, 30 ] W[-1]=35print W[ 10, 25, 35 ] Complete the code: def L2(*args,**kwargs): class L2_class(L): def __getitem__(self, idx): <... YOUR CODE HERE ...> def __setitem__(self, idx, value): <... YOUR CODE HERE ...> return L2_class(*args,**kwargs) W = L2(Node(10, Node(20, Node(30))))print(W)W[1]=25print(W)W[-1]=35print(W)arrow_forwardWrite a template function that takes as parameter a vector of a generic type and reverses the order of elements in the vector, and then add the function to the program you wrote for Programming Challenge 5. Modify the driver program to test the new function by reversing and outputting vectors whose element types are char, int, double, and string.arrow_forward
- void func(vector<string>& names){ sort(names.begin(), names.end()); //(a) for(int i = names.size() - 1; i > 0; --i){. //(b) if(names[i] == names[i - 1]){ names[i] = move(names.back()); names.pop_back(); } } } What are the reasons or consequences for the programmer’s choice to loop backwards in the line marked (b), and under what circumstances would the algorithm behave differently if that line were replaced with: for (int i = 1; i < names.size(); ++i) ? What would change?arrow_forwardPut a checkmark in the column if the address for that row falls on the boundary type for the column.arrow_forwardfast please c++ Insert the elements of A in hash table H of size 10. H is a vector of int is size 10. Do not allow duplicates. Draw the table. The hash function is the number itself; f(n) = n%10arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
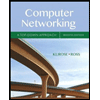
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
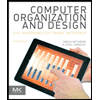
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
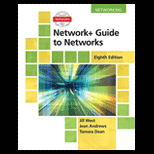
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
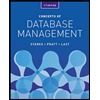
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
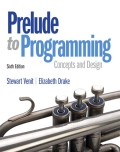
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
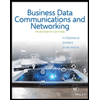
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY